Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/TooTallNate/plist.js
Mac OS X Plist parser/builder for Node.js and browsers
https://github.com/TooTallNate/plist.js
Last synced: about 1 month ago
JSON representation
Mac OS X Plist parser/builder for Node.js and browsers
- Host: GitHub
- URL: https://github.com/TooTallNate/plist.js
- Owner: TooTallNate
- License: mit
- Created: 2010-08-03T08:48:50.000Z (almost 14 years ago)
- Default Branch: master
- Last Pushed: 2024-03-12T12:22:42.000Z (4 months ago)
- Last Synced: 2024-05-28T13:37:07.402Z (about 1 month ago)
- Language: JavaScript
- Homepage:
- Size: 1.88 MB
- Stars: 526
- Watchers: 12
- Forks: 123
- Open Issues: 26
-
Metadata Files:
- Readme: README.md
- Changelog: History.md
- License: LICENSE
Lists
- awesome-stars - plist.js
- awesome-stars - plist.js
- awesome-nodejs-pure-js - plist
README
plist.js
========
### Apple's Property list parser/builder for Node.js and browsers[](https://github.com/TooTallNate/plist.js/actions/workflows/ci.yml)
Provides facilities for reading and writing Plist (property list) files.
These are often used in programming OS X and iOS applications, as well
as the iTunes configuration XML file.Plist files represent stored programming "object"s. They are very similar
to JSON. A valid Plist file is representable as a native JavaScript Object
and vice-versa.## Usage
### Node.js
Install using `npm`:
``` bash
$ npm install --save plist
```Then `require()` the _plist_ module in your file:
``` js
var plist = require('plist');// now use the `parse()` and `build()` functions
var val = plist.parse('Hello World!');
console.log(val); // "Hello World!"
```### Browser
Include the `dist/plist.js` in a `` tag in your HTML file:
``` html
<script src="plist.js">// now use the `parse()` and `build()` functions
var val = plist.parse('<plist><string>Hello World!</string></plist>');
console.log(val); // "Hello World!"```
## API
### Parsing
Parsing a plist from filename:
``` javascript
var fs = require('fs');
var plist = require('plist');var obj = plist.parse(fs.readFileSync('myPlist.plist', 'utf8'));
console.log(JSON.stringify(obj));
```Parsing a plist from string payload:
``` javascript
var plist = require('plist');var xml =
'' +
'' +
'' +
'metadata' +
'' +
'bundle-identifier' +
'com.company.app' +
'bundle-version' +
'0.1.1' +
'kind' +
'software' +
'title' +
'AppName' +
'' +
'';console.log(plist.parse(xml));
// [
// "metadata",
// {
// "bundle-identifier": "com.company.app",
// "bundle-version": "0.1.1",
// "kind": "software",
// "title": "AppName"
// }
// ]
```### Building
Given an existing JavaScript Object, you can turn it into an XML document
that complies with the plist DTD:``` javascript
var plist = require('plist');var json = [
"metadata",
{
"bundle-identifier": "com.company.app",
"bundle-version": "0.1.1",
"kind": "software",
"title": "AppName"
}
];console.log(plist.build(json));
//
//
//
// metadata
//
// bundle-identifier
// com.company.app
// bundle-version
// 0.1.1
// kind
// software
// title
// AppName
//
//
```## Cross Platform Testing Credits
Much thanks to Sauce Labs for providing free resources that enable cross-browser testing on this project!
[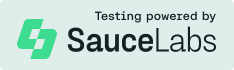](https://saucelabs.com)
## License
[(The MIT License)](LICENSE)