Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/skydoves/Submarine
:speedboat: Floating navigation view for displaying a list of items dynamically on Android.
https://github.com/skydoves/Submarine
android android-library animation dsl kotlin navigation skydoves submarine
Last synced: 3 months ago
JSON representation
:speedboat: Floating navigation view for displaying a list of items dynamically on Android.
- Host: GitHub
- URL: https://github.com/skydoves/Submarine
- Owner: skydoves
- License: apache-2.0
- Created: 2019-06-28T13:20:27.000Z (almost 5 years ago)
- Default Branch: master
- Last Pushed: 2022-01-21T11:07:59.000Z (over 2 years ago)
- Last Synced: 2023-10-20T23:09:31.200Z (8 months ago)
- Topics: android, android-library, animation, dsl, kotlin, navigation, skydoves, submarine
- Language: Kotlin
- Homepage:
- Size: 913 KB
- Stars: 470
- Watchers: 10
- Forks: 41
- Open Issues: 1
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
- License: LICENSE
- Codeowners: .github/CODEOWNERS
Lists
- awesome-list - skydoves/Submarine - :speedboat: Floating navigation view for displaying a list of items dynamically on Android. (Kotlin)
README
Submarine
Fully customizable floating navigation view for listing items dynamically on Android.
![]()
![]()
![]()
## Including in your project
[](https://search.maven.org/search?q=g:%22com.github.skydoves%22%20AND%20a:%22submarine%22)### Gradle
Add the codes below to your **root** `build.gradle` file (not your module build.gradle file).
```gradle
allprojects {
repositories {
mavenCentral()
}
}
```
And add the dependency below to your **module**'s `build.gradle` file.
```gradle
dependencies {
implementation "com.github.skydoves:submarine:1.0.7"
}
```## SNAPSHOT
[](https://oss.sonatype.org/content/repositories/snapshots/com/github/skydoves/submarine/)
Snapshots of the current development version of submarine are available, which track [the latest versions](https://oss.sonatype.org/content/repositories/snapshots/com/github/skydoves/submarine/).```gradle
repositories {
maven { url 'https://oss.sonatype.org/content/repositories/snapshots/' }
}
```## Usage
Add following XML namespace inside your XML layout file.```gradle
xmlns:app="http://schemas.android.com/apk/res-auto"
```### SubmarineView
Here is how to implement like previews using `SubmarineView`.
```gradle```
### Float and Dip
Shows and makes disappear using the below methods.
```kotlin
submarineView.floats() // shows
submarineView.dips() // makes disappear
```### Navigate and Retreat
We can make the SubmarineView always floating (without appear, disappear actions) and navigating when we want.Firstly, add below attributes to SubmarineView on your xml file.
```gradle
app:submarine_autoDip="false"
app:submarine_autoNavigate="false"
```
And call `floats()` method before using `navigates()` method.
```kotlin
submarineView.floats()
```
Unfold and fold using the below methods.
```kotlin
submarineView.navigates() // unfold
submarineView.retreats() // fold
```### SubmarineItem
SubmarineItem is a data class for composing navigation list.
```kotlin
val item = SubmarineItem(ContextCompat.getDrawable(this, R.drawable.ic_edit))
submarineView.addSubmarineItem(item)
```
We can customize the icon drawable using `IconForm.Builder`.
```kotlin
val iconForm = IconForm.Builder(context)
.setIconSize(45)
.setIconTint(ContextCompat.getColor(context, R.color.colorPrimary))
.setIconScaleType(ImageView.ScaleType.CENTER)
.build()// kotlin dsl
val iconForm = iconForm(context) {
iconSize = 45
iconColor = ContextCompat.getColor(context, R.color.colorPrimary)
iconScaleType = ImageView.ScaleType.CENTER
}val item = SubmarineItem(ContextCompat.getDrawable(this, R.drawable.ic_edit), iconForm)
submarineView.addSubmarineItem(item)
```
We can add `SubmarineItem` list, remove and clear all items.
```kotlin
submarineView.addSubmarineItem(item) // adds a SubmarineItem item.
submarineView.addSubmarineItems(itemList) // adds a SubmarineItem item list.
submarineView.removeSubmarineItem(item) // removes a SubmarineItem item.
submarineView.clearAllSubmarineItems() // clears all SubmarineItem items.
```### SubmarineItemClickListener
Interface definition for a callback to be invoked when a item is clicked.
```kotlin
submarineView.submarineItemClickListener = onItemClickListenerprivate object onItemClickListener: SubmarineItemClickListener {
override fun onItemClick(position: Int, submarineItem: SubmarineItem) {
// doSomething
}
}
```### Circle Icon
We can customize the circle icon on the navigation.
Submarine uses [CircleImageView](https://github.com/hdodenhof/CircleImageView). So you can reference the usage of it.
```kotlin
submarineView.circleIcon.setImageDrawable(drawable) // gets circle icon and changes image drawable.
submarineView.circleIcon.borderColor = ContextCompat.getColor(context, R.color.colorPrimary) // gets circle icon and changes border color.
submarineView.circleIcon.borderWidth = 2 // gets circle icon and changes border width.
```### SubmarineCircleClickListener
Interface definition for a callback to be invoked when a circle icon is clicked.
```kotlin
submarineView.submarineCircleClickListener = onCircleIconClickListenerprivate object onCircleIconClickListener: SubmarineCircleClickListener {
override fun onCircleClick() {
// doSomething
}
}
```### Orientation and Anchor
`SubmarineOrientation` and `CircleAnchor` decide the where to spreads and where to moves.
```gradle
app:submarine_circleAnchor="right" // left, right, top, bottom
app:submarine_orientation="horizontal" // horizontal, vertical
```
Horizontal and Left | Horizontal and Right | Vertical and Top | Vertical and Bottom |
| :---------------: | :---------------: | :---------------: | :---------------: |
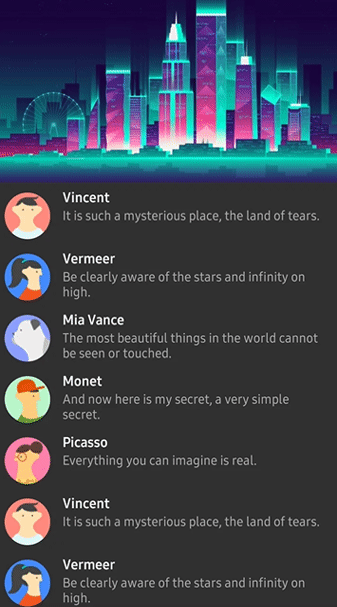| 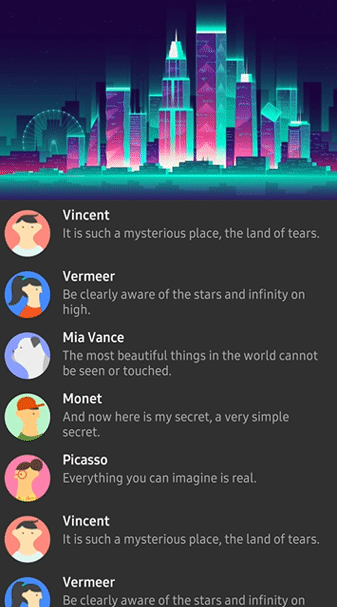 | 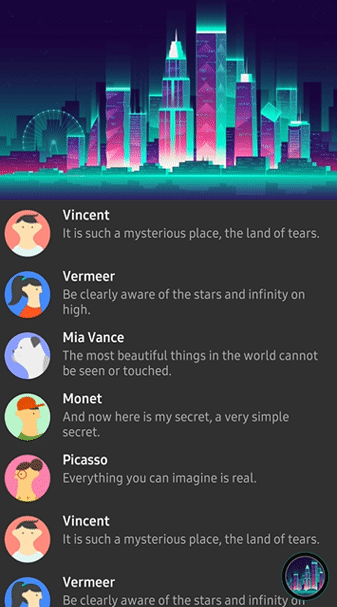| 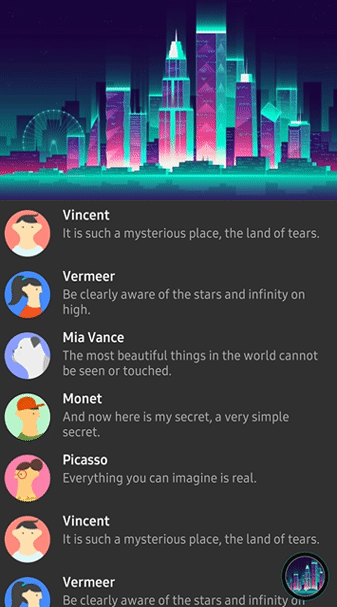### SubmarineAnimation
SubmarineAnimation decides the animation of `float` and `dip` methods.
```gradle
app:submarine_animation="scale" // scale, fade, none
```
SCALE | FADE
------------ | -------------
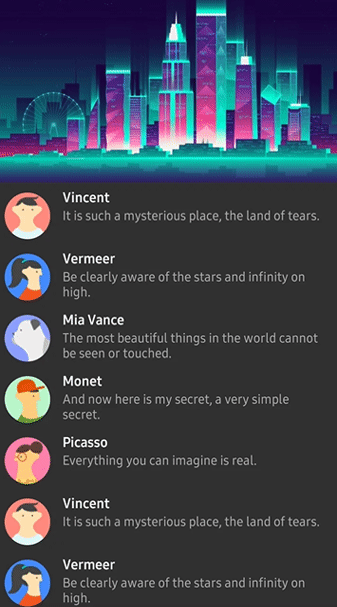| 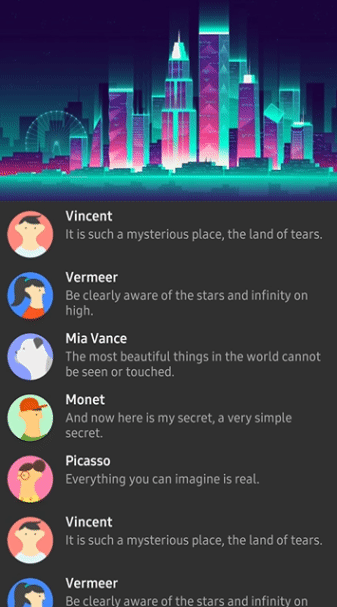## SubmarineView Attributes
Attributes | Type | Default | Description
--- | --- | --- | ---
orientation | SubmarineOrientation | Horizontal | navigation's spreading orientation.
duration | Long | 350L | navigation's spreading duration.
color | Int(Color) | Color.Black | navigation's background color.
animation | SubmarineAnimation | None | floating, dipping animation style.
borderSize | Dimension | 0f | navigation's border thickness.
borderColor | Int(Color) | Color.Green | navigation's border color.
expandSize | Dimension | display width size - 20dp size | navigation's width or height size after expands.
radius | Dimension | 15dp | navigation's corder radius.
autoNavigate | Boolean | true | executes `navigate` method automatically after executing `float` method.
autoDip | Boolean | true | executes `dip` method automatically after executing `retreat` method.
circleAnchor | CircleAnchor | Left | decides where to moves the circle icon.
circleSize | Dimension | 20dp | circle icon's size. It decides the height or width of navigation.
circleDrawable | Drawable | null | circle icon's image drawable.
circleBorderSize | Dimension | 0f | circle icon's border thickness.
circleBorderColor | Int(Color) | 0f | circle icon's border color.
circlePadding | Dimension | 0f | circle icon's padding width.## Find this library useful? :heart:
Support it by joining __[stargazers](https://github.com/skydoves/submarine/stargazers)__ for this repository. :star:# License
```xml
Copyright 2019 skydoves (Jaewoong Eum)Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License athttp://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```