Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/jheinen/GR.jl
Plotting for Julia based on GR, a framework for visualisation applications
https://github.com/jheinen/GR.jl
Last synced: 2 months ago
JSON representation
Plotting for Julia based on GR, a framework for visualisation applications
- Host: GitHub
- URL: https://github.com/jheinen/GR.jl
- Owner: jheinen
- License: other
- Created: 2015-01-13T14:31:54.000Z (over 9 years ago)
- Default Branch: master
- Last Pushed: 2024-03-21T09:56:47.000Z (3 months ago)
- Last Synced: 2024-04-08T02:14:52.320Z (3 months ago)
- Language: Julia
- Homepage:
- Size: 21 MB
- Stars: 352
- Watchers: 12
- Forks: 75
- Open Issues: 173
-
Metadata Files:
- Readme: README.md
- License: LICENSE.md
Lists
- awesome-julia - Plotting for Julia based on GR, a framework for visualisation applications
README
# The GR module for Julia
[](LICENSE.md)
[](https://github.com/jheinen/GR.jl/releases)
[](https://pkgs.genieframework.com?packages=GR)
[](https://zenodo.org/badge/latestdoi/29193648)
[](https://mybinder.org/v2/gh/jheinen/GR.jl/master)
[](https://gitter.im/jheinen/GR.jl?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)[](https://juliaci.github.io/NanosoldierReports/pkgeval_badges/G/GR.html)
[](https://github.com/jheinen/GR.jl/actions/workflows/ci.yml)
[](https://app.codecov.io/gh/jheinen/GR.jl)[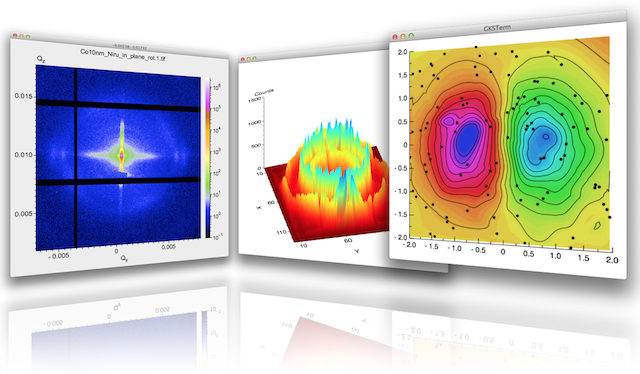](https://gr-framework.org)
This module provides a Julia interface to
[GR](http://gr-framework.org/), a framework for
visualisation applications.## Installation
From the Julia REPL an up to date version can be installed with:
```julia
julia> using Pkg
julia> Pkg.add("GR")
```
or in the [Pkg REPL-mode](https://docs.julialang.org/en/v1/stdlib/Pkg/index.html#Getting-Started-1):
```julia
pkg> add GR
```
The Julia package manager will download and install a pre-compiled
run-time (for your hardware architecture), if the GR software is not
already installed in the recommended locations.## Getting started
In Julia simply type ``using GR`` and begin calling functions
in the [GR framework](http://gr-framework.org/julia-gr.html) API.Let's start with a simple example. We generate 10,000 random numbers and
create a histogram. The histogram function automatically chooses an appropriate
number of bins to cover the range of values in x and show the shape of the
underlying distribution.```julia
using GR
histogram(randn(10000))
```## Using GR as backend for Plots.jl
``Plots`` is a powerful wrapper around other Julia visualization
"backends", where ``GR`` seems to be one of the favorite ones.
To get an impression how complex visualizations may become
easier with [Plots](https://juliaplots.github.io), take a look at
[these](https://docs.juliaplots.org/stable/gallery/gr/) examples.``Plots`` is great on its own, but the real power comes from the ecosystem surrounding it. You can find more information
[here](https://docs.juliaplots.org/latest/ecosystem/).## Alternatives
Besides ``GR`` and ``Plots`` there is a nice package called [GRUtils](https://github.com/heliosdrm/GRUtils.jl) which provides a user-friendly interface to the low-level ``GR`` subsytem, but in a more "Julian" and modular style. Newcomers are recommended to use this package. A detailed documentation can be found [here](https://heliosdrm.github.io/GRUtils.jl/stable/).
``GR`` and ``GRUtils`` are currently still being developed in parallel - but there are plans to merge the two modules in the future.
## Run-time environment
``GR.jl`` is a wrapper for the ``GR`` Framework. Therefore, the ``GR`` run-time libraries are required to use the software. These are provided via the [GR_jll.jl](https://github.com/JuliaBinaryWrappers/GR_jll.jl) package, which is an autogenerated package constructed using [BinaryBuilder](https://github.com/JuliaPackaging/BinaryBuilder.jl). This is the default setting.
Another alternative is the use of binaries from GR tarballs, which are provided directly by the GR developers as stand-alone distributions for selected platforms - regardless of the programming language. In this case, only one GR runtime environment is required for different language environments (Julia, Python, C/C++), whose installation path can be specified by the environment variable `GRDIR`.
```julia
ENV["JULIA_DEBUG"] = "GR" # Turn on debug statements for the GR package
ENV["GRDIR"] = "" # e.g. "/usr/local/gr"
using GR
```For more information about setting up a local GR installation, see the [GR Framework](https://gr-framework.org) website.
However, if you want to permanently use your own GR run-time, you have to set the environment variable ``GRDIR`` accordingly before starting Julia, e.g.
- macOS or Linux: ```export GRDIR=/usr/local/gr```
- Windows: ```set GRDIR=C:\gr```Please note that with the method shown here, `GR_jll` is not imported.
### Switching binaries via GR.GRPreferences
To aid in switching between BinaryBuilder and upstream framework binaries, the `GR.GRPReferences` module implements three methods `use_system_binary()`, `use_upstream_binary()`, and `use_jll_binary()`. These use [Preferences.jl](https://github.com/JuliaPackaging/Preferences.jl) to configure GR.jl
and GR_jll.jl.To use an existing GR install, invoke `use_system_binary`.
```julia
using GR
GR.GRPreferences.use_system_binary("/path/to/gr"; force = true)
```To download and switch to [upstream binaries](https://github.com/sciapp/gr/releases) invoke `use_upstream_binary`.
```julia
using GR # repeat this if there is an error
GR.GRPreferences.use_upstream_binary(; force = true)
````use_system_binary` and `use_upstream_binary` accept an `override` keyword. This may be set to one of the following:
* `:depot` (default) - Use Overrides.toml in the Julia depot. This normally resides in `.julia/artifacts/Overrides.toml`
* `:project` - Use LocalPreferences.toml. This is usually located near the Project.toml of your active project environment.
* `(:depot, :project)` - Use both of the override mechanisms above.To switch back to BinaryBuilder binaries supplied with [GR_jll](https://github.com/JuliaBinaryWrappers/GR_jll.jl), invoke `use_jll_binary`:
```julia
using GR # repeat this if there is an error
GR.GRPreferences.use_jll_binary(; force = true)
```This will reset both the `:depot` and `:project` override mechanisms above.
If you encounter difficulties switching between binaries, the `diagnostics()` function will provide useful information.
Please include this information when asking for assistance.```julia
using GR
GR.GRPreferences.diagnostics()
```