Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/gusgard/react-native-swiper-flatlist
👆 Swiper component implemented with FlatList using Hooks & Typescript + strict automation tests with Detox
https://github.com/gusgard/react-native-swiper-flatlist
bitrise carousel detox e2e-tests expo flatlist hooks images react react-native react-native-swiper react-native-swiper-flatlist scroll scrollview slider swipe swiper swiper-flatlist swipeview typescript
Last synced: about 1 month ago
JSON representation
👆 Swiper component implemented with FlatList using Hooks & Typescript + strict automation tests with Detox
- Host: GitHub
- URL: https://github.com/gusgard/react-native-swiper-flatlist
- Owner: gusgard
- License: apache-2.0
- Created: 2017-11-19T21:58:25.000Z (over 6 years ago)
- Default Branch: master
- Last Pushed: 2024-04-23T03:25:25.000Z (about 1 month ago)
- Last Synced: 2024-04-24T01:29:19.245Z (about 1 month ago)
- Topics: bitrise, carousel, detox, e2e-tests, expo, flatlist, hooks, images, react, react-native, react-native-swiper, react-native-swiper-flatlist, scroll, scrollview, slider, swipe, swiper, swiper-flatlist, swipeview, typescript
- Language: TypeScript
- Homepage:
- Size: 480 MB
- Stars: 496
- Watchers: 11
- Forks: 93
- Open Issues: 6
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Lists
- awesome-react-native - react-native-swiper-flatlist ★★ ★81 - 👆 React Native Swiper component implemented with FlatList (Components / Navigation)
- awesome - react-native-swiper-flatlist ★★ ★81 - 👆 React Native Swiper component implemented with FlatList (Components / Navigation)
- awesome-react-native - react-native-swiper-flatlist ★★ ★81 - 👆 React Native Swiper component implemented with FlatList (Components / Navigation)
- awesome-react-native - react-native-swiper-flatlist ★★ - 👆 React Native Swiper component implemented with FlatList (Components / Navigation)
- awesome-react-native - react-native-swiper-flatlist ★★ - 👆 React Native Swiper component implemented with FlatList (Components / Navigation)
- awesome-react-native - react-native-swiper-flatlist ★★ ★81 - 👆 React Native Swiper component implemented with FlatList (Components / Navigation)
- awesome-react-native - react-native-swiper-flatlist ★★ ★81 - 👆 React Native Swiper component implemented with FlatList (Components / Navigation)
- awesome-react-native - react-native-swiper-flatlist ★★ ★81 - 👆 React Native Swiper component implemented with FlatList (Components / Navigation)
README
# :point_up_2: Swiper FlatList component
[](https://www.npmjs.com/package/react-native-swiper-flatlist)
[](https://www.npmjs.com/package/react-native-swiper-flatlist)
[](https://www.npmjs.com/package/react-native-swiper-flatlist)
[](https://www.npmjs.com/package/react-native-swiper-flatlist)
[](https://www.npmjs.com/package/react-native-swiper-flatlist)
[](https://app.bitrise.io/app/dfeb47a453df37dd)
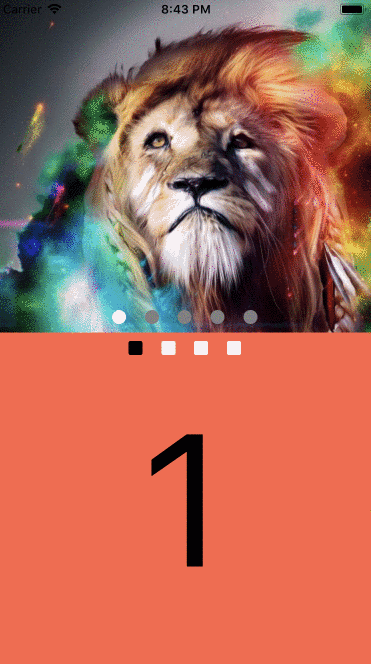
## Installation
```
yarn add react-native-swiper-flatlist
```or
```
npm install react-native-swiper-flatlist --save
```## Notice
Version 2.x was re-implemented using React Hooks so it only works with version 0.59 or above
Version 3.x was re-implemented using Typescript and it works with react-native-web
| react-native-swiper-flatlist | react-native | Detox tests |
| ---------------------------- | ------------ | ----------------------------------------------------------------------------------------------------------------------------------------------------------------- |
| 1.x | <= 0.58 | X |
| 2.x | >= 0.59 | X |
| 3.x | >= 0.59 | [](https://app.bitrise.io/app/dfeb47a453df37dd) |Note: we are using the feature `export type` available in `babel v7.9.0` https://github.com/babel/babel/pull/11171, you might have this issue with React Native between 0.60 and 0.63, please update `babel` to at least to `v7.9.0`
## Examples
[Expo example with renderItems, children and more](https://snack.expo.io/@gusgard/react-native-swiper-flatlist)
[Expo example with children](https://snack.expo.io/@gusgard/react-native-swiper-flatlist-show-next-screen)
[React Native example with renderItems and custom pagination](./example/src/SwiperWithRenderItems.tsx)
[React Native example with children](./example/src/SwiperWithChildren.tsx)
### Code
Using `renderItems` and `data` [](https://snack.expo.io/@gusgard/react-native-swiper-flatlist-simple-with-renderitems-and-data)
```jsx
import React from 'react';
import { Text, Dimensions, StyleSheet, View } from 'react-native';
import { SwiperFlatList } from 'react-native-swiper-flatlist';const colors = ['tomato', 'thistle', 'skyblue', 'teal'];
const App = () => (
(
{item}
)}
/>
);const { width } = Dimensions.get('window');
const styles = StyleSheet.create({
container: { flex: 1, backgroundColor: 'white' },
child: { width, justifyContent: 'center' },
text: { fontSize: width * 0.5, textAlign: 'center' },
});export default App;
```Using `children` [](https://snack.expo.io/@gusgard/react-native-swiper-flatlist-simple-with-children)
```jsx
import React from 'react';
import { Text, Dimensions, StyleSheet, View } from 'react-native';
import { SwiperFlatList } from 'react-native-swiper-flatlist';const App = () => (
1
2
3
4
);const { width } = Dimensions.get('window');
const styles = StyleSheet.create({
container: { flex: 1, backgroundColor: 'white' },
child: { width, justifyContent: 'center' },
text: { fontSize: width * 0.5, textAlign: 'center' },
});export default App;
```### Example project with Detox tests
[Code example](./example/README.md)
To use `react-native-gesture-handler` instead of FlatList import the library like:
```tsx
import { SwiperFlatListWithGestureHandler } from 'react-native-swiper-flatlist/WithGestureHandler';
```## Props
| Prop | Default | Type | Description |
| :-------------------------- | :------------------------------------------------: | :-------------------------------------: | :------------------------------------------------------------------------------------ |
| data | _not required if children is used_ | `array` | Data to use in renderItem |
| children | - | `node` | Children elements |
| renderItem | _not required if children is used_ | `FlatListProps['renderItem']` | Takes an item from data and renders it into the list |
| onMomentumScrollEnd | - | `(item: { index: number }, event: any)` | Called after scroll end and the first parameter is the current index |
| vertical | false | `boolean` | Show vertical swiper |
| index | 0 | `number` | Index to start |
| renderAll | false | `boolean` | Render all the items before display it |
| **Pagination** |
| showPagination | false | `boolean` | Show pagination |
| paginationDefaultColor | gray | `string` | Pagination color |
| paginationActiveColor | white | `string` | Pagination color |
| paginationStyle | {} | `ViewStyle` | Style object for the container |
| paginationStyleItem | {} | `ViewStyle` | Style object for the item (dot) |
| paginationStyleItemActive | {} | `ViewStyle` | Style object for the active item (dot) |
| paginationStyleItemInactive | {} | `ViewStyle` | Style object for the inactive item (dot) |
| onPaginationSelectedIndex | - | `() => void` | Executed when the user presses the pagination index, similar properties onChangeIndex |
| PaginationComponent | [Component](./src/components/Pagination/index.tsx) | `node` | Overwrite Pagination component |
| **Autoplay** |
| autoplay | false | `boolean` | Change index automatically |
| autoplayDelay | 3 | `number` | Delay between every page in seconds |
| autoplayLoop | false | `boolean` | Continue playing after reach end |
| autoplayLoopKeepAnimation | false | `boolean` | Show animation when reach the end of the list |
| autoplayInvertDirection | false | `boolean` | Invert auto play direction |
| disableGesture | false | `boolean` | Disable swipe gesture |**More props**
This is a wrapper around [Flatlist](http://facebook.github.io/react-native/docs/flatlist.html#props), all their `props` works well and the inherited `props` too (from [ScrollView](http://facebook.github.io/react-native/docs/scrollview#props) and [VirtualizedList](http://facebook.github.io/react-native/docs/virtualizedlist#props))
## Functions
| Name | Type | Use |
| :-------------- | :----------------------------------------------- | :-------------------------------------------------------------------------------------------------- |
| scrollToIndex | `({ index: number, animated?: boolean}) => void` | Scroll to the index |
| getCurrentIndex | `() => number` | Returns the current index |
| getPrevIndex | `() => number` | Returns the previous index |
| onChangeIndex | `({ index: number, prevIndex: number}) => void` | Executed every time the index change, the index change when the user reaches 60% of the next screen |
| goToFirstIndex | `() => void` | Go to the first index |
| goToLastIndex | `() => void` | Go to the last index |## Right To Left
This library support RTL languages, when `I18nManager.isRTL` is `true`.
## Limitations
- Vertical pagination is not supported on Android. [Doc](https://github.com/facebook/react-native/blob/a48da14800013659e115bf2b58e31aa396e678e5/Libraries/Components/ScrollView/ScrollView.js#L274), that is way we have `useReactNativeGestureHandler` which is a workaround for this issue.
## Author
Gustavo Gard