Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/App-vNext/Polly
Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. From version 6.0.1, Polly targets .NET Standard 1.1 and 2.0+.
https://github.com/App-vNext/Polly
circuit-breaker circuit-breaker-pattern dotnet fault-handler resilience resiliency-patterns retry-strategies transient-fault-handling
Last synced: 18 days ago
JSON representation
Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. From version 6.0.1, Polly targets .NET Standard 1.1 and 2.0+.
- Host: GitHub
- URL: https://github.com/App-vNext/Polly
- Owner: App-vNext
- License: bsd-3-clause
- Created: 2013-05-05T04:40:04.000Z (about 11 years ago)
- Default Branch: main
- Last Pushed: 2024-05-02T17:11:01.000Z (21 days ago)
- Last Synced: 2024-05-03T12:07:17.599Z (20 days ago)
- Topics: circuit-breaker, circuit-breaker-pattern, dotnet, fault-handler, resilience, resiliency-patterns, retry-strategies, transient-fault-handling
- Language: C#
- Homepage: https://www.thepollyproject.org
- Size: 33.3 MB
- Stars: 13,009
- Watchers: 351
- Forks: 1,193
- Open Issues: 12
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Contributing: CONTRIBUTING.md
- Funding: .github/FUNDING.yml
- License: LICENSE
- Code of conduct: CODE_OF_CONDUCT.md
- Security: SECURITY.md
Lists
- awesome-xamarin - Polly ★4,666 - Exception handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker. (General)
- awesome-dotnet - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner (Frameworks, Libraries and Tools / Distributed Computing)
- Xaramin-Guide - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (Tools)
- awsome-dotnet - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- Oracle-Cloud-Guide - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (Tools)
- Firmware-Guide - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (Tools)
- macOS-iOS-iPadOS-Guide - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (Tools / Objective-C Tools, Libraries, and Frameworks)
- VMware-Guide - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (Tools)
- VSCode-Guide - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (.NET Tools and Frameworks)
- Windows-Terminal-Guide - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (.NET Tools and Frameworks)
- Developer-Handbook - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (Tools / Mesh networks)
- awesome-stars - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. From version 6.0.1, Polly targets .NET Standard 1.1 and 2.0+. | App-vNext | 11006 | (C#)
- awesome-dotnet-core - Polly - Polly是一个.NET弹性和瞬态故障处理库,允许开发人员以流畅和线程安全的方式表达诸如重试,断路器,超时,隔离头和回退之类的策略。 (框架, 库和工具 / 分布式计算)
- awesome-asp-net-core-api - Polly
- awesome-stars - App-vNext/Polly - Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thr (C# #)
- awesome-csharp - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- awesome - App-vNext/Polly - Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. From version 6.0.1, Polly targets .NET Standard 1.1 and 2.0+. (C\#)
- awesome-starred-test - App-vNext/Polly - Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thr (C# #)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner (Frameworks, Libraries and Tools / Distributed Computing)
- awesome-reference-tools - Polly
- awesome-stars - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. From version 6.0.1, Polly targets .NET Standard 1.1 and 2.0+. | App-vNext | 10820 | (C#)
- awesome-dotnet - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- awesome-dot-dev - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- awesome-dotnet - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner. (Frameworks, Libraries and Tools / Distributed Computing)
- awesome-dotnet - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- awesome-dotnet - Polly - Express transient exception handling policies such as Retry, Retry Forever, Wait andRetry or Circuit Breaker in a fluent manner. (.NET 3.5 / 4.0 / 4.5 / PCL / Xamarin) (Misc)
- awesome-dotnet-core-master - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner (Frameworks, Libraries and Tools / Distributed Computing)
- awesome - Polly - Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. From version 6.0.1, Polly targets .NET Standard 1.1 and 2.0+. (C# #)
- awesome-dotnet - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .Net Core / .Net Standard / Xamarin). (Misc)
- AWS-Guide - Polly - fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (Tools / Interfaces)
- awesome-stars - App-vNext/Polly - Polly is a .NET resilience and transient-fault-handling library that allows developers to express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thr (C# #)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner. (Frameworks, Libraries and Tools / Distributed Computing)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner. (Frameworks, Libraries and Tools / Distributed Computing)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner (Frameworks, Libraries and Tools / Distributed Computing)
- awesome-dotnet-cn - Polly - 表达诸如Retry、Wait-and-Retry、Circuit Breaker 和 Bulkhead Isolation的瞬时异常处理和恢复策略,线程安全且支持异步(4.0 / 4.5 / .NET Core / .NET Standard / Xamarin)。 (杂项)
- awesome-dotnet - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner (Frameworks, Libraries and Tools / Distributed Computing)
- system-architecture-awesome - Polly - Express transient-exception-handling and resilience policies such as Retry, Wait-and-Retry, Circuit Breaker, and Bulkhead Isolation in a fluent manner. Fully thread-safe and full async support. (4.0 / 4.5 / .NET Core / .NET Standard / Xamarin). (Misc)
- awesome-xamarin - **Polly ★4,666** - Exception handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker (General)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner (Frameworks, Libraries and Tools / Distributed Computing)
- awesome-dotnet - Polly - Express transient exception handling policies such as Retry, Retry Forever, Wait andRetry or Circuit Breaker in a fluent manner. (.NET 3.5 / 4.0 / 4.5 / PCL / Xamarin) (Misc)
- awesome-csharp - Polly - Express policies such as Retry, Circuit Breaker, Timeout, Bulkhead Isolation, and Fallback in a fluent and thread-safe manner. (Cheat sheets / Libraries)
- awesome-dotnet-core - Polly - .NET 3.5 / 4.0 / 4.5 / PCL library that allows developers to express transient exception and fault handling policies such as Retry, Retry Forever, Wait and Retry or Circuit Breaker in a fluent manner. (Frameworks, Libraries and Tools / Misc)
README
# Polly
Polly is a .NET resilience and transient-fault-handling library that allows developers to express resilience strategies such as Retry, Circuit Breaker, Hedging, Timeout, Rate Limiter and Fallback in a fluent and thread-safe manner.
[
](https://www.dotnetfoundation.org/)
We are a member of the [.NET Foundation](https://www.dotnetfoundation.org/about)!**Keep up to date with new feature announcements, tips & tricks, and other news through [www.thepollyproject.org](https://www.thepollyproject.org)**
[](https://github.com/App-vNext/Polly/actions?query=workflow%3Abuild+branch%3Amain+event%3Apush) [](https://codecov.io/gh/App-vNext/Polly) [](https://securityscorecards.dev/viewer/?uri=github.com/App-vNext/Polly)
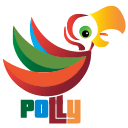
> [!IMPORTANT]
> This documentation describes the new Polly v8 API. If you are using the v7 API, please refer to the [previous version](https://github.com/App-vNext/Polly/tree/7.2.4) of the documentation.## NuGet Packages
| **Package** | **Latest Version** |
|:--|:--|
| Polly | [](https://www.nuget.org/packages/Polly/ "Download Polly from NuGet.org") |
| Polly.Core | [](https://www.nuget.org/packages/Polly.Core/ "Download Polly.Core from NuGet.org") |
| Polly.Extensions | [](https://www.nuget.org/packages/Polly.Extensions/ "Download Polly.Extensions from NuGet.org") |
| Polly.RateLimiting | [](https://www.nuget.org/packages/Polly.RateLimiting/ "Download Polly.RateLimiting from NuGet.org") |
| Polly.Testing | [](https://www.nuget.org/packages/Polly.Testing/ "Download Polly.Testing from NuGet.org") |## Documentation
This README aims to give a quick overview of some Polly features - including enough to get you started with any resilience strategy. For deeper detail on any resilience strategy, and many other aspects of Polly, be sure also to check out [pollydocs.org][polly-docs].
## Quick start
To use Polly, you must provide a callback and execute it using [**resilience pipeline**](https://www.pollydocs.org/pipelines). A resilience pipeline is a combination of one or more [**resilience strategies**](https://www.pollydocs.org/strategies) such as retry, timeout, and rate limiter. Polly uses **builders** to integrate these strategies into a pipeline.
To get started, first add the [Polly.Core](https://www.nuget.org/packages/Polly.Core/) package to your project by running the following command:
```sh
dotnet add package Polly.Core
```You can create a `ResiliencePipeline` using the `ResiliencePipelineBuilder` class as shown below:
```cs
// Create an instance of builder that exposes various extensions for adding resilience strategies
ResiliencePipeline pipeline = new ResiliencePipelineBuilder()
.AddRetry(new RetryStrategyOptions()) // Add retry using the default options
.AddTimeout(TimeSpan.FromSeconds(10)) // Add 10 seconds timeout
.Build(); // Builds the resilience pipeline// Execute the pipeline asynchronously
await pipeline.ExecuteAsync(static async token => { /* Your custom logic goes here */ }, cancellationToken);
```### Dependency injection
If you prefer to define resilience pipelines using [`IServiceCollection`](https://learn.microsoft.com/dotnet/api/microsoft.extensions.dependencyinjection.iservicecollection), you'll need to install the [Polly.Extensions](https://www.nuget.org/packages/Polly.Extensions/) package:
```sh
dotnet add package Polly.Extensions
```You can then define your resilience pipeline using the `AddResiliencePipeline(...)` extension method as shown:
```cs
var services = new ServiceCollection();// Define a resilience pipeline with the name "my-pipeline"
services.AddResiliencePipeline("my-pipeline", builder =>
{
builder
.AddRetry(new RetryStrategyOptions())
.AddTimeout(TimeSpan.FromSeconds(10));
});// Build the service provider
var serviceProvider = services.BuildServiceProvider();// Retrieve a ResiliencePipelineProvider that dynamically creates and caches the resilience pipelines
var pipelineProvider = serviceProvider.GetRequiredService>();// Retrieve your resilience pipeline using the name it was registered with
ResiliencePipeline pipeline = pipelineProvider.GetPipeline("my-pipeline");// Alternatively, you can use keyed services to retrieve the resilience pipeline
pipeline = serviceProvider.GetRequiredKeyedService("my-pipeline");// Execute the pipeline
await pipeline.ExecuteAsync(static async token =>
{
// Your custom logic goes here
});
```## Resilience strategies
Polly provides a variety of resilience strategies. Alongside the comprehensive guides for each strategy, the wiki also includes an [overview of the role each strategy plays in resilience engineering](https://github.com/App-vNext/Polly/wiki/Transient-fault-handling-and-proactive-resilience-engineering).
Polly categorizes resilience strategies into two main groups:
### Reactive
These strategies handle specific exceptions that are thrown, or results that are returned, by the callbacks executed through the strategy.
| Strategy | Premise | AKA | Mitigation |
| ------------- | ------------- | -------------- | ------------ |
|[**Retry** family](#retry) |Many faults are transient and may self-correct after a short delay.| *Maybe it's just a blip* | Allows configuring automatic retries. |
|[**Circuit-breaker** family](#circuit-breaker)|When a system is seriously struggling, failing fast is better than making users/callers wait.
Protecting a faulting system from overload can help it recover. | *Stop doing it if it hurts*
*Give that system a break* | Breaks the circuit (blocks executions) for a period, when faults exceed some pre-configured threshold. |
|[**Fallback**](#fallback)|Things will still fail - plan what you will do when that happens.| *Degrade gracefully* |Defines an alternative value to be returned (or action to be executed) on failure. |
|[**Hedging**](#hedging)|Things can be slow sometimes, plan what you will do when that happens.| *Hedge your bets* | Executes parallel actions when things are slow and waits for the fastest one. |### Proactive
Unlike reactive strategies, proactive strategies do not focus on handling errors by the callbacks might throw or return. They can make pro-active decisions to cancel or reject the execution of callbacks.
| Strategy | Premise | AKA | Prevention |
| ----------- | ------------- | -------------- | ------------ |
|[**Timeout**](#timeout)|Beyond a certain wait, a success result is unlikely.| *Don't wait forever* |Guarantees the caller won't have to wait beyond the timeout. |
|[**Rate Limiter**](#rate-limiter)|Limiting the rate a system handles requests is another way to control load.
This can apply to the way your system accepts incoming calls, and/or to the way you call downstream services. | *Slow down a bit, will you?* |Constrains executions to not exceed a certain rate. |Visit [resilience strategies](https://www.pollydocs.org/strategies) docs to explore how to configure individual resilience strategies in more detail.
### Retry
```cs
// Retry using the default options.
// See https://www.pollydocs.org/strategies/retry#defaults for defaults.
var optionsDefaults = new RetryStrategyOptions();// For instant retries with no delay
var optionsNoDelay = new RetryStrategyOptions
{
Delay = TimeSpan.Zero
};// For advanced control over the retry behavior, including the number of attempts,
// delay between retries, and the types of exceptions to handle.
var optionsComplex = new RetryStrategyOptions
{
ShouldHandle = new PredicateBuilder().Handle(),
BackoffType = DelayBackoffType.Exponential,
UseJitter = true, // Adds a random factor to the delay
MaxRetryAttempts = 4,
Delay = TimeSpan.FromSeconds(3),
};// To use a custom function to generate the delay for retries
var optionsDelayGenerator = new RetryStrategyOptions
{
MaxRetryAttempts = 2,
DelayGenerator = static args =>
{
var delay = args.AttemptNumber switch
{
0 => TimeSpan.Zero,
1 => TimeSpan.FromSeconds(1),
_ => TimeSpan.FromSeconds(5)
};// This example uses a synchronous delay generator,
// but the API also supports asynchronous implementations.
return new ValueTask(delay);
}
};// To extract the delay from the result object
var optionsExtractDelay = new RetryStrategyOptions
{
DelayGenerator = static args =>
{
if (args.Outcome.Result is HttpResponseMessage responseMessage &&
TryGetDelay(responseMessage, out TimeSpan delay))
{
return new ValueTask(delay);
}// Returning null means the retry strategy will use its internal delay for this attempt.
return new ValueTask((TimeSpan?)null);
}
};// To get notifications when a retry is performed
var optionsOnRetry = new RetryStrategyOptions
{
MaxRetryAttempts = 2,
OnRetry = static args =>
{
Console.WriteLine("OnRetry, Attempt: {0}", args.AttemptNumber);// Event handlers can be asynchronous; here, we return an empty ValueTask.
return default;
}
};// To keep retrying indefinitely or until success use int.MaxValue.
var optionsIndefiniteRetry = new RetryStrategyOptions
{
MaxRetryAttempts = int.MaxValue,
};// Add a retry strategy with a RetryStrategyOptions{} instance to the pipeline
new ResiliencePipelineBuilder().AddRetry(optionsDefaults);
new ResiliencePipelineBuilder().AddRetry(optionsExtractDelay);
```If all retries fail, a retry strategy rethrows the final exception back to the calling code.
For more details, visit the [retry strategy](https://www.pollydocs.org/strategies/retry) documentation.
### Circuit Breaker
```cs
// Circuit breaker with default options.
// See https://www.pollydocs.org/strategies/circuit-breaker#defaults for defaults.
var optionsDefaults = new CircuitBreakerStrategyOptions();// Circuit breaker with customized options:
// The circuit will break if more than 50% of actions result in handled exceptions,
// within any 10-second sampling duration, and at least 8 actions are processed.
var optionsComplex = new CircuitBreakerStrategyOptions
{
FailureRatio = 0.5,
SamplingDuration = TimeSpan.FromSeconds(10),
MinimumThroughput = 8,
BreakDuration = TimeSpan.FromSeconds(30),
ShouldHandle = new PredicateBuilder().Handle()
};// Circuit breaker using BreakDurationGenerator:
// The break duration is dynamically determined based on the properties of BreakDurationGeneratorArguments.
var optionsBreakDurationGenerator = new CircuitBreakerStrategyOptions
{
FailureRatio = 0.5,
SamplingDuration = TimeSpan.FromSeconds(10),
MinimumThroughput = 8,
BreakDurationGenerator = static args => new ValueTask(TimeSpan.FromMinutes(args.FailureCount)),
};// Handle specific failed results for HttpResponseMessage:
var optionsShouldHandle = new CircuitBreakerStrategyOptions
{
ShouldHandle = new PredicateBuilder()
.Handle()
.HandleResult(response => response.StatusCode == HttpStatusCode.InternalServerError)
};// Monitor the circuit state, useful for health reporting:
var stateProvider = new CircuitBreakerStateProvider();
var optionsStateProvider = new CircuitBreakerStrategyOptions
{
StateProvider = stateProvider
};var circuitState = stateProvider.CircuitState;
/*
CircuitState.Closed - Normal operation; actions are executed.
CircuitState.Open - Circuit is open; actions are blocked.
CircuitState.HalfOpen - Recovery state after break duration expires; actions are permitted.
CircuitState.Isolated - Circuit is manually held open; actions are blocked.
*/// Manually control the Circuit Breaker state:
var manualControl = new CircuitBreakerManualControl();
var optionsManualControl = new CircuitBreakerStrategyOptions
{
ManualControl = manualControl
};// Manually isolate a circuit, e.g., to isolate a downstream service.
await manualControl.IsolateAsync();// Manually close the circuit to allow actions to be executed again.
await manualControl.CloseAsync();// Add a circuit breaker strategy with a CircuitBreakerStrategyOptions{} instance to the pipeline
new ResiliencePipelineBuilder().AddCircuitBreaker(optionsDefaults);
new ResiliencePipelineBuilder().AddCircuitBreaker(optionsStateProvider);
```For more details, visit the [circuit breaker strategy](https://www.pollydocs.org/strategies/circuit-breaker) documentation.
### Fallback
```cs
// A fallback/substitute value if an operation fails.
var optionsSubstitute = new FallbackStrategyOptions
{
ShouldHandle = new PredicateBuilder()
.Handle()
.HandleResult(r => r is null),
FallbackAction = static args => Outcome.FromResultAsValueTask(UserAvatar.Blank)
};// Use a dynamically generated value if an operation fails.
var optionsFallbackAction = new FallbackStrategyOptions
{
ShouldHandle = new PredicateBuilder()
.Handle()
.HandleResult(r => r is null),
FallbackAction = static args =>
{
var avatar = UserAvatar.GetRandomAvatar();
return Outcome.FromResultAsValueTask(avatar);
}
};// Use a default or dynamically generated value, and execute an additional action if the fallback is triggered.
var optionsOnFallback = new FallbackStrategyOptions
{
ShouldHandle = new PredicateBuilder()
.Handle()
.HandleResult(r => r is null),
FallbackAction = static args =>
{
var avatar = UserAvatar.GetRandomAvatar();
return Outcome.FromResultAsValueTask(UserAvatar.Blank);
},
OnFallback = static args =>
{
// Add extra logic to be executed when the fallback is triggered, such as logging.
return default; // Returns an empty ValueTask
}
};// Add a fallback strategy with a FallbackStrategyOptions instance to the pipeline
new ResiliencePipelineBuilder().AddFallback(optionsOnFallback);
```For more details, visit the [fallback strategy](https://www.pollydocs.org/strategies/fallback) documentation.
### Hedging
```cs
// Hedging with default options.
// See https://www.pollydocs.org/strategies/hedging#defaults for defaults.
var optionsDefaults = new HedgingStrategyOptions();// A customized hedging strategy that retries up to 3 times if the execution
// takes longer than 1 second or if it fails due to an exception or returns an HTTP 500 Internal Server Error.
var optionsComplex = new HedgingStrategyOptions
{
ShouldHandle = new PredicateBuilder()
.Handle()
.HandleResult(response => response.StatusCode == HttpStatusCode.InternalServerError),
MaxHedgedAttempts = 3,
Delay = TimeSpan.FromSeconds(1),
ActionGenerator = static args =>
{
Console.WriteLine("Preparing to execute hedged action.");// Return a delegate function to invoke the original action with the action context.
// Optionally, you can also create a completely new action to be executed.
return () => args.Callback(args.ActionContext);
}
};// Subscribe to hedging events.
var optionsOnHedging = new HedgingStrategyOptions
{
OnHedging = static args =>
{
Console.WriteLine($"OnHedging: Attempt number {args.AttemptNumber}");
return default;
}
};// Add a hedging strategy with a HedgingStrategyOptions instance to the pipeline
new ResiliencePipelineBuilder().AddHedging(optionsDefaults);
```If all hedged attempts fail, the hedging strategy will either re-throw the original exception or return the original failed result to the caller.
For more details, visit the [hedging strategy](https://www.pollydocs.org/strategies/hedging) documentation.
### Timeout
The timeout resilience strategy assumes delegates you execute support [co-operative cancellation](https://learn.microsoft.com/dotnet/standard/threading/cancellation-in-managed-threads). You must use `Execute/Async(...)` overloads taking a `CancellationToken`, and the executed delegate must honor that `CancellationToken`.
```cs
// To add a timeout with a custom TimeSpan duration
new ResiliencePipelineBuilder().AddTimeout(TimeSpan.FromSeconds(3));// Timeout using the default options.
// See https://www.pollydocs.org/strategies/timeout#defaults for defaults.
var optionsDefaults = new TimeoutStrategyOptions();// To add a timeout using a custom timeout generator function
var optionsTimeoutGenerator = new TimeoutStrategyOptions
{
TimeoutGenerator = static args =>
{
// Note: the timeout generator supports asynchronous operations
return new ValueTask(TimeSpan.FromSeconds(123));
}
};// To add a timeout and listen for timeout events
var optionsOnTimeout = new TimeoutStrategyOptions
{
TimeoutGenerator = static args =>
{
// Note: the timeout generator supports asynchronous operations
return new ValueTask(TimeSpan.FromSeconds(123));
},
OnTimeout = static args =>
{
Console.WriteLine($"{args.Context.OperationKey}: Execution timed out after {args.Timeout.TotalSeconds} seconds.");
return default;
}
};// Add a timeout strategy with a TimeoutStrategyOptions instance to the pipeline
new ResiliencePipelineBuilder().AddTimeout(optionsDefaults);
```Timeout strategies throw `TimeoutRejectedException` when a timeout occurs.
For more details, visit the [timeout strategy](https://www.pollydocs.org/strategies/timeout) documentation.
### Rate Limiter
```cs
// Add rate limiter with default options.
// See https://www.pollydocs.org/strategies/rate-limiter#defaults for defaults.
new ResiliencePipelineBuilder()
.AddRateLimiter(new RateLimiterStrategyOptions());// Create a rate limiter to allow a maximum of 100 concurrent executions and a queue of 50.
new ResiliencePipelineBuilder()
.AddConcurrencyLimiter(100, 50);// Create a rate limiter that allows 100 executions per minute.
new ResiliencePipelineBuilder()
.AddRateLimiter(new SlidingWindowRateLimiter(
new SlidingWindowRateLimiterOptions
{
PermitLimit = 100,
Window = TimeSpan.FromMinutes(1)
}));
```Rate limiter strategy throws `RateLimiterRejectedException` if execution is rejected.
For more details, visit the [rate limiter strategy](https://www.pollydocs.org/strategies/rate-limiter) documentation.
## Chaos engineering
Starting with version `8.3.0`, Polly has integrated [Simmy](https://github.com/Polly-Contrib/Simmy), a chaos engineering library, directly into its core. For more information, please refer to the dedicated [chaos engineering documentation](https://www.pollydocs.org/chaos/).
## Next steps
To learn more about Polly, visit [pollydocs.org][polly-docs].
## Samples
- [Samples](samples/README.md): Samples in this repository that serve as an introduction to Polly.
- [Polly-Samples](https://github.com/App-vNext/Polly-Samples): Contains practical examples for using various implementations of Polly. Please feel free to contribute to the Polly-Samples repository in order to assist others who are either learning Polly for the first time, or are seeking advanced examples and novel approaches provided by our generous community.
- Microsoft's [eShopOnContainers project](https://github.com/dotnet-architecture/eShopOnContainers): Sample project demonstrating a .NET Micro-services architecture and using Polly for resilience.## License
Licensed under the terms of the [New BSD License](https://opensource.org/license/bsd-3-clause/)
[polly-docs]: https://www.pollydocs.org/