Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/jellydn/indie-stack-simple-notes
Remix Indie Stack - Simple notes app
https://github.com/jellydn/indie-stack-simple-notes
remix remix-stack
Last synced: about 2 months ago
JSON representation
Remix Indie Stack - Simple notes app
- Host: GitHub
- URL: https://github.com/jellydn/indie-stack-simple-notes
- Owner: jellydn
- Created: 2022-03-18T01:53:38.000Z (over 2 years ago)
- Default Branch: main
- Last Pushed: 2023-12-15T02:39:23.000Z (6 months ago)
- Last Synced: 2024-05-02T02:28:09.010Z (about 2 months ago)
- Topics: remix, remix-stack
- Language: TypeScript
- Homepage: https://indie-stack-simple-notes-5a5a-staging.fly.dev/
- Size: 251 KB
- Stars: 2
- Watchers: 2
- Forks: 0
- Open Issues: 1
-
Metadata Files:
- Readme: README.md
Lists
- awesome-remix - Remix Indie Stack Simple Notes
- awesome-remix - Remix Indie Stack Simple Notes
README
# Remix Indie Stack
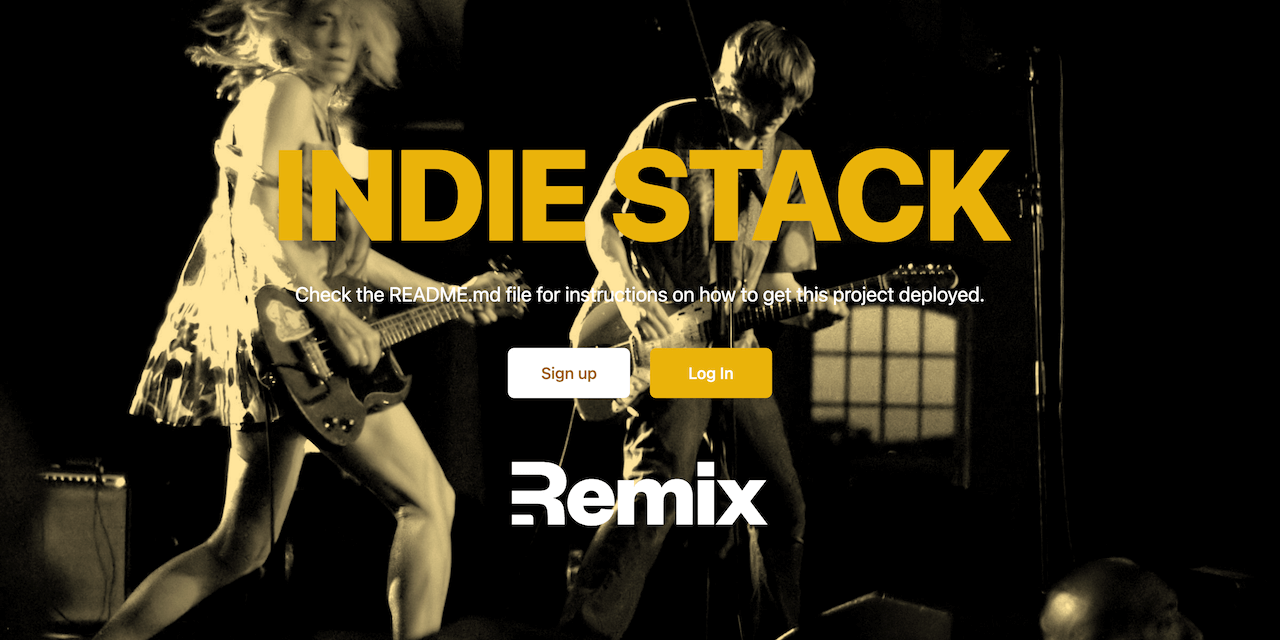
Learn more about [Remix Stacks](https://remix.run/stacks).
```
npx create-remix --template remix-run/indie-stack
```## What's in the stack
- [Fly app deployment](https://fly.io) with [Docker](https://www.docker.com/)
- Production-ready [SQLite Database](https://sqlite.org)
- Healthcheck endpoint for [Fly backups region fallbacks](https://fly.io/docs/reference/configuration/#services-http_checks)
- [GitHub Actions](https://github.com/features/actions) for deploy on merge to production and staging environments
- Email/Password Authentication with [cookie-based sessions](https://remix.run/docs/en/v1/api/remix#createcookiesessionstorage)
- Database ORM with [Prisma](https://prisma.io)
- Styling with [Tailwind](https://tailwindcss.com/)
- End-to-end testing with [Cypress](https://cypress.io)
- Local third party request mocking with [MSW](https://mswjs.io)
- Unit testing with [Vitest](https://vitest.dev) and [Testing Library](https://testing-library.com)
- Code formatting with [Prettier](https://prettier.io)
- Linting with [ESLint](https://eslint.org)
- Static Types with [TypeScript](https://typescriptlang.org)Not a fan of bits of the stack? Fork it, change it, and use `npx create-remix --template your/repo`! Make it your own.
## Development
- Initial setup: _If you just generated this project, this step has been done for you._
```sh
npm run setup
```- Start dev server:
```sh
npm run dev
```This starts your app in development mode, rebuilding assets on file changes.
The database seed script creates a new user with some data you can use to get started:
- Email: `[email protected]`
- Password: `rachelrox`### Relevant code:
This is a pretty simple note-taking app, but it's a good example of how you can build a full stack app with Prisma and Remix. The main functionality is creating users, logging in and out, and creating and deleting notes.
- creating users, and logging in and out [./app/models/user.server.ts](./app/models/user.server.ts)
- user sessions, and verifying them [./app/session.server.ts](./app/session.server.ts)
- creating, and deleting notes [./app/models/note.server.ts](./app/models/note.server.ts)## Deployment
This Remix Stack comes with two GitHub Actions that handle automatically deploying your app to production and staging environments.
Prior to your first deployment, you'll need to do a few things:
- [Install Fly](https://fly.io/docs/getting-started/installing-flyctl/)
- Sign up and log in to Fly
```sh
fly auth signup
```- Create two apps on Fly, one for staging and one for production:
```sh
fly create indie-stack-simple-notes-5a5a
fly create indie-stack-simple-notes-5a5a-staging
```- Create a new [GitHub Repository](https://repo.new)
- Add a `FLY_API_TOKEN` to your GitHub repo. To do this, go to your user settings on Fly and create a new [token](https://web.fly.io/user/personal_access_tokens/new), then add it to [your repo secrets](https://docs.github.com/en/actions/security-guides/encrypted-secrets) with the name `FLY_API_TOKEN`.
- Add a `SESSION_SECRET` to your fly app secrets, to do this you can run the following commands:
```sh
fly secrets set SESSION_SECRET=$(openssl rand -hex 32) --app indie-stack-simple-notes-5a5a
fly secrets set SESSION_SECRET=$(openssl rand -hex 32) --app indie-stack-simple-notes-5a5a-staging
```If you don't have openssl installed, you can also use [1password](https://1password.com/generate-password) to generate a random secret, just replace `$(openssl rand -hex 32)` with the generated secret.
- Create a persistent volume for the sqlite database for both your staging and production environments. Run the following:
```sh
fly volumes create data --size 1 --app indie-stack-simple-notes-5a5a
fly volumes create data --size 1 --app indie-stack-simple-notes-5a5a-staging
```Now that every is set up you can commit and push your changes to your repo. Every commit to your `main` branch will trigger a deployment to your production environment, and every commit to your `dev` branch will trigger a deployment to your staging environment.
## GitHub Actions
We use GitHub Actions for continuous integration and deployment. Anything that gets into the `main` branch will be deployed to production after running tests/build/etc. Anything in the `dev` branch will be deployed to staging.
## Testing
### Cypress
We use Cypress for our End-to-End tests in this project. You'll find those in the `cypress` directory. As you make changes, add to an existing file or create a new file in the `cypress/e2e` directory to test your changes.
We use [`@testing-library/cypress`](https://testing-library.com/cypress) for selecting elements on the page semantically.
To run these tests in development, run `npm run test:e2e:dev` which will start the dev server for the app as well as the Cypress client. Make sure the database is running in docker as described above.
We have a utility for testing authenticated features without having to go through the login flow:
```ts
cy.login();
// you are now logged in as a new user
```We also have a utility to auto-delete the user at the end of your test. Just make sure to add this in each test file:
```ts
afterEach(() => {
cy.cleanupUser();
});
```That way, we can keep your local db clean and keep your tests isolated from one another.
### Vitest
For lower level tests of utilities and individual components, we use `vitest`. We have DOM-specific assertion helpers via [`@testing-library/jest-dom`](https://testing-library.com/jest-dom).
### Type Checking
This project uses TypeScript. It's recommended to get TypeScript set up for your editor to get a really great in-editor experience with type checking and auto-complete. To run type checking across the whole project, run `npm run typecheck`.
### Linting
This project uses ESLint for linting. That is configured in `.eslintrc.js`.
### Formatting
We use [Prettier](https://prettier.io/) for auto-formatting in this project. It's recommended to install an editor plugin (like the [VSCode Prettier plugin](https://marketplace.visualstudio.com/items?itemName=esbenp.prettier-vscode)) to get auto-formatting on save. There's also a `npm run format` script you can run to format all files in the project.