Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/igniteram/protractor-cucumber-typescript
e2e kickstarter test framework which consists of protractor, cucumber frameworks using typescript lang!
https://github.com/igniteram/protractor-cucumber-typescript
angular bdd-framework cucumber-framework cucumber-js nodejs protractor protractor-cucumber-typescript testing typescript
Last synced: about 1 month ago
JSON representation
e2e kickstarter test framework which consists of protractor, cucumber frameworks using typescript lang!
- Host: GitHub
- URL: https://github.com/igniteram/protractor-cucumber-typescript
- Owner: igniteram
- License: mit
- Created: 2016-08-28T12:03:13.000Z (almost 8 years ago)
- Default Branch: master
- Last Pushed: 2023-03-03T21:17:25.000Z (over 1 year ago)
- Last Synced: 2024-04-14T05:36:45.684Z (2 months ago)
- Topics: angular, bdd-framework, cucumber-framework, cucumber-js, nodejs, protractor, protractor-cucumber-typescript, testing, typescript
- Language: TypeScript
- Size: 2.5 MB
- Stars: 196
- Watchers: 35
- Forks: 170
- Open Issues: 32
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Lists
- awesome-bdd - Protractor-Cucumber-TypeScript Setup Guide - by Ram Pasala. (Resources / Guides)
README
![]()
This project demonstrates the basic protractor-cucumber-typescript framework project setup.
---
### Protractor-Cucumber-TypeScript Setup Guide
### Medium Article
Please do checkout my medium article which would give you more insight on this setup. [protractor-cucumber-typescript(Medium)](https://medium.com/@igniteram/e2e-testing-with-protractor-cucumber-using-typescript-564575814e4a)### Features
* No typings.json or typings folder, they have been replaced by better **'@types'** modules in package.json.
* ts-node(typescript execution environment for node) in cucumberOpts.
* All scripts written with > Typescript2.0 & Cucumber2.0.
* Neat folder structures with transpiled js files in separate output folder.
* Page Object design pattern implementation.
* Extensive hooks implemented for BeforeFeature, AfterScenarios etc.
* Screenshots on failure feature scenarios.### To Get Started
#### Pre-requisites
1.NodeJS installed globally in the system.
https://nodejs.org/en/download/2.Chrome or Firefox browsers installed.
3.Text Editor(Optional) installed-->Sublime/Visual Studio Code/Brackets.
#### Setup Scripts
* Clone the repository into a folder
* Go inside the folder and run following command from terminal/command prompt
```
npm install
```
* All the dependencies from package.json and ambient typings would be installed in node_modules folder.#### Run Scripts
* First step is to fire up the selenium server which could be done in many ways, **webdriver-manager** proves very handy for this.The below command should download the **chrome & gecko driver** binaries locally for you!
```
npm run webdriver-update
```* Then you should start your selenium server!
```
npm run webdriver-start
```* The below command would create an output folder named 'typeScript' and transpile the .ts files to .js.
```
npm run build
```* Now just run the test command which launches the Chrome Browser and runs the scripts.
```
npm test
```
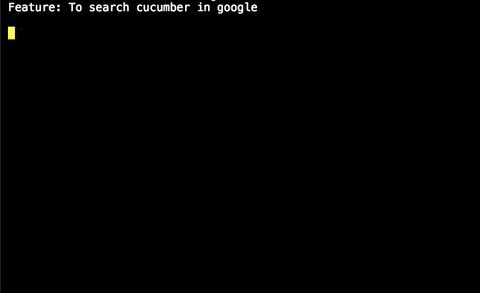#### Writing Features
```
Feature: To search typescript in google
@TypeScriptScenarioScenario: Typescript Google Search
Given I am on google page
When I type "Typescript"
Then I click on search button
Then I clear the search text
```
#### Writing Step Definitions
```
import { browser } from "protractor";
import { SearchPageObject } from "../pages/searchPage";
const { Given } = require("cucumber");
const chai = require("chai").use(require("chai-as-promised"));
const expect = chai.expect;const search: SearchPageObject = new SearchPageObject();
Given(/^I am on google page$/, async () => {
await expect(browser.getTitle()).to.eventually.equal("Google");
});
```#### Writing Page Objects
```
import { $ } from "protractor";export class SearchPageObject {
public searchTextBox: any;
public searchButton: any;constructor() {
this.searchTextBox = $("#lst-ib");
this.searchButton = $("input[value='Google Search']");
}
}
```
#### Cucumber Hooks
Following method takes screenshot on failure of each scenario
```
After(async function(scenario) {
if (scenario.result.status === Status.FAILED) {
// screenShot is a base-64 encoded PNG
const screenShot = await browser.takeScreenshot();
this.attach(screenShot, "image/png");
}
});
```
#### CucumberOpts Tags
Following configuration shows to call specific tags from feature files
```
cucumberOpts: {
compiler: "ts:ts-node/register",
format: "json:./reports/json/cucumber_report.json",
require: ["../../stepdefinitions/*.ts", "../../support/*.ts"],
strict: true,
tags: "@TypeScriptScenario or @CucumberScenario or @ProtractorScenario",
},
```
#### HTML Reports
Currently this project has been integrated with [cucumber-html-reporter](https://github.com/gkushang/cucumber-html-reporter), which is generated in the `reports` folder when you run `npm test`.
They can be customized according to user's specific needs.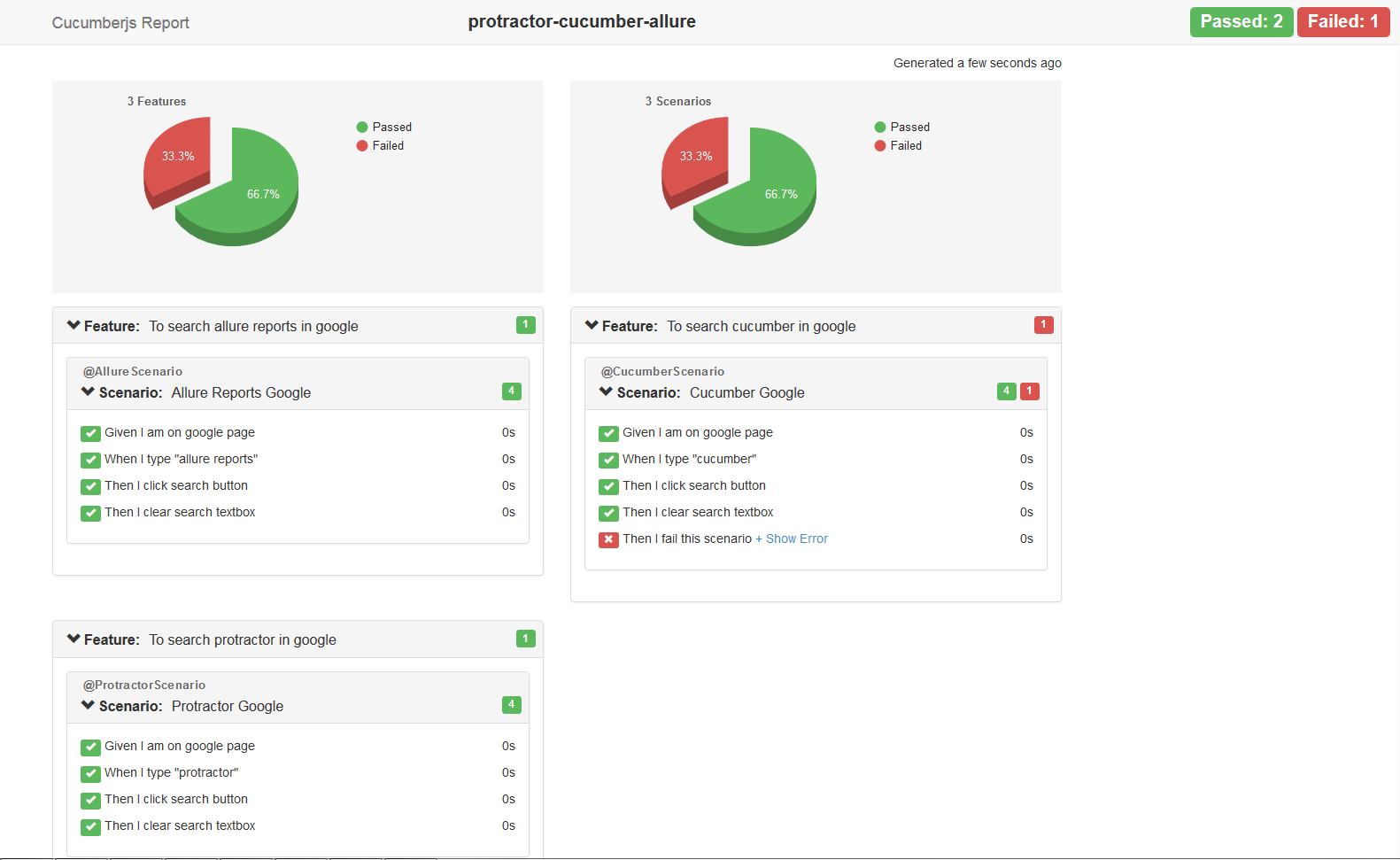
## Contributions
For contributors who want to improve this repo by contributing some code, reporting bugs, issues or improving documentation - PR's are highly welcome, please maintain the coding style , folder structure , detailed description of documentation and bugs/issues with examples if possible.## Contributors
| [
Ram Pasala](https://in.linkedin.com/in/rpasala)
[💻](https://github.com/igniteram/protractor-cucumber-typescript/commits?author=igniteram "Code") [📖](https://github.com/igniteram/protractor-cucumber-typescript/commits?author=igniteram "Documentation") [⚠️](https://github.com/igniteram/protractor-cucumber-typescript/commits?author=igniteram "Tests") [🐛](https://github.com/igniteram/protractor-cucumber-typescript/issues?q=author%3Aigniteram "Bug reports") | [
Burk Hufnagel](https://github.com/BurkHufnagel)
[💻](https://github.com/igniteram/protractor-cucumber-typescript/commits?author=BurkHufnagel "Code") | [
Alejandro](https://github.com/sanko1983)
[💻](https://github.com/igniteram/protractor-cucumber-typescript/commits?author=sanko1983 "Code") [🐛](https://github.com/igniteram/protractor-cucumber-typescript/issues?q=author%3Asanko1983 "Bug reports") | [
David Jimenez](https://github.com/runnerdave)
[💻](https://github.com/igniteram/protractor-cucumber-typescript/commits?author=runnerdave "Code") |
| :---: | :---: | :---: | :---: |Thanks goes to these wonderful people ([emoji key](https://github.com/kentcdodds/all-contributors#emoji-key)):
This project follows the [all-contributors](https://github.com/kentcdodds/all-contributors) specification. Contributions of any kind welcome!
## License
```
MIT LicenseCopyright (c) 2019 Ram Pasala
```