Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/jkriege2/TinyTIFF
lightweight TIFF reader/writer library (C/C++)
https://github.com/jkriege2/TinyTIFF
file-format lib tiff-encoder tiff-files tiff-ios
Last synced: about 2 months ago
JSON representation
lightweight TIFF reader/writer library (C/C++)
- Host: GitHub
- URL: https://github.com/jkriege2/TinyTIFF
- Owner: jkriege2
- License: lgpl-3.0
- Created: 2015-07-11T09:14:10.000Z (almost 9 years ago)
- Default Branch: master
- Last Pushed: 2023-06-30T18:11:17.000Z (12 months ago)
- Last Synced: 2023-11-07T18:18:40.927Z (8 months ago)
- Topics: file-format, lib, tiff-encoder, tiff-files, tiff-ios
- Language: C++
- Homepage: http://jkriege2.github.io/TinyTIFF/
- Size: 2.21 MB
- Stars: 116
- Watchers: 13
- Forks: 33
- Open Issues: 8
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Lists
- awesome-cpp - TinyTIFF - lightweight TIFF reader/writer library. [GPL-3.0] (Image Processing)
- fucking-awesome-cpp - TinyTIFF - lightweight TIFF reader/writer library. [GPL-3.0] (Image Processing)
README
# TinyTIFF
(c) 2014-2020 by Jan W. Krieger
This is a lightweight C/C++ library, which is able to read and write basic TIFF
files. It is significantly faster than libTIFF, especially in writing large
multi-frame TIFFs.This software is licensed under the term of the [GNU Lesser General Public License 3.0
(LGPL 3.0)](https://raw.githubusercontent.com/jkriege2/TinyTIFF/master/LICENSE).[](https://github.com/jkriege2/TinyTIFF/releases)

[](http://jkriege2.github.io/TinyTIFF/index.html)
[](https://github.com/jkriege2/TinyTIFF/pulse)
[](https://github.com/jkriege2/TinyTIFF/graphs/contributors)[](https://github.com/jkriege2/TinyTIFF/issues)
[](https://github.com/jkriege2/TinyTIFF/issues?q=is%3Aissue+is%3Aclosed)[](https://github.com/jkriege2/TinyTIFF/pulls)
[](https://github.com/jkriege2/TinyTIFF/pulls?q=is%3Apr+is%3Aclosed)[](https://github.com/jkriege2/TinyTIFF/actions/workflows/build_msvc.yml)
[](https://github.com/jkriege2/TinyTIFF/actions/workflows/msvc-codeanalysis.yml)
[](https://github.com/jkriege2/TinyTIFF/actions/workflows/build_docs.yml)# TinyTIFFReader
## Basiscs
The methods in this file allow to read TIFF files with limited capabilites, but very fast (comapred to libtiff) and also more frames from a multi-frame TIFF than libtiff (which is currently limited to 65535 frames due to internal data sizes!).
This library currently support TIFF files, which meet the following criteria:
* TIFF-only (no BigTIFF), i.e. max. 4GB
* uncompressed frames
* one, or more samples per frame
* data types: UINT, INT, FLOAT, 8-64bit
* planar and chunky data organization, for multi-sample data
* no suppoer for palleted images
* stripped TIFFs only, tiling is not supported## Usage
This example reads all frames from a TIFF file:
```C++
TinyTIFFReaderFile* tiffr=NULL;
tiffr=TinyTIFFReader_open(filename);
if (!tiffr) {
std::cout<<" ERROR reading (not existent, not accessible or no TIFF file)\n";
} else {
if (TinyTIFFReader_wasError(tiffr)) {
std::cout<<" ERROR:"<1000) frames are written into a TIFF-file. LibTIFF does not need constant time per frame (i.e. the time to write a multi-frame TIFF grows linearly with the number of frames), but the time to write a frame increases with the number of frames.
The following performance measurement shows this. It was acquired using `tinytiffwriter_speedtest` from this repository and shows the average time required to write one frame (64x64x pixels, 16-bit integer) out of a number (10, 100, 1000, ...) of frames. It compares the performance of libTIFF, TinyTIFFWriter and simply writing the dtaa using `fwrite()` ("RAW"). It was acquired on an Ryzen 5 3600+, Win10, 32-bit Release-build, writing onto a Harddisk (not a SSD)| Ryzen 5 3600+, Win10, 32-bit Release-build, writing onto a Harddisk, libTiff 3.8.2 | Ryzen 7 5800H, Win11, 64-bit Release-build, writing onto an SSD, libTiff 4.6.0 |
:------------------------------------------------------------------------------------:|:--------------------------------------------------------------------------------:
 | 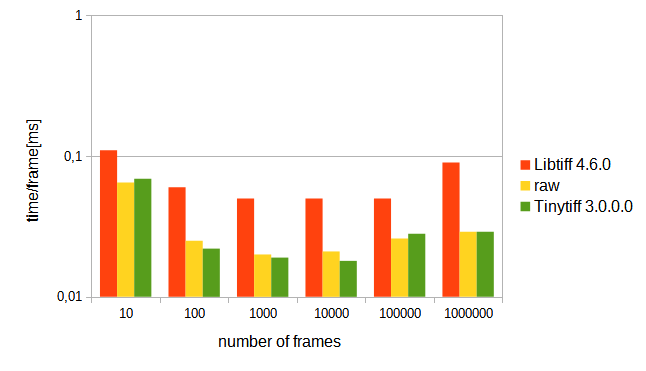For a microscope developed during my PhD thesis, it was necessary to write 100000 frames and more with acceptable duration. Therefore libTIFF was unusable and TinyTIFFWriter was developed.
As can be seen in the graph above. The performance of TinyTIFFWriter and `fwrite()`/RAW is comparable, whereas the performance of LibTIFF falls off towards large files on harddisks. On SSDs the performance of libTIFF does not show an increase with number of images, but is still significantly (1.5-3x, note the logarithmic y-axis) faster than libTIFF.The following image shows another performance measurement, this time for different frame sizes (64x64-4096x4096, acquired on an Ryzen 5 3600+, Win10, 32-bit Release-build, writing onto a Harddisk (not a SSD)):
| Ryzen 5 3600+, Win10, 32-bit Release-build, writing onto a Harddisk, libTiff 3.8.2 | Ryzen 7 5800H, Win11, 64-bit Release-build, writing onto an SSD, libTiff 4.6.0 |
:------------------------------------------------------------------------------------:|:--------------------------------------------------------------------------------:
 | 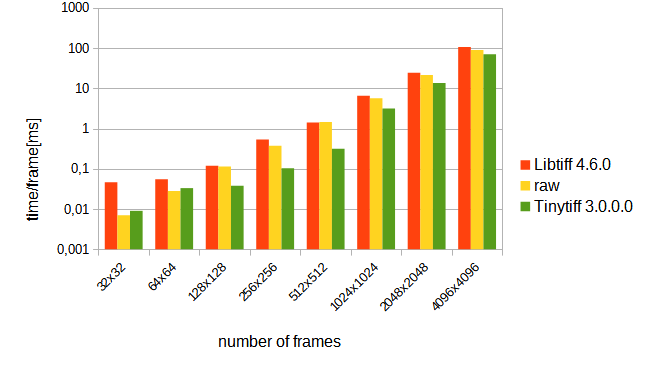This suggests that for harddisks the performance of TinyTIFFWriter and `fwrite()` are comparable for all image sizes. For larger images, also the performance of libTIFF is in the same range, whereas for small images, libTIFF falls off somewhat. For SSDs, the libraries are closer together, but still TinyTIFFWriter is faster than libTIFF by a factor of 1.5-5x (again note the logarithmic scale on the y-axis!).
# Documentation
* library docukentation: https://travis-ci.org/jkriege2/TinyTIFF
* API documentation: http://jkriege2.github.io/TinyTIFF/modules.html
* build instructions: http://jkriege2.github.io/TinyTIFF/page_buildinstructions.html
* usage instructions: http://jkriege2.github.io/TinyTIFF/page_useinstructions.html