https://github.com/14f3v/14f3v.mssql-adapter
Node.js package for streamlined MSSQL parameter binding with JavaScript and TypeScript compatibility
https://github.com/14f3v/14f3v.mssql-adapter
bun javascript-library library mssql nodejs typescript-library
Last synced: 3 months ago
JSON representation
Node.js package for streamlined MSSQL parameter binding with JavaScript and TypeScript compatibility
- Host: GitHub
- URL: https://github.com/14f3v/14f3v.mssql-adapter
- Owner: 14f3v
- License: mit
- Created: 2024-08-12T02:30:44.000Z (11 months ago)
- Default Branch: main
- Last Pushed: 2024-10-18T12:41:16.000Z (9 months ago)
- Last Synced: 2025-02-10T21:43:48.090Z (5 months ago)
- Topics: bun, javascript-library, library, mssql, nodejs, typescript-library
- Language: TypeScript
- Homepage:
- Size: 3.61 MB
- Stars: 0
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# 14f3v.bun-library
The primary purpose of this package is to allow developers to handle MSSQL parameter binding effortlessly. By using mssql-param-binding-adapter, developers can ensure that their SQL queries are protected against injection attacks and are easier to manage, leading to more secure and reliable database interactions.
## Key Features
This package simplifies SQL operations by providing parameter binding for common MSSQL statements, ensuring secure and efficient database interactions.
- **Selection Parameter Binding**: Easily bind parameters in `SELECT` queries to retrieve data safely.
- **Insertion Parameter Binding**: Bind parameters in `INSERT` queries to add data securely.
- **Update Parameter Binding**: Bind parameters in `UPDATE` queries to modify data effectively.
- **Deletion Parameter Binding**: Bind parameters in `DELETE` queries to remove data securely.## Development RoadMaps
The development plan focuses on enhancing the package to support a wider range of SQL operations. Upcoming features include:
- **Support for Advanced `SELECT` Queries**: Implement parameter binding for complex SELECT statements, including `SELECT-IN` and `SELECT-LIKE`.
- **Bulk Operations**: Introduce support for bulk operations, including `BULK-INSERT`, `BULK-UPDATE`, and `BULK-DELETE`, allowing efficient handling of large datasets.
- **Comprehensive Documentation**: Expand documentation with examples and best practices for using the package in various scenarios.
- **Performance Optimizations**: Continuously improve the package's performance, particularly for high-volume operations.## Installation
Package not available on npm package manager platform. So, in-case of install package via git, follow an instruction below.- Using bun package manager.
```bash
bun add --dev git+https://github.com/14f3v/14f3v.mssql-adapter.git
```- Using npm package manager.
```bash
npm install git+https://github.com/14f3v/14f3v.mssql-adapter.git
```- Using yarn package manager.
```bash
yarn add git+https://github.com/14f3v/14f3v.mssql-adapter.git
```## Usage
### Selection params binding usecase.
a usecase of using mssql adaptor selection params binding.
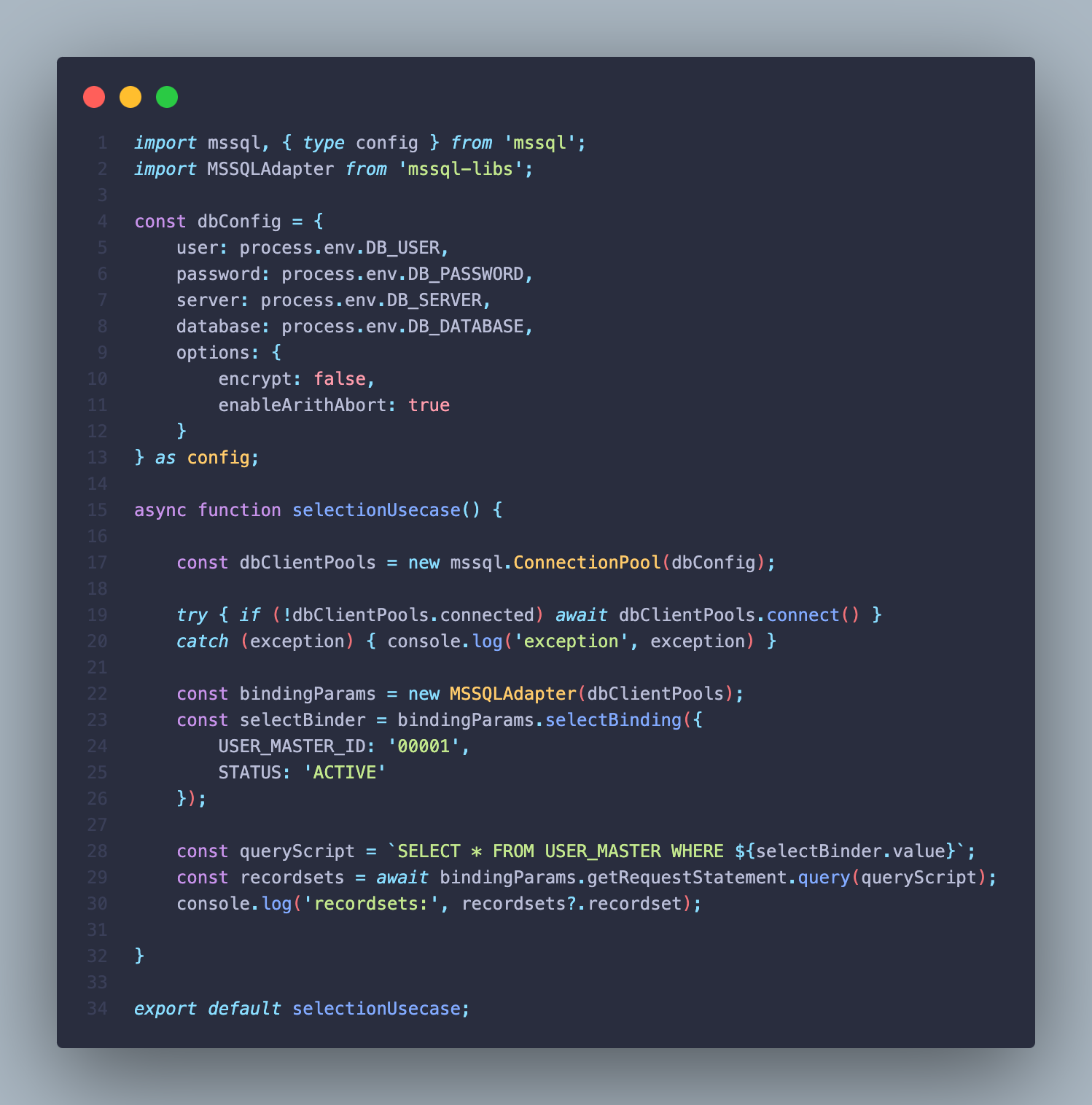
- code example to use mssql adaptor selection param binding.
```typescript
import mssql, { type config } from 'mssql';
import MSSQLAdapter from 'mssql-libs';const dbConfig = {
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
server: process.env.DB_SERVER,
database: process.env.DB_DATABASE,
options: {
encrypt: false,
enableArithAbort: true
}
} as config;async function selectionUsecase() {
const dbClientPools = new mssql.ConnectionPool(dbConfig);
try { if (!dbClientPools.connected) await dbClientPools.connect() }
catch (exception) { console.log('exception', exception) }const bindingParams = new MSSQLAdapter(dbClientPools);
const selectBinder = bindingParams.selectBinding({
USER_MASTER_ID: '00001',
STATUS: 'ACTIVE'
});const queryScript = `SELECT * FROM USER_MASTER WHERE ${selectBinder.value}`;
const recordsets = await bindingParams.getRequestStatement.query(queryScript);
console.log('recordsets:', recordsets?.recordset);}
export default selectionUsecase;
```### Insertion params binding usecase.
a usecase of using mssql adaptor insertion params binding.
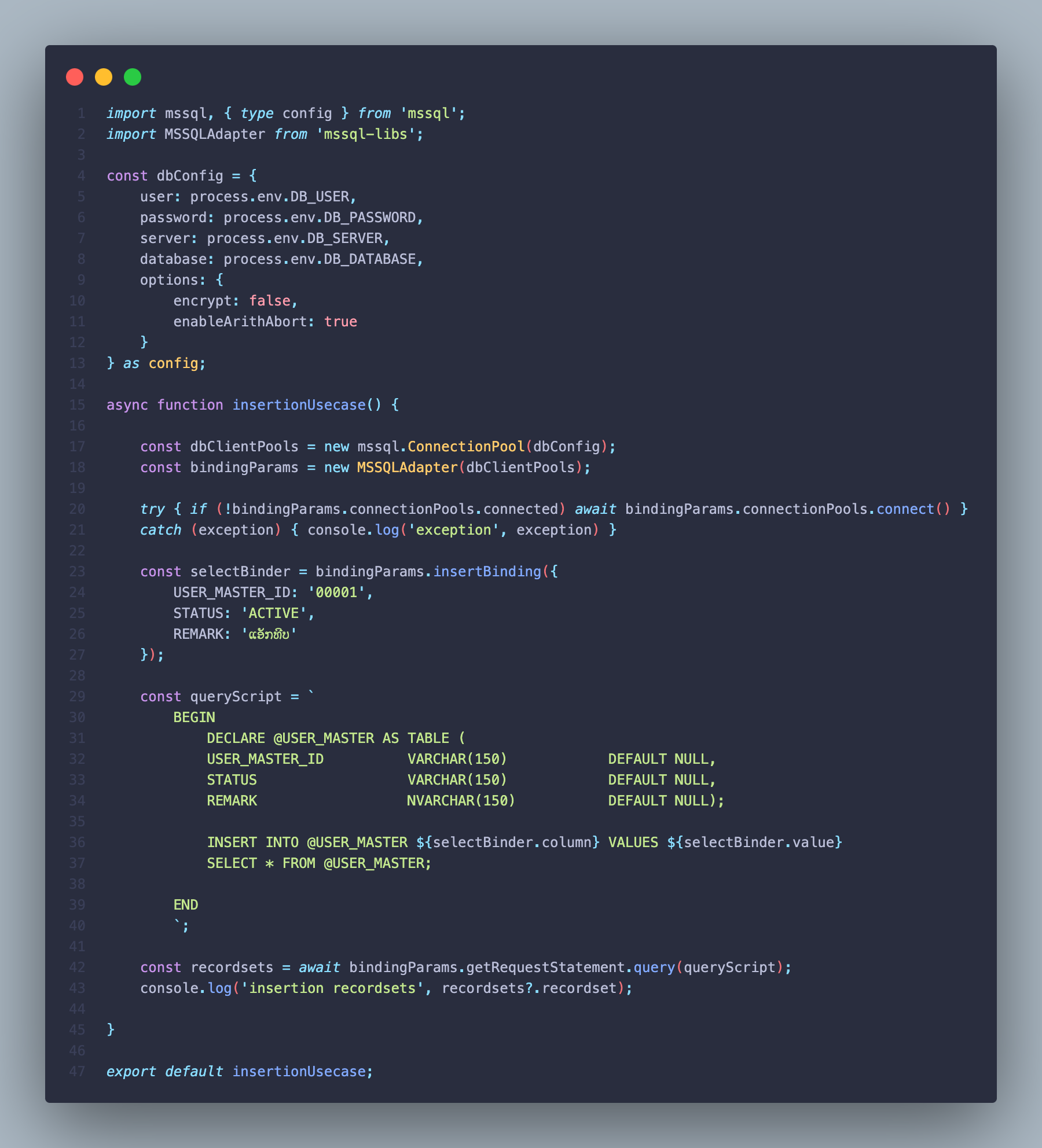
- code example to use mssql adaptor insertion param binding.
```typescript
import mssql, { type config } from 'mssql';
import MSSQLAdapter from 'mssql-libs';const dbConfig = {
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
server: process.env.DB_SERVER,
database: process.env.DB_DATABASE,
options: {
encrypt: false,
enableArithAbort: true
}
} as config;async function insertionUsecase() {
const dbClientPools = new mssql.ConnectionPool(dbConfig);
const bindingParams = new MSSQLAdapter(dbClientPools);try { if (!bindingParams.connectionPools.connected) await bindingParams.connectionPools.connect() }
catch (exception) { console.log('exception', exception) }const selectBinder = bindingParams.insertBinding({
USER_MASTER_ID: '00001',
STATUS: 'ACTIVE',
REMARK: 'ແອັກທີບ'
});const queryScript = `
BEGIN
DECLARE @USER_MASTER AS TABLE (
USER_MASTER_ID VARCHAR(150) DEFAULT NULL,
STATUS VARCHAR(150) DEFAULT NULL,
REMARK NVARCHAR(150) DEFAULT NULL);INSERT INTO @USER_MASTER ${selectBinder.column} VALUES ${selectBinder.value}
SELECT * FROM @USER_MASTER;END
`;const recordsets = await bindingParams.getRequestStatement.query(queryScript);
console.log('insertion recordsets', recordsets?.recordset);}
export default insertionUsecase;
```### Update params binding usecase.
a usecase of using mssql adaptor update params binding.
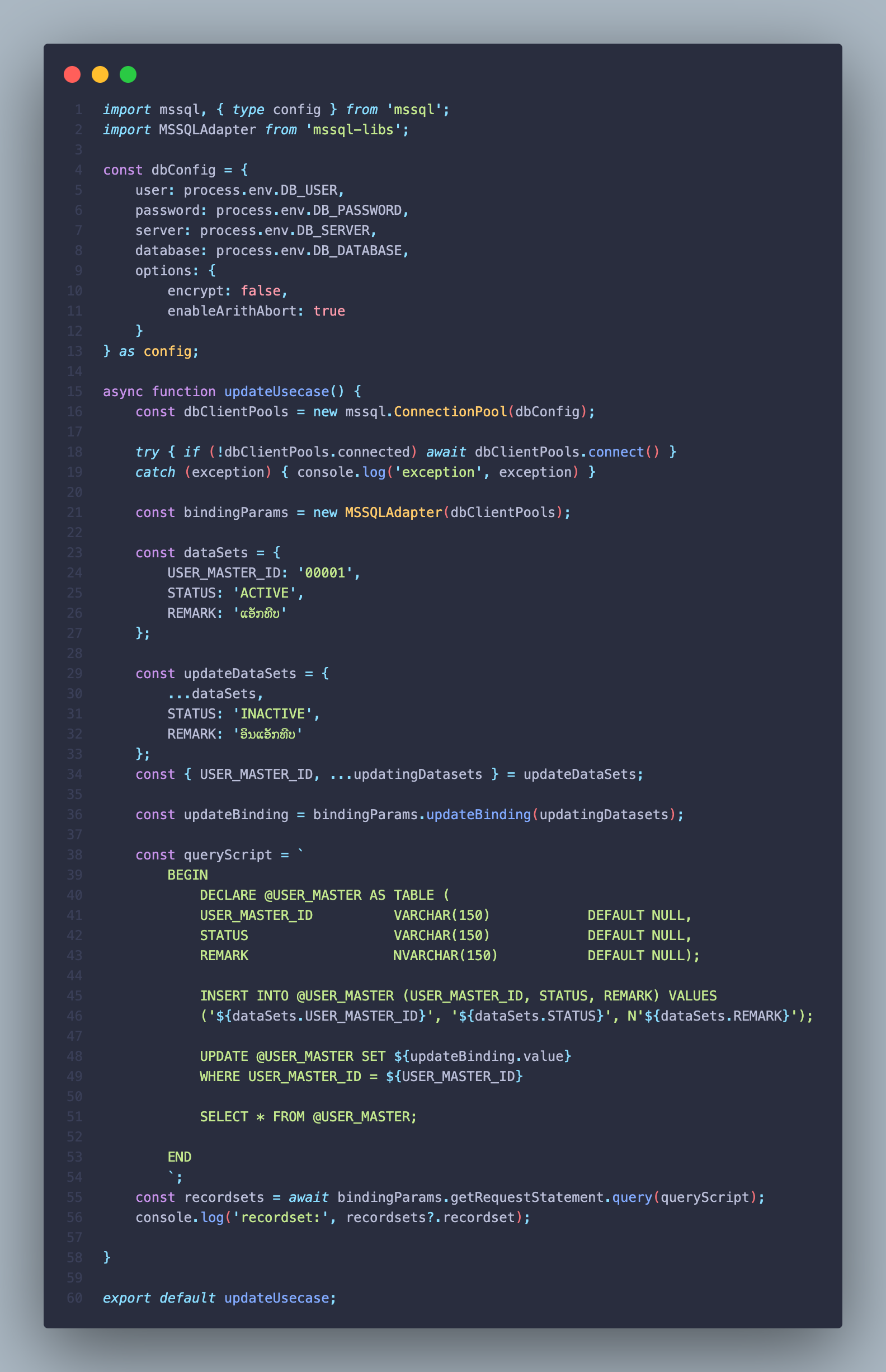
- code example to use mssql adaptor update param binding.
```typescript
import mssql, { type config } from 'mssql';
import MSSQLAdapter from 'mssql-libs';const dbConfig = {
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
server: process.env.DB_SERVER,
database: process.env.DB_DATABASE,
options: {
encrypt: false,
enableArithAbort: true
}
} as config;async function updateUsecase() {
const dbClientPools = new mssql.ConnectionPool(dbConfig);try { if (!dbClientPools.connected) await dbClientPools.connect() }
catch (exception) { console.log('exception', exception) }const bindingParams = new MSSQLAdapter(dbClientPools);
const dataSets = {
USER_MASTER_ID: '00001',
STATUS: 'ACTIVE',
REMARK: 'ແອັກທີບ'
};const updateDataSets = {
...dataSets,
STATUS: 'INACTIVE',
REMARK: 'ອິນແອັກທີບ'
};
const { USER_MASTER_ID, ...updatingDatasets } = updateDataSets;const updateBinding = bindingParams.updateBinding(updatingDatasets);
const queryScript = `
BEGIN
DECLARE @USER_MASTER AS TABLE (
USER_MASTER_ID VARCHAR(150) DEFAULT NULL,
STATUS VARCHAR(150) DEFAULT NULL,
REMARK NVARCHAR(150) DEFAULT NULL);INSERT INTO @USER_MASTER (USER_MASTER_ID, STATUS, REMARK) VALUES
('${dataSets.USER_MASTER_ID}', '${dataSets.STATUS}', N'${dataSets.REMARK}');UPDATE @USER_MASTER SET ${updateBinding.value}
WHERE USER_MASTER_ID = ${USER_MASTER_ID}SELECT * FROM @USER_MASTER;
END
`;
const recordsets = await bindingParams.getRequestStatement.query(queryScript);
console.log('recordset:', recordsets?.recordset);}
export default updateUsecase;
```### Deletion params binding usecase.
a usecase of using mssql adaptor deletion params binding.
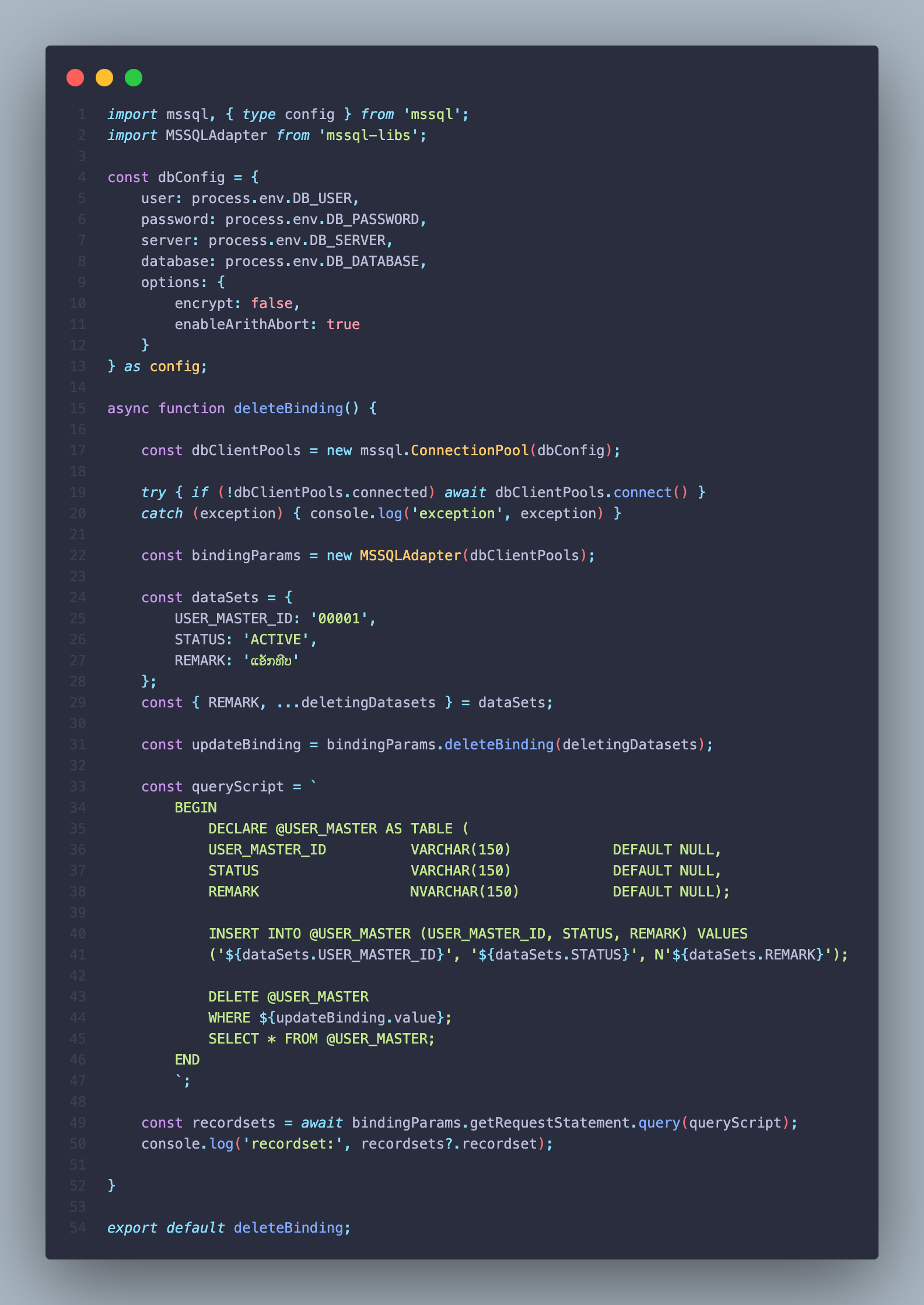
- code example to use mssql adaptor deletion param binding.
```typescript
import mssql, { type config } from 'mssql';
import MSSQLAdapter from 'mssql-libs';const dbConfig = {
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
server: process.env.DB_SERVER,
database: process.env.DB_DATABASE,
options: {
encrypt: false,
enableArithAbort: true
}
} as config;async function deleteBinding() {
const dbClientPools = new mssql.ConnectionPool(dbConfig);
try { if (!dbClientPools.connected) await dbClientPools.connect() }
catch (exception) { console.log('exception', exception) }const bindingParams = new MSSQLAdapter(dbClientPools);
const dataSets = {
USER_MASTER_ID: '00001',
STATUS: 'ACTIVE',
REMARK: 'ແອັກທີບ'
};
const { REMARK, ...deletingDatasets } = dataSets;const updateBinding = bindingParams.deleteBinding(deletingDatasets);
const queryScript = `
BEGIN
DECLARE @USER_MASTER AS TABLE (
USER_MASTER_ID VARCHAR(150) DEFAULT NULL,
STATUS VARCHAR(150) DEFAULT NULL,
REMARK NVARCHAR(150) DEFAULT NULL);INSERT INTO @USER_MASTER (USER_MASTER_ID, STATUS, REMARK) VALUES
('${dataSets.USER_MASTER_ID}', '${dataSets.STATUS}', N'${dataSets.REMARK}');DELETE @USER_MASTER
WHERE ${updateBinding.value};
SELECT * FROM @USER_MASTER;
END
`;const recordsets = await bindingParams.getRequestStatement.query(queryScript);
console.log('recordset:', recordsets?.recordset);}
export default deleteBinding;
```### Feedback and Suggestions
If you have any questions, feedback, or suggestions, please open an issue on the repository. We're open to discussions and appreciate any input you may have.## License
This project is licensed under the MIT License - see the [LICENSE](./LICENSE) file for details.Feel free to customize the content according to your project's needs and guidelines. A clear and welcoming contribution section can help foster a collaborative and inclusive community around your project.
## Contributing
Contributions are welcome! Feel free to submit issues and pull requests.### Package modules.
To updating a modules navigate to here [src/cores/services/mssql.binding.ts](./src/cores/services/mssql.binding.ts) to see a detail.
### Module testing.
After make a change completed. run package.json script to test a module updated completion by using a script below.
```bash
bun run build && bun run test
```After run test successfully with out error occrur to module updated or previous change. then create Pull request to main branch and wait for review an approval before make a release.