https://github.com/Cap-go/native-audio
Capacitor plugin for native audio engine.
https://github.com/Cap-go/native-audio
audio capacitor capacitor-plugin typescript
Last synced: 5 months ago
JSON representation
Capacitor plugin for native audio engine.
- Host: GitHub
- URL: https://github.com/Cap-go/native-audio
- Owner: Cap-go
- License: mit
- Created: 2022-11-01T08:05:22.000Z (over 2 years ago)
- Default Branch: main
- Last Pushed: 2024-10-31T21:46:40.000Z (6 months ago)
- Last Synced: 2024-11-07T21:26:47.106Z (5 months ago)
- Topics: audio, capacitor, capacitor-plugin, typescript
- Language: Java
- Homepage: https://capgo.app
- Size: 6.81 MB
- Stars: 38
- Watchers: 3
- Forks: 16
- Open Issues: 15
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Contributing: .github/CONTRIBUTING.md
- Funding: .github/FUNDING.yml
- License: LICENSE
- Code of conduct: .github/CODE_OF_CONDUCT.md
- Codeowners: .github/CODEOWNERS.txt
Awesome Lists containing this project
- awesome-capacitorjs - @capgo/native-audio - Capacitor plugin for native audio engine (fork). (Plugins / Community Plugins)
- awesome-capacitor - Native audio - Capacitor plugin for native audio engine (fork). ([Capgo plugins](https://capgo.app/))
README
# Native audio
β‘οΈ Get Instant updates for your App with Capgo π
Fix your annoying bug now, Hire a Capacitor expert πͺ
Native Audio
@capgo/native-audio
Capacitor plugin for playing sounds.
# Capacitor Native Audio Plugin
Capacitor plugin for native audio engine.
Capacitor V6 - β Support!Click on video to see example π₯
[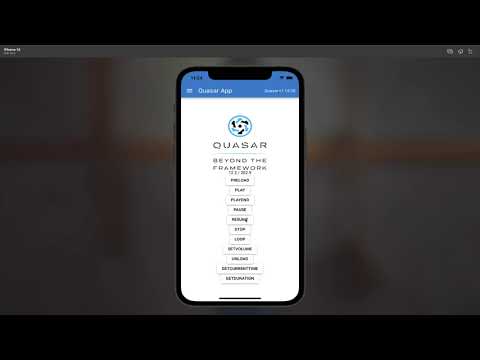](https://www.youtube.com/watch?v=XpUGlWWtwHs)
## Maintainers
| Maintainer | GitHub | Social |
| --------------- | ----------------------------------- | --------------------------------------- |
| Martin Donadieu | [riderx](https://github.com/riderx) | [Telegram](https://t.me/martindonadieu) |Mainteinance Status: Actively Maintained
## Preparation
All audio files must be with the rest of your source files.
First make your sound file end up in your builded code folder, example in folder `BUILDFOLDER/assets/sounds/FILENAME.mp3`
Then use it in preload like that `assets/sounds/FILENAME.mp3`## Installation
To use npm
```bash
npm install @capgo/native-audio
```To use yarn
```bash
yarn add @capgo/native-audio
```Sync native files
```bash
npx cap sync
```On iOS, Android and Web, no further steps are needed.
## Configuration
No configuration required for this plugin.
## Supported methods
| Name | Android | iOS | Web |
| :------------- | :------ | :-- | :-- |
| configure | β | β | β |
| preload | β | β | β |
| play | β | β | β |
| pause | β | β | β |
| resume | β | β | β |
| loop | β | β | β |
| stop | β | β | β |
| unload | β | β | β |
| setVolume | β | β | β |
| getDuration | β | β | β |
| getCurrentTime | β | β | β |
| isPlaying | β | β | β |## Usage
[Example repository](https://github.com/bazuka5801/native-audio-example)
```typescript
import {NativeAudio} from '@capgo/native-audio'/**
* This method will load more optimized audio files for background into memory.
* @param assetPath - relative path of the file, absolute url (file://) or remote url (https://)
* assetId - unique identifier of the file
* audioChannelNum - number of audio channels
* isUrl - pass true if assetPath is a `file://` url
* @returns void
*/
NativeAudio.preload({
assetId: "fire",
assetPath: "assets/sounds/fire.mp3",
audioChannelNum: 1,
isUrl: false
});/**
* This method will play the loaded audio file if present in the memory.
* @param assetId - identifier of the asset
* @param time - (optional) play with seek. example: 6.0 - start playing track from 6 sec
* @returns void
*/
NativeAudio.play({
assetId: 'fire',
// time: 6.0 - seek time
});/**
* This method will loop the audio file for playback.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.loop({
assetId: 'fire',
});/**
* This method will stop the audio file if it's currently playing.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.stop({
assetId: 'fire',
});/**
* This method will unload the audio file from the memory.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.unload({
assetId: 'fire',
});/**
* This method will set the new volume for a audio file.
* @param assetId - identifier of the asset
* volume - numerical value of the volume between 0.1 - 1.0 default 1.0
* @returns void
*/
NativeAudio.setVolume({
assetId: 'fire',
volume: 0.4,
});/**
* this method will getΒ the duration of an audio file.
* only works if channels == 1
*/
NativeAudio.getDuration({
assetId: 'fire'
})
.then(result => {
console.log(result.duration);
})/**
* this method will get the current time of a playing audio file.
* only works if channels == 1
*/
NativeAudio.getCurrentTime({
assetId: 'fire'
});
.then(result => {
console.log(result.currentTime);
})/**
* This method will return false if audio is paused or not loaded.
* @param assetId - identifier of the asset
* @returns {isPlaying: boolean}
*/
NativeAudio.isPlaying({
assetId: 'fire'
})
.then(result => {
console.log(result.isPlaying);
})
```## API
### configure(...)
```typescript
configure(options: ConfigureOptions) => Promise
```Configure the audio player
| Param | Type |
| ------------- | ------------------------------------------------------------- |
| **`options`** |ConfigureOptions
|**Since:** 5.0.0
--------------------
### preload(...)
```typescript
preload(options: PreloadOptions) => Promise
```Load an audio file
| Param | Type |
| ------------- | --------------------------------------------------------- |
| **`options`** |PreloadOptions
|**Since:** 5.0.0
--------------------
### isPreloaded(...)
```typescript
isPreloaded(options: PreloadOptions) => Promise<{ found: boolean; }>
```Check if an audio file is preloaded
| Param | Type |
| ------------- | --------------------------------------------------------- |
| **`options`** |PreloadOptions
|**Returns:**
Promise<{ found: boolean; }>
**Since:** 6.1.0
--------------------
### play(...)
```typescript
play(options: { assetId: string; time?: number; delay?: number; }) => Promise
```Play an audio file
| Param | Type |
| ------------- | ---------------------------------------------------------------- |
| **`options`** |{ assetId: string; time?: number; delay?: number; }
|**Since:** 5.0.0
--------------------
### pause(...)
```typescript
pause(options: Assets) => Promise
```Pause an audio file
| Param | Type |
| ------------- | ----------------------------------------- |
| **`options`** |Assets
|**Since:** 5.0.0
--------------------
### resume(...)
```typescript
resume(options: Assets) => Promise
```Resume an audio file
| Param | Type |
| ------------- | ----------------------------------------- |
| **`options`** |Assets
|**Since:** 5.0.0
--------------------
### loop(...)
```typescript
loop(options: Assets) => Promise
```Stop an audio file
| Param | Type |
| ------------- | ----------------------------------------- |
| **`options`** |Assets
|**Since:** 5.0.0
--------------------
### stop(...)
```typescript
stop(options: Assets) => Promise
```Stop an audio file
| Param | Type |
| ------------- | ----------------------------------------- |
| **`options`** |Assets
|**Since:** 5.0.0
--------------------
### unload(...)
```typescript
unload(options: Assets) => Promise
```Unload an audio file
| Param | Type |
| ------------- | ----------------------------------------- |
| **`options`** |Assets
|**Since:** 5.0.0
--------------------
### setVolume(...)
```typescript
setVolume(options: { assetId: string; volume: number; }) => Promise
```Set the volume of an audio file
| Param | Type |
| ------------- | ------------------------------------------------- |
| **`options`** |{ assetId: string; volume: number; }
|**Since:** 5.0.0
--------------------
### setRate(...)
```typescript
setRate(options: { assetId: string; rate: number; }) => Promise
```Set the rate of an audio file
| Param | Type |
| ------------- | ----------------------------------------------- |
| **`options`** |{ assetId: string; rate: number; }
|**Since:** 5.0.0
--------------------
### getCurrentTime(...)
```typescript
getCurrentTime(options: { assetId: string; }) => Promise<{ currentTime: number; }>
```Set the current time of an audio file
| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|**Returns:**
Promise<{ currentTime: number; }>
**Since:** 5.0.0
--------------------
### getDuration(...)
```typescript
getDuration(options: Assets) => Promise<{ duration: number; }>
```Get the duration of an audio file
| Param | Type |
| ------------- | ----------------------------------------- |
| **`options`** |Assets
|**Returns:**
Promise<{ duration: number; }>
**Since:** 5.0.0
--------------------
### isPlaying(...)
```typescript
isPlaying(options: Assets) => Promise<{ isPlaying: boolean; }>
```Check if an audio file is playing
| Param | Type |
| ------------- | ----------------------------------------- |
| **`options`** |Assets
|**Returns:**
Promise<{ isPlaying: boolean; }>
**Since:** 5.0.0
--------------------
### addListener('complete', ...)
```typescript
addListener(eventName: "complete", listenerFunc: CompletedListener) => Promise
```Listen for complete event
| Param | Type |
| ------------------ | --------------------------------------------------------------- |
| **`eventName`** |'complete'
|
| **`listenerFunc`** |CompletedListener
|**Returns:**
Promise<PluginListenerHandle>
**Since:** 5.0.0
return {@link CompletedEvent}--------------------
### Interfaces
#### ConfigureOptions
| Prop | Type | Description |
| ---------------- | -------------------- | ------------------------------------------------------- |
| **`fade`** |boolean
| Play the audio with Fade effect, only available for IOS |
| **`focus`** |boolean
| focus the audio with Audio Focus |
| **`background`** |boolean
| Play the audio in the background |#### PreloadOptions
| Prop | Type | Description |
| --------------------- | -------------------- | -------------------------------------------------------------------------------------------------- |
| **`assetPath`** |string
| Path to the audio file, relative path of the file, absolute url (file://) or remote url (https://) |
| **`assetId`** |string
| Asset Id, unique identifier of the file |
| **`volume`** |number
| Volume of the audio, between 0.1 and 1.0 |
| **`audioChannelNum`** |number
| Audio channel number, default is 1 |
| **`isUrl`** |boolean
| Is the audio file a URL, pass true if assetPath is a `file://` url |#### Assets
| Prop | Type | Description |
| ------------- | ------------------- | --------------------------------------- |
| **`assetId`** |string
| Asset Id, unique identifier of the file |#### PluginListenerHandle
| Prop | Type |
| ------------ | ----------------------------------------- |
| **`remove`** |() => Promise<void>
|#### CompletedEvent
| Prop | Type | Description | Since |
| ------------- | ------------------- | -------------------------- | ----- |
| **`assetId`** |string
| Emit when a play completes | 5.0.0 |### Type Aliases
#### CompletedListener
(state: CompletedEvent): void