Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/EngRajabi/Enum.Source.Generator
A C# source generator to create an enumeration class from an enum type. With this package, you can work on enums very, very fast without using reflection.
https://github.com/EngRajabi/Enum.Source.Generator
codegeneration codegenerator csharp-sourcegenerator dotnet enum-fast enum-generator enumgenerator enums fast-enum roslyn roslyn-generator source-generator source-generators
Last synced: about 1 month ago
JSON representation
A C# source generator to create an enumeration class from an enum type. With this package, you can work on enums very, very fast without using reflection.
- Host: GitHub
- URL: https://github.com/EngRajabi/Enum.Source.Generator
- Owner: EngRajabi
- License: mit
- Created: 2022-05-26T13:24:37.000Z (over 2 years ago)
- Default Branch: master
- Last Pushed: 2024-11-01T01:35:43.000Z (about 1 month ago)
- Last Synced: 2024-11-02T15:38:26.374Z (about 1 month ago)
- Topics: codegeneration, codegenerator, csharp-sourcegenerator, dotnet, enum-fast, enum-generator, enumgenerator, enums, fast-enum, roslyn, roslyn-generator, source-generator, source-generators
- Language: C#
- Homepage:
- Size: 435 KB
- Stars: 179
- Watchers: 7
- Forks: 14
- Open Issues: 15
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
- RSCG_Examples - Enum.Source.Generator
- csharp-source-generators - Enum.Source.Generator -   A C# source generator to create an enumeration (enum) class from an enum type. With this package, you can work on enums very, very fast without using reflection. (Source Generators / Enums)
README
[](https://raw.githubusercontent.com/EngRajabi/Enum.Source.Generator/master/LICENSE)
[](https://www.nuget.org/packages/Supernova.Enum.Generators)
[](https://www.nuget.org/packages/Supernova.Enum.Generators)# Supernova.Enum.Generators
# The best Source Generator for working with enums in C#
A C# source generator to create an enumeration class from an enum type.
With this package, you can work on enums very, very fast without using reflection.**Package** - [Supernova.Enum.Generators](https://www.nuget.org/packages/Supernova.Enum.Generators/)
Add the package to your application using
```bash
dotnet add package Supernova.Enum.Generators
```Adding the package will automatically add a marker attribute, `[EnumGenerator]`, to your project.
To use the generator, add the `[EnumGenerator]` attribute to an enum. For example:
```csharp
[EnumGenerator]
public enum UserTypeTest
{
[Display(Name = "مرد", Description = "Descمرد")] Men = 3,[Display(Name = "زن", Description = "Descزن")] Women = 4,
//[Display(Name = "نامشخص")]
None
}
```This will generate a class called `EnumNameEnumExtensions` (`UserTypeTest` + `EnumExtensions`), which contains a number of helper methods.
For example:```csharp
///
/// Provides extension methods for operations related to the enumeration.
///
[GeneratedCodeAttribute("Supernova.Enum.Generators", null)]
public static class UserTypeTestEnumExtensions
{
///
/// Provides a dictionary that maps values to their corresponding display names.
///
public static readonly ImmutableDictionary DisplayNamesDictionary = new Dictionary
{
{UnitTests.UserTypeTest.Men, "مرد"},
{UnitTests.UserTypeTest.Women, "زن"},
{UnitTests.UserTypeTest.None, "None"},}.ToImmutableDictionary();
///
/// Provides a dictionary that maps values to their corresponding descriptions.
///
public static readonly ImmutableDictionary DisplayDescriptionsDictionary = new Dictionary
{
{UnitTests.UserTypeTest.Men, "Descمرد"},
{UnitTests.UserTypeTest.Women, "Descزن"},
{UnitTests.UserTypeTest.None, "None"},}.ToImmutableDictionary();
///
/// Converts the enumeration value to its string representation.
///
/// The enumeration value.
/// The default value to return if the enumeration value is not recognized.
/// The string representation of the value.
public static string ToStringFast(this UnitTests.UserTypeTest states, string defaultValue = null)
{
return states switch
{
UnitTests.UserTypeTest.Men => nameof(UnitTests.UserTypeTest.Men),
UnitTests.UserTypeTest.Women => nameof(UnitTests.UserTypeTest.Women),
UnitTests.UserTypeTest.None => nameof(UnitTests.UserTypeTest.None),
_ => defaultValue ?? throw new ArgumentOutOfRangeException(nameof(states), states, null)
};
}///
/// Checks if the specified value is defined.
///
/// The value to check.
/// True if the value is defined; otherwise, false.
public static bool IsDefinedFast(UnitTests.UserTypeTest states)
{
return states switch
{
UnitTests.UserTypeTest.Men => true,
UnitTests.UserTypeTest.Women => true,
UnitTests.UserTypeTest.None => true,
_ => false
};
}///
/// Checks if the specified string represents a defined value.
///
/// The string representing a value.
/// True if the string represents a defined value; otherwise, false.
public static bool IsDefinedFast(string states)
{
return states switch
{
nameof(UnitTests.UserTypeTest.Men) => true,
nameof(UnitTests.UserTypeTest.Women) => true,
nameof(UnitTests.UserTypeTest.None) => true,
_ => false
};
}///
/// Converts the enumeration value to its display string.
///
/// The enumeration value.
/// The default value to return if the enumeration value is not recognized.
/// The display string of the value.
public static string ToDisplayFast(this UnitTests.UserTypeTest states, string defaultValue = null)
{
return states switch
{
UnitTests.UserTypeTest.Men => "مرد",
UnitTests.UserTypeTest.Women => "زن",
UnitTests.UserTypeTest.None => "None",
_ => defaultValue ?? throw new ArgumentOutOfRangeException(nameof(states), states, null)
};
}///
/// Gets the description of the enumeration value.
///
/// The enumeration value.
/// The default value to return if the enumeration value is not recognized.
/// The description of the value.
public static string ToDescriptionFast(this UnitTests.UserTypeTest states, string defaultValue = null)
{
return states switch
{
UnitTests.UserTypeTest.Men => "Descمرد",
UnitTests.UserTypeTest.Women => "Descزن",
UnitTests.UserTypeTest.None => "None",
_ => defaultValue ?? throw new ArgumentOutOfRangeException(nameof(states), states, null)
};
}///
/// Retrieves an array of all enumeration values.
///
/// An array containing all enumeration values.
public static UnitTests.UserTypeTest[] GetValuesFast()
{
return new[]
{
UnitTests.UserTypeTest.Men,
UnitTests.UserTypeTest.Women,
UnitTests.UserTypeTest.None,
};
}///
/// Retrieves an array of strings containing the names of all enumeration values.
///
/// An array of strings containing the names of all enumeration values.
public static string[] GetNamesFast()
{
return new[]
{
nameof(UnitTests.UserTypeTest.Men),
nameof(UnitTests.UserTypeTest.Women),
nameof(UnitTests.UserTypeTest.None),
};
}///
/// Gets the length of the enumeration.
///
/// The length of the enumeration.
public static int GetLengthFast()
{
return 3;}
///
/// Try parse a string to value.
///
/// The string representing a value.
/// The enum parse result.
/// True if the string is parsed successfully; otherwise, false.
public static bool TryParseFast(string states, out UnitTests.UserTypeTest result)
{
switch (states)
{
case "Men":
{
result = UnitTests.UserTypeTest.Men;
return true;
}
case "Women":
{
result = UnitTests.UserTypeTest.Women;
return true;
}
case "None":
{
result = UnitTests.UserTypeTest.None;
return true;
}
default: {
result = default;
return false;
}
}
}
}
```You do not see this file inside the project. But you can use it.
Usage
```csharp
var stringEnum = UserTypeTest.Men.ToStringFast(); //Men;var isDefined = UserTypeTestEnumExtensions.IsDefinedFast(UserType.Men); //true;
var displayEnum = UserTypeTest.Men.ToDisplayFast(); //مرد
var descriptionEnum = UserTypeTest.Men.ToDescriptionFast(); //Descمرد
var names = UserTypeTestEnumExtensions.GetNamesFast(); //string[]
var values = UserTypeTestEnumExtensions.GetValuesFast(); //UserType[]
var length = UserTypeTestEnumExtensions.GetLengthFast(); //3
var menString = "Men";
var result = UserTypeTestEnumExtensions.TryParseFast(menString, out var enumValue);
```If you had trouble using UserTypeTestEnumExtensions and the IDE did not recognize it. This is an IDE problem and you need to restart the IDE once.
Benchmark
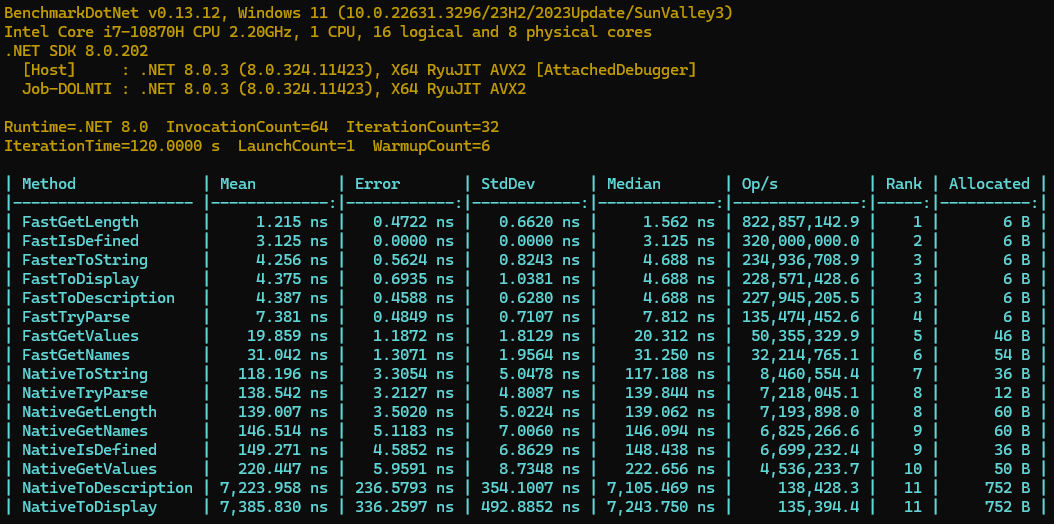
## Contributing
Create an [issue](https://github.com/EngRajabi/Enum.Source.Generator/issues/new) if you find a BUG or have a Suggestion or Question. If you want to develop this project :
1. Fork it!
2. Create your feature branch: `git checkout -b my-new-feature`
3. Commit your changes: `git commit -am 'Add some feature'`
4. Push to the branch: `git push origin my-new-feature`
5. Submit a pull request## Give a Star! ⭐️
If you find this repository useful, please give it a star. Thanks!
## License
Supernova.Enum.Generators is Copyright © 2022 [Mohsen Rajabi](https://github.com/EngRajabi) under the MIT License.