Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/FaridSafi/react-native-gifted-form
๐ ยซ One React-Native form component to rule them all ยป
https://github.com/FaridSafi/react-native-gifted-form
Last synced: about 1 month ago
JSON representation
๐ ยซ One React-Native form component to rule them all ยป
- Host: GitHub
- URL: https://github.com/FaridSafi/react-native-gifted-form
- Owner: FaridSafi
- License: mit
- Created: 2015-11-03T12:37:07.000Z (about 9 years ago)
- Default Branch: master
- Last Pushed: 2020-08-17T02:05:49.000Z (over 4 years ago)
- Last Synced: 2024-10-29T20:56:27.482Z (3 months ago)
- Language: JavaScript
- Homepage:
- Size: 1.24 MB
- Stars: 1,443
- Watchers: 33
- Forks: 214
- Open Issues: 80
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
- awesome-react-native - react-native-gifted-form โ 1317 - Form component for react-native (Components / Forms)
- awesome-list - react-native-gifted-form - Native form component to rule them all ยป | FaridSafi | 1447 | (JavaScript)
- awesome-react-native - react-native-gifted-form โ 1317 - Form component for react-native (Components / Forms)
- awesome-react-native - react-native-gifted-form - Form component for react-native.  (Components / Forms)
- awesome-react-native - react-native-gifted-form โ 1317 - Form component for react-native (Components / Forms)
- awesome-react-native-ui - react-native-gifted-form โ 699 - Form component for react-native (Components / Forms)
- awesome-react-native - react-native-gifted-form โ 1317 - Form component for react-native (Components / Forms)
- awesome-react-native - react-native-gifted-form
- ReactNativeMaterials - react-native-gifted-form
README
# Gifted Form
[](https://www.npmjs.com/package/react-native-gifted-form)
[](https://www.npmjs.com/package/react-native-gifted-form)
[](https://github.com/FaridSafi/react-native-gifted-form)Form component for React Native.
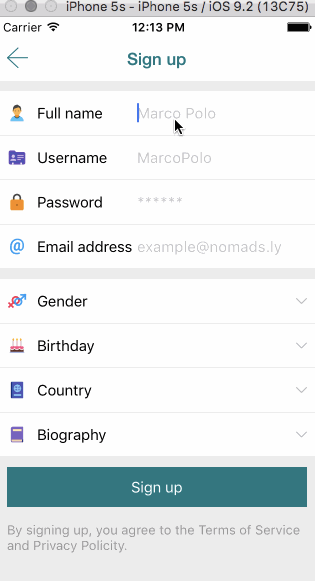
### Example
```js
var { GiftedForm, GiftedFormManager } = require('react-native-gifted-form');var FormComponent = createReactClass({
render() {
return (
{
navigator.push(route); // The ModalWidget will be opened using this method. Tested with ExNavigator
}}
clearOnClose={false} // delete the values of the form when unmounted
defaults={{
/*
username: 'Farid',
'gender{M}': true,
password: 'abcdefg',
country: 'FR',
birthday: new Date(((new Date()).getFullYear() - 18)+''),
*/
}}
validators={{
fullName: {
title: 'Full name',
validate: [{
validator: 'isLength',
arguments: [1, 23],
message: '{TITLE} must be between {ARGS[0]} and {ARGS[1]} characters'
}]
},
username: {
title: 'Username',
validate: [{
validator: 'isLength',
arguments: [3, 16],
message: '{TITLE} must be between {ARGS[0]} and {ARGS[1]} characters'
},{
validator: 'matches',
arguments: /^[a-zA-Z0-9]*$/,
message: '{TITLE} can contains only alphanumeric characters'
}]
},
password: {
title: 'Password',
validate: [{
validator: 'isLength',
arguments: [6, 16],
message: '{TITLE} must be between {ARGS[0]} and {ARGS[1]} characters'
}]
},
emailAddress: {
title: 'Email address',
validate: [{
validator: 'isLength',
arguments: [6, 255],
},{
validator: 'isEmail',
}]
},
bio: {
title: 'Biography',
validate: [{
validator: 'isLength',
arguments: [0, 512],
message: '{TITLE} must be between {ARGS[0]} and {ARGS[1]} characters'
}]
},
gender: {
title: 'Gender',
validate: [{
validator: (...args) => {
if (args[0] === undefined) {
return false;
}
return true;
},
message: '{TITLE} is required',
}]
},
birthday: {
title: 'Birthday',
validate: [{
validator: 'isBefore',
arguments: [moment().utc().subtract(18, 'years').format('YYYY-MM-DD')],
message: 'You must be at least 18 years old'
}, {
validator: 'isAfter',
arguments: [moment().utc().subtract(100, 'years').format('YYYY-MM-DD')],
message: '{TITLE} is not valid'
}]
},
country: {
title: 'Country',
validate: [{
validator: 'isLength',
arguments: [2],
message: '{TITLE} is required'
}]
},
}}
>
{
if (!currentText) {
let fullName = GiftedFormManager.getValue('signupForm', 'fullName');
if (fullName) {
return fullName.replace(/[^a-zA-Z0-9-_]/g, '');
}
}
return currentText;
}}
/>
{
return new Date(((new Date()).getFullYear() - 18)+'');
}}
/>
{
if (isValid === true) {
// prepare object
values.gender = values.gender[0];
values.birthday = moment(values.birthday).format('YYYY-MM-DD');/* Implement the request to your server using values variable
** then you can do:
** postSubmit(); // disable the loader
** postSubmit(['An error occurred, please try again']); // disable the loader and display an error message
** postSubmit(['Username already taken', 'Email already taken']); // disable the loader and display an error message
** GiftedFormManager.reset('signupForm'); // clear the states of the form manually. 'signupForm' is the formName used
*/
}
}}
/>
);
}
});
```### Storing form's state elsewhere (could be used with Redux) - Beta feature
Pass `value` prop to your widgets and `onValueChange` to your GiftedForm to store your state outside of GiftedFormManager's store.
IMPORTANT: currently only TextInputWidget and HiddenWidget support this feature. PR's are welcome for the other widgets ;)
```js
import React, { AppRegistry, Component } from 'react-native'
import { GiftedForm, GiftedFormManager } from 'react-native-gifted-form'class Form extends Component {
constructor(props, context) {
super(props, context)
this.state = {
form: {
fullName: 'Marco Polo',
tos: false,
}
}
}handleValueChange(values) {
console.log('handleValueChange', values)
this.setState({ form: values })
}render() {
const { fullName, tos, gender } = this.state.form
console.log('render', this.state.form)
return (
{ this.props.navigator.push(route) }}
onValueChange={this.handleValueChange.bind(this)}
>
)
}
}AppRegistry.registerComponent('Form', () => Form)
```### Installation
```console
npm install react-native-gifted-form --save
# OR
yarn add react-native-gifted-form
```### Available widgets
- TextInputWidget - A text input
- TextAreaWidget - A text area
- GooglePlacesWidget - A Google Places picker based on react-native-google-places-autocomplete
- ModalWidget - A route opener for nested forms
- GroupWidget - A widgets container with a title
- HiddenWidget - A non-displayed widget. The value will be passed to SubmitWidget
- LoadingWidget - A loader
- RowWidget - A touchable row with title/image
- RowValueWidget - A touchable row with title/image and a value
- SelectCountryWidget - A country picker. Flags made by www.IconDrawer.com
- SelectWidget - A select menu
- SeparatorWidget - A 10px widgets separator
- SubmitWidget - A submit button that trigger form validators and error displaying
- SwitchWidget - A switch
- DatePickerIOSWidget - Date picker for iOS
- NoticeWidget - A notice information - PR wanted for onPress handlerSee the [widget sources](https://github.com/FaridSafi/react-native-gifted-form/tree/master/widgets) for full props details.
### Custom widgets
Widgets must implement the mixin `GiftedForm.WidgetMixin`. See [TextAreaWidget](https://github.com/FaridSafi/react-native-gifted-form/blob/master/widgets/TextAreaWidget.js) for a good example.
### Contributing
Pull requests are welcome! The author is very busy at the moment but there are also some contributors who are also helping out.
### License
[MIT](LICENSE)
Feel free to ask me questions on Twitter [@FaridSafi](https://www.twitter.com/FaridSafi)!