https://github.com/JHBitencourt/timeline_tile
A package to help build customisable timelines in Flutter.
https://github.com/JHBitencourt/timeline_tile
Last synced: about 1 month ago
JSON representation
A package to help build customisable timelines in Flutter.
- Host: GitHub
- URL: https://github.com/JHBitencourt/timeline_tile
- Owner: JHBitencourt
- License: mit
- Created: 2020-06-06T20:50:25.000Z (almost 5 years ago)
- Default Branch: master
- Last Pushed: 2024-08-02T08:33:22.000Z (9 months ago)
- Last Synced: 2025-03-01T00:57:55.400Z (2 months ago)
- Language: Dart
- Homepage: https://pub.dev/packages/timeline_tile
- Size: 14.9 MB
- Stars: 766
- Watchers: 5
- Forks: 149
- Open Issues: 26
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE
Awesome Lists containing this project
- my-awesome-list - timeline_tile
- awesome-flutter-cn - Timeline Tile - 用于构建美观和可自定义时间轴的瓷砖小部件,由[Julio Bitencourt](https://github.com/JHBitencourt)提供。 (组件 / UI)
- awesome-flutter - Timeline Tile - A package to help build customisable timelines in Flutter. ` 📝 4 months ago ` (UI [🔝](#readme))
- awesome-flutter - Timeline Tile - Tile to help build beautiful and customisable timelines by [Julio Bitencourt](https://github.com/JHBitencourt). (Components / UI)
- awesome-flutter-cn - Timeline Tile - 一个用于构建精美可自定义的时间轴的工具库,[Julio Bitencourt](https://github.com/JHBitencourt). (组件 / UI)
- fucking-awesome-flutter - Timeline Tile - Tile to help build beautiful and customisable timelines by [Julio Bitencourt](https://github.com/JHBitencourt). (Components / UI)
README
# TimelineTile
A package to help build customisable timelines in Flutter.
---
## Example
- You can access the [example](https://github.com/JHBitencourt/timeline_tile/tree/master/example) project for a Timeline Showcase.
- The [Beautiful Timelines](https://github.com/JHBitencourt/beautiful_timelines) contains real world design examples.
- Or try the [web demo](https://jhbitencourt.github.io/beautiful-timelines)Some use cases:
![]()
Timeline Showcase
![]()
Football Timeline
![]()
Activity Timeline
![]()
Success Timeline
![]()
Delivery Timeline
![]()
Weather Timeline
![]()
Horizontal Timelines
## Getting Started
A Timeline consists in a group of TimelineTiles. To build a tile you can simply use:
```dart
TimelineTile()
```Which will build a default tile with a `vertical` axis, that aligns to the start, with a `height` of 100:
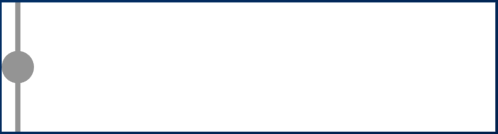
The axis can be switched to render an `horizontal` tile, aligned to the start, with a default `width` of 100:
```dart
TimelineTile(axis: TimelineAxis.horizontal)
```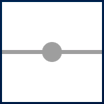
There are 4 types of alignment.
- `TimelineAlign.start`
- `TimelineAlign.end`
- `TimelineAlign.center`
- `TimelineAlign.manual`The `start` and `end` alignment allows a child in their opposite sides. On the other hand, both `center` and `manual` allows children on both sides. For example, one tile with alignment to the center:
```dart
TimelineTile(
alignment: TimelineAlign.center,
endChild: Container(
constraints: const BoxConstraints(
minHeight: 120,
),
color: Colors.lightGreenAccent,
),
startChild: Container(
color: Colors.amberAccent,
),
);
```When providing children to the `vertical` tile, the height will be as minimum as possible, so you can control it with a height constraint (at least minHeight). This way the tile knows how to size it properly.
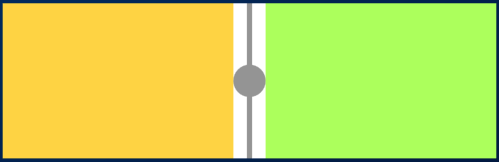
If the `axis` is `horizontal`, the things are the opposite. The width will be as minimum as possible, so you can control it with a width constraint (at least minWidth). This way the tile knows how to size it properly.
```dart
TimelineTile(
axis: TimelineAxis.horizontal,
alignment: TimelineAlign.center,
endChild: Container(
constraints: const BoxConstraints(
minWidth: 120,
),
color: Colors.lightGreenAccent,
),
startChild: Container(
color: Colors.amberAccent,
),
);
```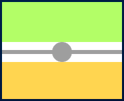
### Manual aligning the idicator
With `TimelineAlign.manual` you can provide the `lineXY`, which allows you to specify a value from 0.0 to 1.0, that represents a size percentage. For example, aligning at 30% of the width or height:
```dart
TimelineTile(
alignment: TimelineAlign.manual,
lineXY: 0.3,
endChild: Container(
constraints: const BoxConstraints(
minHeight: 120,
),
color: Colors.lightGreenAccent,
),
startChild: Container(
color: Colors.amberAccent,
),
);
```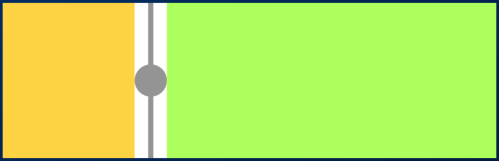
```dart
TimelineTile(
axis: TimelineAxis.horizontal,
alignment: TimelineAlign.manual,
lineXY: 0.3,
endChild: Container(
constraints: const BoxConstraints(
minWidth: 120,
),
color: Colors.lightGreenAccent,
),
startChild: Container(
color: Colors.amberAccent,
),
);
```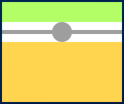
### Is it the first or the last?
You can decide if a tile is the first os the last in a timeline. This way you control whether a `before` or `after` line must be rendered.
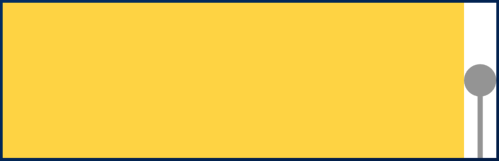
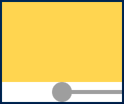
See the implementation [here](https://github.com/JHBitencourt/timeline_tile/blob/master/example/lib/src/example/example_4.dart)
### Start to make a timeline
You can finally start to combine some tiles to make a Timeline. The flag `hasIndicator` can control whether an indicator should or shouldn't be rendered.
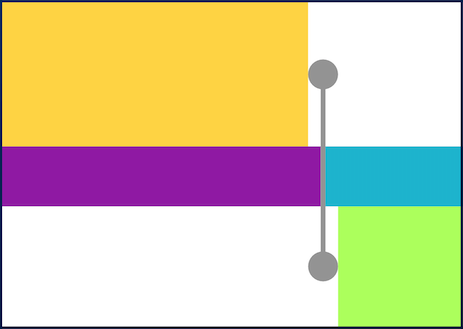
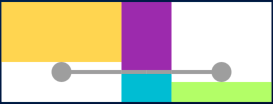
See the implementation [here](https://github.com/JHBitencourt/timeline_tile/blob/master/example/lib/src/example/example_5.dart)
### Customize the indicator as you wish
The default indicator is a circle, and you can customize it as you wish. With `IndicatorStyle` you can change the color, the X/Y position based on values from 0.0 to 1.0 or give it a padding. You must explicitly provide its width (vertical) or height (horizontal) though.
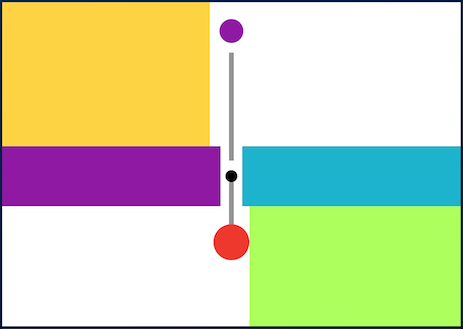
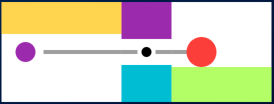
See the implementation [here](https://github.com/JHBitencourt/timeline_tile/blob/master/example/lib/src/example/example_6.dart)
### Give an icon to the indicator
With `IconStyle` you can provide an Icon to be rendered inside the default indicator.


See the implementation [here](https://github.com/JHBitencourt/timeline_tile/blob/master/example/lib/src/example/example_7.dart)
### Or provide your custom indicator
With the indicator parameter you can customize the tile with your own indicator. However, you must control its size through both width and height parameters.
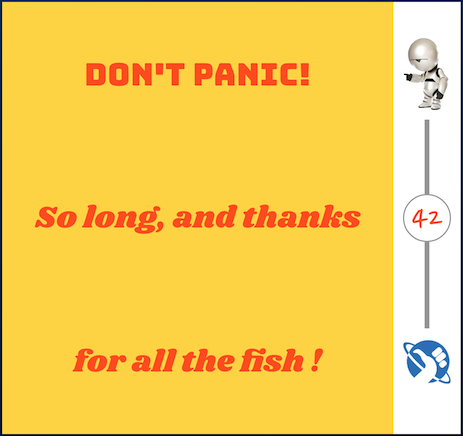
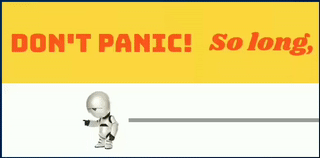
See the implementation [here](https://github.com/JHBitencourt/timeline_tile/blob/master/example/lib/src/example/example_8.dart)
### Customize the tile's line
With `LineStyle` you can customize both `beforeLine` and `afterLine`.
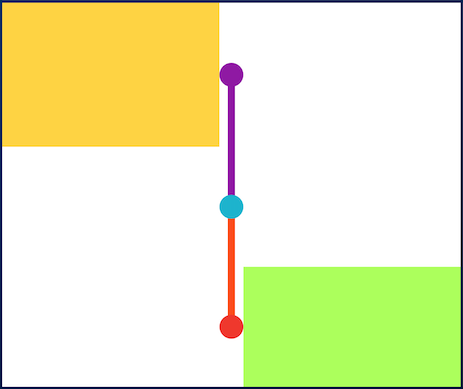

See the implementation [here](https://github.com/JHBitencourt/timeline_tile/blob/master/example/lib/src/example/example_9.dart)
### Connect tiles with TimelineDivider
The `TimelineDivider` widget allows you to connect tiles that are aligned in different X/Y axis, when combined with `TimelineAlign.manual`.

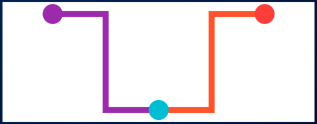
See the implementation [here](https://github.com/JHBitencourt/timeline_tile/blob/master/example/lib/src/example/example_10.dart)