Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/OpenFlutter/mini_calendar
Date component developed with Flutter, plans to support display, swipe left and right, add date mark, radio, display week, etc.
https://github.com/OpenFlutter/mini_calendar
calender dart flutter package
Last synced: 4 months ago
JSON representation
Date component developed with Flutter, plans to support display, swipe left and right, add date mark, radio, display week, etc.
- Host: GitHub
- URL: https://github.com/OpenFlutter/mini_calendar
- Owner: OpenFlutter
- License: other
- Created: 2019-12-09T06:21:12.000Z (about 5 years ago)
- Default Branch: master
- Last Pushed: 2024-06-24T13:08:30.000Z (8 months ago)
- Last Synced: 2024-08-01T12:19:34.984Z (7 months ago)
- Topics: calender, dart, flutter, package
- Language: Dart
- Homepage: https://pub.flutter-io.cn/packages/mini_calendar
- Size: 3.71 MB
- Stars: 113
- Watchers: 4
- Forks: 18
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE
Awesome Lists containing this project
README
## mini_calendar
[](https://pub.flutter-io.cn/packages/mini_calendar) 
Date component developed with Flutter, plans to support display, swipe left and right, add date mark, radio, display week, etc.
使用Flutter开发的日期组件,计划支持显示,左右滑动,添加日期标记,单选,显示星期等功能。
- [更新记录](CHANGELOG.md)
### 主要想实现的内容

## 使用
### 引入库```dart
dependencies:
mini_calendar: ^2.0.0
```### 导包
```dart
import 'package:mini_calendar/mini_calendar.dart';
```### 月视图(MonthWidget)
```dart
MonthWidget();// 默认当月
```- 可通过控制器参数来控制显示的月份以及选择的日期
```dart
MonthWidget(
controller: MonthController.init(
MonthOption(
currentDay: DateDay.now().copyWith(month: index + 1, day: Random().nextInt(27) + 1),
currentMonth: DateMonth.now().copyWith(month: index + 1),
)
),
)
```- 支持显示连选
```dart
MonthWidget(
controller: MonthController.init(MonthOption(
currentMonth: DateMonth.now().copyWith(month: 1),
enableContinuous: true,
firstSelectDay: DateDay.now().copyWith(month: 1, day: 8),
secondSelectDay: DateDay.now().copyWith(month: 1, day: 18),
)),
)
```
- 支持多选```dart
MonthWidget(
controller: MonthController.init(MonthOption(
currentMonth: DateMonth.now().copyWith(month: 1),
enableMultiple: true,
multipleDays: [
DateDay.now().copyWith(month: 1, day: 3),
DateDay.now().copyWith(month: 1, day: 5),
DateDay.now().copyWith(month: 1, day: 8),
],
)),
)
```- 支持添加标记
- ……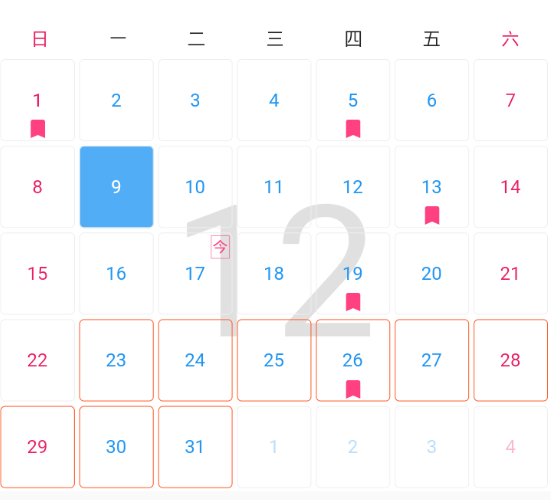
### 滚动日历(MonthPageView)
> 控制器需要创建后获取 `onCreated`
```dart
MonthPageView(
padding: EdgeInsets.all(1),
scrollDirection: Axis.horizontal,// 水平滑动或者竖直滑动
option: MonthOption(
enableContinuous: true,// 单选、连选控制
marks: {
DateDay.now().copyWith(day: 1): '111',
DateDay.now().copyWith(day: 5): '222',
DateDay.now().copyWith(day: 13): '333',
DateDay.now().copyWith(day: 19): '444',
DateDay.now().copyWith(day: 26): '444',
},
),
showWeekHead: true, // 显示星期头部
onContinuousSelectListen: (DateDay?firstDay,DateDay?endFay) {
},// 连选回调
onMultipleSelectListen: (list) {
},// 多选回调
onMonthChange: (month) {
},// 月份更改回调
onDaySelected: (DateDay day, T? markData, bool enable) {
// enable : 是否是可选日期
},// 日期选中会迪欧啊
onCreated: (controller){
}, // 控制器回调
onClear: () {
}// 点击清空按钮,设置为空时不显示清空按钮
),
```### 控制器
#### 参数初始化
```dart
MonthOption({
DateDay currentDay,//选择的日期
DateMonth currentMonth,//当前月份
int firstWeek = 7,//第一列显示的星期 [1,7]
DateDay firstSelectDay,//连选第一个日期
DateDay secondSelectDay,//连选第二个日期
bool enableContinuous = false,//是否支持连选
Map marks = const {},//标记
List? multipleDays, // 多选日期
List? enableDays, // 能选择的日期集合
bool enableMultiple = false,//是否支持多选
DateDay minDay,//可选的最小日期
DateDay maxDay,//可选的最大日期
});
```
#### 注销
```dart
MonthPageController#dispose();
```
#### 更新
```dart
MonthPageController#reLoad();
```
#### 下一月
```dart
MonthPageController#next();
```
#### 上一月
```dart
MonthPageController#last();
```
#### 跳转到指定月份
```dart
MonthPageController#goto(DateMonth month);
```
### 高级功能
> 自定义#### 自定义月视图背景
```dart
buildMonthBackground: (_, width, height, month) => Image.network(
'https://ssyerv1.oss-cn-hangzhou.aliyuncs.com/picture/b0c57bd90abd49d59920924010ab66a9.png!sswm',
height: height,
width: width,
fit: BoxFit.cover,
),
```#### 自定义月视图头部
```dart
buildMonthHead: (ctx, width, height, month) => Container(
padding: EdgeInsets.all(5),
child: Row(
mainAxisAlignment: MainAxisAlignment.start,
children: [
Text(
"${month.year}年",
style: TextStyle(fontSize: 40, color: Colors.white),
),
Container(
margin: EdgeInsets.only(left: 5, right: 5),
width: 1,
color: Colors.yellow,
height: 50,
),
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
"${month.month}月",
style: TextStyle(fontSize: 18, color: Colors.orange),
),
Text("这是一个自定义的月头部"),
],
)
],
),
),
```- 自定义星期头部
- 自定义日视图
- ……|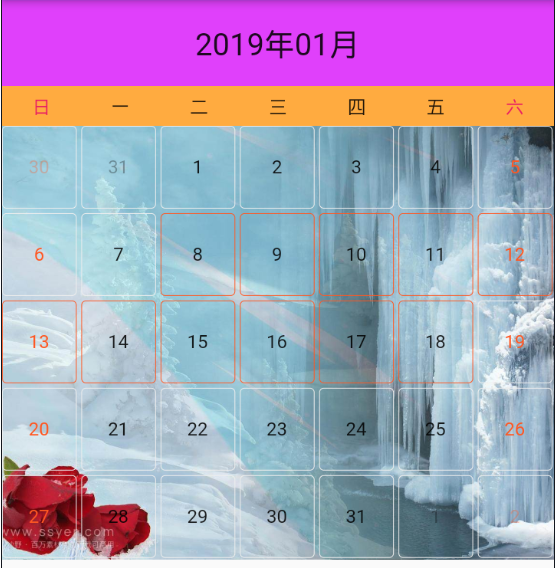|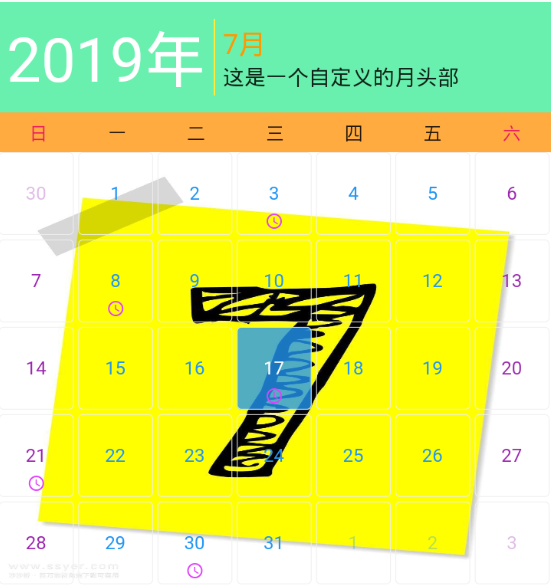|
| :---: | :---: |> 更多功能clone项目,运行demo
### 开源不易,老铁们多多支持,点赞也是支持 😃 !