Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/RafalFilipek/styled-props
Simple lib that allows you to set styled props in your styled-components without stress.
https://github.com/RafalFilipek/styled-props
css-in-js react styled-components styled-props
Last synced: 23 days ago
JSON representation
Simple lib that allows you to set styled props in your styled-components without stress.
- Host: GitHub
- URL: https://github.com/RafalFilipek/styled-props
- Owner: RafalFilipek
- License: mit
- Archived: true
- Created: 2017-01-10T09:15:48.000Z (about 8 years ago)
- Default Branch: master
- Last Pushed: 2019-08-13T19:53:54.000Z (over 5 years ago)
- Last Synced: 2024-12-17T19:58:23.786Z (27 days ago)
- Topics: css-in-js, react, styled-components, styled-props
- Language: JavaScript
- Homepage: https://github.com/RafalFilipek/styled-props
- Size: 1.64 MB
- Stars: 241
- Watchers: 5
- Forks: 9
- Open Issues: 16
-
Metadata Files:
- Readme: README.md
- License: LICENSE
- Code of conduct: CODE_OF_CONDUCT.md
Awesome Lists containing this project
README
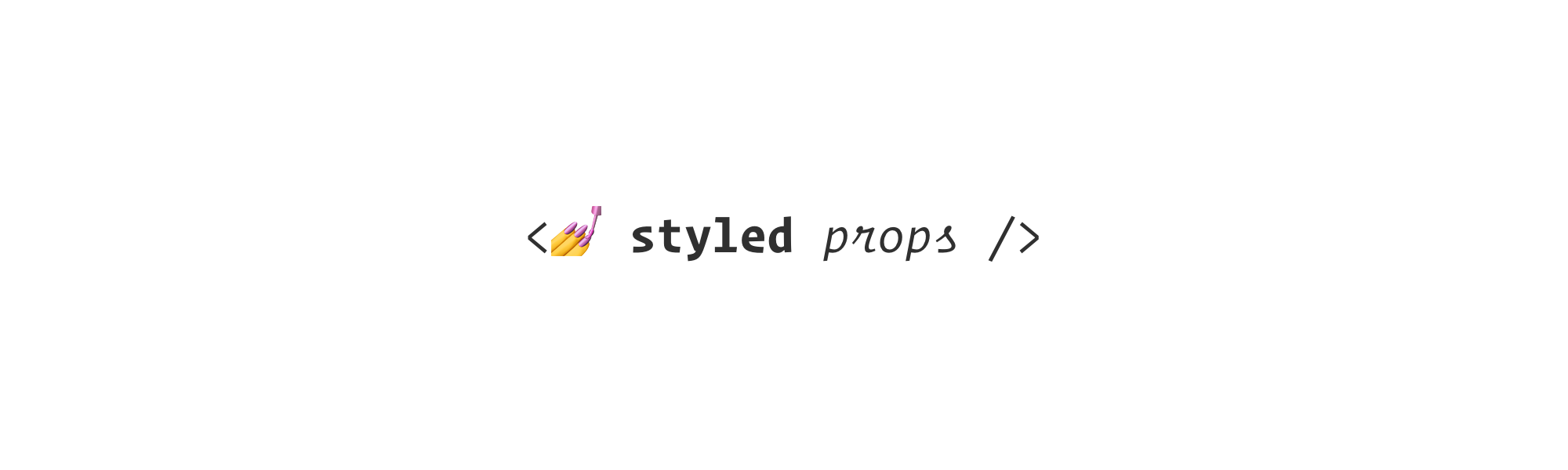
---
[](https://badge.fury.io/js/styled-props)
[](https://greenkeeper.io/)
[](https://travis-ci.org/RafalFilipek/styled-props)
[](https://codecov.io/gh/RafalFilipek/styled-props)

[](https://github.com/prettier/prettier)Simple lib that allows you to set *styled props* in your [*styled-components*](https://styled-components.com) without stress. Let's take `Button` component from styled-components web page. Here it is:
```jsx
const Button = styled.button`
font-size: 1em;
margin: 1em;
padding: 0.25 em 1em;
border: 2px solid palevioletred;
border radius: 3px;background: ${props => props.primary && 'palevioletred'}
color: ${props => props.primary ? 'white' : 'palevioletred'}
`;
```Now you can simply write
```jsx
Hello or World!
```But your application is probably much bigger than single button. And you want to keep your colors, sizes etc. in one place. So let's create simple `styles.js` file.
```js
// styles.jsexport const background = {
primary: '#F5F5F5',
danger: '#DD2C00',
success: '#7CB342',
info: '#BBDEFB',
};export const color = {
primary: '#263238',
default: '#FAFAFA',
};export const size = {
padding: {
small: 10,
medium: 20,
big: 30,
},
borderRadius: {
small: 5,
default: 10,
},
};
```> `styles.js` file is cool because you can access them anywhere! You can also generate some style guides and of course keep all information in one place.
> **IMPORTANT** It is better to use singular forms for keys. In `bind` mode keys are used to set fallbacks so `color` is better than `colors` as a prop.
So how can I help? `styled-props` package exports single function called `styledProps`. You can use it in all your components.
### TL;DR; Examples
- [Basic usage](https://codesandbox.io/s/n5X21gBYR)
- [Default props](https://codesandbox.io/s/2k6VL6wgz)
- [Bind](https://codesandbox.io/s/gJxQvB1Bk)### Installation
```
yarn add styled-props// or
npm install styled-props
```### Basic usage
```jsx
import styledProps from 'styled-props';
import styled from 'styled-components';
import {
background,
color,
size,
} from './styles.js';const Button = styled.button`
background: ${styledProps(background)};
color: ${styledProps(color)};
padding: ${styledProps(size.padding)}px;
border-radius: ${styledProps(size.borderRadius)}px;font-size: 1em;
margin: 1em;
border: 2px solid palevioletred;
`;export default () => (
This
is
so
cool!
)
```As you can see each prop can be mapped into specific value for selected css rule. If you need another combination, you just add it in `styles.js`.
### Default values
Everything is based on props. As we know in React you can set `defaultProps` for each component. You can also use them to set default values for styles. For example:
```jsx
const Button = styled.button`
color: ${styledProps(color, 'color')}
`;
Button.defaultProps = {
color: 'default',
};
```If you will not provide `primary` or `default` property for Button component `styledProps` function will check value of `color` property and use it as a key in `color` map. In our case default color is `color.white`. This is quite cool because you can also set styles the old way:
```jsx
```
### Bind
When your component is full of dynamic styles you can ( from `v0.1.0`) use `bind` options to simplify things.
```js
//styles.js
export default {
color: {
red: '#990000',
white: '#ffffff',
black: '#000000',
},
size: {
small: 10,
medium: 20,
big: 30,
}
}
``````jsx
import styles from './styles';
import { bindStyles } from 'styled-props';// or alternatively
// import { bind } from 'styled-props';
s = bindStyles(styles);
export default styled.button`
color: ${s.color};
padding: ${s.size};
`;
```> As you can see `bind` is available as `bind` or `bindStyles` in case you're using lodash or any other library to for e.g bind functions context.
Each key in `s` provides `styledProps` function. Also default value is set automaticly with `key` from map.
```
s.color === styledProps(styles.color, 'color');
```### API
```
styledProps(stylesMap:Object, [fallbackKey]:string)styledPropsBind(collectionsMap:Object)
```