Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/abassel/flask-restglue
๐ Integrates Flask + MongoDB(mongoengine) + OpenAPI in a simple and elegant way!
https://github.com/abassel/flask-restglue
flask flask-restglue mongoengine openapi openapi3 python-3 python3 rest
Last synced: 11 days ago
JSON representation
๐ Integrates Flask + MongoDB(mongoengine) + OpenAPI in a simple and elegant way!
- Host: GitHub
- URL: https://github.com/abassel/flask-restglue
- Owner: abassel
- License: mit
- Created: 2021-01-03T18:56:36.000Z (about 4 years ago)
- Default Branch: main
- Last Pushed: 2025-01-13T02:31:40.000Z (about 1 month ago)
- Last Synced: 2025-01-31T02:36:22.982Z (20 days ago)
- Topics: flask, flask-restglue, mongoengine, openapi, openapi3, python-3, python3, rest
- Language: Python
- Homepage: https://abassel.github.io/Flask-RestGlue
- Size: 2.73 MB
- Stars: 2
- Watchers: 2
- Forks: 0
- Open Issues: 17
-
Metadata Files:
- Readme: README.md
- Contributing: CONTRIBUTING.md
- License: LICENSE
- Security: SECURITY.md
Awesome Lists containing this project
README
Flask-RestGlue(ALPHA)
Integrates Flask + MongoDB + OpenAPI in a simple and elegant way!
Explore the docs ยป
View Demo
ยท
Report Bug
ยท
Request Feature
## Contents
Table of Contents
# Example
For a fullstack boilerplate, visit [https://github.com/abassel/Flask_RestGlue_Svelte_Docker](https://github.com/abassel/Flask_RestGlue_Svelte_Docker)
```python
import mongoengine as mongo
from flask_rest_glue import FlaskRestGluemongo.connect("pyglue", host='localhost:27017')
api = FlaskRestGlue()
@api.rest_model()
class User(mongo.Document):
# id = mongo.StringField(primary_key=True)
email = mongo.StringField(primary_key=True)
password = mongo.StringField()api.run()
```
Go to [http://127.0.0.1:5000/spec_doc](http://127.0.0.1:5000/spec_doc) or [http://127.0.0.1:5000/spec_rdoc](http://127.0.0.1:5000/spec_rdoc) to see the documentation below:
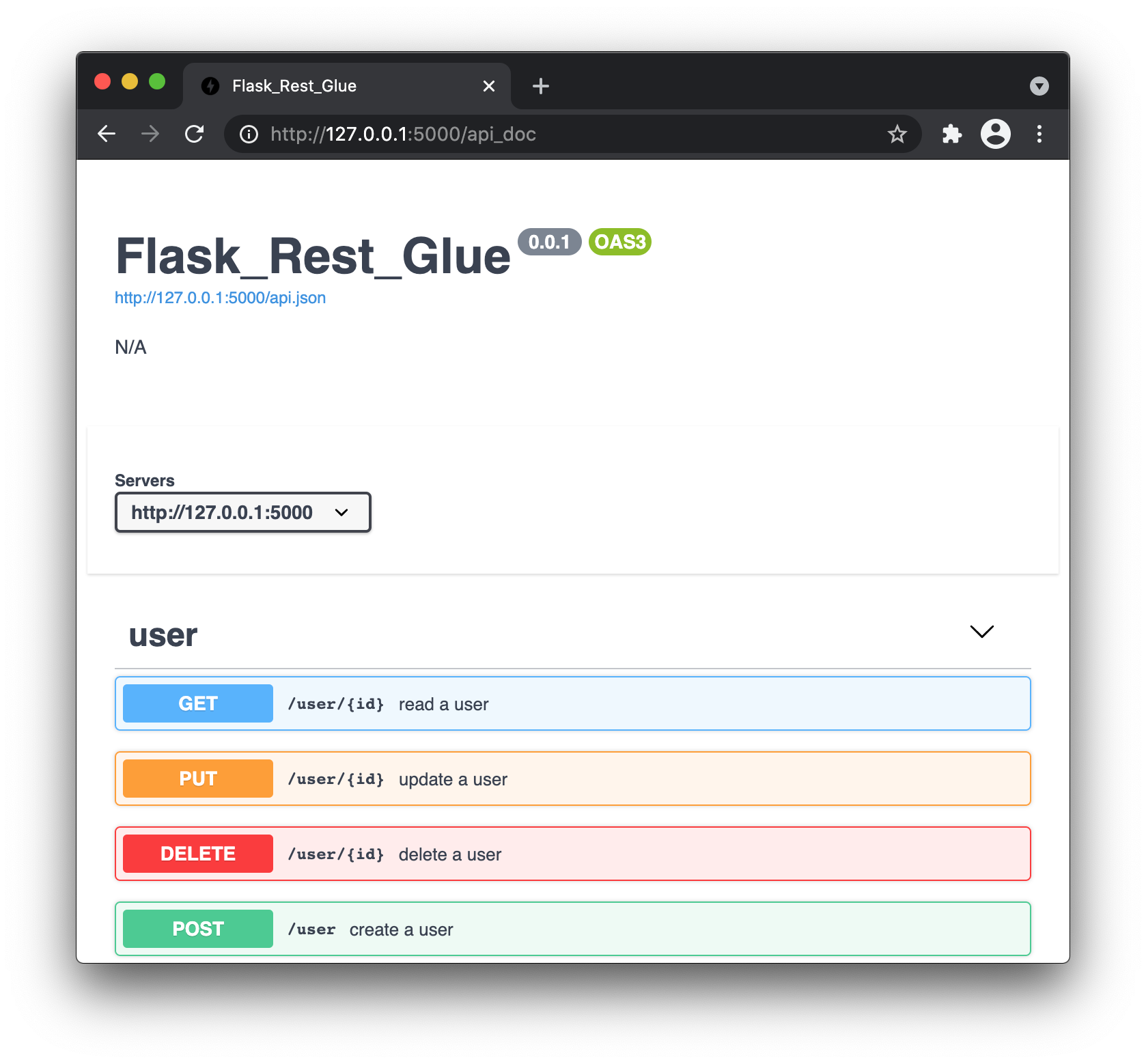
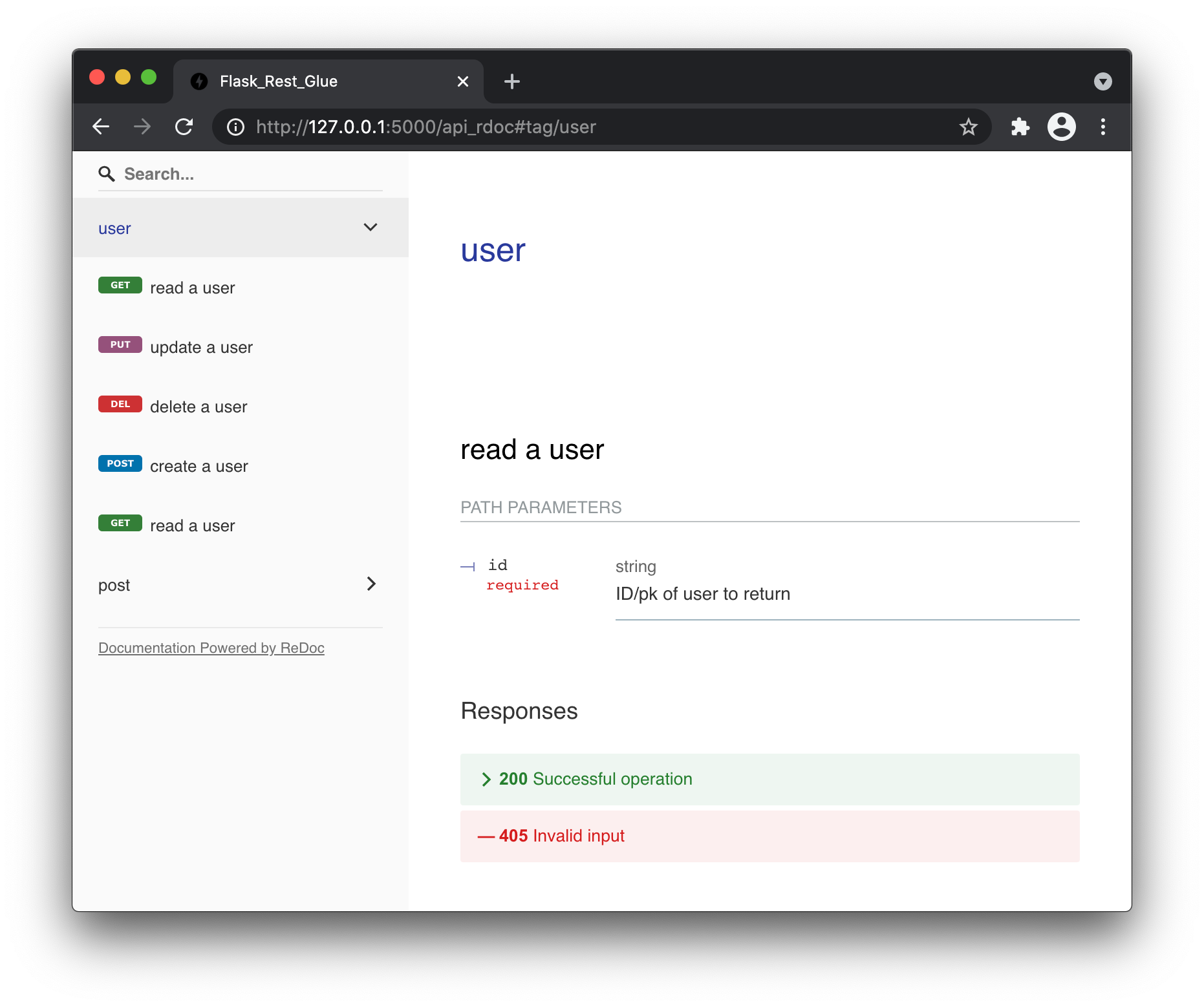
### Expected output:
```bash
curl -v -d '{"email":"[email protected]","password":"xyz"}' \
-H "Content-Type: application/json" http://localhost:5000/user#< HTTP/1.0 200 OK
#< Content-Type: application/json
#< Content-Length: 45
#<
#{
# "_id": "[email protected]",
# "password": "xyz"
#}curl -v http://localhost:5000/users
#< HTTP/1.0 200 OK
#< Content-Type: application/json
#< Content-Length: 57
#<
#[
# {
# "_id": "[email protected]",
# "password": "xyz"
# }
#]curl -v http://localhost:5000/user/[email protected]
#< HTTP/1.0 200 OK
#< Content-Type: application/json
#< Content-Length: 45
#<
#{
# "_id": "[email protected]",
# "password": "xyz"
#}curl -v -X PUT -d '{"password":"new_pass"}' \
-H "Content-Type: application/json" http://localhost:5000/user/[email protected]
#< HTTP/1.0 200 OK
#< Content-Type: application/json
#< Content-Length: 50
#<
#{
# "_id": "[email protected]",
# "password": "new_pass"
#}curl -v -X DELETE http://localhost:5000/user/[email protected]
#< HTTP/1.0 200 OK
#< Content-Type: application/json
#< Content-Length: 45
#<
#{
# "_id": "[email protected]",
# "password": "xyz"
#}
```# Quick Start
Requires **docker** and **python 3.9**
### 1 - install local MongoDB
```bash
mkdir -p ~/mongodatadocker run -d --rm -p 27017:27017 -v ~/mongodata:/data/db --name mongodb mongo
```### 2 - Install this library
```bash
pip install Flask-RestGlue
```### 3 - Pull the code
```bash
curl -s -O -L https://github.com/abassel/Flask-RestGlue/blob/master/example/tut01_hello_world.py
curl -s -O -L https://github.com/abassel/Flask-RestGlue/blob/master/example/tut01_hello_world.sh
```### 4 - Run the code
```bash
python tut01_hello_world.py
```
In another terminal window```bash
bash tut01_hello_world.sh
```## Road Map :car:
- Evaluate https://testdriven.io/blog/fastapi-mongo/
- Evaluate https://github.com/David-Lor/FastAPI-Pydantic-Mongo_Sample_CRUD_API
- Evaluate https://art049.github.io/odmantic/
- Example user model that stores password as hash - https://aaronluna.dev/series/flask-api-tutorial/part-2/
- ORM examples for FastAPI - https://github.com/mjhea0/awesome-fastapi#orms
- ODM examples for FastAPI - https://github.com/mjhea0/awesome-fastapi#odms
- Authorization at row level - https://github.com/mjhea0/awesome-fastapi?tab=readme-ov-file#auth
- Develop pagination
- Develop Pydantic
- Google search about flask api with sqlalchemy https://www.google.com/search?q=flask+api+sqlalchemy+tutorial
- Evaluate flask api with migration https://aaronluna.dev/series/flask-api-tutorial/part-2/
- Develop tools to generate typescript or svelte stubs
- Create a template like https://github.com/tiangolo/full-stack-fastapi-template## References :notebook:
- [Flask_RestGlue_Svelte_Docker boilerplate](https://github.com/abassel/Flask_RestGlue_Svelte_Docker)
- [OpenAPI 3.0.3](https://github.com/OAI/OpenAPI-Specification/blob/master/versions/3.0.3.md)
- [Flask](http://flask.pocoo.org)
- [Mongoengine](https://github.com/MongoEngine/mongoengine)