Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/alanshaw/embed-video
🎥 Get embed HTML code for youtube/vimeo/whatever from URL or ID
https://github.com/alanshaw/embed-video
embed html iframe screenshot share thumbnails video vimeo youtube
Last synced: 6 days ago
JSON representation
🎥 Get embed HTML code for youtube/vimeo/whatever from URL or ID
- Host: GitHub
- URL: https://github.com/alanshaw/embed-video
- Owner: alanshaw
- License: mit
- Created: 2014-05-01T10:33:12.000Z (almost 11 years ago)
- Default Branch: master
- Last Pushed: 2023-05-08T03:55:01.000Z (almost 2 years ago)
- Last Synced: 2025-01-29T15:09:01.958Z (13 days ago)
- Topics: embed, html, iframe, screenshot, share, thumbnails, video, vimeo, youtube
- Language: JavaScript
- Homepage: http://npm.im/embed-video
- Size: 127 KB
- Stars: 72
- Watchers: 9
- Forks: 27
- Open Issues: 7
-
Metadata Files:
- Readme: README.md
- License: LICENSE.md
Awesome Lists containing this project
README
# embed-video
[](https://travis-ci.org/alanshaw/embed-video) [](https://david-dm.org/alanshaw/embed-video#info=devDependencies) [](https://standardjs.com)
> Get embed code for embedding youtube/vimeo/dailymotion/whatever video in websites from URL or ID.
Currently supports YouTube, Vimeo and DailyMotion. Please pull request to add others!
## Example
```js
var embed = require("embed-video")var vimeoUrl = "http://vimeo.com/19339941"
var youtubeUrl = "https://www.youtube.com/watch?v=twE64AuqE9A"
var dailymotionUrl = "https://www.dailymotion.com/video/x20qnej_red-bull-presents-wild-ride-bmx-mtb-dirt_sport"console.log(embed(vimeoUrl))
console.log(embed(youtubeUrl))
console.log(embed(dailymotionUrl))var vimeoId = "6964150"
var youtubeId = "9XeNNqeHVDw"
var dailymotionId = "x20qnej"console.log(embed.vimeo(vimeoId))
console.log(embed.youtube(youtubeId))
console.log(embed.dailymotion(dailymotionId))
```Output:
```html
```
## Usage
```js
var embed = require("embed-video")
```### embed(url, [options])
Return an HTML fragment embed code (string) for the given video URL. Returns `undefined` if unrecognised.
### embed.image(url, [options], callback)
Returns an HTML `
` tag (string) for the given url and the `src` in a callback. Works for **youtube**, **vimeo** and **dailymotion**. Returns `undefined` if unrecognised.
```js
{
src: http://img.youtube.com/vi/eob7V_WtAVg/default.jpg,
html:![]()
}
```### embed.info(url)
Returns an `object` containing the video ID, video source (`"youtube"`, `"vimeo"`, `"dailymotion"`), and the original url. Works for **youtube**, **vimeo** and **dailymotion**. Returns `undefined` if unrecognised.
```js
{
id: String,
url: String,
source: Enum "youtube", "vimeo", "dailymotion"
}
```## Options
### `query`
Object to be serialized as a querystring and appended to the embedded content url.
#### Example
```js
console.log(embed.vimeo("19339941", {query: {portrait: 0, color: '333'}}))
```Output:
```html
```
### `attr`Object to add additional attributes (any) to the iframe
#### Example
```js
console.log(embed('https://youtu.be/jglUWD3KMh4', {query: {portrait: 0, color: '333'}, attr:{width:400, height:200}}))
```Output:
```html```
### `image`
#### Youtube Image options
|option|image|
|:------|:-----:|
|default|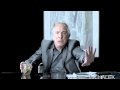|
|mqdefault|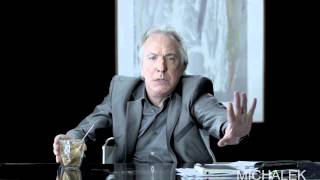|
|hqdefault|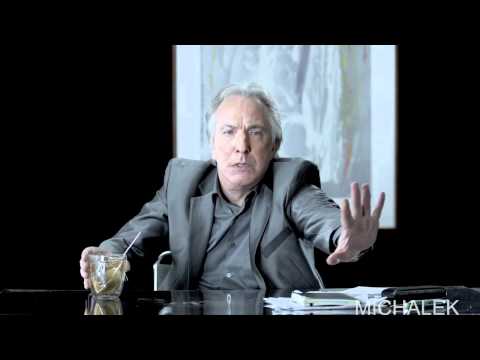|
|sddefault|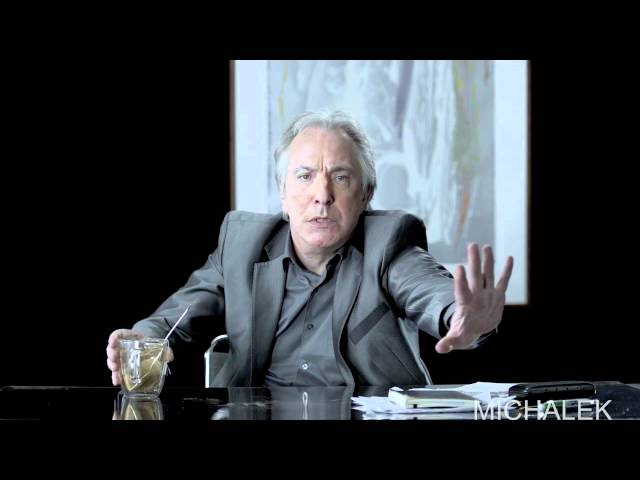|
|maxresdefault|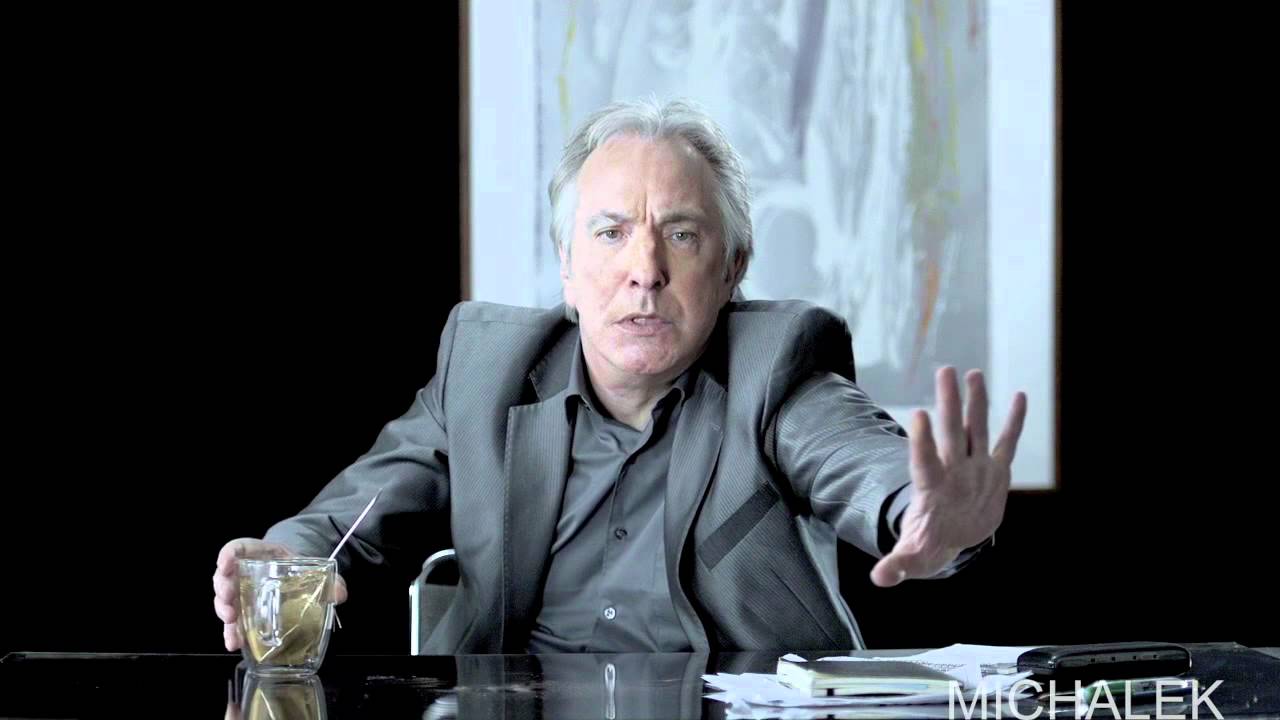|```js
embedVideo.image('https://www.youtube.com/watch?v=ekETjYMo6QE', {image: 'mqdefault'}, function (err, thumbnail) {
if (err) throw err
console.log(thumbnail.src)
// https://img.youtube.com/vi/ekETjYMo6QE/mqdefault.jpg
console.log(thumbnail.html)
//![]()
})
```#### Vimeo Image options
|option|image|
|:---|:---|
|thumbnail_small|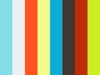|
|thumbnail_medium|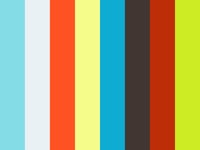|
|thumbnail_large|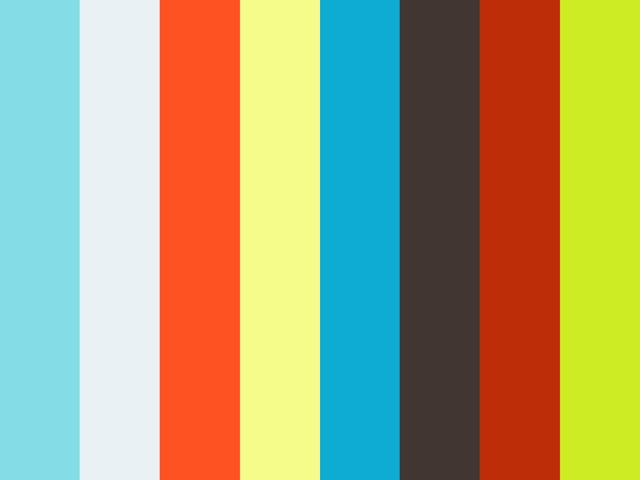|```js
embedVideo.image('https://vimeo.com/19339941', {image: 'thumbnail_medium'}, function (err, thumbnail) {
if (err) throw err
console.log(thumbnail.src)
// http://i.vimeocdn.com/video/122513613_200x150.jpg
console.log(thumbnail.html)
//![]()
})
```#### DailyMotion Image options
|option|image|
|:---|:---|
|thumbnail_60_url||
|thumbnail_120_url||
|thumbnail_180_url||
|thumbnail_240_url||
|thumbnail_360_url||
|thumbnail_480_url||
|thumbnail_720_url||
|thumbnail_1080_url||```js
embedVideo.image('https://www.dailymotion.com/video/x20qnej_red-bull-presents-wild-ride-bmx-mtb-dirt_sport', {image: 'thumbnail_720_url'}, function (err, thumbnail) {
if (err) throw err
console.log(thumbnail.src)
// http://s1.dmcdn.net/IgPVQ/x720-d_h.jpg
console.log(thumbnail.html)
//![]()
})
```## Contribute
Feel free to dive in! [Open an issue](https://github.com/alanshaw/embed-video/issues/new) or submit PRs.
## License
[MIT](LICENSE) © Alan Shaw