Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/amazingandyyy/use-form-react
⚡ React form hook made blazing fast and easy.
https://github.com/amazingandyyy/use-form-react
form form-hook hook react react-hook
Last synced: 2 months ago
JSON representation
⚡ React form hook made blazing fast and easy.
- Host: GitHub
- URL: https://github.com/amazingandyyy/use-form-react
- Owner: amazingandyyy
- License: mit
- Created: 2019-04-30T06:13:08.000Z (over 5 years ago)
- Default Branch: main
- Last Pushed: 2022-04-19T14:42:51.000Z (over 2 years ago)
- Last Synced: 2024-10-29T20:08:17.689Z (3 months ago)
- Topics: form, form-hook, hook, react, react-hook
- Language: JavaScript
- Homepage: https://www.npmjs.com/package/use-form-react
- Size: 24.4 KB
- Stars: 34
- Watchers: 2
- Forks: 4
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- License: license.md
Awesome Lists containing this project
- awesome-blazingly-fast - use-form-react - ⚡ React form hook made blazing fast and easy. (JavaScript)
README
⚡ useForm
Form hook made blazing fast and easy.> The most unopinionated form hook.
## Installation
```shell
npm i --save use-form-react
# or
yarn add use-form-react
```## Have a good to use form in 10 seconds
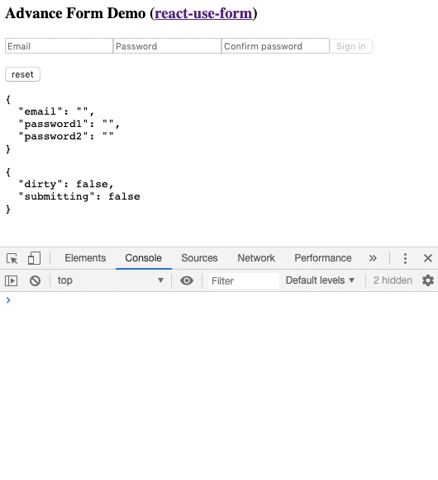
## Usage
### Basic Usage
check [basic example](https://github.com/amazingandyyy/use-form-react/blob/master/examples/basic/index.js)
```jsx
import useForm from 'use-form-react'const Form = () => {
const { onSubmit, onChange, inputs } = useForm('sampleForm', {
initialValues: { 'name': '' },
callback: (inputs) => console.log(inputs)
}
)
return (
Hello {inputs.name}
Sign in
);
}
```### Advance Usage
check [advance example](https://github.com/amazingandyyy/use-form-react/blob/master/examples/advance/index.js)
```jsx
import React, { useEffect } from 'react';
import useForm from 'use-form-react'const SignUp = () => {
const options = {
initialValues: {
email: '',
password1: '',
password2: ''
},
validation: {
email: (inputs) => {
const emailRegex = /^(([^<>()\[\]\\.,;:\s@"]+(\.[^<>()\[\]\\.,;:\s@"]+)*)|(".+"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/
if (!inputs.email || inputs.email === '') {
return 'email is required';
}if (!emailRegex.test(inputs.email.toLowerCase())) {
return 'invalid email';
}
},
password1: (inputs) => {
const passwordRegex = /((?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*[\W]).{6,20})/if (!inputs.password1 || inputs.password1 === '') {
return 'password is required';
}if (!passwordRegex.test(inputs.password1)) {
return 'password is too simple';
}
},
password2: (inputs) => {
if (!inputs.password2 || inputs.password2 === '') {
return 'password is required';
}if (inputs.password1 !== inputs.password2) {
return 'password are differents';
}
}
},
callback: () => console.log('works!', inputs)
}const { onSubmit, onChange, inputs, dirty, submitting, reset, errors, valid } = useForm('AdvanceForm', options)
return (
{errors.email}
{errors.password1}
{errors.password2}
Sign up
);
}
```## To Do
- [ ] better test case
- [ ] debounce the error
- [x] built-in validation - done by [#3](https://github.com/amazingandyyy/use-form-react/pull/3)
- [x] support ts - done by [#4](https://github.com/amazingandyyy/use-form-react/pull/4)
- [ ] ts docs## License
MIT