Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/andy-aa/mydb
The lightweight ORM for MySql and MariaDB
https://github.com/andy-aa/mydb
crud mariadb mysql orm pagination php query-builder
Last synced: about 2 months ago
JSON representation
The lightweight ORM for MySql and MariaDB
- Host: GitHub
- URL: https://github.com/andy-aa/mydb
- Owner: andy-aa
- License: mit
- Created: 2019-09-13T18:52:04.000Z (over 5 years ago)
- Default Branch: master
- Last Pushed: 2020-08-07T21:56:02.000Z (over 4 years ago)
- Last Synced: 2024-06-08T11:48:42.857Z (7 months ago)
- Topics: crud, mariadb, mysql, orm, pagination, php, query-builder
- Language: PHP
- Homepage: https://packagist.org/packages/texlab/mydb
- Size: 183 KB
- Stars: 1
- Watchers: 1
- Forks: 7
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
[](https://github.com/andy-aa/mydb)
[](https://travis-ci.com/andy-aa/mydb)
[](https://opensource.org/licenses/MIT)
[](https://php.net/)
[](https://packagist.org/packages/texlab/mydb)
[](https://phpstan.org/)
[](https://psalm.dev/)
[](https://coveralls.io/github/andy-aa/mydb?branch=master)# MyDB
- [Install](#install-via-composer)
- [Class diagram](#class-diagram)
- [Database for examples](#database-for-examples)
- [Usage example](#usage-example)
- [CRUD](#crud)
- [Adding data](#adding-data)
- [Reading data](#reading-data)
- [Updating data](#updating-data)
- [Data deletion](#data-deletion)
- [Query builder](#query-builder)
- [Error handling](#error-handling)
- [Pagination](#pagination)## Install via composer
Command line
```
composer require texlab/mydb
```
Example **composer.json** file
```
{
"require": {
"texlab/mydb": "^0.0.5"
}
}
```## Class diagram
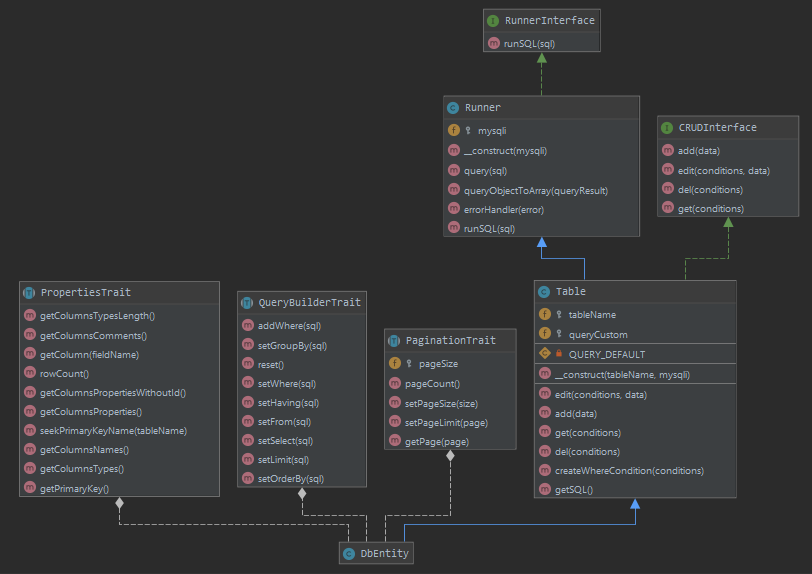
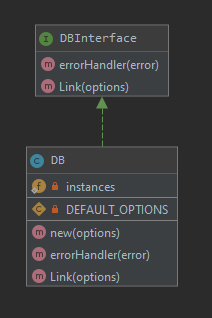## Database for examples
```sql
CREATE DATABASE IF NOT EXISTS `mydb`;USE `mydb`;
CREATE TABLE IF NOT EXISTS `table1` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(50) NOT NULL,
`description` varchar(200) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
```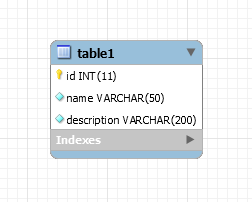
## Usage example
```php
'localhost',
'username' => 'root',
'password' => '',
'dbname' => 'mydb'
]);$table1 = new DbEntity('table1', $link);
echo json_encode($table1->get());
```## CRUD
### Adding data:
```php
$table1->add([
'name' => 'Peter',
'description' => 'Director'
]);
```### Reading data:
```php
$table1->get();
```
or a row with the given id```php
$table1->get(['id' => 3]);
```### Updating data:
```php
$table1->edit(['id' => 2], [
'name' => 'Alex',
'description' => 'Manager'
]);
```### Data deletion:
```php
$table1->del(['id' => 1]);
```
## Custom queries```php
echo json_encode($table1->runSQL("SELECT * FROM table1"));
```
## Query builder```php
echo json_encode(
$table1
->reset()
->setSelect('id, name')
->setWhere("name like 'A%'")
->get()
);
``````php
$table1
->reset()
->setSelect('name, description')
->setWhere("description = 'Manager'")
->setOrderBy('name');echo json_encode(
$table1->get()
);$table1->setSelect('*');
echo json_encode(
$table1->get()
);
```
## Error handling```php
'localhost',
'username' => 'root',
'password' => 'root',
'dbname' => 'test_db'
]
)
);$runner->setErrorHandler(
function ($mysqli, $sql) {
//put your error handling code here
print_r([$mysqli->error, $mysqli->errno, $sql]);
}
);$runner->runSQL("SELECT * FROM unknown_table");
```
Result:
```
Array
(
[0] => Table 'test_db.unknown_table' doesn't exist
[1] => 1146
[2] => SELECT * FROM unknown_table
)
```
## Pagination```php
echo $table1
->setPageSize(2)
->pageCount();
``````php
echo json_encode($table1->setPageSize(2)->getPage(1));
```