Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/andyfleming/interval-promise
setInterval with setTimeout semantics for promises and async/await
https://github.com/andyfleming/interval-promise
Last synced: 4 months ago
JSON representation
setInterval with setTimeout semantics for promises and async/await
- Host: GitHub
- URL: https://github.com/andyfleming/interval-promise
- Owner: andyfleming
- License: mit
- Created: 2017-11-23T08:51:04.000Z (about 7 years ago)
- Default Branch: master
- Last Pushed: 2023-01-04T21:35:56.000Z (about 2 years ago)
- Last Synced: 2024-08-11T11:05:46.311Z (6 months ago)
- Language: JavaScript
- Size: 354 KB
- Stars: 106
- Watchers: 5
- Forks: 15
- Open Issues: 25
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# Interval Promise
[](https://npmjs.org/package/interval-promise)
[](https://travis-ci.org/andyfleming/interval-promise)
[](https://coveralls.io/github/andyfleming/interval-promise?branch=master)## Overview
This library provides a simple mechanism for running a promise with a given amount of time between executions.
#### Standard Javascript » setInterval()
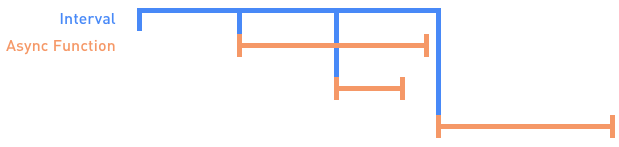
#### interval-promise » interval()

## Installation
```bash
npm install interval-promise
```## Usage
**Simple example using async-await**
```javascript
const interval = require('interval-promise')// Run a function 10 times with 1 second between each iteration
interval(async () => {
await someOtherPromiseReturningFunction()
await another()
}, 1000, {iterations: 10})
```## API
```javascript
interval(func, intervalLength, options = {}) // returns Promise
```### Arguments
Argument
Attritubes
Description
func
function
Required
Function to execute for each interval. MUST return a promise.
Two arguments are passed to this function.
-
iterationNumber
number — The iteration number (starting at 1) -
stop
function — used to "stop" (skipping all remaining iterations)
intervalLength
number | function
Required
Length in ms to wait between iterations. Should be (or return) a non-negative integer.
If a function is used, one parameter
iterationNumber
(starting at 1) is passed.
options
object
Optional settings (detailed below).
options.iterations
number
Default:
Infinity
The number of times to execute the function. Must be Infinity or an integer greater than 0.
options.stopOnError
boolean
Default:
true
If true, no subsequent calls will be made. The promise returned by interval() will be rejected and pass through the error thrown.
## Project Values
* **Approachability** — Basic usage should be concise and readable.
* **Debuggability** — Error feedback should be helpful and error handling options should be flexible.
* **Stability** — Functionality should be well-tested and reliable.
## Acknowledgements
This library was inspired by [reissue](https://github.com/DonutEspresso/reissue).
## FAQ
### How can I stop the interval from _outside_ the interval function?
There isn't currently direct feature to stop the iterations externally. You can, however, achieve this by checking a variable in the parent scope (of where the function is defined). Check out the code below.
```js
const interval = require('interval-promise')
let stoppedExternally = false
const stopExternally = () => { stoppedExternally = true }
interval(async (iteration, stop) => {
if (stoppedExternally) {
stop()
}
// ... normal functionality ...
}, 1000)
// Some other work...
someOtherWork().then(() => {
// Now that our "other work" is done, we can stop our interval above with:
stopExternally()
})
```