https://github.com/atulmy/gql-query-builder
🔧 Simple GraphQL Query Builder
https://github.com/atulmy/gql-query-builder
generator graphql nodejs query-builder query-generator
Last synced: 3 months ago
JSON representation
🔧 Simple GraphQL Query Builder
- Host: GitHub
- URL: https://github.com/atulmy/gql-query-builder
- Owner: atulmy
- License: mit
- Created: 2018-09-30T08:44:32.000Z (almost 7 years ago)
- Default Branch: master
- Last Pushed: 2023-12-18T15:32:47.000Z (over 1 year ago)
- Last Synced: 2025-04-01T05:33:56.380Z (3 months ago)
- Topics: generator, graphql, nodejs, query-builder, query-generator
- Language: TypeScript
- Homepage: https://npmjs.com/package/gql-query-builder
- Size: 725 KB
- Stars: 398
- Watchers: 4
- Forks: 47
- Open Issues: 25
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# GraphQL Query Builder
A simple helper function to generate GraphQL queries using plain JavaScript Objects (JSON).
## Install
`npm install gql-query-builder --save` or `yarn add gql-query-builder`
## Usage
```typescript
import * as gql from 'gql-query-builder'const query = gql.query(options: object)
const mutation = gql.mutation(options: object)
const subscription = gql.subscription(options: object)
```You can also import `query` or `mutation` or `subscription` individually:
```typescript
import { query, mutation, subscription } from 'gql-query-builder'query(options: object)
mutation(options: object)
subscription(options: object)
```### Options
`options` is `{ operation, fields, variables }` or an array of `options`
Name
Description
Type
Required
Example
operation
Name of operation to be executed on server
String | Object
Yes
getThoughts, createThought
{ name: 'getUser', alias: 'getAdminUser' }
fields
Selection of fields
Array
No
['id', 'name', 'thought']
['id', 'name', 'thought', { user: ['id', 'email'] }]
variables
Variables sent to the operation
Object
No
{ key: value } eg:{ id: 1 }
{ key: { value: value, required: true, type: GQL type, list: true, name: argument name } eg:
{
email: { value: "[email protected]", required: true },
password: { value: "123456", required: true },
secondaryEmails: { value: [], required: false, type: 'String', list: true, name: secondaryEmail }
}
### Adapter
An optional second argument `adapter` is a typescript/javascript class that implements `src/adapters/IQueryAdapter` or `src/adapters/IMutationAdapter`.
If adapter is undefined then `src/adapters/DefaultQueryAdapter` or `src/adapters/DefaultMutationAdapter` is used.
```
import * as gql from 'gql-query-builder'const query = gql.query(options: object, adapter?: MyCustomQueryAdapter,config?: object)
const mutation = gql.mutation(options: object, adapter?: MyCustomQueryAdapter)
const subscription = gql.subscription(options: object, adapter?: MyCustomSubscriptionAdapter)
```### Config
Name
Description
Type
Required
Example
operationName
Name of operation to be sent to the server
String
No
getThoughts, createThought
## Examples
1. Query
2. Query (with variables)
3. Query (with nested fields selection)
4. Query (with required variables)
5. Query (with custom argument name)
6. Query (with operation name)
7. Query (with empty fields)
8. Query (with alias)
9. Query (with adapter defined)
10. Mutation
11. Mutation (with required variables)
12. Mutation (with custom types)
13. Mutation (with adapter defined)
14. Mutation (with operation name)
15. Subscription
16. Subscription (with adapter defined)
17. Example with Axios#### Query:
```javascript
import * as gql from 'gql-query-builder'const query = gql.query({
operation: 'thoughts',
fields: ['id', 'name', 'thought']
})console.log(query)
// Output
query {
thoughts {
id,
name,
thought
}
}
```[↑ all examples](#examples)
#### Query (with variables):
```javascript
import * as gql from 'gql-query-builder'const query = gql.query({
operation: 'thought',
variables: { id: 1 },
fields: ['id', 'name', 'thought']
})console.log(query)
// Output
query ($id: Int) {
thought (id: $id) {
id, name, thought
}
}// Variables
{ "id": 1 }
```[↑ all examples](#examples)
#### Query (with nested fields selection):
```javascript
import * as gql from 'gql-query-builder'const query = gql({
operation: 'orders',
fields: [
'id',
'amount',
{
user: [
'id',
'name',
'email',
{
address: [
'city',
'country'
]
}
]
}
]
})console.log(query)
// Output
query {
orders {
id,
amount,
user {
id,
name,
email,
address {
city,
country
}
}
}
}
```[↑ all examples](#examples)
#### Query (with required variables):
```javascript
import * as gql from 'gql-query-builder'const query = gql.query({
operation: 'userLogin',
variables: {
email: { value: '[email protected]', required: true },
password: { value: '123456', required: true }
},
fields: ['userId', 'token']
})console.log(query)
// Output
query ($email: String!, $password: String!) {
userLogin (email: $email, password: $password) {
userId, token
}
}// Variables
{
email: "[email protected]",
password: "123456"
}
```[↑ all examples](#examples)
#### Query (with custom argument name):
```javascript
import * as gql from 'gql-query-builder'const query = gql.query([{
operation: "someoperation",
fields: [{
operation: "nestedoperation",
fields: ["field1"],
variables: {
id2: {
name: "id",
type: "ID",
value: 123,
},
},
}, ],
variables: {
id1: {
name: "id",
type: "ID",
value: 456,
},
},
}, ]);console.log(query)
// Output
query($id2: ID, $id1: ID) {
someoperation(id: $id1) {
nestedoperation(id: $id2) {
field1
}
}
}// Variables
{
"id1": 1,
"id2": 1
}
```[↑ all examples](#examples)
#### Query (with operation name):
```javascript
import * as gql from 'gql-query-builder'const query = gql.query({
operation: 'userLogin',
fields: ['userId', 'token']
}, null, {
operationName: 'someoperation'
})console.log(query)
// Output
query someoperation {
userLogin {
userId
token
}
}
```[↑ all examples](#examples)
#### Query (with empty fields):
```javascript
import * as gql from 'gql-query-builder'const query = gql.query([{
operation: "getFilteredUsersCount",
},
{
operation: "getAllUsersCount",
fields: []
},
operation: "getFilteredUsers",
fields: [{
count: [],
}, ],
]);console.log(query)
// Output
query {
getFilteredUsersCount
getAllUsersCount
getFilteredUsers {
count
}
}
```[↑ all examples](#examples)
#### Query (with alias):
```javascript
import * as gql from 'gql-query-builder'const query = gql.query({
operation: {
name: 'thoughts',
alias: 'myThoughts',
},
fields: ['id', 'name', 'thought']
})console.log(query)
// Output
query {
myThoughts: thoughts {
id,
name,
thought
}
}
```[↑ all examples](#examples)
#### Query (with inline fragment):
```javascript
import * as gql from 'gql-query-builder'const query = gql.query({
operation: "thought",
fields: [
"id",
"name",
"thought",
{
operation: "FragmentType",
fields: ["emotion"],
fragment: true,
},
],
});console.log(query)
// Output
query {
thought {
id,
name,
thought,
... on FragmentType {
emotion
}
}
}
```[↑ all examples](#examples)
#### Query (with adapter defined):
For example, to inject `SomethingIDidInMyAdapter` in the `operationWrapperTemplate` method.
```javascript
import * as gql from 'gql-query-builder'
import MyQueryAdapter from 'where/adapters/live/MyQueryAdapter'const query = gql.query({
operation: 'thoughts',
fields: ['id', 'name', 'thought']
}, MyQueryAdapter)console.log(query)
// Output
query SomethingIDidInMyAdapter {
thoughts {
id,
name,
thought
}
}
```Take a peek at [DefaultQueryAdapter](src/adapters/DefaultQueryAdapter.ts) to get an understanding of how to make a new adapter.
[↑ all examples](#examples)
#### Mutation:
```javascript
import * as gql from 'gql-query-builder'const query = gql.mutation({
operation: 'thoughtCreate',
variables: {
name: 'Tyrion Lannister',
thought: 'I drink and I know things.'
},
fields: ['id']
})console.log(query)
// Output
mutation ($name: String, $thought: String) {
thoughtCreate (name: $name, thought: $thought) {
id
}
}// Variables
{
"name": "Tyrion Lannister",
"thought": "I drink and I know things."
}
```[↑ all examples](#examples)
#### Mutation (with required variables):
```javascript
import * as gql from 'gql-query-builder'const query = gql.mutation({
operation: 'userSignup',
variables: {
name: { value: 'Jon Doe' },
email: { value: '[email protected]', required: true },
password: { value: '123456', required: true }
},
fields: ['userId']
})console.log(query)
// Output
mutation ($name: String, $email: String!, $password: String!) {
userSignup (name: $name, email: $email, password: $password) {
userId
}
}// Variables
{
name: "Jon Doe",
email: "[email protected]",
password: "123456"
}
```[↑ all examples](#examples)
#### Mutation (with custom types):
```javascript
import * as gql from 'gql-query-builder'const query = gql.mutation({
operation: "userPhoneNumber",
variables: {
phone: {
value: { prefix: "+91", number: "9876543210" },
type: "PhoneNumber",
required: true
}
},
fields: ["id"]
})console.log(query)
// Output
mutation ($phone: PhoneNumber!) {
userPhoneNumber (phone: $phone) {
id
}
}// Variables
{
phone: {
prefix: "+91", number: "9876543210"
}
}
```[↑ all examples](#examples)
#### Mutation (with adapter defined):
For example, to inject `SomethingIDidInMyAdapter` in the `operationWrapperTemplate` method.
```javascript
import * as gql from 'gql-query-builder'
import MyMutationAdapter from 'where/adapters/live/MyQueryAdapter'const query = gql.mutation({
operation: 'thoughts',
fields: ['id', 'name', 'thought']
}, MyMutationAdapter)console.log(query)
// Output
mutation SomethingIDidInMyAdapter {
thoughts {
id,
name,
thought
}
}
```[↑ all examples](#examples)
Take a peek at [DefaultMutationAdapter](src/adapters/DefaultMutationAdapter.ts) to get an understanding of how to make a new adapter.
#### Mutation (with operation name):
```javascript
import * as gql from 'gql-query-builder'const query = gql.mutation({
operation: 'thoughts',
fields: ['id', 'name', 'thought']
}, undefined, {
operationName: 'someoperation'
})console.log(query)
// Output
mutation someoperation {
thoughts {
id
name
thought
}
}
```[↑ all examples](#examples)
#### Subscription:
```javascript
import axios from "axios";
import { subscription } from "gql-query-builder";async function saveThought() {
try {
const response = await axios.post(
"http://api.example.com/graphql",
subscription({
operation: "thoughtCreate",
variables: {
name: "Tyrion Lannister",
thought: "I drink and I know things.",
},
fields: ["id"],
})
);console.log(response);
} catch (error) {
console.log(error);
}
}
```[↑ all examples](#examples)
#### Subscription (with adapter defined):
For example, to inject `SomethingIDidInMyAdapter` in the `operationWrapperTemplate` method.
```javascript
import * as gql from 'gql-query-builder'
import MySubscriptionAdapter from 'where/adapters/live/MyQueryAdapter'const query = gql.subscription({
operation: 'thoughts',
fields: ['id', 'name', 'thought']
}, MySubscriptionAdapter)console.log(query)
// Output
subscription SomethingIDidInMyAdapter {
thoughts {
id,
name,
thought
}
}
```Take a peek at [DefaultSubscriptionAdapter](src/adapters/DefaultSubscriptionAdapter.ts) to get an understanding of how to make a new adapter.
[↑ all examples](#examples)
#### Example with [Axios](https://github.com/axios/axios)
**Query:**
```javascript
import axios from "axios";
import { query } from "gql-query-builder";async function getThoughts() {
try {
const response = await axios.post(
"http://api.example.com/graphql",
query({
operation: "thoughts",
fields: ["id", "name", "thought"],
})
);console.log(response);
} catch (error) {
console.log(error);
}
}
```[↑ all examples](#examples)
**Mutation:**
```javascript
import axios from "axios";
import { mutation } from "gql-query-builder";async function saveThought() {
try {
const response = await axios.post(
"http://api.example.com/graphql",
mutation({
operation: "thoughtCreate",
variables: {
name: "Tyrion Lannister",
thought: "I drink and I know things.",
},
fields: ["id"],
})
);console.log(response);
} catch (error) {
console.log(error);
}
}
```[↑ all examples](#examples)
# Showcase
Following projects are using [gql-query-builder](https://github.com/atulmy/gql-query-builder)
- Crate - Get monthly subscription of trendy clothes and accessories - [GitHub](https://github.com/atulmy/crate)
- Fullstack GraphQL Application - [GitHub](https://github.com/atulmy/fullstack-graphql)
- Would really appreciate if you add your project to this list by sending a PR## Author
- Atul Yadav - [GitHub](https://github.com/atulmy) · [Twitter](https://twitter.com/atulmy)
## Contributors
**If you are interested in actively maintaining / enhancing this project, get in touch.**
- Juho Vepsäläinen - [GitHub](https://github.com/bebraw) · [Twitter](https://twitter.com/bebraw)
- Daniel Hreben - [GitHub](https://github.com/DanielHreben) · [Twitter](https://twitter.com/DanielHreben)
- Todd Baur - [GitHub](https://github.com/toadkicker) · [Twitter](https://twitter.com/toadkicker)
- Alireza Hariri - [GitHub](https://github.com/ARHariri)
- Cédric - [GitHub](https://github.com/cbonaudo)
- Clayton Collie - [GitHub](https://github.com/ccollie)
- Devon Reid - [GitHub](https://github.com/Devorein)
- Harry Brundage - [GitHub](https://github.com/airhorns) · [Twitter](https://twitter.com/harrybrundage)
- Clément Berard - [GitHub](https://github.com/clement-berard) · [Twitter](https://twitter.com/clementberard)
- Lee Rose - [GitHub](https://github.com/leeroyrose)
- Christian Westgaard - [GitHub](https://github.com/ComLock)
- [YOUR NAME HERE] - Feel free to contribute to the codebase by resolving any open issues, refactoring, adding new features, writing test cases or any other way to make the project better and helpful to the community. Feel free to fork and send pull requests.## Donate
If you liked this project, you can donate to support it ❤️
[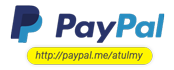](http://paypal.me/atulmy)
## License
Copyright (c) 2018 Atul Yadav
The MIT License ()