https://github.com/bbmokhtari/django-translations
Django model translation for perfectionists with deadlines.
https://github.com/bbmokhtari/django-translations
django internationalization localization model model-translations translation translations
Last synced: about 2 months ago
JSON representation
Django model translation for perfectionists with deadlines.
- Host: GitHub
- URL: https://github.com/bbmokhtari/django-translations
- Owner: bbmokhtari
- License: bsd-3-clause
- Created: 2018-07-03T09:27:51.000Z (about 7 years ago)
- Default Branch: master
- Last Pushed: 2025-04-19T15:41:44.000Z (3 months ago)
- Last Synced: 2025-05-09T12:51:25.303Z (about 2 months ago)
- Topics: django, internationalization, localization, model, model-translations, translation, translations
- Language: Python
- Homepage: http://bbmokhtari.github.io/django-translations
- Size: 2.51 MB
- Stars: 144
- Watchers: 9
- Forks: 23
- Open Issues: 4
-
Metadata Files:
- Readme: .github/README.md
- Contributing: .github/CONTRIBUTING.md
- License: LICENSE
- Code of conduct: .github/CODE_OF_CONDUCT.md
Awesome Lists containing this project
README
# Django Translations
[](https://pypi.org/project/django-translations/)
[](https://pypi.org/project/django-translations/)Django model translation for perfectionists with deadlines.
## Goal
There are two types of content, each of which has its own challenges for translation:
- Static content: This is the content which is defined in the code.
_e.g. "Please enter a valid email address."_Django already provides a
[solution](https://docs.djangoproject.com/en/2.2/topics/i18n/translation/)
for translating static content.- Dynamic content: This is the content which is stored in the database.
_(We can't know it beforehand!)_Django Translations provides a solution
for translating dynamic content.## Compatibility
Currently, this project is incompatible with PostgreSQL.
## Requirements
- Python (\>=3.7, \<4)
- Django (\>=2.2, \<6)## Installation
1. Install Django Translations using pip:
```bash
$ pip install django-translations
```2. Add `translations` to the `INSTALLED_APPS` in the settings of your
project:```python
INSTALLED_APPS += [
'translations',
]
```3. Run `migrate`:
```bash
$ python manage.py migrate
```4. Configure Django internationalization and localization settings:
```python
USE_I18N = True # use internationalization
USE_L10N = True # use localizationMIDDLEWARE += [ # locale middleware
'django.middleware.locale.LocaleMiddleware',
]LANGUAGE_CODE = 'en-us' # default (fallback) language
LANGUAGES = ( # supported languages
('en', 'English'),
('en-gb', 'English (Great Britain)'),
('de', 'German'),
('tr', 'Turkish'),
)
```Please note that these settings are for Django itself.
## Basic Usage
### Model
Inherit `Translatable` in any model you want translated:
```python
from translations.models import Translatableclass Continent(Translatable):
code = models.Charfield(...)
name = models.Charfield(...)
denonym = models.Charfield(...)class TranslatableMeta:
fields = ['name', 'denonym']
```No migrations needed afterwards.
### Admin
Use the admin extensions:
```python
from translations.admin import TranslatableAdmin, TranslationInlineclass ContinentAdmin(TranslatableAdmin):
inlines = [TranslationInline,]
```This provides specialized translation inlines for the model.
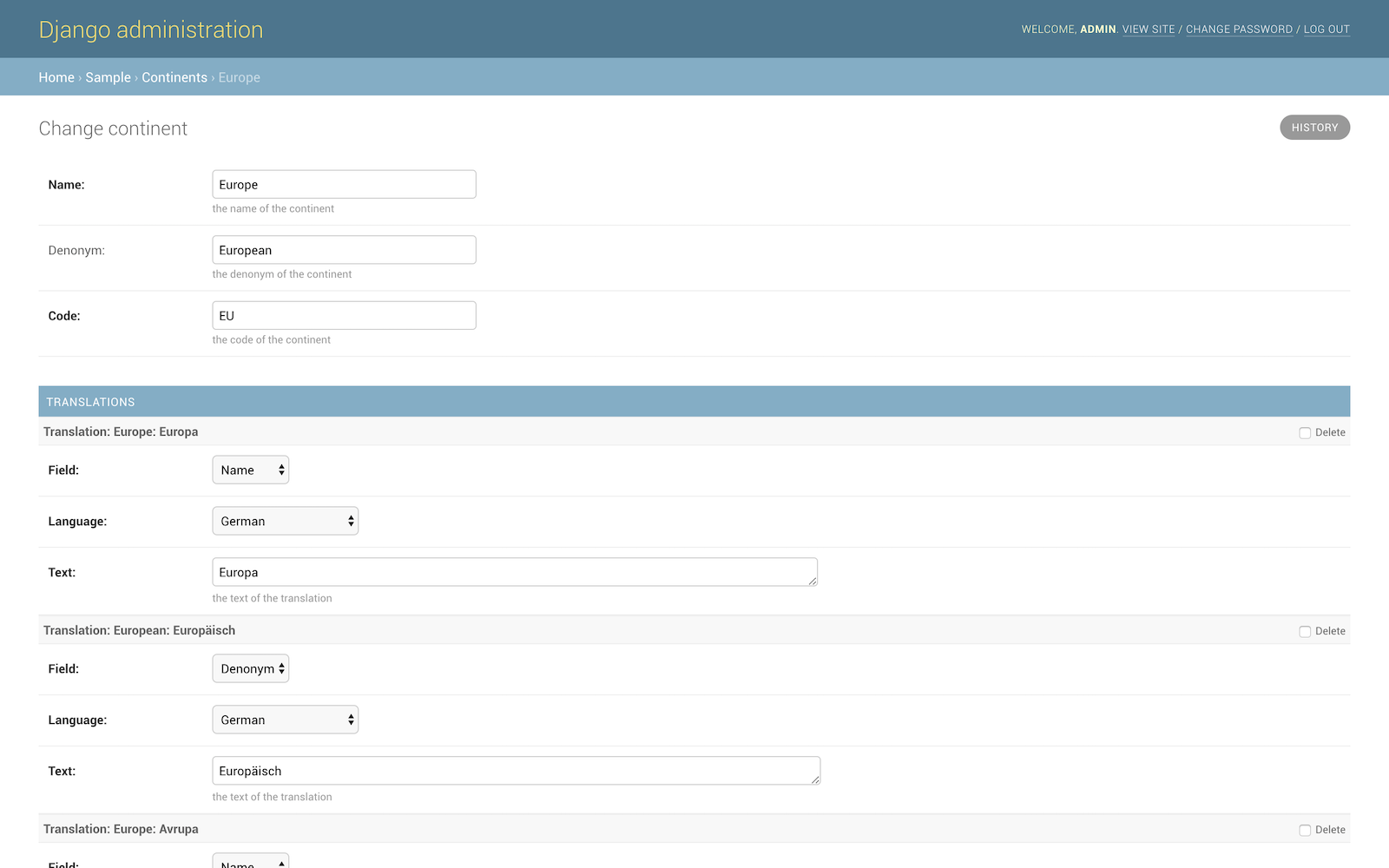
## QuerySet
Use the queryset extensions:
```python
>>> from sample.models import Continent
>>> continents = Continent.objects.all(
... ).distinct( # familiar distinct
... ).probe(['en', 'de'] # probe (filter, exclude, etc.) in English and German
... ).filter( # familiar filtering
... countries__cities__name__startswith='Köln'
... ).translate('de' # translate the results in German
... ).translate_related( # translate these relations as well
... 'countries', 'countries__cities',
... )
>>> print(continents)
,
]>
>>> print(continents[0].countries.all())
,
]>
>>> print(continents[0].countries.all()[0].cities.all())
,
]>
```This provides a powerful yet familiar interface to work with the querysets.
## Context
Use the translation context:
```python
>>> from translations.context import Context
>>> from sample.models import Continent
>>> continents = Continent.objects.all()
>>> relations = ('countries', 'countries__cities',)
>>> with Context(continents, *relations) as context:
... context.read('de') # read the translations onto the context
... print(':') # use the objects like before
... print(continents)
... print(continents[0].countries.all())
... print(continents[0].countries.all()[0].cities.all())
...
... continents[0].countries.all()[0].name = 'Change the name'
... context.update('de') # update the translations from the context
...
... context.delete('de') # delete the translations of the context
...
... context.reset() # reset the translations of the context
... print(':') # use the objects like before
... print(continents)
... print(continents[0].countries.all())
... print(continents[0].countries.all()[0].cities.all())
:
,
,
]>
,
]>
,
]>
:
,
,
]>
,
]>
,
]>
```This can CRUD the translations of any objects (instance, queryset, list) and their relations.
## Documentation
For more interesting capabilities browse through the
[documentation](http://bbmokhtari.github.io/django-translations).## Still Stuck?
Email [[email protected]](mailto:[email protected]) if you're really stuck.
## Support the project
To support the project you can:
- ⭐️: [Star](http://github.com/bbmokhtari/django-translations/) it on GitHub.
- 💻: [Contribute](https://bbmokhtari.github.io/django-translations/contribution.html) to the code base.
- ☕️: [Buy](https://bbmokhtari.github.io/django-translations/donation.html) the maintainers coffee.