https://github.com/bcapathshala/color-schema-switcher-project
This is a JavaScript practice project [ Color Schema Switcher Project ]
https://github.com/bcapathshala/color-schema-switcher-project
Last synced: 4 months ago
JSON representation
This is a JavaScript practice project [ Color Schema Switcher Project ]
- Host: GitHub
- URL: https://github.com/bcapathshala/color-schema-switcher-project
- Owner: BCAPATHSHALA
- Created: 2024-02-02T04:24:55.000Z (over 1 year ago)
- Default Branch: master
- Last Pushed: 2024-02-02T04:42:47.000Z (over 1 year ago)
- Last Synced: 2024-12-31T02:41:20.469Z (6 months ago)
- Language: CSS
- Homepage: https://color-schema-switcher-project.vercel.app
- Size: 25.4 KB
- Stars: 1
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: Readme.md
Awesome Lists containing this project
README
# Color Schema Switcher Project
This simple JavaScript project allows users to switch between predefined color schemes and generate a random background color. Below are the three main files for this project:
## Screenshots
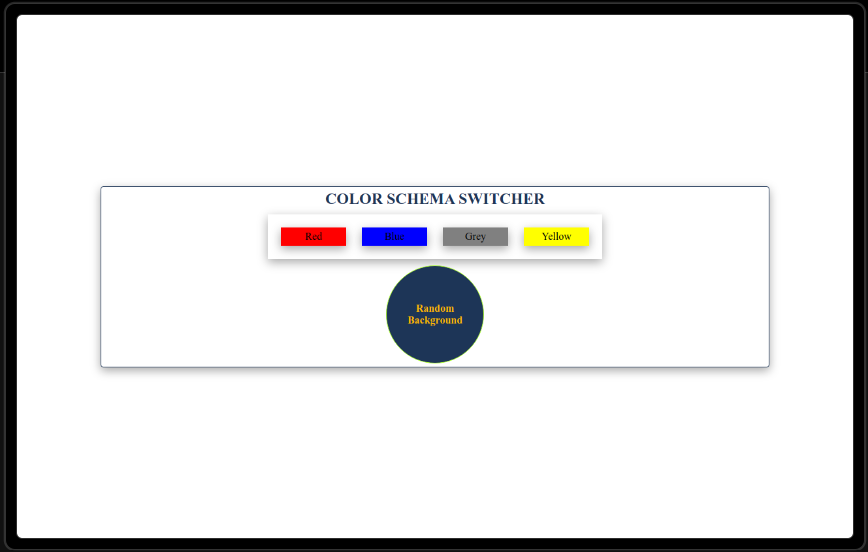
## HTML Code
```html
Color Schema Switcher
COLOR SCHEMA SWITCHER
Random
Background
```
## CSS Code
```css
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}body {
width: 100vw;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
position: relative;
}.container {
min-width: 80%;
border: 1px solid #1d3557;
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
gap: 10px;
border-radius: 4px;
box-shadow: #00000059 0px 5px 15px;
padding: 5px;
background-color: #ffffff;
}.container h1 {
color: #1d3557;
width: 100%;
text-align: center;
}
.buttons-container {
padding: 10px;
box-shadow: #00000059 0px 5px 15px;
}
.buttons-container > span {
display: inline-block;
width: 100px;
padding: 5px;
margin: 10px;
text-align: center;
box-shadow: #00000059 0px 5px 15px;
cursor: pointer;
}
#red {
background-color: red;
}
#blue {
background-color: blue;
}
#grey {
background-color: grey;
}
#yellow {
background-color: yellow;
}.random-background-btn {
background: #ffffff;
border: 1px solid greenyellow;
width: 150px;
height: 150px;
border-radius: 50%;
display: flex;
justify-content: center;
align-items: center;
text-align: center;
font-weight: 800;
background-color: #1d3557;
color: #ffb703;
cursor: pointer;
}
```## JavaScript Code
```javascript
const buttons = document.querySelectorAll(".buttons");
const body = document.body;buttons.forEach((button) => {
button.addEventListener("click", (e) => {
let color = e.target.id;
switch (color) {
case "red":
body.style.backgroundColor = color;
break;
case "blue":
body.style.backgroundColor = color;
break;
case "grey":
body.style.backgroundColor = color;
break;
case "yellow":
body.style.backgroundColor = color;
break;
}
});
});// Random Background Switcher
const randomBTN = document.querySelector(".random-background-btn");// Create function to generate the random color and return rgb
const randomColor = () => {
const min = 0;
const max = 255;
const red = Math.floor(Math.random() * (max - min + 1)) + min;
const green = Math.floor(Math.random() * (max - min + 1)) + min;
const blue = Math.floor(Math.random() * (max - min + 1)) + min;
const rgb = `rgb(${red}, ${green}, ${blue})`;
return rgb;
};randomBTN.addEventListener("click", () => {
const bg_color = randomColor();
body.style.backgroundColor = bg_color;
});
```