https://github.com/bezkoder/node-js-postgresql-crud-example
Node.js PostgreSQL CRUD example with Express Rest APIs
https://github.com/bezkoder/node-js-postgresql-crud-example
crud crud-api express express-js expressjs node node-js nodejs postgres postgresql rest-api restful-api
Last synced: 2 months ago
JSON representation
Node.js PostgreSQL CRUD example with Express Rest APIs
- Host: GitHub
- URL: https://github.com/bezkoder/node-js-postgresql-crud-example
- Owner: bezkoder
- Created: 2021-01-04T09:55:15.000Z (over 4 years ago)
- Default Branch: master
- Last Pushed: 2023-06-08T02:31:38.000Z (about 2 years ago)
- Last Synced: 2025-03-31T17:08:17.078Z (4 months ago)
- Topics: crud, crud-api, express, express-js, expressjs, node, node-js, nodejs, postgres, postgresql, rest-api, restful-api
- Language: JavaScript
- Homepage:
- Size: 3.91 KB
- Stars: 132
- Watchers: 3
- Forks: 103
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Node.js PostgreSQL CRUD example with Express Rest APIs
Full Article with implementation:
> [Node.js PostgreSQL CRUD example with Express Rest APIs](https://www.bezkoder.com/node-express-sequelize-postgresql/)We will build Rest Apis that can create, retrieve, update, delete and find Tutorials by title.
The following table shows overview of the Rest APIs that will be exported:
- GET `api/tutorials` get all Tutorials
- GET `api/tutorials/:id` get Tutorial by id
- POST `api/tutorials` add new Tutorial
- PUT `api/tutorials/:id` update Tutorial by id
- DELETE `api/tutorials/:id` remove Tutorial by id
- DELETE `api/tutorials` remove all Tutorials
- GET `api/tutorials/published` find all published Tutorials
- GET `api/tutorials?title=[kw]` find all Tutorials which title contains 'kw'Front-end that works well with this Back-end
> [Axios Client](https://www.bezkoder.com/axios-request/)> [Angular 8](https://www.bezkoder.com/angular-crud-app/) / [Angular 10](https://www.bezkoder.com/angular-10-crud-app/) / [Angular 11](https://www.bezkoder.com/angular-11-crud-app/) / [Angular 12](https://www.bezkoder.com/angular-12-crud-app/) / [Angular 13](https://www.bezkoder.com/angular-13-crud-example/) / [Angular 14](https://www.bezkoder.com/angular-14-crud-example/) / [Angular 15](https://www.bezkoder.com/angular-15-crud-example/) / [Angular 16](https://www.bezkoder.com/angular-16-crud-example/)
> [Vue 2 Client](https://www.bezkoder.com/vue-js-crud-app/) / [Vue 3 Client](https://www.bezkoder.com/vue-3-crud/) / [Vuetify Client](https://www.bezkoder.com/vuetify-data-table-example/)
> [React Client](https://www.bezkoder.com/react-crud-web-api/) / [React Redux Client](https://www.bezkoder.com/react-redux-crud-example/)
## Demo Video
This is our Node.js PostgreSQL CRUD example using Express & Sequelize application demo, test Rest Apis with Postman.[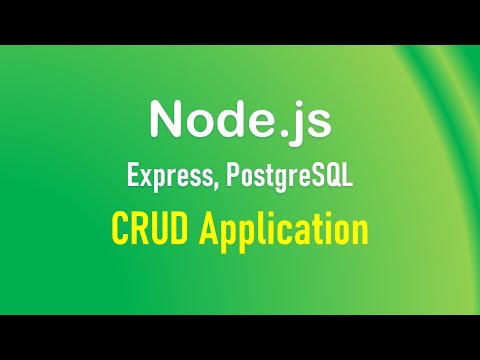](http://www.youtube.com/watch?v=x1pZHN_sjGk "Node.js PostgreSQL CRUD example Github")
### Test the APIs
Run our Node.js application with command: `node server.js`.Using Postman, we're gonna test all the Apis above.
- Create a new Tutorial using `POST /tutorials` Api
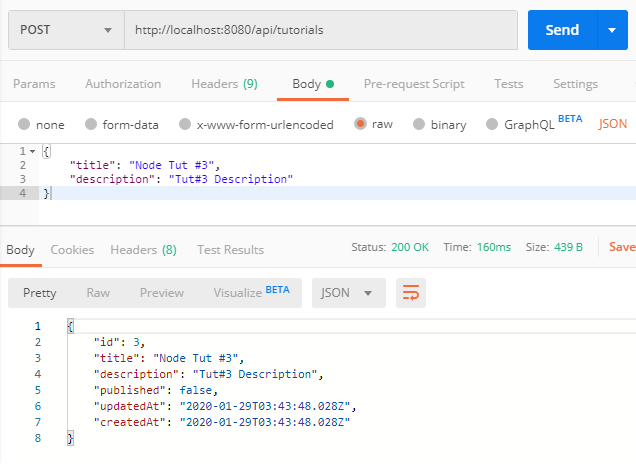
After creating some new Tutorials, you can check PostgreSQL table:
```testdb=# select * from tutorials;
id | title | description | published | createdAt | updatedAt
----+-------------+-------------------+-----------+----------------------------+----------------------------
1 | Node Tut #1 | Tut#1 Description | f | 2020-01-29 10:42:57.121+07 | 2020-01-29 10:42:57.121+07
2 | Node Tut #2 | Tut#2 Description | f | 2020-01-29 10:43:05.131+07 | 2020-01-29 10:43:05.131+07
3 | Node Tut #3 | Tut#3 Description | f | 2020-01-29 10:43:48.028+07 | 2020-01-29 10:43:48.028+07
4 | Js Tut #4 | Tut#4 Desc | f | 2020-01-29 10:45:40.016+07 | 2020-01-29 10:45:40.016+07
5 | Js Tut #5 | Tut#5 Desc | f | 2020-01-29 10:45:44.289+07 | 2020-01-29 10:45:44.289+07
```- Retrieve all Tutorials using `GET /tutorials` Api
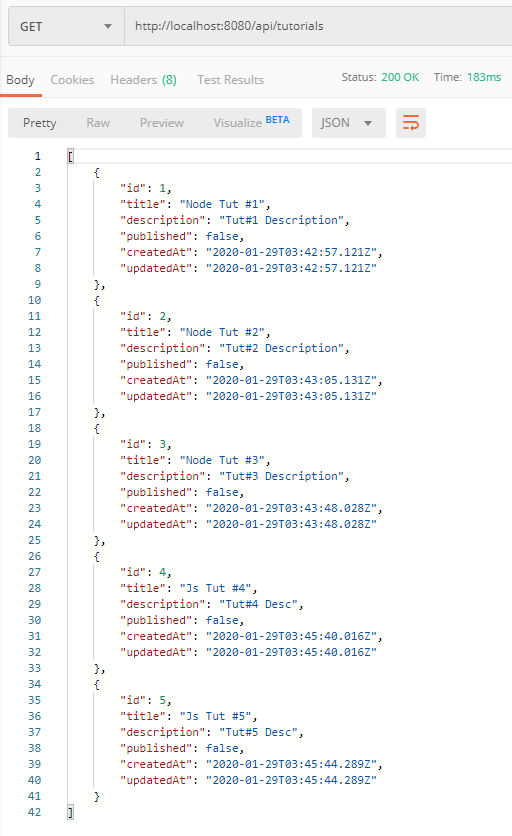
- Retrieve a single Tutorial by id using `GET /tutorials/:id` Api
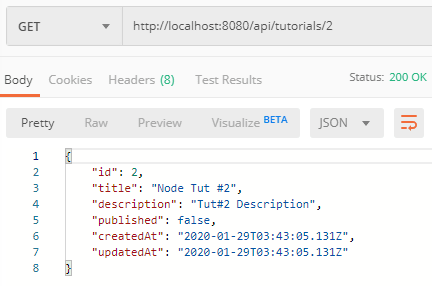
- Update a Tutorial using `PUT /tutorials/:id` Api
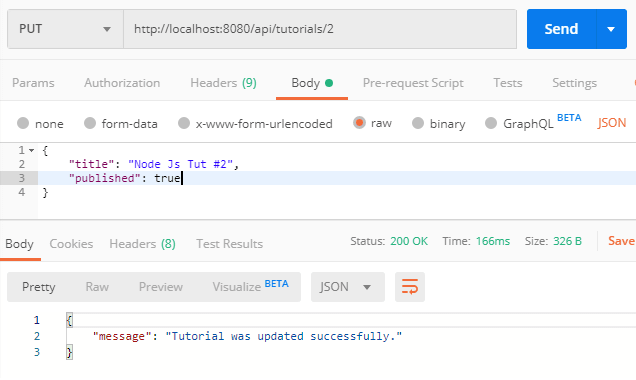
Check `tutorials` table after some rows were updated:
```testdb=# select * from tutorials;
id | title | description | published | createdAt | updatedAt
----+----------------+-------------------+-----------+----------------------------+----------------------------
1 | Node Tut #1 | Tut#1 Description | f | 2020-01-29 10:42:57.121+07 | 2020-01-29 10:42:57.121+07
3 | Node Tut #3 | Tut#3 Description | f | 2020-01-29 10:43:48.028+07 | 2020-01-29 10:43:48.028+07
2 | Node Js Tut #2 | Tut#2 Description | t | 2020-01-29 10:43:05.131+07 | 2020-01-29 10:51:55.235+07
4 | Js Tut #4 | Tut#4 Desc | t | 2020-01-29 10:45:40.016+07 | 2020-01-29 10:54:17.468+07
5 | Js Tut #5 | Tut#5 Desc | t | 2020-01-29 10:45:44.289+07 | 2020-01-29 10:54:20.544+07
```- Find all Tutorials which title contains 'js': `GET /tutorials?title=js`
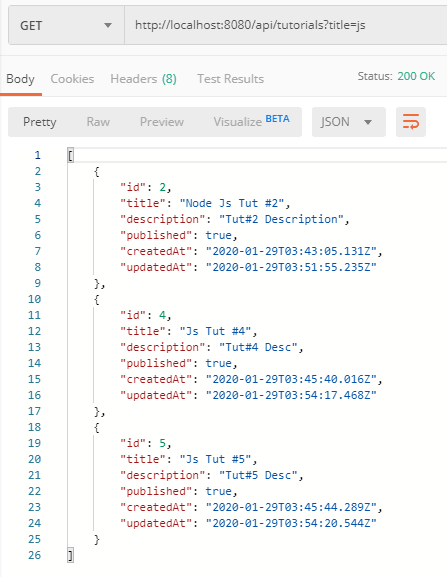
- Find all published Tutorials using `GET /tutorials/published` Api
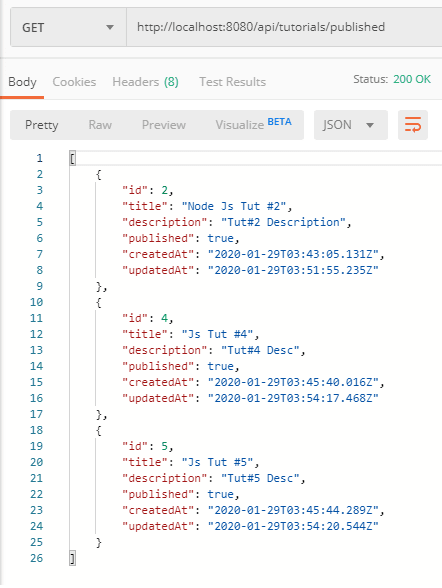
- Delete a Tutorial using `DELETE /tutorials/:id` Api
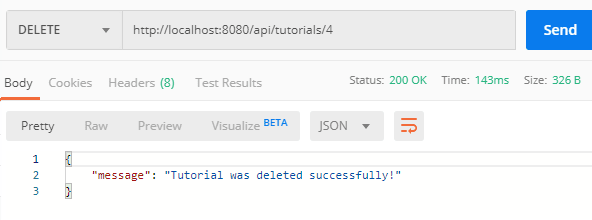
Tutorial with id=4 was removed from `tutorials` table:
```testdb=# select * from tutorials;
id | title | description | published | createdAt | updatedAt
----+----------------+-------------------+-----------+----------------------------+----------------------------
1 | Node Tut #1 | Tut#1 Description | f | 2020-01-29 10:42:57.121+07 | 2020-01-29 10:42:57.121+07
3 | Node Tut #3 | Tut#3 Description | f | 2020-01-29 10:43:48.028+07 | 2020-01-29 10:43:48.028+07
2 | Node Js Tut #2 | Tut#2 Description | t | 2020-01-29 10:43:05.131+07 | 2020-01-29 10:51:55.235+07
5 | Js Tut #5 | Tut#5 Desc | t | 2020-01-29 10:45:44.289+07 | 2020-01-29 10:54:20.544+07
```- Delete all Tutorials using `DELETE /tutorials` Api
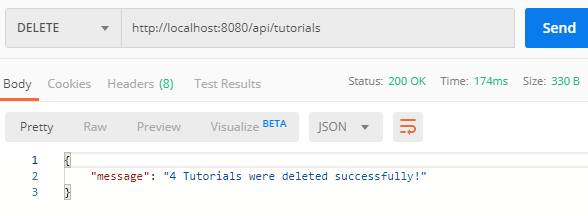
Now there are no rows in `tutorials` table:
```testdb=# select * from tutorials;
id | title | description | published | createdAt | updatedAt
----+-------+-------------+-----------+-----------+-----------
```For more detail, please visit:
> [Node.js PostgreSQL CRUD example with Express Rest APIs](https://www.bezkoder.com/node-express-sequelize-postgresql/)> [Node.js Express Pagination with PostgreSQL example](https://www.bezkoder.com/node-js-pagination-postgresql/)
Security:
> [Node.js JWT Authentication & Authorization with PostgreSQL example](https://www.bezkoder.com/node-js-jwt-authentication-postgresql/)Associations:
> [Sequelize Associations: One-to-Many Relationship example](https://www.bezkoder.com/sequelize-associate-one-to-many/)> [Sequelize Associations: Many-to-Many Relationship example](https://www.bezkoder.com/sequelize-associate-many-to-many/)
Fullstack:
> [Vue + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/vue-node-express-postgresql/)> [React + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/react-node-express-postgresql/)
> [Angular 8 + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/angular-node-express-postgresql/)
> [Angular 10 + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/angular-10-node-express-postgresql/)
> [Angular 11 + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/angular-11-node-js-express-postgresql/)
> [Angular 12 + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/angular-12-node-js-express-postgresql/)
> [Angular 13 + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/angular-13-node-js-express-postgresql/)
> [Angular 14 + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/angular-14-node-js-express-postgresql/)
> [Angular 15 + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/angular-15-node-js-express-postgresql/)
> [Angular 16 + Node.js + Express + PostgreSQL example](https://www.bezkoder.com/angular-16-node-js-express-postgresql/)
Integration (run back-end & front-end on same server/port):
> [Integrate React with Node.js Restful Services](https://www.bezkoder.com/integrate-react-express-same-server-port/)> [Integrate Angular with Node.js Restful Services](https://www.bezkoder.com/integrate-angular-12-node-js/)
> [Integrate Vue with Node.js Restful Services](https://www.bezkoder.com/serve-vue-app-express/)
## Project setup
```
npm install
```### Run
```
node server.js
```