Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/borgo-lang/borgo
Borgo is a statically typed language that compiles to Go.
https://github.com/borgo-lang/borgo
compiler golang programming-language rust-lang
Last synced: 3 days ago
JSON representation
Borgo is a statically typed language that compiles to Go.
- Host: GitHub
- URL: https://github.com/borgo-lang/borgo
- Owner: borgo-lang
- Created: 2023-02-17T03:04:27.000Z (almost 2 years ago)
- Default Branch: main
- Last Pushed: 2024-10-27T10:07:54.000Z (3 months ago)
- Last Synced: 2025-01-15T23:03:40.806Z (10 days ago)
- Topics: compiler, golang, programming-language, rust-lang
- Language: Rust
- Homepage: https://borgo-lang.github.io
- Size: 1000 KB
- Stars: 4,299
- Watchers: 30
- Forks: 58
- Open Issues: 29
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
- awesome-ccamel - borgo-lang/borgo - Borgo is a statically typed language that compiles to Go. (Rust)
- awesome-rainmana - borgo-lang/borgo - Borgo is a statically typed language that compiles to Go. (Rust)
README
# The Borgo Programming Language
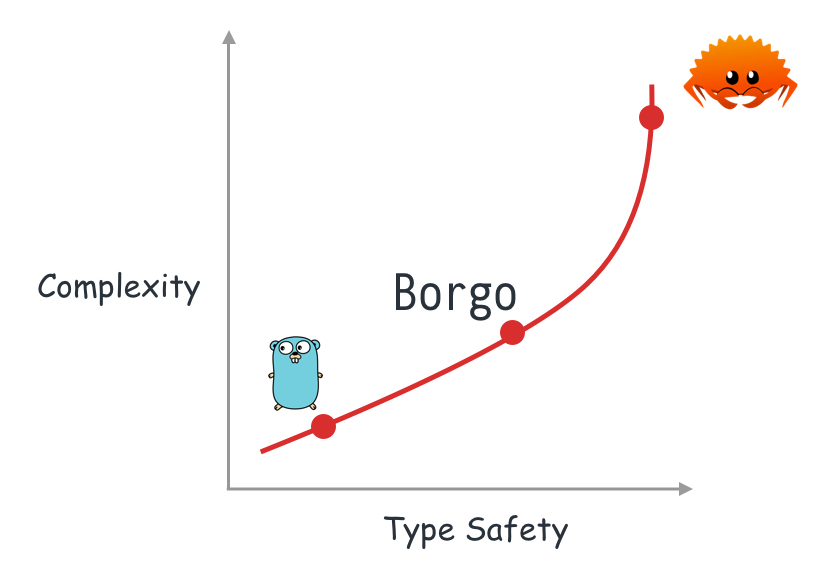
---

I want a language for writing applications that is more expressive than Go but
less complex than Rust.Go is simple and straightforward, but I often wish it offered more type safety.
Rust is very nice to work with (at least for single threaded code) but it's too
broad and complex, sometimes painfully so.**Borgo is a new language that transpiles to Go**. It's fully compatible with
existing Go packages.Borgo syntax is similar to Rust, with optional semi-colons.
# Tutorial
Check out the **[online playground](https://borgo-lang.github.io/)** for a tour
of the language.You can also take a look at test files for working Borgo code:
- [codegen-emit.md](compiler/test/codegen-emit.md)
- [infer-expr.md](compiler/test/infer-expr.md)
- [infer-file.md](compiler/test/infer-file.md)# Features
## Algebraic data types and pattern matching
```rust
use fmtenum NetworkState {
Loading,
Failed(int),
Success(string),
}let msg = match state {
NetworkState.Loading => "still loading",
NetworkState.Failed(code) => fmt.Sprintf("Got error code: %d", code),
NetworkState.Success(res) => res,
}
```---
## `Option` instead of `nil`
```rust
// import packages from Go stdlib
use fmt
use oslet key = os.LookupEnv("HOME")
match key {
Some(s) => fmt.Println("home dir:", s),
None => fmt.Println("Not found in env"),
}
```---
## `Result` instead of multiple return values
```rust
use fmt
use net.httpfn makeRequest() -> Result {
let request = http.Get("http://example.com")match request {
Ok(resp) => Ok(resp.StatusCode),
Err(err) => Err(fmt.Errorf("failed http request %w", err))
}
}
```---
## Error handling with `?` operator
```rust
use fmt
use io
use osfn copyFile(src: string, dst: string) -> Result<(), error> {
let stat = os.Stat(src)?if !stat.Mode().IsRegular() {
return Err(fmt.Errorf("%s is not a regular file", src))
}let source = os.Open(src)?
defer source.Close()let destination = os.Create(dst)?
defer destination.Close()// ignore number of bytes copied
let _ = io.Copy(destination, source)?Ok(())
}
```---
## Guessing game example
Small game from the Rust book, implemented in Borgo.
Things to note:
- import packages from Go stdlib
- `strconv.Atoi` returns an `Option`
- `Reader.ReadString` returns a `Result` (which can be unwrapped)```rust
use bufio
use fmt
use math.rand
use os
use strconv
use stringsfn main() {
let reader = bufio.NewReader(os.Stdin)let secret = rand.Intn(100) + 1
loop {
fmt.Println("Please input your guess.")let text = reader.ReadString('\n').Unwrap()
let text = strings.TrimSpace(text)let guess = match strconv.Atoi(text) {
Ok(n) => n,
Err(_) => continue,
}fmt.Println("You guessed: ", guess)
if guess < secret {
fmt.Println("Too small!")
} else if guess > secret {
fmt.Println("Too big!")
} else {
fmt.Println("Correct!")
break
}
}
}
```## Running locally
Borgo is written in Rust, so you'll need `cargo`.
To compile all `.brg` files in the current folder:
```bash
$ cargo run -- build
```The compiler will generate `.go` files, which you can run as normal:
```bash
# generate a go.mod file if needed
# $ go mod init foo
$ go run .
```