Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/brentvatne/react-native-overlay
An <Overlay /> component that brings content inside to the front of the view regardless of its current position in the component tree.
https://github.com/brentvatne/react-native-overlay
Last synced: about 2 months ago
JSON representation
An <Overlay /> component that brings content inside to the front of the view regardless of its current position in the component tree.
- Host: GitHub
- URL: https://github.com/brentvatne/react-native-overlay
- Owner: brentvatne
- Created: 2015-04-12T05:28:10.000Z (over 9 years ago)
- Default Branch: master
- Last Pushed: 2016-09-22T16:57:56.000Z (about 8 years ago)
- Last Synced: 2024-05-03T01:54:13.563Z (5 months ago)
- Language: Objective-C
- Size: 1.59 MB
- Stars: 639
- Watchers: 13
- Forks: 82
- Open Issues: 24
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
- awesome-react-native-native-modules - react-native-overlay ★588
README
## react-native-overlay
An `` component that brings content inside to the front of the view regardless of its current position in the component tree. This was extracted from [react-native-modal](https://github.com/brentvatne/react-native-modal) because a modal is not the only time that you want to bring something to the front of the screen.
### Should you use this?
Ideally, no. This should probably only be used as a last resort. You can usually accomplish what you need to by just absolute positioning an view at the bottom of your root component.
*In fact, as of 0.29.0 zIndex is supported on iOS and Android, so you should probably never use this.*
### Add it to your project
1. Run `npm install react-native-overlay --save`
2. Open your project in XCode, right click on `Libraries` and click `Add
Files to "Your Project Name"` [(Screenshot)](http://url.brentvatne.ca/jQp8) then [(Screenshot)](http://url.brentvatne.ca/1gqUD).
3. Add `libRNOverlay.a` to `Build Phases -> Link Binary With Libraries`
[(Screenshot)](http://url.brentvatne.ca/17Xfe).
4. Whenever you want to use it within React code now you can: `var Overlay = require('react-native-overlay');`## Example - Loading Overlay
This shows how you might implement a loading overlay and uses
[react-native-blur](http://github.com/kureev/react-native-blur) to blur
the background. Notice that all we need to do is wrap the content that
we want to bring to the front in an `Overlay` element!```javascript
var React = require('react-native');
var Overlay = require('react-native-overlay');
var BlurView = require('react-native-blur').BlurView;var {
View,
ActivityIndicatorIOS,
StyleSheet,
} = React;var LoadingOverlay = React.createClass({
getDefaultProps(): StateObject {
return {
isVisible: false
}
},render(): ReactElement {
return (
);
}
});var styles = StyleSheet.create({
background: {
flex: 1,
justifyContent: 'center',
},
})module.exports = LoadingOverlay;
```Elsewhere in our app, we can render this:
```javascript
var LoadingOverlayExampleApp = React.createClass({
render: function() {
return (
{ /* It doesn't matter where we put this component, it can be nested */ }
{ /* anywhere within your component tree */ }
);
}
});
```
This would produce something like this: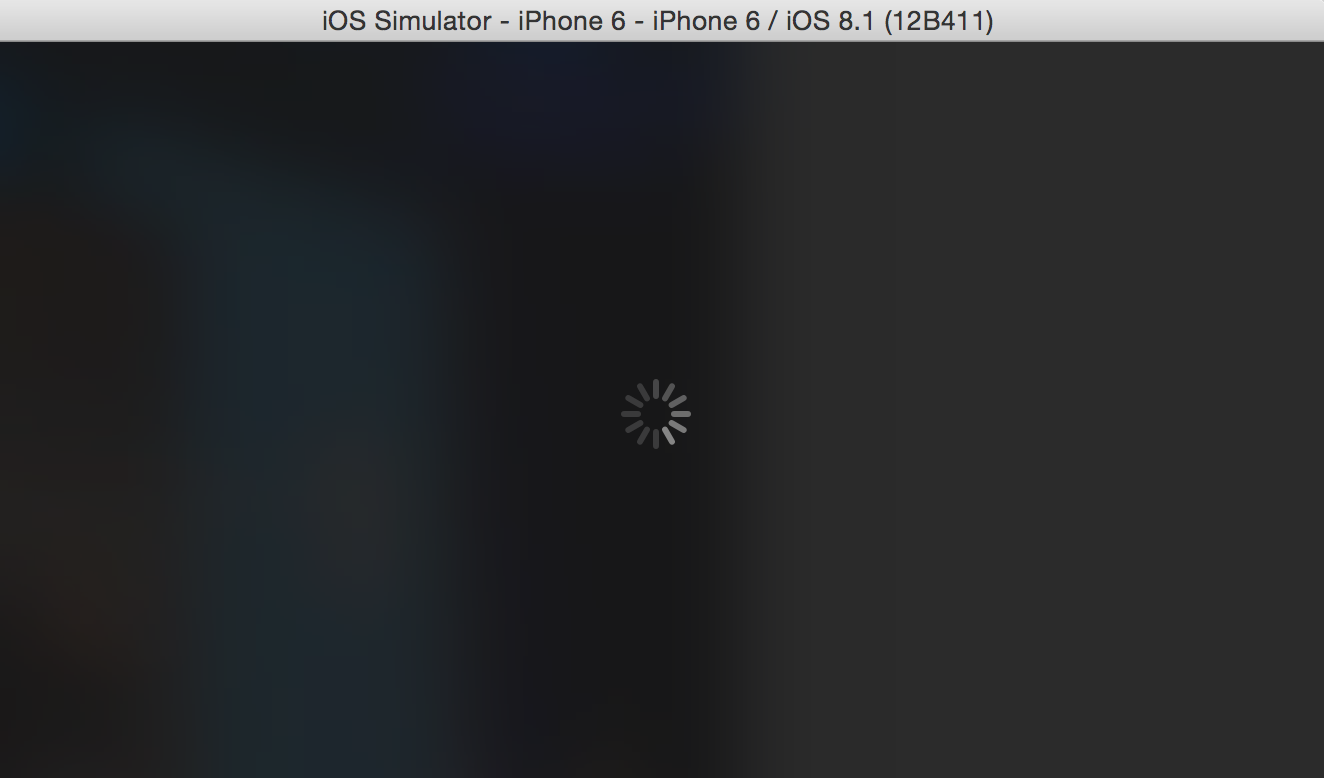
You can try this code yourself by cloning this repo and running
`Examples/LoadingOverlay`.## Example - Toast
There are so many other types of overlays but I thought I'd give another
simple example to stir your imagination.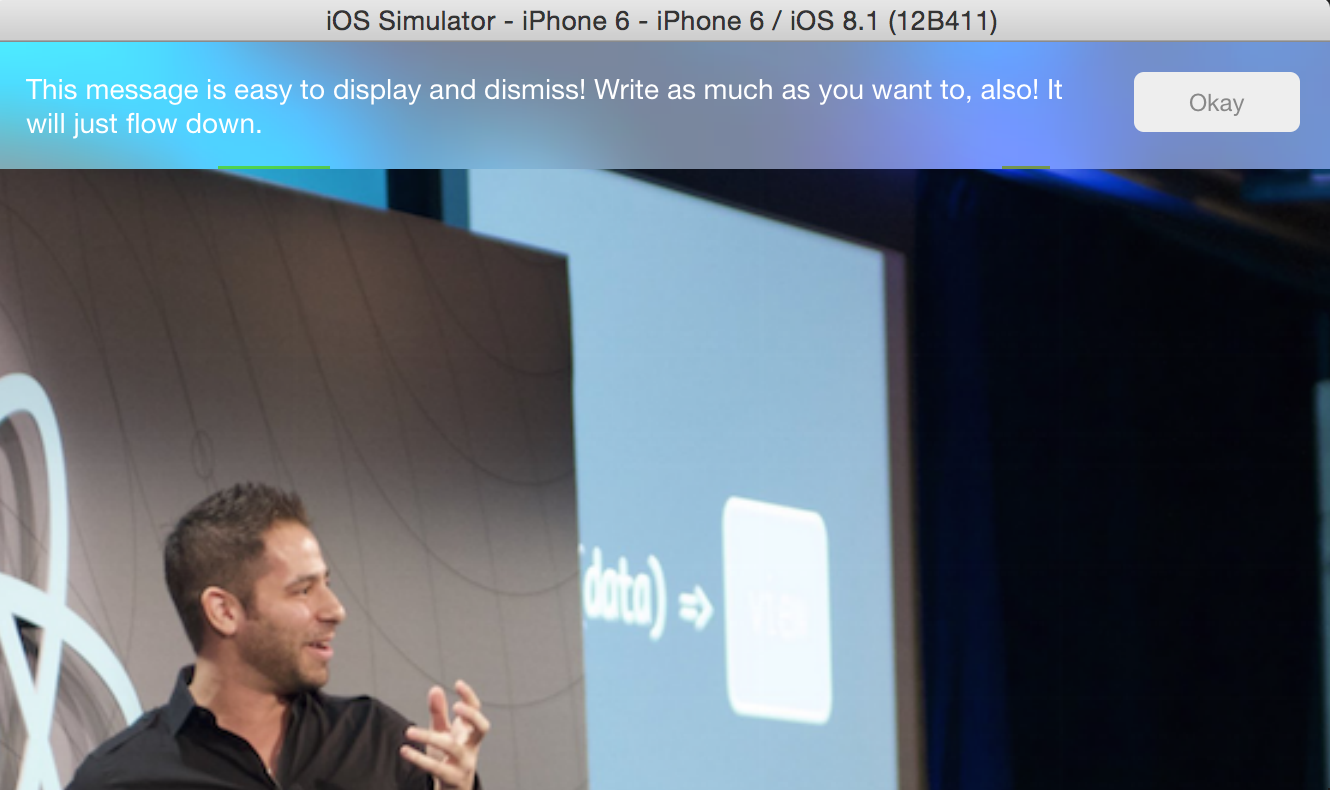
Check it out in `Examples/Toast`.