https://github.com/bryanmylee/svelte-previous
A Svelte store that remembers previous values
https://github.com/bryanmylee/svelte-previous
history previous svelte svelte-stores
Last synced: 4 months ago
JSON representation
A Svelte store that remembers previous values
- Host: GitHub
- URL: https://github.com/bryanmylee/svelte-previous
- Owner: bryanmylee
- License: mit
- Created: 2020-12-20T11:02:49.000Z (over 4 years ago)
- Default Branch: master
- Last Pushed: 2023-10-17T11:17:33.000Z (over 1 year ago)
- Last Synced: 2024-12-14T02:41:47.431Z (5 months ago)
- Topics: history, previous, svelte, svelte-stores
- Language: TypeScript
- Homepage:
- Size: 1.08 MB
- Stars: 83
- Watchers: 1
- Forks: 2
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- License: LICENSE
- Security: SECURITY.md
Awesome Lists containing this project
- awesome-svelte-stores - svelte-previous
README
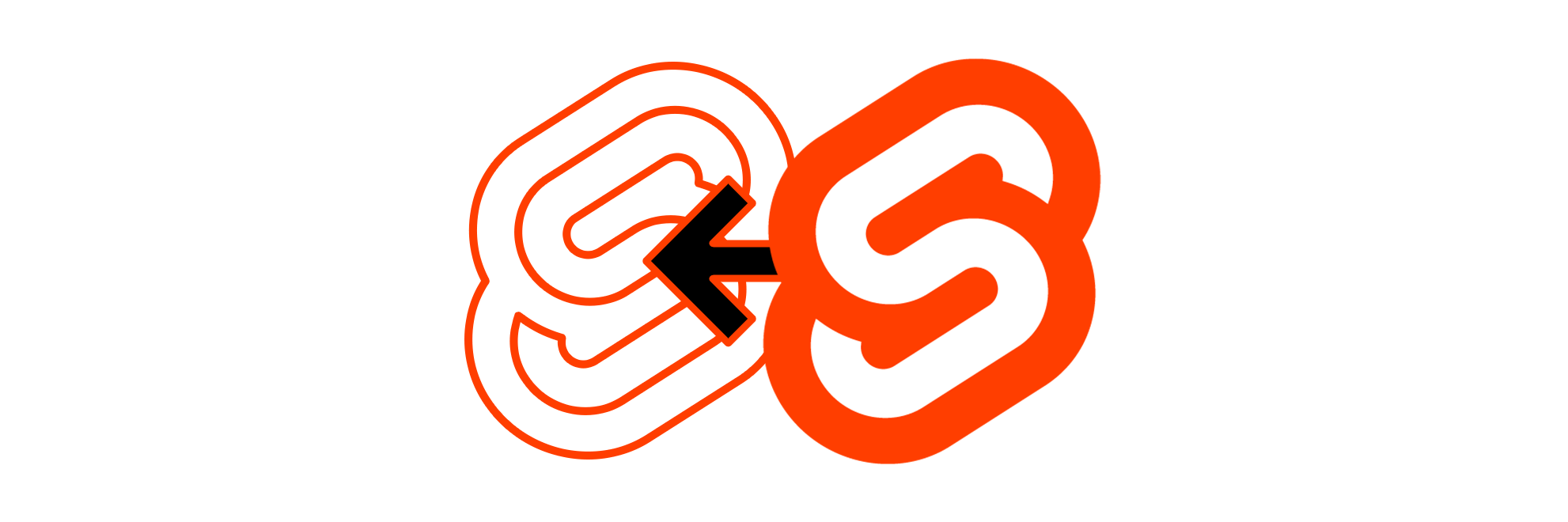
# svelte-previous
[](https://www.npmjs.com/package/svelte-previous)
[](https://www.npmjs.com/package/svelte-previous)


[](https://coveralls.io/github/bryanmylee/svelte-previous?branch=master)
[](https://bundlephobia.com/result?p=svelte-previous)Svelte stores that remember previous values!
This allows us to perform actions that depend on previous values, such as transitions between old and new values.
## Installation
```bash
$ npm i -D svelte-previous
```Since Svelte automatically bundles all required dependencies, you only need to install this package as a dev dependency with the -D flag.
## Demo
Visit the [REPL demo](https://svelte.dev/repl/1d3e752c51b848e6af264f3244f3e85c?version=3.31.0).
## Usage
`withPrevious` accepts an initial value, and returns a tuple comprising a [Writable](https://svelte.dev/tutorial/writable-stores) and a [Readable](https://svelte.dev/tutorial/readable-stores) store.
```svelte
import { withPrevious } from 'svelte-previous';
export let name;
// current is writable, while previous is read-only.
const [currentName, previousName] = withPrevious(0);
// To update the values, assign to the writable store.
$: $currentName = name;transition from {$previousName} to {$currentName}.
```## Options
`withPrevious` takes an options object as its second argument.
### `numToTrack: number`
By default, `withPrevious` tracks one previous value.
To track more than one value, set `numToTrack`.
```svelte
const [current, prev1, prev2] = withPrevious(0, { numToTrack: 2 });
from {$prev2} to {$prev1} to {$current}.
```### `initPrevious: T[]`
To initialize previous values with something besides `null`, pass an array of values from newest to oldest.
Missing values will be filled with `null` and extra values will be ignored.
```svelte
const [current, prev1, prev2] = withPrevious(0, { numToTrack: 2, initPrevious: [1, 2, 3] })
from {$prev2} to {$prev1} to {$current}.
```### `requireChange: boolean`
Due to how reactivity is handled in Svelte, some assignments may assign the same value multiple times to a variable. Therefore, to prevent a single value from overwriting all previous values, a change in value is required before the current and previous values are updated.
Set `requireChange = false` to change this behaviour.
```ts
const [current, previous] = withPrevious(0, { requireChange: false });
```### `isEqual: (a: T, b: T) => boolean`
By default, equality is determined with the `===` operator. However, `===` only checks equality by reference when comparing objects.
Provide a custom `isEqual` function to compare objects.
```ts
const [current, previous] = withPrevious(0, {
isEqual: (a, b) => a.name === b.name && a.age === b.age,
});
```It is also possible to use [lodash.isequal](https://www.npmjs.com/package/lodash.isequal).
```ts
import isEqual from 'lodash.isequal';const [current, previous] = withPrevious(0, {
isEqual: isEqual,
});
```