https://github.com/bunlong/react-papaparse
react-papaparse is the fastest in-browser CSV (or delimited text) parser for React. It is full of useful features such as CSVReader, CSVDownloader, readString, jsonToCSV, readRemoteFile, ... etc.
https://github.com/bunlong/react-papaparse
browser-csv csv csv-parser csv-reader csv-to-json csvreader csvtojson input json-to-csv jsontocsv multithread parsers react react-csv react-csv-parser react-csv-reader react-papaparse stream thread typescript
Last synced: 6 days ago
JSON representation
react-papaparse is the fastest in-browser CSV (or delimited text) parser for React. It is full of useful features such as CSVReader, CSVDownloader, readString, jsonToCSV, readRemoteFile, ... etc.
- Host: GitHub
- URL: https://github.com/bunlong/react-papaparse
- Owner: Bunlong
- License: mit
- Created: 2020-02-17T01:46:49.000Z (over 5 years ago)
- Default Branch: master
- Last Pushed: 2024-08-11T14:04:36.000Z (9 months ago)
- Last Synced: 2025-05-13T19:14:33.961Z (8 days ago)
- Topics: browser-csv, csv, csv-parser, csv-reader, csv-to-json, csvreader, csvtojson, input, json-to-csv, jsontocsv, multithread, parsers, react, react-csv, react-csv-parser, react-csv-reader, react-papaparse, stream, thread, typescript
- Language: TypeScript
- Homepage: https://react-papaparse.js.org
- Size: 15.8 MB
- Stars: 380
- Watchers: 2
- Forks: 63
- Open Issues: 55
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE
- Code of conduct: CODE_OF_CONDUCT.md
- Support: supports/create-next-app/.eslintrc.json
Awesome Lists containing this project
README
# react-papaparse
react-papaparse is the fastest in-browser CSV (or delimited text) parser for React. It is full of useful features such as CSVReader, CSVDownloader, readString, jsonToCSV, readRemoteFile, ... etc.
[](https://www.npmjs.com/package/react-papaparse) [](https://www.npmjs.com/package/react-papaparse)
[](https://www.npmjs.com/package/react-papaparse)  [](https://travis-ci.com/Bunlong/react-papaparse) [](https://standardjs.com)
## 🎁 Features
* Compatible with both JavaScript and TypeScript
* Easy to use
* Parse CSV files directly (local or over the network)
* Fast mode (is really fast)
* Stream large files (even via HTTP)
* Reverse parsing (converts JSON to CSV)
* Auto-detect delimiter
* Worker threads to keep your web page reactive
* Header row support
* Pause, resume, abort
* Can convert numbers and booleans to their types
* One of the only parsers that correctly handles line-breaks and quotations## 🔧 Install
react-papaparse is available on npm. It can be installed with the following command:
```
npm install react-papaparse --save
```react-papaparse is available on yarn as well. It can be installed with the following command:
```
yarn add react-papaparse --save
```## 📖 Demo & Documentation
* [Demo](https://react-papaparse.github.io/demo)
To learn how to use react-papaparse:
* [Documentation](https://react-papaparse.github.io/docs)
## 📚 Useful Features
* [CSVReader](https://github.com/Bunlong/react-papaparse#-csvreader) – React component that handles csv files input and returns its content as array.
* [CSVDownloader](https://github.com/Bunlong/react-papaparse#-csvdownloader) – React component that render the link/button which is clicked to download the data provided in CSV format.
* [readString](https://github.com/Bunlong/react-papaparse#-readstring) – The function that read CSV comma separated string and returns its content as array.
* [readRemoteFile](https://github.com/Bunlong/react-papaparse#-readremotefile) – The function that read remote CSV files and returns its content as array.
* [jsonToCSV](https://github.com/Bunlong/react-papaparse#-jsontocsv) – The function that read an array of object (json) and returns its content as CSV comma separated string.## 💡 Usage
### 🎀 CSVReader
#### Basic Upload

```javascript
import React, { CSSProperties } from 'react';import { useCSVReader } from 'react-papaparse';
const styles = {
csvReader: {
display: 'flex',
flexDirection: 'row',
marginBottom: 10,
} as CSSProperties,
browseFile: {
width: '20%',
} as CSSProperties,
acceptedFile: {
border: '1px solid #ccc',
height: 45,
lineHeight: 2.5,
paddingLeft: 10,
width: '80%',
} as CSSProperties,
remove: {
borderRadius: 0,
padding: '0 20px',
} as CSSProperties,
progressBarBackgroundColor: {
backgroundColor: 'red',
} as CSSProperties,
};export default function CSVReader() {
const { CSVReader } = useCSVReader();return (
{
console.log('---------------------------');
console.log(results);
console.log('---------------------------');
}}
>
{({
getRootProps,
acceptedFile,
ProgressBar,
getRemoveFileProps,
}: any) => (
<>
Browse file
{acceptedFile && acceptedFile.name}
Remove
>
)}
);
}
```#### Click and Drag Upload
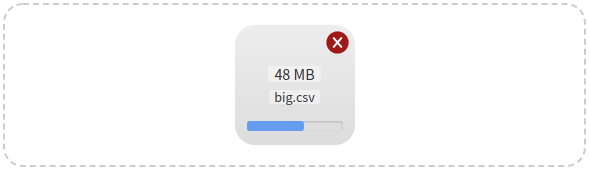
```javascript
import React, { useState, CSSProperties } from 'react';import {
useCSVReader,
lightenDarkenColor,
formatFileSize,
} from 'react-papaparse';const GREY = '#CCC';
const GREY_LIGHT = 'rgba(255, 255, 255, 0.4)';
const DEFAULT_REMOVE_HOVER_COLOR = '#A01919';
const REMOVE_HOVER_COLOR_LIGHT = lightenDarkenColor(
DEFAULT_REMOVE_HOVER_COLOR,
40
);
const GREY_DIM = '#686868';const styles = {
zone: {
alignItems: 'center',
border: `2px dashed ${GREY}`,
borderRadius: 20,
display: 'flex',
flexDirection: 'column',
height: '100%',
justifyContent: 'center',
padding: 20,
} as CSSProperties,
file: {
background: 'linear-gradient(to bottom, #EEE, #DDD)',
borderRadius: 20,
display: 'flex',
height: 120,
width: 120,
position: 'relative',
zIndex: 10,
flexDirection: 'column',
justifyContent: 'center',
} as CSSProperties,
info: {
alignItems: 'center',
display: 'flex',
flexDirection: 'column',
paddingLeft: 10,
paddingRight: 10,
} as CSSProperties,
size: {
backgroundColor: GREY_LIGHT,
borderRadius: 3,
marginBottom: '0.5em',
justifyContent: 'center',
display: 'flex',
} as CSSProperties,
name: {
backgroundColor: GREY_LIGHT,
borderRadius: 3,
fontSize: 12,
marginBottom: '0.5em',
} as CSSProperties,
progressBar: {
bottom: 14,
position: 'absolute',
width: '100%',
paddingLeft: 10,
paddingRight: 10,
} as CSSProperties,
zoneHover: {
borderColor: GREY_DIM,
} as CSSProperties,
default: {
borderColor: GREY,
} as CSSProperties,
remove: {
height: 23,
position: 'absolute',
right: 6,
top: 6,
width: 23,
} as CSSProperties,
};export default function CSVReader() {
const { CSVReader } = useCSVReader();
const [zoneHover, setZoneHover] = useState(false);
const [removeHoverColor, setRemoveHoverColor] = useState(
DEFAULT_REMOVE_HOVER_COLOR
);return (
{
console.log('---------------------------');
console.log(results);
console.log('---------------------------');
setZoneHover(false);
}}
onDragOver={(event: DragEvent) => {
event.preventDefault();
setZoneHover(true);
}}
onDragLeave={(event: DragEvent) => {
event.preventDefault();
setZoneHover(false);
}}
>
{({
getRootProps,
acceptedFile,
ProgressBar,
getRemoveFileProps,
Remove,
}: any) => (
<>
{acceptedFile ? (
<>
{formatFileSize(acceptedFile.size)}
{acceptedFile.name}
{
event.preventDefault();
setRemoveHoverColor(REMOVE_HOVER_COLOR_LIGHT);
}}
onMouseOut={(event: Event) => {
event.preventDefault();
setRemoveHoverColor(DEFAULT_REMOVE_HOVER_COLOR);
}}
>
>
) : (
'Drop CSV file here or click to upload'
)}
>
)}
);
}
```#### Drag ( No Click ) Upload

```javascript
import React, { useState, CSSProperties } from 'react';import {
useCSVReader,
lightenDarkenColor,
formatFileSize,
} from 'react-papaparse';const GREY = '#CCC';
const GREY_LIGHT = 'rgba(255, 255, 255, 0.4)';
const DEFAULT_REMOVE_HOVER_COLOR = '#A01919';
const REMOVE_HOVER_COLOR_LIGHT = lightenDarkenColor(
DEFAULT_REMOVE_HOVER_COLOR,
40
);
const GREY_DIM = '#686868';const styles = {
zone: {
alignItems: 'center',
border: `2px dashed ${GREY}`,
borderRadius: 20,
display: 'flex',
flexDirection: 'column',
height: '100%',
justifyContent: 'center',
padding: 20,
} as CSSProperties,
file: {
background: 'linear-gradient(to bottom, #EEE, #DDD)',
borderRadius: 20,
display: 'flex',
height: 120,
width: 120,
position: 'relative',
zIndex: 10,
flexDirection: 'column',
justifyContent: 'center',
} as CSSProperties,
info: {
alignItems: 'center',
display: 'flex',
flexDirection: 'column',
paddingLeft: 10,
paddingRight: 10,
} as CSSProperties,
size: {
backgroundColor: GREY_LIGHT,
borderRadius: 3,
marginBottom: '0.5em',
justifyContent: 'center',
display: 'flex',
} as CSSProperties,
name: {
backgroundColor: GREY_LIGHT,
borderRadius: 3,
fontSize: 12,
marginBottom: '0.5em',
} as CSSProperties,
progressBar: {
bottom: 14,
position: 'absolute',
width: '100%',
paddingLeft: 10,
paddingRight: 10,
} as CSSProperties,
zoneHover: {
borderColor: GREY_DIM,
} as CSSProperties,
default: {
borderColor: GREY,
} as CSSProperties,
remove: {
height: 23,
position: 'absolute',
right: 6,
top: 6,
width: 23,
} as CSSProperties,
};export default function CSVReader() {
const { CSVReader } = useCSVReader();
const [zoneHover, setZoneHover] = useState(false);
const [removeHoverColor, setRemoveHoverColor] = useState(
DEFAULT_REMOVE_HOVER_COLOR
);return (
{
console.log('---------------------------');
console.log(results);
console.log('---------------------------');
setZoneHover(false);
}}
onDragOver={(event: DragEvent) => {
event.preventDefault();
setZoneHover(true);
}}
onDragLeave={(event: DragEvent) => {
event.preventDefault();
setZoneHover(false);
}}
noClick
>
{({
getRootProps,
acceptedFile,
ProgressBar,
getRemoveFileProps,
Remove,
}: any) => (
<>
{acceptedFile ? (
<>
{formatFileSize(acceptedFile.size)}
{acceptedFile.name}
{
event.preventDefault();
setRemoveHoverColor(REMOVE_HOVER_COLOR_LIGHT);
}}
onMouseOut={(event: Event) => {
event.preventDefault();
setRemoveHoverColor(DEFAULT_REMOVE_HOVER_COLOR);
}}
>
>
) : (
'Drop CSV file here to upload'
)}
>
)}
);
}
```#### Click ( No Drag ) Upload

```javascript
import React, { useState, CSSProperties } from 'react';import {
useCSVReader,
lightenDarkenColor,
formatFileSize,
} from 'react-papaparse';const GREY = '#CCC';
const GREY_LIGHT = 'rgba(255, 255, 255, 0.4)';
const DEFAULT_REMOVE_HOVER_COLOR = '#A01919';
const REMOVE_HOVER_COLOR_LIGHT = lightenDarkenColor(
DEFAULT_REMOVE_HOVER_COLOR,
40
);
const GREY_DIM = '#686868';const styles = {
zone: {
alignItems: 'center',
border: `2px dashed ${GREY}`,
borderRadius: 20,
display: 'flex',
flexDirection: 'column',
height: '100%',
justifyContent: 'center',
padding: 20,
} as CSSProperties,
file: {
background: 'linear-gradient(to bottom, #EEE, #DDD)',
borderRadius: 20,
display: 'flex',
height: 120,
width: 120,
position: 'relative',
zIndex: 10,
flexDirection: 'column',
justifyContent: 'center',
} as CSSProperties,
info: {
alignItems: 'center',
display: 'flex',
flexDirection: 'column',
paddingLeft: 10,
paddingRight: 10,
} as CSSProperties,
size: {
backgroundColor: GREY_LIGHT,
borderRadius: 3,
marginBottom: '0.5em',
justifyContent: 'center',
display: 'flex',
} as CSSProperties,
name: {
backgroundColor: GREY_LIGHT,
borderRadius: 3,
fontSize: 12,
marginBottom: '0.5em',
} as CSSProperties,
progressBar: {
bottom: 14,
position: 'absolute',
width: '100%',
paddingLeft: 10,
paddingRight: 10,
} as CSSProperties,
zoneHover: {
borderColor: GREY_DIM,
} as CSSProperties,
default: {
borderColor: GREY,
} as CSSProperties,
remove: {
height: 23,
position: 'absolute',
right: 6,
top: 6,
width: 23,
} as CSSProperties,
};export default function CSVReader() {
const { CSVReader } = useCSVReader();
const [zoneHover, setZoneHover] = useState(false);
const [removeHoverColor, setRemoveHoverColor] = useState(
DEFAULT_REMOVE_HOVER_COLOR
);return (
{
console.log('---------------------------');
console.log(results);
console.log('---------------------------');
setZoneHover(false);
}}
onDragOver={(event: DragEvent) => {
event.preventDefault();
setZoneHover(true);
}}
onDragLeave={(event: DragEvent) => {
event.preventDefault();
setZoneHover(false);
}}
noDrag
>
{({
getRootProps,
acceptedFile,
ProgressBar,
getRemoveFileProps,
Remove,
}: any) => (
<>
{acceptedFile ? (
<>
{formatFileSize(acceptedFile.size)}
{acceptedFile.name}
{
event.preventDefault();
setRemoveHoverColor(REMOVE_HOVER_COLOR_LIGHT);
}}
onMouseOut={(event: Event) => {
event.preventDefault();
setRemoveHoverColor(DEFAULT_REMOVE_HOVER_COLOR);
}}
>
>
) : (
'Click to upload'
)}
>
)}
);
}
```### 🎀 CSVDownloader
Just pass in the js object with an optional [configuration](https://react-papaparse.js.org/docs#config) ( setting delimiter / separator ).
**Note:** If you want to open your CSV files in Excel, you might want to set `bom={true}` or `bom`, default is `false`. This option adds the so called BOM byte `'\ufeff'` to the beginning of your CSV files and tells Excel that the encoding is UTF8.
#### Button
```javascript
import React from 'react';import { useCSVDownloader } from 'react-papaparse';
export default function CSVDownloader() {
const { CSVDownloader, Type } = useCSVDownloader();return (
Download
);
}
```#### Link
```javascript
import React from 'react';import { useCSVDownloader } from 'react-papaparse';
export default function CSVDownloader() {
const { CSVDownloader, Type } = useCSVDownloader();return (
Download
);
}
```#### Data as a Function/Callback
`data={}` can be a synchronous or asynchronous function that returns a data object.
```javascript
import React from 'react';import { useCSVDownloader } from 'react-papaparse';
export default function CSVDownloader() {
const { CSVDownloader } = useCSVDownloader();return (
{
return [
{
"Column 1": "1-1",
"Column 2": "1-2",
"Column 3": "1-3",
"Column 4": "1-4",
}
]}
}
>
Download
);
}
```### 🎀 readString
```javascript
import React from 'react';import { usePapaParse } from 'react-papaparse';
export default function ReadString() {
const { readString } = usePapaParse();const handleReadString = () => {
const csvString = `Column 1,Column 2,Column 3,Column 4
1-1,1-2,1-3,1-4
2-1,2-2,2-3,2-4
3-1,3-2,3-3,3-4
4,5,6,7`;readString(csvString, {
worker: true,
complete: (results) => {
console.log('---------------------------');
console.log(results);
console.log('---------------------------');
},
});
};return handleReadString()}>readString;
}
```### 🎀 readRemoteFile
```javascript
import React from 'react';import { usePapaParse } from 'react-papaparse';
export default function ReadRemoteFile() {
const { readRemoteFile } = usePapaParse();const handleReadRemoteFile = () => {
readRemoteFile(url, {
complete: (results) => {
console.log('---------------------------');
console.log('Results:', results);
console.log('---------------------------');
},
});
};return handleReadRemoteFile()}>readRemoteFile;
}
```### 🎀 jsonToCSV
```javascript
import React from 'react';import { usePapaParse } from 'react-papaparse';
export default function JsonToCSV() {
const { jsonToCSV } = usePapaParse();const handleJsonToCSV = () => {
const jsonData = [
{
"Column 1": "1-1",
"Column 2": "1-2",
"Column 3": "1-3",
"Column 4": "1-4"
},
{
"Column 1": "2-1",
"Column 2": "2-2",
"Column 3": "2-3",
"Column 4": "2-4"
},
{
"Column 1": "3-1",
"Column 2": "3-2",
"Column 3": "3-3",
"Column 4": "3-4"
},
{
"Column 1": 4,
"Column 2": 5,
"Column 3": 6,
"Column 4": 7
}
];
const results = jsonToCSV(jsonData);
console.log('---------------------------');
console.log('Results:', results);
console.log('---------------------------');
};return handleJsonToCSV()}>jsonToCSV;
}
```#### Header Row Support
If you tell react-papaparse there is a header row, each row will be organized by field name instead of index.
```javascript
import { usePapaParse } from 'react-papaparse';const { readString } = usePapaParse();
readString(csvString, {
header: true,
worker: true,
complete: (results) => {
console.log('---------------------------');
console.log(results);
console.log('---------------------------');
},
});
```#### Stream
That's what streaming is for. Specify a step callback to receive the results row-by-row. This way, you won't load the whole file into memory and crash the browser.
```javascript
import { usePapaParse } from 'react-papaparse';const { readRemoteFile } = usePapaParse();
readRemoteFile(url, {
step: (row) => {
console.log('Row:', row.data);
},
complete: () => {
console.log('All done!');
}
});
```## 📜 Changelog
Latest version 4.4.0 (2023-10-14):
* Handle parsing utf-8 bom encoded files
* Rename duplicate headers
* Improve iso-date regexVersion 4.3.0 (2023-10-10):
* Enable async callback function for CSVDownloader
Version 4.2.2 (2023-10-09):
* Fix type
Version 4.2.0 (2023-10-07):
* Upgrade dependencies
Version 4.1.0 (2022-08-07):
* Import readString, readRemoteFile and jsonToCSV as pure function
Version 4.0.4 (2022-08-06):
* Add optional required prop for input file
Version 4.0.2 (2022-01-26):
* Fix onUploadAccepted signature when a preview is set
Version 4.0.1 (2022-01-21):
* Fix config props does not work in CSVReader
Version 4.0.0 (2022-01-18):
* Improve code performance
* Rewrite any existing based components to hooksDetails changes for each release are documented in the [CHANGELOG.md](https://github.com/Bunlong/react-papaparse/blob/master/CHANGELOG.md).
## 🛣️ Roadmap
### 🆕 v4.4.x
* CSVReader multiple files drag and drop
## ❗ Issues
If you think any of the `react-papaparse` can be improved, please do open a PR with any updates and submit any issues. Also, I will continue to improve this, so you might want to watch/star this repository to revisit.
## 💪 Contribution
We'd love to have your helping hand on contributions to `react-papaparse` by forking and sending a pull request!
Your contributions are heartily ♡ welcome, recognized and appreciated. (✿◠‿◠)
How to contribute:
- Open pull request with improvements
- Discuss ideas in issues
- Spread the word
- Reach out with any feedback## 🏆 Contributors
![]()
Bunlong
![]()
Tim Tutt
![]()
Pieter Kuppens
![]()
Jack Zhao
![]()
Pablo Menichini
![]()
Mystical Tech
![]()
Bruno
![]()
Samuel Hulla
![]()
glinkot
![]()
Paul Leavitt
![]()
Gabriel
![]()
Izaak
![]()
Oliver
![]()
Ole Skaar
![]()
Des
![]()
Karl
![]()
Max
![]()
Kostas
![]()
Dalitzky
![]()
John Quinlivan
![]()
Gareth Jones
![]()
Chrys Exaucet
![]()
Stefee
![]()
Christopher Thomas
![]()
Venelin Banov
![]()
Joey Baker
![]()
Michiel De Mey
![]()
Mohammed Hussam
![]()
Xiaochao Liu
![]()
Jake Haitsma
## 👨👩👦 Advertisement
You maybe interested.
* [React Patterns](https://github.com/reactpatterns/reactpatterns) – React patterns & techniques to use in development for React Developer.
* [Next Share](https://github.com/Bunlong/next-share) – Social media share buttons for your next React apps.
* [Next QRCode](https://github.com/bunlong/next-qrcode) – React hooks for generating QR code for your next React apps.
* [Next Time Ago](https://github.com/Bunlong/next-time-ago) – A lightweight tiny time-ago component for your next React apps.## ⚖️ License
The MIT License [](https://opensource.org/licenses/MIT)