https://github.com/caiyunapp/leibniz
Leibniz is a python package which provide facilities to express learnable partial differential equations with PyTorch
https://github.com/caiyunapp/leibniz
adjoint-method bottleneck cnn differential-equations hyperbolic-block learnable pde physical-informed python pytorch pytorch-cnn pytorch-implementation resnet resunet senet unet unet-3d unet-pytorch
Last synced: 15 days ago
JSON representation
Leibniz is a python package which provide facilities to express learnable partial differential equations with PyTorch
- Host: GitHub
- URL: https://github.com/caiyunapp/leibniz
- Owner: caiyunapp
- License: mit
- Created: 2019-09-17T02:22:52.000Z (over 5 years ago)
- Default Branch: master
- Last Pushed: 2021-09-13T15:55:04.000Z (over 3 years ago)
- Last Synced: 2025-04-16T02:02:50.865Z (about 1 month ago)
- Topics: adjoint-method, bottleneck, cnn, differential-equations, hyperbolic-block, learnable, pde, physical-informed, python, pytorch, pytorch-cnn, pytorch-implementation, resnet, resunet, senet, unet, unet-3d, unet-pytorch
- Language: Python
- Homepage:
- Size: 1.46 MB
- Stars: 15
- Watchers: 10
- Forks: 7
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# Leibniz
[](https://zenodo.org/badge/latestdoi/208940378)
[](http://travis-ci.com/caiyunapp/leibniz)Leibniz is a python package which provide facilities to express learnable differential equations with PyTorch
We also provide UNet, ResUNet and their variations, especially the Hyperbolic blocks for ResUNet.
Install
--------```bash
pip install leibniz
```How to use
-----------### Physics-informed
As an example we solve a very simple advection problem, a box-shaped material transported by a constant steady wind.
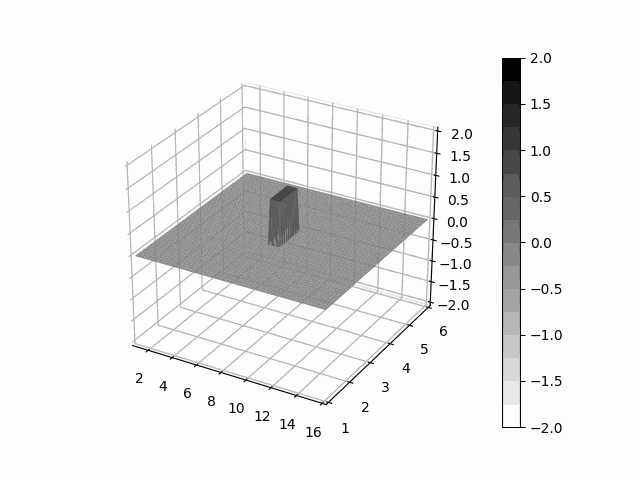
```python
import torch as th
import leibniz as lbnzfrom leibniz.core3d.gridsys.regular3 import RegularGrid
from leibniz.diffeq import odeint as odeintdef binary(tensor):
return th.where(tensor > lbnz.zero, lbnz.one, lbnz.zero)# setup grid system
lbnz.bind(RegularGrid(
basis='x,y,z',
W=51, L=151, H=51,
east=16.0, west=1.0,
north=6.0, south=1.0,
upper=6.0, lower=1.0
))
lbnz.use('x,y,z') # use xyz coordinate# giving a material field as a box
fld = binary((lbnz.x - 8) * (9 - lbnz.x)) * \
binary((lbnz.y - 3) * (4 - lbnz.y)) * \
binary((lbnz.z - 3) * (4 - lbnz.z))# construct a constant steady wind
wind = lbnz.one, lbnz.zero, lbnz.zero# transport value by wind
def derivitive(t, clouds):
return - lbnz.upwind(wind, clouds)# integrate the system with rk4
pred = odeint(derivitive, fld, th.arange(0, 7, 1 / 100), method='rk4')
```### UNet, ResUNet and variations
```python
from leibniz.unet import UNet
from leibniz.nn.layer.hyperbolic import HyperBottleneck
from leibniz.nn.activation import CappingReluunet = UNet(6, 1, normalizor='batch', spatial=(32, 64), layers=5, ratio=-1,
vblks=[4, 4, 4, 4, 4], hblks=[1, 1, 1, 1, 1],
scales=[-1, -1, -1, -1, -1], factors=[1, 1, 1, 1, 1],
block=HyperBottleneck, relu=CappingRelu(), final_normalized=False)
```We provide a ResUNet implementation, which is a UNet variation can insert ResNet blocks between layers.
The supported ResNet blocks are include
* Pure ResNet: Basic, Bottleneck block
* SENet variations: Basic, Bottleneck block
* Hyperbolic variations: Basic, Bottleneck blockWe support 1d, 2d, 3d UNet.
normalizor are include:
* batch: BatchNorm
* layer: LayerNorm
* instance: InstanceNormOther hyperparameters are include:
* spatial: the sizes of the spatial dimentions
* ratio: the ratio to decide the intial number of channels into the UNet
* vblks: how many vertical blocks is inserted between two layers
* hblks: how many horizontal blocks is inserted in the skip connections
* scales: scale factors(power-2-based) on the spatial dimentions
* factors: expand or shrink factors(power-2-based) on the channels
* final_normalized: wheather to scale to final result between 0 to 1### Piecewise Linear normalizor
Piecewise Linear normalizor provide an learnable monotonic peicewise linear functions and its inverse fucntion.
The API is shown as below```python
from leibniz.nn.normalizor import PWLNormalizor
# on 3 channels, given 128 segmented pieces, and assuming the input data have a zero mean and 1.0 std
pwln = PWLNormalizor(3, 128, mean=0.0, std=1.0)normed = pwln(input)
output = pwln.inverse(normed)
```How to release
---------------```bash
python3 setup.py sdist bdist_wheel
python3 -m twine upload dist/*git tag va.b.c master
git push origin va.b.c
```Contributors
------------* Mingli Yuan ([Mountain](https://github.com/mountain))
* Xiang Pan ([Panpanx](https://github.com/Panpanx))
* Yi Liu ([YiLiu](https://github.com/YiLiu-Lly))Acknowledge
-----------We included source code with minor changes from [torchdiffeq](https://github.com/rtqichen/torchdiffeq) by Ricky Chen,
because of two purpose:
1. package torchdiffeq is not indexed by pypi
2. package torchdiffeq is very convenient and mandatoryAll our contribution is based on Ricky's Neural ODE paper (NIPS 2018) and his package.