Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/capacitor-community/native-audio
https://github.com/capacitor-community/native-audio
Last synced: 5 days ago
JSON representation
- Host: GitHub
- URL: https://github.com/capacitor-community/native-audio
- Owner: capacitor-community
- License: mit
- Created: 2020-05-28T19:26:54.000Z (over 4 years ago)
- Default Branch: master
- Last Pushed: 2024-07-17T22:07:53.000Z (5 months ago)
- Last Synced: 2024-09-29T05:29:50.533Z (2 months ago)
- Language: Java
- Size: 5.79 MB
- Stars: 108
- Watchers: 12
- Forks: 66
- Open Issues: 29
-
Metadata Files:
- Readme: README.md
- Contributing: .github/CONTRIBUTING.md
- License: LICENSE
- Code of conduct: .github/CODE_OF_CONDUCT.md
- Codeowners: .github/CODEOWNERS.txt
Awesome Lists containing this project
- awesome-capacitorjs - @capacitor-community/native-audio - Capacitor community plugin for playing sounds. (Plugins / Community Plugins)
- awesome-capacitor - Native audio - A native plugin for native audio engine. (Community plugins)
README
Native Audio
@capacitor-community/native-audio
Capacitor community plugin for playing sounds natively.# Capacitor Native Audio Plugin
Capacitor plugin for native audio engine.
Capacitor v6 - β Support!Click on video to see example π₯
[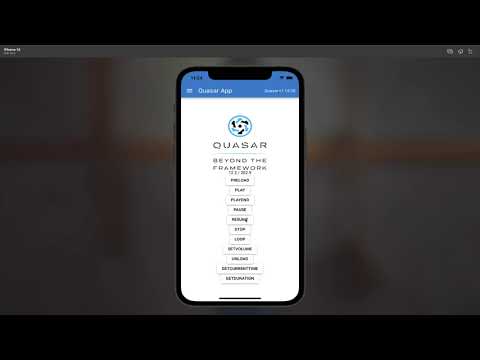](https://www.youtube.com/watch?v=XpUGlWWtwHs)
## Maintainers
| Maintainer | GitHub | Social |
| ------------- | ------------------------------------------- | ----------------------------------- |
| Maxim Bazuev | [bazuka5801](https://github.com/bazuka5801) | [Telegram](https://t.me/bazuka5801) |## Preparation
All audio place in specific platform folderAndoid: `android/app/src/assets`
iOS: `ios/App/App/sounds`
Web: `assets/sounds`
## Installation
To use npm
```bash
npm install @capacitor-community/native-audio
```To use yarn
```bash
yarn add @capacitor-community/native-audio
```Sync native files
```bash
npx cap sync
```On iOS, Android and Web, no further steps are needed.
## Configuration
No configuration required for this plugin.
## Supported methods
| Name | Android | iOS | Web |
| :------------- | :------ | :-- | :-- |
| configure | β | β | β |
| preload | β | β | β |
| play | β | β | β |
| pause | β | β | β |
| resume | β | β | β |
| loop | β | β | β |
| stop | β | β | β |
| unload | β | β | β |
| setVolume | β | β | β |
| getDuration | β | β | β |
| getCurrentTime | β | β | β |
| isPlaying | β | β | β |## Usage
[Example repository](https://github.com/bazuka5801/native-audio-example)
```typescript
import {NativeAudio} from '@capacitor-community/native-audio'/**
* This method will load more optimized audio files for background into memory.
* @param assetPath - relative path of the file or absolute url (file://)
* assetId - unique identifier of the file
* audioChannelNum - number of audio channels
* isUrl - pass true if assetPath is a `file://` url
* @returns void
*/
NativeAudio.preload({
assetId: "fire",
assetPath: "fire.mp3",
audioChannelNum: 1,
isUrl: false
});/**
* This method will play the loaded audio file if present in the memory.
* @param assetId - identifier of the asset
* @param time - (optional) play with seek. example: 6.0 - start playing track from 6 sec
* @returns void
*/
NativeAudio.play({
assetId: 'fire',
// time: 6.0 - seek time
});/**
* This method will loop the audio file for playback.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.loop({
assetId: 'fire',
});/**
* This method will stop the audio file if it's currently playing.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.stop({
assetId: 'fire',
});/**
* This method will unload the audio file from the memory.
* @param assetId - identifier of the asset
* @returns void
*/
NativeAudio.unload({
assetId: 'fire',
});/**
* This method will set the new volume for a audio file.
* @param assetId - identifier of the asset
* volume - numerical value of the volume between 0.1 - 1.0
* @returns void
*/
NativeAudio.setVolume({
assetId: 'fire',
volume: 0.4,
});/**
* this method will getΒ the duration of an audio file.
* only works if channels == 1
*/
NativeAudio.getDuration({
assetId: 'fire'
})
.then(result => {
console.log(result.duration);
})/**
* this method will get the current time of a playing audio file.
* only works if channels == 1
*/
NativeAudio.getCurrentTime({
assetId: 'fire'
});
.then(result => {
console.log(result.currentTime);
})/**
* This method will return false if audio is paused or not loaded.
* @param assetId - identifier of the asset
* @returns {isPlaying: boolean}
*/
NativeAudio.isPlaying({
assetId: 'fire'
})
.then(result => {
console.log(result.isPlaying);
})
```## API
### configure(...)
```typescript
configure(options: ConfigureOptions) => Promise
```| Param | Type |
| ------------- | ------------------------------------------------------------- |
| **`options`** |ConfigureOptions
|--------------------
### preload(...)
```typescript
preload(options: PreloadOptions) => Promise
```| Param | Type |
| ------------- | --------------------------------------------------------- |
| **`options`** |PreloadOptions
|--------------------
### play(...)
```typescript
play(options: { assetId: string; time?: number; }) => Promise
```| Param | Type |
| ------------- | ------------------------------------------------ |
| **`options`** |{ assetId: string; time?: number; }
|--------------------
### pause(...)
```typescript
pause(options: { assetId: string; }) => Promise
```| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|--------------------
### resume(...)
```typescript
resume(options: { assetId: string; }) => Promise
```| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|--------------------
### loop(...)
```typescript
loop(options: { assetId: string; }) => Promise
```| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|--------------------
### stop(...)
```typescript
stop(options: { assetId: string; }) => Promise
```| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|--------------------
### unload(...)
```typescript
unload(options: { assetId: string; }) => Promise
```| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|--------------------
### setVolume(...)
```typescript
setVolume(options: { assetId: string; volume: number; }) => Promise
```| Param | Type |
| ------------- | ------------------------------------------------- |
| **`options`** |{ assetId: string; volume: number; }
|--------------------
### getCurrentTime(...)
```typescript
getCurrentTime(options: { assetId: string; }) => Promise<{ currentTime: number; }>
```| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|**Returns:**
Promise<{ currentTime: number; }>
--------------------
### getDuration(...)
```typescript
getDuration(options: { assetId: string; }) => Promise<{ duration: number; }>
```| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|**Returns:**
Promise<{ duration: number; }>
--------------------
### isPlaying(...)
```typescript
isPlaying(options: { assetId: string; }) => Promise<{ isPlaying: boolean; }>
```| Param | Type |
| ------------- | --------------------------------- |
| **`options`** |{ assetId: string; }
|**Returns:**
Promise<{ isPlaying: boolean; }>
--------------------
### addListener('complete', ...)
```typescript
addListener(eventName: 'complete', listenerFunc: (event: { assetId: string; }) => void) => Promise
```Listen for asset completed playing event
| Param | Type |
| ------------------ | ----------------------------------------------------- |
| **`eventName`** |'complete'
|
| **`listenerFunc`** |(event: { assetId: string; }) => void
|**Returns:**
Promise<PluginListenerHandle>
**Since:** 5.0.1
--------------------
### Interfaces
#### ConfigureOptions
| Prop | Type | Description | Default |
| ----------- | -------------------- | ------------------------------------------------- | ------------------ |
| **`fade`** |boolean
| Indicating whether or not to fade audio. |false
|
| **`focus`** |boolean
| Indicating whether or not to disable mixed audio. |false
|#### PreloadOptions
| Prop | Type |
| --------------------- | -------------------- |
| **`assetPath`** |string
|
| **`assetId`** |string
|
| **`volume`** |number
|
| **`audioChannelNum`** |number
|
| **`isUrl`** |boolean
|#### PluginListenerHandle
| Prop | Type |
| ------------ | ----------------------------------------- |
| **`remove`** |() => Promise<void>
|