https://github.com/charkour/react-reactions
😲 Create custom reaction pickers and counters or use your favorites!
https://github.com/charkour/react-reactions
custom emoji facebook github pokemon react react-reactions reactions slack youtube
Last synced: 2 months ago
JSON representation
😲 Create custom reaction pickers and counters or use your favorites!
- Host: GitHub
- URL: https://github.com/charkour/react-reactions
- Owner: charkour
- License: mit
- Created: 2021-01-22T19:14:20.000Z (over 4 years ago)
- Default Branch: main
- Last Pushed: 2023-10-18T05:46:42.000Z (over 1 year ago)
- Last Synced: 2025-04-02T22:08:14.707Z (3 months ago)
- Topics: custom, emoji, facebook, github, pokemon, react, react-reactions, reactions, slack, youtube
- Language: TypeScript
- Homepage:
- Size: 3.85 MB
- Stars: 75
- Watchers: 1
- Forks: 17
- Open Issues: 21
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
README
# 
Create your own reaction bar or use one of your favorites!
- **4 Different Selectors** - Slack, Facebook, Pokemon and GitHub
- **5 Different Counters** - GitHub, YouTube, Facebook, Pokemon, and Slack[](https://www.npmjs.com/package/@charkour/react-reactions)
Install [via npm](https://www.npmjs.com/package/@charkour/react-reactions) (or from the [GitHub Package Registry](https://github.com/charkour/react-reactions/packages/623096)):
```sh
npm i @charkour/react-reactions
```> This originated as a fork of [casesandberg/react-reactions](https://github.com/casesandberg/react-reactions) which is been modified under the MIT license to include additional features.
## New Features
- [x] Add ability to pass **custom icons**
- [x] Fixed security vulnerabilities
- [x] CJS and ESM support
- [x] Add [ref forwarding](https://reactjs.org/docs/forwarding-refs.html) support
- [x] Zero dependencies
- [x] Built in Typescript and modern React (with [TSDX](https://github.com/formium/tsdx))
- [x] Works with React 16.8+ and Next 10## Road Map
- [ ] Update current Selectors and Counter to match 2021 styles
- [ ] Add Discord Selector and Counter
- [ ] Add unit testing
- [ ] More??? Suggest a feature on [Github Issues](https://github.com/charkour/react-reactions/issues)## Custom Selectors
### Reaction Bar Selector
```tsx
import React from 'react';
import { ReactionBarSelector } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`iconSize?: number` — String icon pixel size. Defaults to `38px`
`reactions?: Reaction[];` — Array of Reaction objects `{label: "haha", node:
😄, key: "smile"}` to display.`onSelect: (key: string) => void;` — Function callback when emoji is selected
`style?: React.CSSProperties` - Pass a style object to the selector container
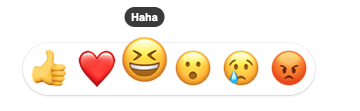
Also works with images.
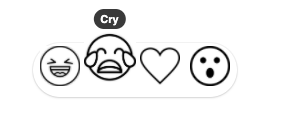
_Note_: When passing an `
` as a Reaction. Specify the `iconSize` as the height of the image. `
`
### Reaction Counter
```tsx
import React from 'react';
import { ReactionCounter } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:***
`iconSize?: number` - String icon pixel size. Defaults to `24px`
`bg?: string` - String of hex color for outline of overlapping reactions. Defaults to `#fff`
`reactions: ReactionCounterObject[]` - Array of emoji to dispay
`user?: string` - String name of user so that user displays as `You`
`important?: string[]` - Array of strings for important users to display their name
`showReactsOnly?: boolean` - If `true`, only show the Reactions and no text. Defaults to `false`
`showTotalOnly?: boolean` - If `true`, only show the number of Reactions and no specific names. Defaults to `false`
`showOthersAlways?: boolean` - Will display "and 0 others" if you are the only person who reacted. Defaults to `true`
`className?: string` - Pass a string that applies to the counter container
`onClick?: () => void` - Pass a callback that is added to the `onClick` property to the counter container
`style?: React.CSSProperties` - Pass a style object to the counter container
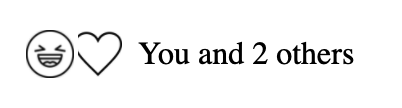
## Selectors
### Slack Selector
```tsx
import React from 'react';
import { SlackSelector } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`active`: String of active tab. Defaults to `mine`
`scrollHeight`: String pixel height of scroll container. Defaults to `270px`
`removeEmojis`: Array of emojis to remove from emoji list
`frequent`: Array of emojis to set Frequently Used. Defaults to `['👍', '🐉', '🙌', '🗿', '😊', '🐬', '😹', '👻', '🚀', '🚁', '🏇', '🇨🇦']`
`onSelect`: Function callback when emoji is selected
**Fonts**: To use the Slack fonts, download the font files [here](https://github.com/charkour/react-reactions/tree/main/example/public/fonts) and include them in `public/fonts`.
---
### Github Selector
```tsx
import React from 'react';
import { GithubSelector } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`reactions`: Array of emoji to dispay. Defaults to `['👍', '👎', '😄', '🎉', '😕', '❤️']`
`onSelect`: Function callback when emoji is selected
---
### Facebook Selector
```tsx
import React from 'react';
import { FacebookSelector } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`reactions`: Array of strings for reactions to display. Defaults to `['like', 'love', 'haha', 'wow', 'sad', 'angry']`
`iconSize`: String icon pixel size. Defaults to `38px`
`onSelect`: Function callback when emoji is selected
---
### Pokemon Selector
```tsx
import React from 'react';
import { PokemonSelector } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`reactions`: Array of strings for reactions to display. Defaults to `['like', 'love', 'haha', 'wow', 'sad', 'angry']`
`iconSize`: String icon pixel size. Defaults to `38px`
`onSelect`: Function callback when emoji is selected
---
## Counters
### Github Counter
```tsx
import React from 'react';
import { GithubCounter } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`counters`: Array of counter objects structured such that:
```tsx
{
emoji: '👍', // String emoji reaction
by: 'case', // String of persons name
}
````user`: String name of user so that user displays as `You`
`onSelect`: Function callback when emoji is selected
`onAdd`: Function callback when add reaction is clicked
---
### Youtube Counter
```tsx
import React from 'react';
import { YoutubeCounter } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`like`: String number of likes
`dislike`: String number of dislikes
`onLikeClick`: Function callback when like is clicked
`onDislikeClick`: Function callback when dislike is clicked
---
### Facebook Counter
```tsx
import React from 'react';
import { FacebookCounter } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`counters`: Array of counter objects structured such that:
```tsx
{
emoji: 'like', // String name of reaction
by: 'Case Sandberg', // String of persons name
}
````user`: String name of user so that user displays as `You`
`important`: Array of strings for important users to display their name
`bg`: String of hex color for outline of overlapping reactions. Defaults to `#fff`
`onClick`: Function callback when clicked
`alwaysShowOthers`: boolean. Will display "and 0 others" if you are the only person who reacted.
---
### Pokemon Counter
```tsx
import React from 'react';
import { PokemonCounter } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`counters`: Array of counter objects structured such that:
```tsx
{
emoji: 'like', // String name of reaction
by: 'Charles Kornoelje', // String of persons name
}
````user`: String name of user so that user displays as `You`
`important`: Array of strings for important users to display their name
`bg`: String of hex color for outline of overlapping reactions. Defaults to `#fff`
`onClick`: Function callback when clicked
`alwaysShowOthers`: boolean. Will display "and 0 others" if you are the only person who reacted.
---
### Slack Counter
```tsx
import React from 'react';
import { SlackCounter } from '@charkour/react-reactions';const Component = () => {
return ;
};
```**Props:**
`counters`: Array of counter objects structured such that:
```tsx
{
emoji: '🗿', // String emoji reaction
by: 'case', // String of persons name
}
````user`: String name of user so that user displays as `You`
`onSelect`: Function callback when emoji is selected
`onAdd`: Function callback when add reaction is clicked
**Fonts**: To use the Slack fonts, download the font files [here](https://github.com/charkour/react-reactions/tree/main/example/public/fonts) and include them in `public/fonts`.
---
## Animations
A simple animation can be done on the components using CSS. See this [demo](https://codesandbox.io/s/sweet-burnell-oh5vg?file=/src/App.js).
More advaned animations can be done using dynamic styles. See this [demo](https://codesandbox.io/s/competent-curran-cn4tv?file=/src/App.js)## Development
```bash
npm start
```This builds to `/dist` and runs the project in watch mode so any edits you save inside `src` causes a rebuild to `/dist`.
Then run either Storybook or the example playground:
### Storybook
Run inside another terminal:
```bash
npm run storybook
```This loads the stories from `./stories`.
> NOTE: Stories should reference the components as if using the library, similar to the example playground. This means importing from the root project directory. This has been aliased in the tsconfig and the storybook webpack config as a helper.
### Example
Then run the example inside another:
```bash
cd example
npm i
npm start
```The default example imports and live reloads whatever is in `/dist`, so if you are seeing an out of date component, make sure TSDX is running in watch mode like we recommend above. **No symlinking required**, we use [Parcel's aliasing](https://parceljs.org/module_resolution.html#aliases).
To do a one-off build, use `npm run build`.
To run tests, use `npm test`.
## Configuration
Code quality is set up for you with `prettier`, `husky`, and `lint-staged`. Adjust the respective fields in `package.json` accordingly.
### Jest
Jest tests are set up to run with `npm test`.
### GitHub Actions
- `main` which installs deps w/ cache, lints, tests, and builds on all pushes against a Node and OS matrix
---
> Pokemon Illustrations by [Chris Owens](https://dribbble.com/monkee1895)