Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/charlie0077/graphql-server-crud
Build GraphQL CRUD api server without boilerplate code
https://github.com/charlie0077/graphql-server-crud
Last synced: 3 months ago
JSON representation
Build GraphQL CRUD api server without boilerplate code
- Host: GitHub
- URL: https://github.com/charlie0077/graphql-server-crud
- Owner: charlie0077
- License: mit
- Created: 2020-03-26T05:19:37.000Z (almost 5 years ago)
- Default Branch: master
- Last Pushed: 2023-01-11T02:29:31.000Z (almost 2 years ago)
- Last Synced: 2024-09-28T21:43:11.822Z (3 months ago)
- Language: JavaScript
- Homepage: https://nostalgic-perlman-fe9f48.netlify.com/guide/
- Size: 4.25 MB
- Stars: 68
- Watchers: 2
- Forks: 4
- Open Issues: 19
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
[](https://www.npmjs.com/package/graphql-server-crud)
[](https://circleci.com/gh/charlie0077/graphql-server-crud)
[](https://codecov.io/gh/charlie0077/graphql-server-crud)Please go to [https://nostalgic-perlman-fe9f48.netlify.com](https://nostalgic-perlman-fe9f48.netlify.com/guide/) for the complete documentation.
# Introduction
This is a lightweight javascript framework/library to help you build a GraphQL server efficiently. It aims to reduce your code size, save you time and gives you the flexibilities you need.## Philosophy
* Reduce the CRUD related code you need to write.
* It is code, not black box service, you have full control about logic, deployment, etc.
* You don't need to learn new random definitions: syntax, directive, magic, etc.
* It coexists with your existing project.
* Support multiple databases: it should support what [knex](http://knexjs.org/) supports.
* It is not discouraged to build your own complicate queries to support complicate use case.# Getting Started
The following is to give you a quick idea of how to get started. You may want to refer to a full example [here](https://nostalgic-perlman-fe9f48.netlify.app/example/), which contains test data.
## Install package
``` sh
npm install graphql-server-crud
```
## Define the "model"
```js
const { ModelBase } = require('graphql-server-crud')
const { knex } = require('../db') // this is your typical knex dbclass Company extends ModelBase {
knex = knex
table = 'companies'
fields = {
id: 'Int',
domain: 'String',
public: 'Boolean',
phone: 'String',
sales: 'Float',
customers: 'Int'
}
}
```## Add auto-generated typeDefs and resolvers to your root
Add these followinng lines to your root typeDefs and resolvers variables.``` js
// Add default typeDefs and resolvers
// You only need to do this once regardless of the number of models you have
const { addTypeDefs, addResolvers } = require('graphql-server-crud')
const models = require('./models')
addTypeDefs(typeDefs, models)
addResolvers(resolvers, models)
```You can check a full example file [here](https://github.com/charlie0077/graphql-server-crud/blob/master/example/server.js).
## That is it!
You have a basic CRUD endpoint for **Company** now.Run a simple query:
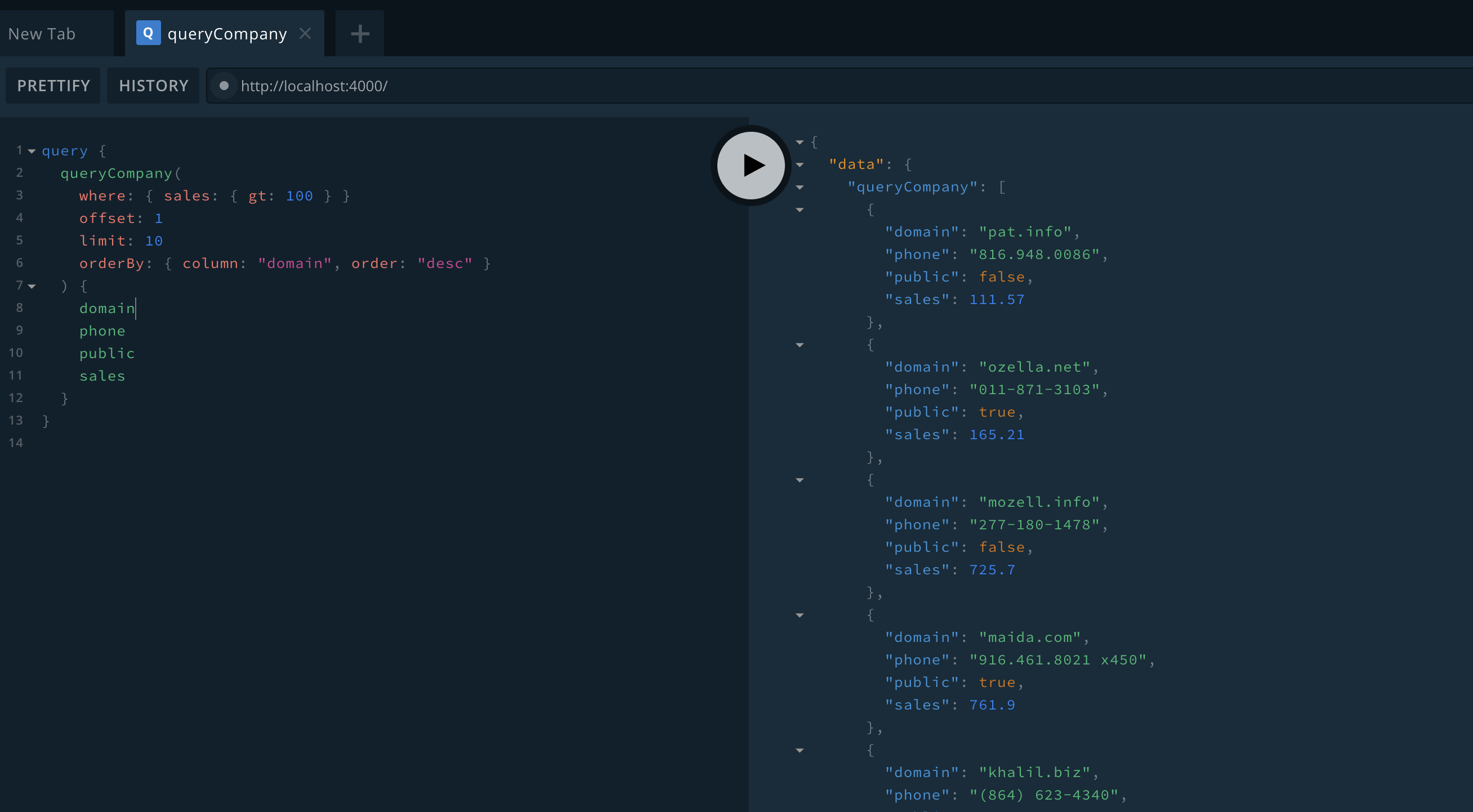
The schema:
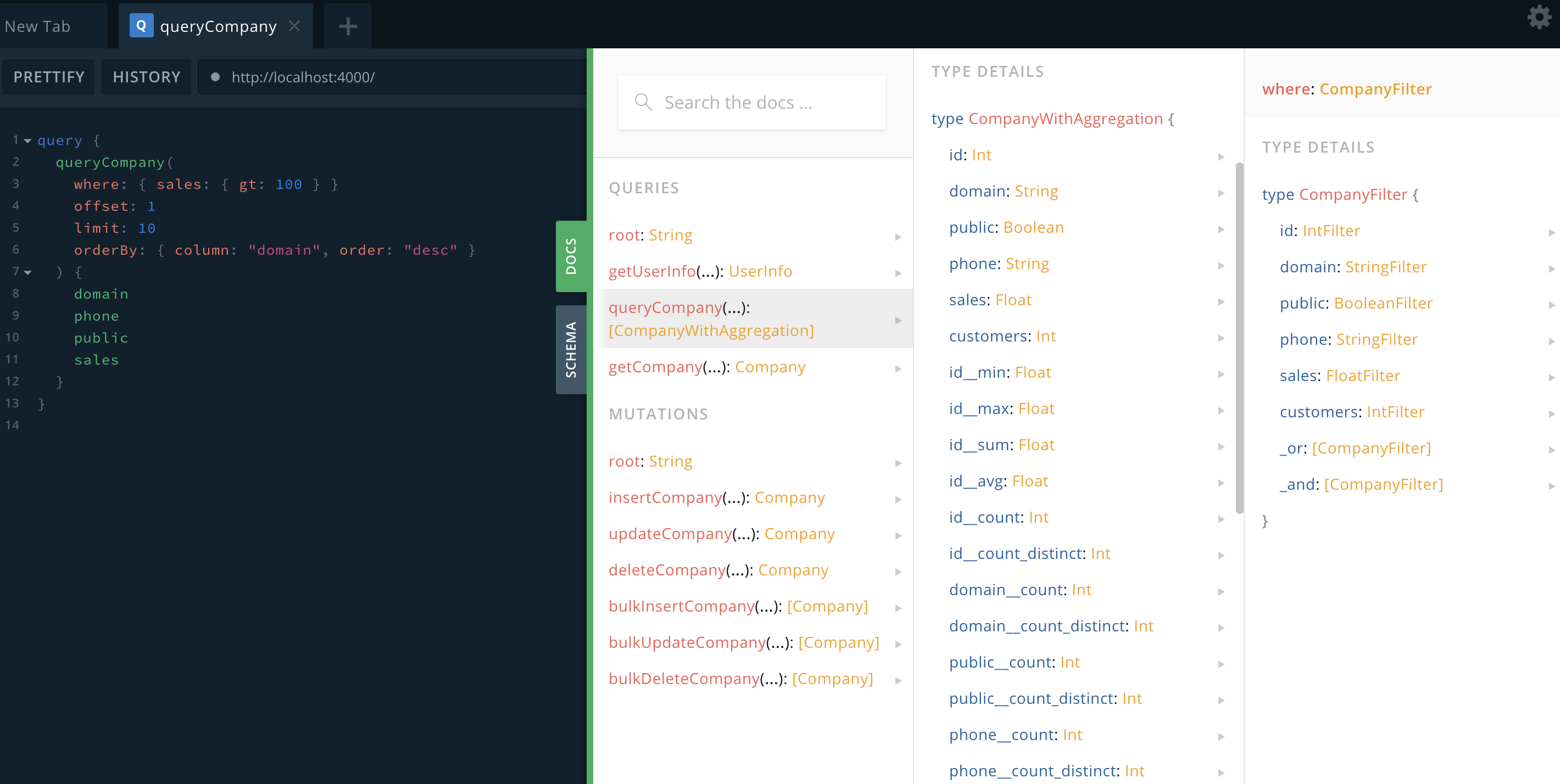
## A more complicate query example
```graphql
query {
# queryAuthorJoinPost is a derived table(runtime view)
queryAuthorJoinPost(
# complicate filter condition
where: {
_and: [
{ _or: [{ id: { gt: 10 }, email: { gt: "k" } }, { id: { lt: 5 } }] }
{ first_name: { gt: "OK" } }
]
}
# top level orderBy
orderBy: [{ column: "score__max", order: "desc" }]
limit: 10
offset: 0
# support aggregation
groupBy: ["id", "email"]
having: {
age__avg: { gt: 30 }
id: { nin: [1, 2, 6] }
email: { null: false }
}
) {
id
# aggregations
age__avg
age__count
age__count_distinct
score__max
# nested field in group by
review(
on: { star: { gt: 2 } }
limit: 5
offset: 1
orderBy: [{ column: "star", order: "desc" }]
) {
id
star
}
}
}
```## How it works
You define a **model**. The library generates common GraphQL schema, resolvers logic for you. To support complicate search queries(filter, groupBy, join, nested fields, etc), it also has a built-in **compiler** to compile the filter input to [knex](http://knexjs.org/) code. A **ModelBase** class is provided to you so that you can build your custom logic on top of it. You can also use the **model** as your database client in any place of your logic.## Features
* logic generation for schema
* logic generation for common resolvers
* support queries: get, list(search by filters)
* support mutation: insert, delete, update, bulk insert, bulk delete, bulk update
* support common where filters: =, <>, >, <, between, in, or, and, etc.
* support common aggregations: groupBy, having, sum, avg, min, max, count, distinct, etc.
* support common components in a query: offset, limit, order by, etc.
* support nested objects queries
* no N+1 problem for common queries
* coexists with your current code, custom schema, custom resolver.
* it does not care if you are using graphql-server, graphql-lambda, or graphql-express, etc.Please go to [https://nostalgic-perlman-fe9f48.netlify.com](https://nostalgic-perlman-fe9f48.netlify.com/guide/) for the complete documentation.
# Contributing
You are always welcome to contribute to the project.Thanks for all your wonderful PRs, issues and ideas.
[](https://sourcerer.io/fame/charlie0077/charlie0077/graphql-server-crud/links/0)[](https://sourcerer.io/fame/charlie0077/charlie0077/graphql-server-crud/links/1)[](https://sourcerer.io/fame/charlie0077/charlie0077/graphql-server-crud/links/2)[](https://sourcerer.io/fame/charlie0077/charlie0077/graphql-server-crud/links/3)[](https://sourcerer.io/fame/charlie0077/charlie0077/graphql-server-crud/links/4)[](https://sourcerer.io/fame/charlie0077/charlie0077/graphql-server-crud/links/5)[](https://sourcerer.io/fame/charlie0077/charlie0077/graphql-server-crud/links/6)[](https://sourcerer.io/fame/charlie0077/charlie0077/graphql-server-crud/links/7)