https://github.com/chenyahui/gin-cache
:rocket: A high performance gin middleware to cache http response. Compared to gin-contrib/cache, It has a huge performance improvement. 高性能gin缓存中间件,相比于官方版本,有巨大性能提升。
https://github.com/chenyahui/gin-cache
cache gin golang middleware redis singleflight
Last synced: 3 months ago
JSON representation
:rocket: A high performance gin middleware to cache http response. Compared to gin-contrib/cache, It has a huge performance improvement. 高性能gin缓存中间件,相比于官方版本,有巨大性能提升。
- Host: GitHub
- URL: https://github.com/chenyahui/gin-cache
- Owner: chenyahui
- License: mit
- Created: 2021-06-14T13:51:42.000Z (about 4 years ago)
- Default Branch: main
- Last Pushed: 2024-05-28T18:02:27.000Z (about 1 year ago)
- Last Synced: 2025-03-28T06:05:06.321Z (3 months ago)
- Topics: cache, gin, golang, middleware, redis, singleflight
- Language: Go
- Homepage: https://pkg.go.dev/github.com/chenyahui/gin-cache
- Size: 81.1 KB
- Stars: 263
- Watchers: 4
- Forks: 50
- Open Issues: 9
-
Metadata Files:
- Readme: README_ZH.md
- License: LICENSE
Awesome Lists containing this project
- awesome-go - gin-cache - high performance gin middleware to cache http response. Compared to gin-contrib/cache, It has a huge performance improvement. (Middlewares & framework add-ons)
README
# gin-cache
[](https://github.com/chenyahui/gin-cache/releases)
[](https://pkg.go.dev/github.com/chenyahui/gin-cache)
[](https://goreportcard.com/report/github.com/chenyahui/gin-cache)

[](https://codecov.io/gh/chenyahui/gin-cache)[English](README_ZH.md) | 🇨🇳中文
一个用于缓存http接口内容的gin高性能中间件。相比于官方的gin-contrib/cache,gin-cache有巨大的性能提升。
# 特性
* 相比于gin-contrib/cache,性能提升巨大。
* 同时支持本机内存和redis作为缓存后端。
* 支持用户根据请求来指定cache策略。
* 使用singleflight解决了缓存击穿问题。
* 仅缓存http状态码为2xx的回包# 用法
## 安装
```
go get -u github.com/chenyahui/gin-cache
```## 例子
## 使用本地缓存
```go
package mainimport (
"time""github.com/chenyahui/gin-cache"
"github.com/chenyahui/gin-cache/persist"
"github.com/gin-gonic/gin"
)func main() {
app := gin.New()memoryStore := persist.NewMemoryStore(1 * time.Minute)
app.GET("/hello",
cache.CacheByRequestURI(memoryStore, 2*time.Second),
func(c *gin.Context) {
c.String(200, "hello world")
},
)if err := app.Run(":8080"); err != nil {
panic(err)
}
}
```### 使用redis作为缓存
```go
package mainimport (
"time""github.com/chenyahui/gin-cache"
"github.com/chenyahui/gin-cache/persist"
"github.com/gin-gonic/gin"
"github.com/go-redis/redis/v8"
)func main() {
app := gin.New()redisStore := persist.NewRedisStore(redis.NewClient(&redis.Options{
Network: "tcp",
Addr: "127.0.0.1:6379",
}))app.GET("/hello",
cache.CacheByRequestURI(redisStore, 2*time.Second),
func(c *gin.Context) {
c.String(200, "hello world")
},
)
if err := app.Run(":8080"); err != nil {
panic(err)
}
}
```# 压测
```
wrk -c 500 -d 1m -t 5 http://127.0.0.1:8080/hello
```## MemoryStore
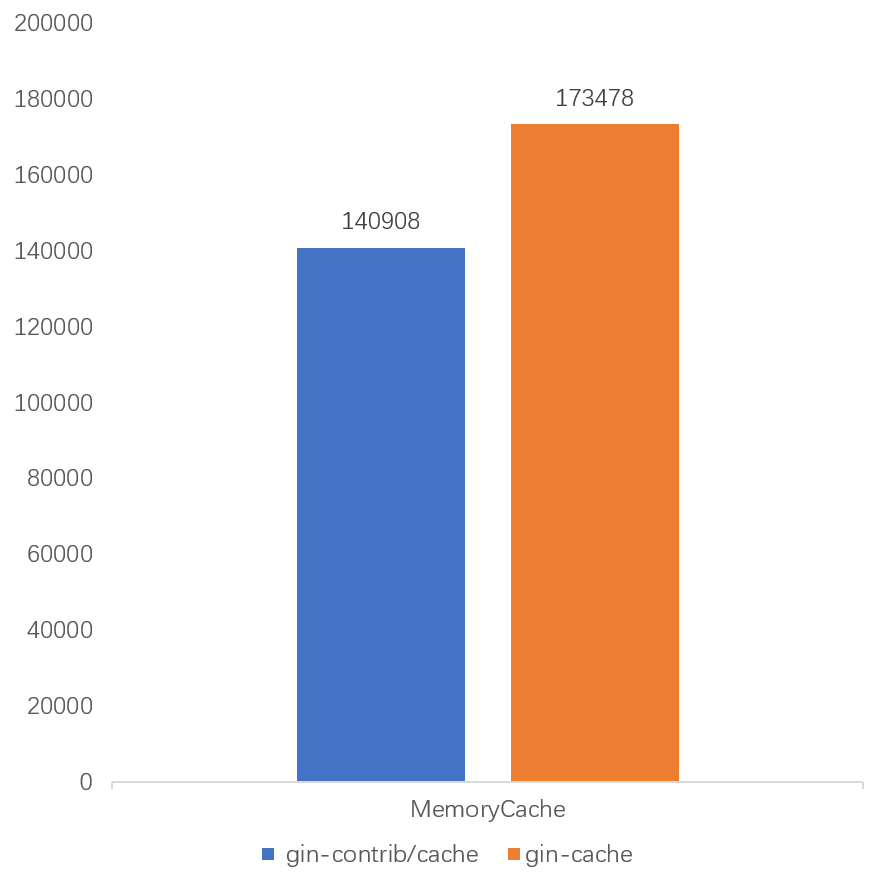
## RedisStore
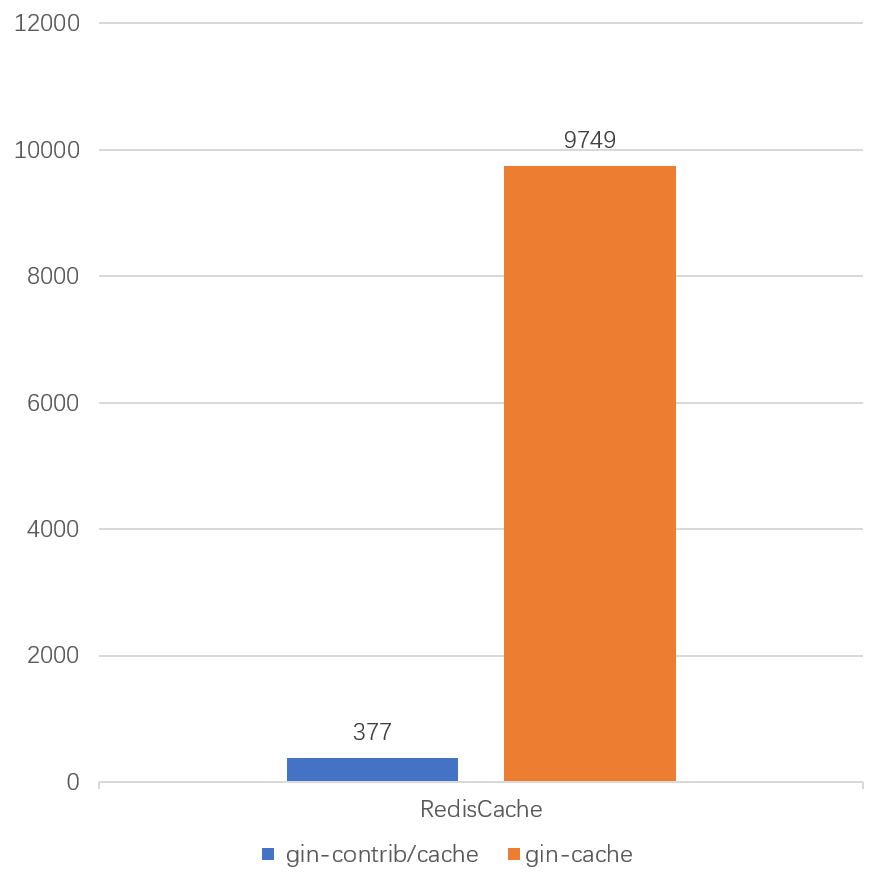