Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/ciromirkin/inputcollection_react
Input Collection React Component
https://github.com/ciromirkin/inputcollection_react
component react typescript
Last synced: about 5 hours ago
JSON representation
Input Collection React Component
- Host: GitHub
- URL: https://github.com/ciromirkin/inputcollection_react
- Owner: CiroMirkin
- Created: 2024-08-21T14:28:44.000Z (3 months ago)
- Default Branch: main
- Last Pushed: 2024-09-10T00:26:48.000Z (2 months ago)
- Last Synced: 2024-09-10T04:09:18.899Z (2 months ago)
- Topics: component, react, typescript
- Language: TypeScript
- Homepage:
- Size: 66.4 KB
- Stars: 0
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Input Collection React Component
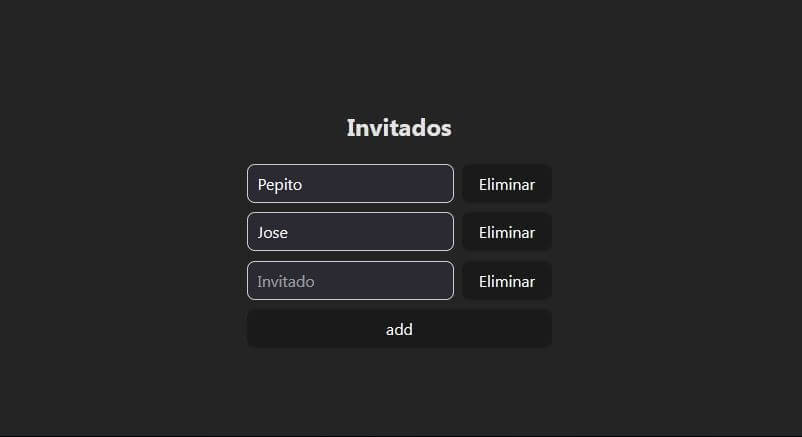
```jsx
import { useState } from 'react'
import './App.css'
import InputCollection, { InputList, InputCollectionConfig } from './InputCollection'function App() {
const [ names, setNames ] = useState([] as InputList)const config: InputCollectionConfig = {
textOfDeleteInputBtn: 'Delete',
classOfDeleteInputBtn: 'btn',
textOfAddInputBtn: 'Add',
classOfAddInputBtn: 'btn',
inputsPlaceholder: 'Write the name...',
inputsClassName: 'input',
inputsType: "text",
}return (
)
}
```Component with a custom button:
```jsx
import { useState } from 'react'
import InputCollection, { InputList, InputCollectionConfig } from './InputCollection'function App() {
const [ names, setNames ] = useState([] as InputList)const config: InputCollectionConfig = {
customBtnComponent: Add
}return (
)
}
```Component without optional attributes:
```jsx
function App() {
const [ inputs, setInputs ] = useState([
{
id: '1',
value: 'Lorem 1'
},
{
id: '2',
value: 'Lorem 2'
}
] as InputList)return (
)
}
```You can copy and paste the [InputCollection.tsx](./src/InputCollection.tsx) file into your project.
## Running The Project Locally
Clone The Project:
```
git clone [email protected]:CiroMirkin/InputCollection_React.git
```Install Dependencies:
```
npm i
```Start The Development Server:
```
npm run dev
```# Component Testing
Run [Cypress](https://docs.cypress.io/app/component-testing/react/overview) with:
```
npx cypress open
```Click on `Component Testing` and choose a browser.