https://github.com/cpp-for-yourself/lectures-and-homeworks
📚 A place for all lecture scripts, projects etc. for the "C++ for yourself" course
https://github.com/cpp-for-yourself/lectures-and-homeworks
clang cmake coding cplusplus cpp education gcc lecture lecture-notes lectures markdown marp modern-cpp slides software software-architecture software-development software-engineering software-testing testing
Last synced: about 1 month ago
JSON representation
📚 A place for all lecture scripts, projects etc. for the "C++ for yourself" course
- Host: GitHub
- URL: https://github.com/cpp-for-yourself/lectures-and-homeworks
- Owner: cpp-for-yourself
- License: mit
- Created: 2022-08-04T18:47:21.000Z (almost 3 years ago)
- Default Branch: main
- Last Pushed: 2025-03-29T11:38:25.000Z (about 1 month ago)
- Last Synced: 2025-03-29T12:27:38.153Z (about 1 month ago)
- Topics: clang, cmake, coding, cplusplus, cpp, education, gcc, lecture, lecture-notes, lectures, markdown, marp, modern-cpp, slides, software, software-architecture, software-development, software-engineering, software-testing, testing
- Language: TypeScript
- Homepage: https://www.youtube.com/code-for-yourself
- Size: 2.19 MB
- Stars: 108
- Watchers: 5
- Forks: 19
- Open Issues: 3
-
Metadata Files:
- Readme: readme.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
README
# Lectures and projects for the [C++ for yourself](https://youtube.com/playlist?list=PLwhKb0RIaIS1sJkejUmWj-0lk7v_xgCuT) course

[](https://www.youtube.com/code-for-yourself)
This repository is a collection of Markdown scripts used for all the videos in the [C++ for yourself](https://youtube.com/playlist?list=PLwhKb0RIaIS1sJkejUmWj-0lk7v_xgCuT) course.
These can be used to follow-along the video or as a standalone learning material.
## 🙏 Support this work
[](https://github.com/sponsors/niosus)
[](https://www.audibletrial.com/CodeForYourself)
[](https://github.com/cpp-for-yourself/sponsor/blob/main/amazon.md)Please remember that there is a human behind all of this work. I write scripts, create animations, record and edit videos at night after a full-time work day. It is at times hard.
But _you_ can make it easier. Here is what you can do:- 💶 Become a [**sponsor on GitHub**](https://github.com/sponsors/niosus)!
- 💸 Can't support monetarily?
+ 👍 **Like** my videos!
+ 💬 **Leave comments** on YouTube!
+ 📢 **Spread the word** among your friends!
- 🤬 Don't like something? 🗣️ **Talk to me about it!** I am always eager to improve.## :bulb: How to follow this course
The course is designed to be consumed from top to bottom, so start at the beginning and you will always have enough knowledge for the next video.
That being said, I aim to leave links in the videos so that one could watch them out of order without much hassle.
Enjoy! 😎
## 📕 C++ for yourself lectures
Hello world program dissection
----------------------------------------------------------
[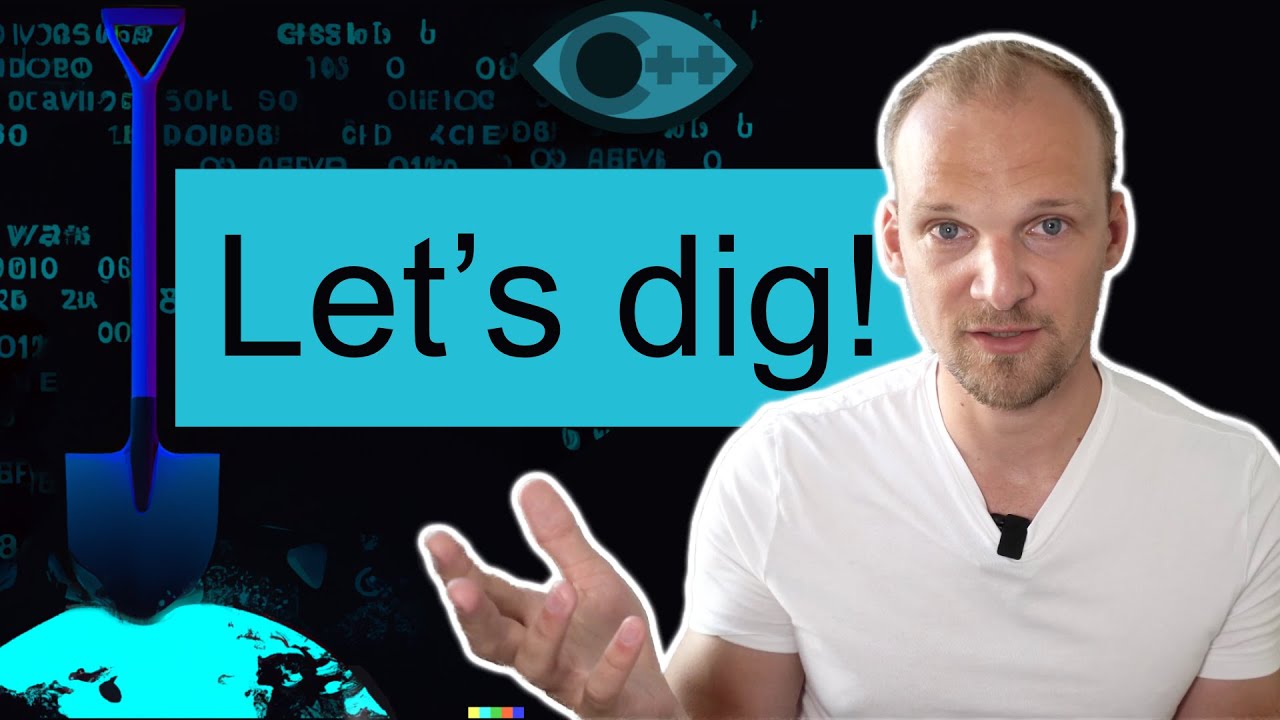](https://youtu.be/t2h1geGSww4)[Lecture script](lectures/hello_world_dissection.md)
- First keywords
- What brackets mean
- What do different signs mean
- Intro to "scopes"
- Intro to functions
- Intro to includes
----------------------------------------------------------
Project
: hello world program----------------------------------------------------------
[Homework script](homeworks/homework_1/homework.md)
- Write a simple program that prints `Hello World!`
- Learn to compile and run simple programs
----------------------------------------------------------Variables of fundamental types
----------------------------------------------------------
[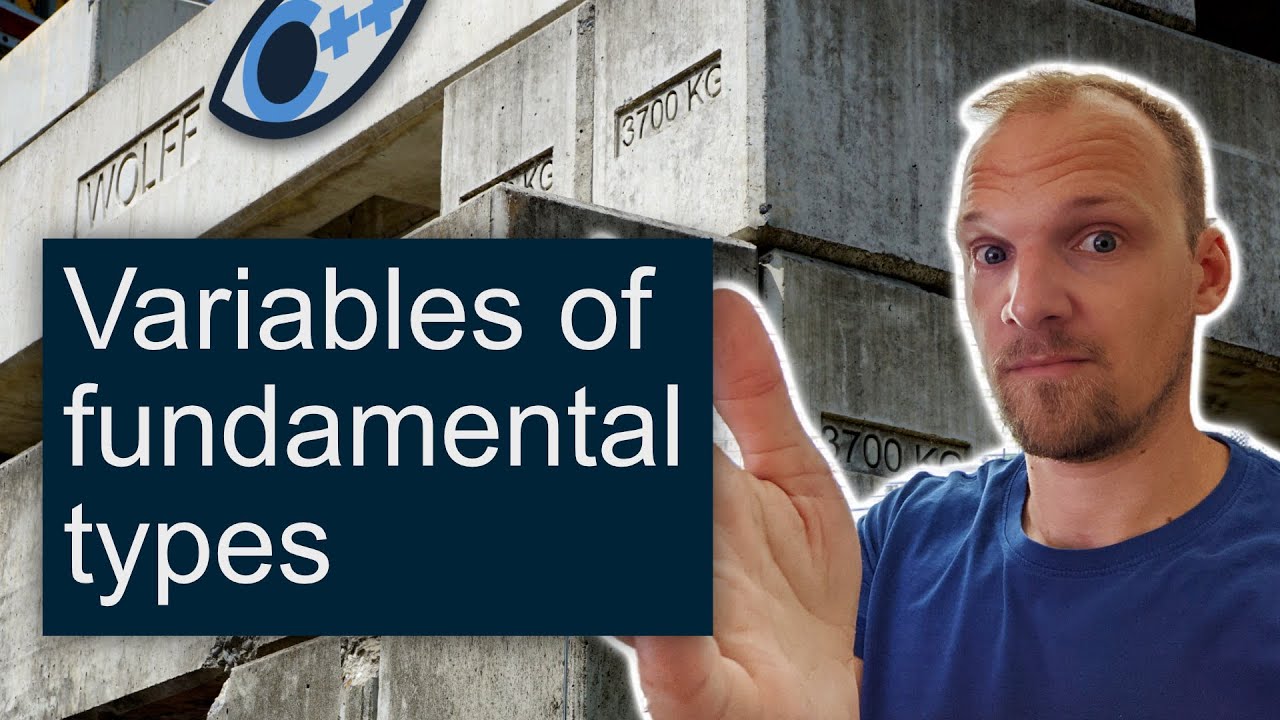](https://youtu.be/0z0gvv_Tb_U)[Lecture script](lectures/cpp_basic_types_and_variables.md)
- How to create variables of fundamental types
- Naming variables
- Using `const`, `constexpr` with variables
- References to variables
----------------------------------------------------------Namespaces for variables
-----------------------------------------------------------
[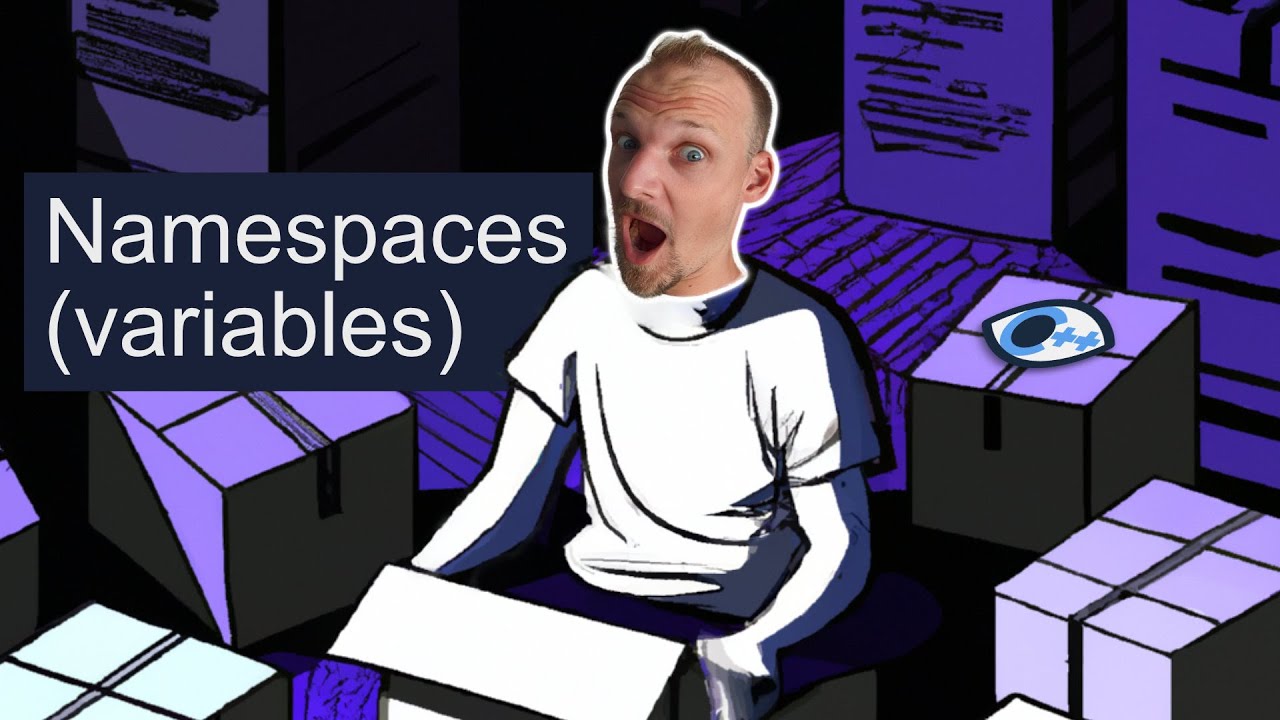](https://youtu.be/cP2IDg4_BRk)[Lecture script](lectures/namespaces_using.md)
- Namespaces with variables
- The word `using` with variables
----------------------------------------------------------Input/output streams
----------------------------------------------------------
[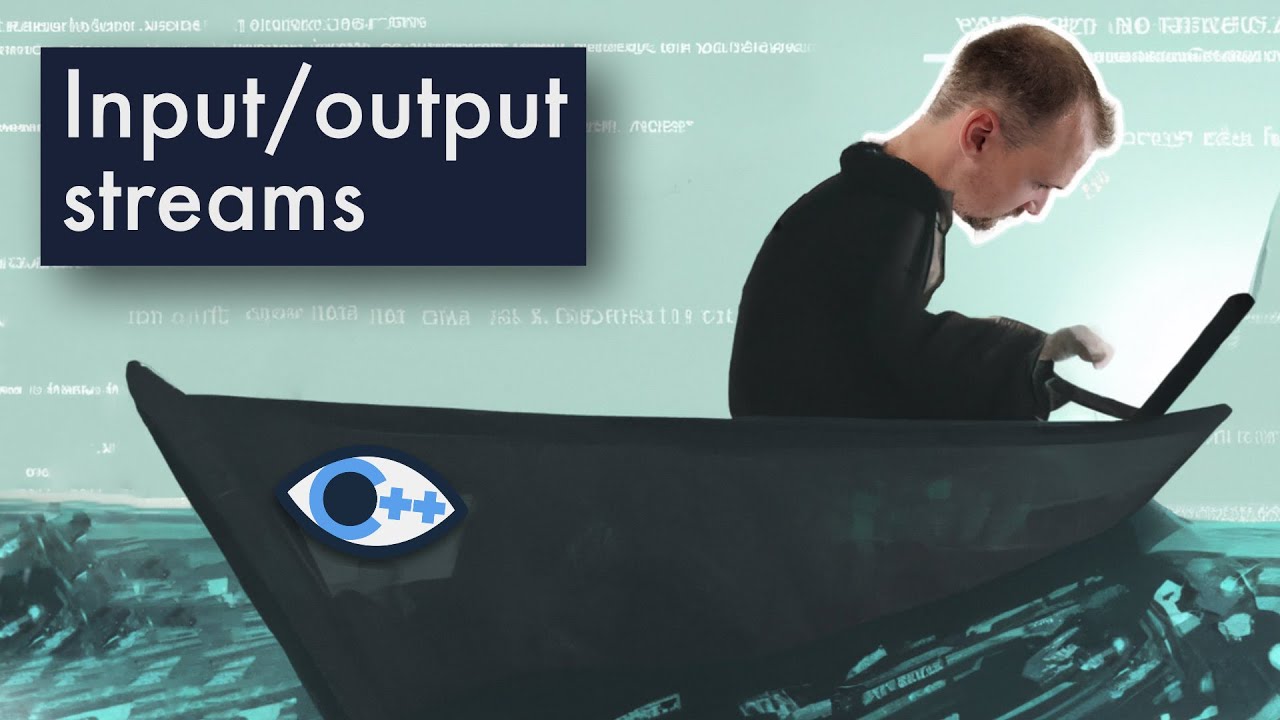](https://youtu.be/hy3eOpZmxbY)[Lecture script](lectures/more_useful_types.md)
- `std::cout`, `std::cerr`, `std::cin`
----------------------------------------------------------Sequence and utility containers
----------------------------------------------------------
[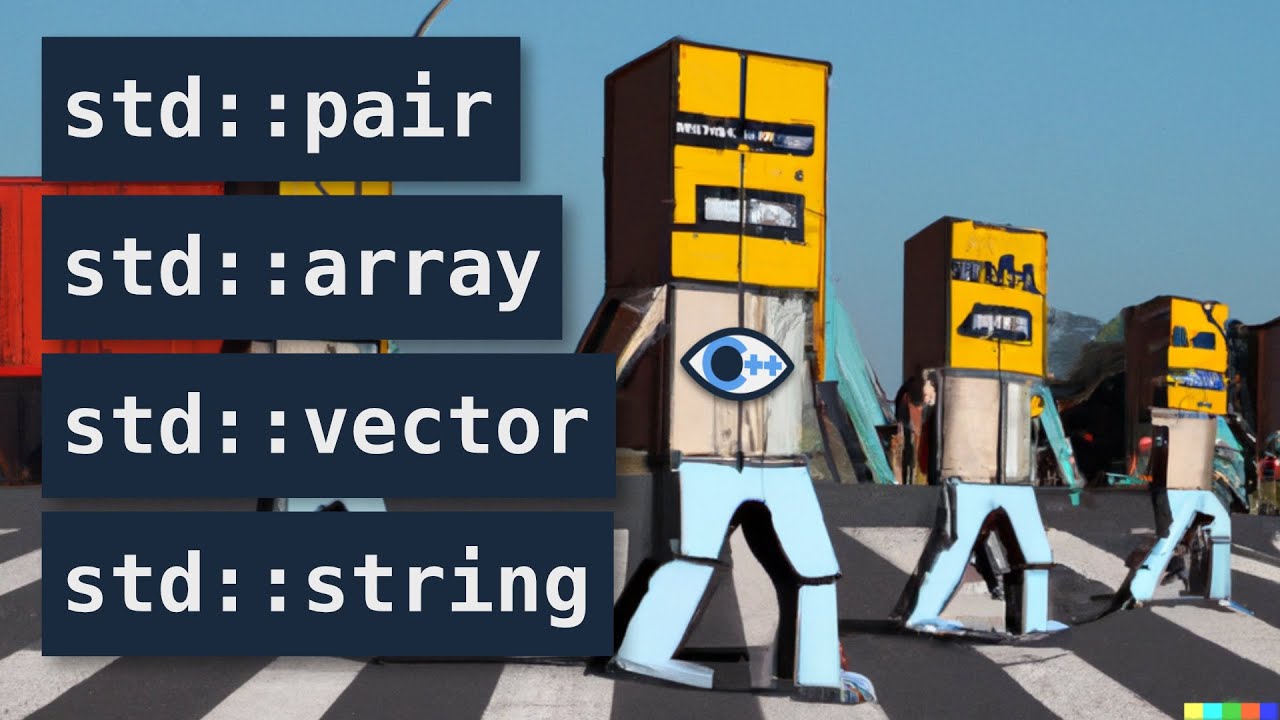](https://youtu.be/dwkSVkGsvFk)[Lecture script](lectures/more_useful_types.md)
- Sequence containers: `std::array`, `std::vector`, their usage and some caveats
- Pair container: `std::pair`
- Strings from STL: `std::string`
- Conversion to/from strings: `to_string`, `stoi`, `stod`, `stof`, etc.
- Aggregate initialization
----------------------------------------------------------Associative containers
----------------------------------------------------------
[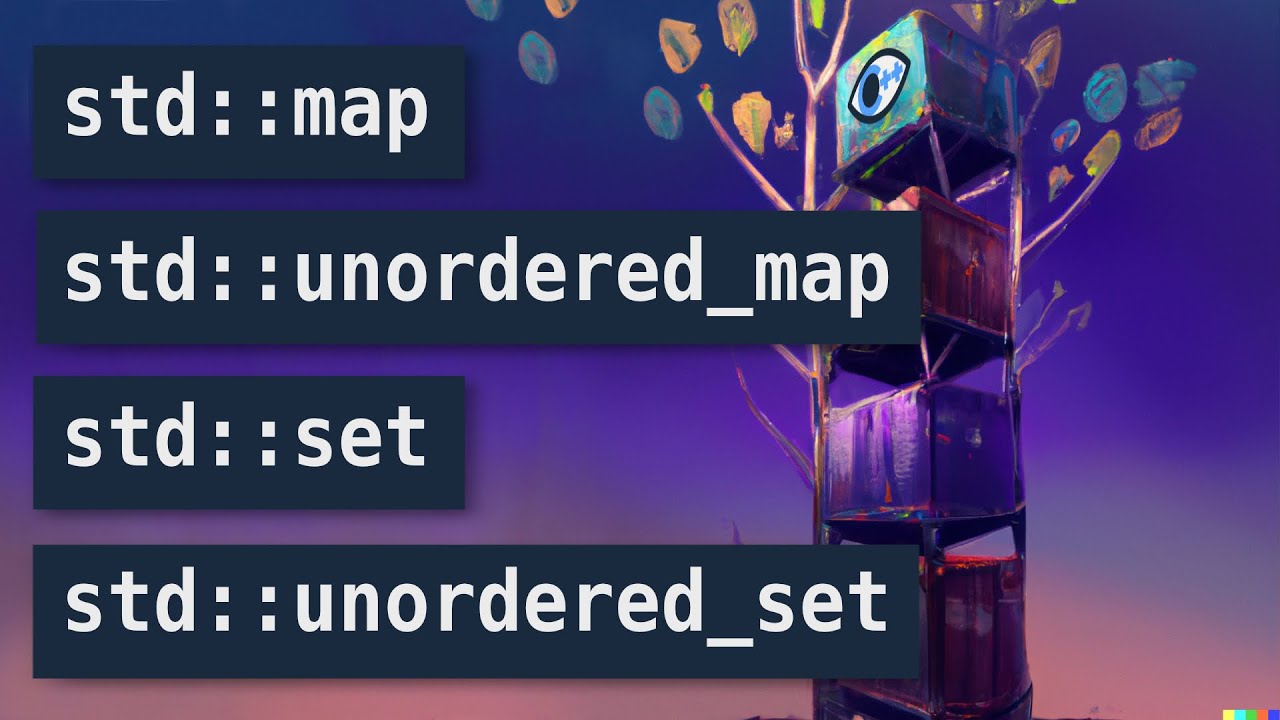](https://youtu.be/TCu76SYmVCg)[Lecture script](lectures/associative_containers.md)
- `std::map` and `std::unordered_map`
- Touch up on `std::set` and `std::unordered_set`
----------------------------------------------------------
Project
: fortune teller program----------------------------------------------------------
[Homework script](homeworks/homework_2/homework.md)
- Write a program that tells your C++ fortune
- It reads and writes data from and to terminal
- Stores and accesses these data in containers
----------------------------------------------------------Control structures
----------------------------------------------------------
[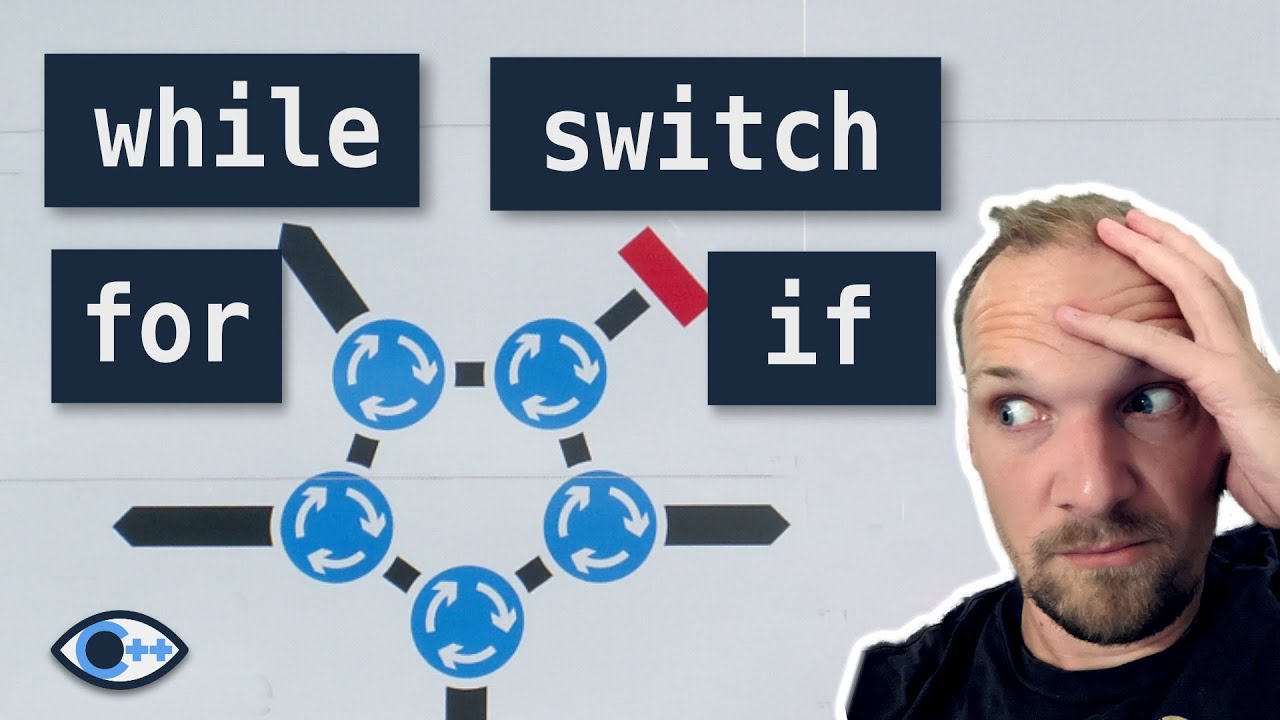](https://youtu.be/jzgTxosgGIA)[Lecture script](lectures/control_structures.md)
- `if`, `switch` and ternary operator
- `for`, `while` and `do ... while`
----------------------------------------------------------Random number generation
----------------------------------------------------------
[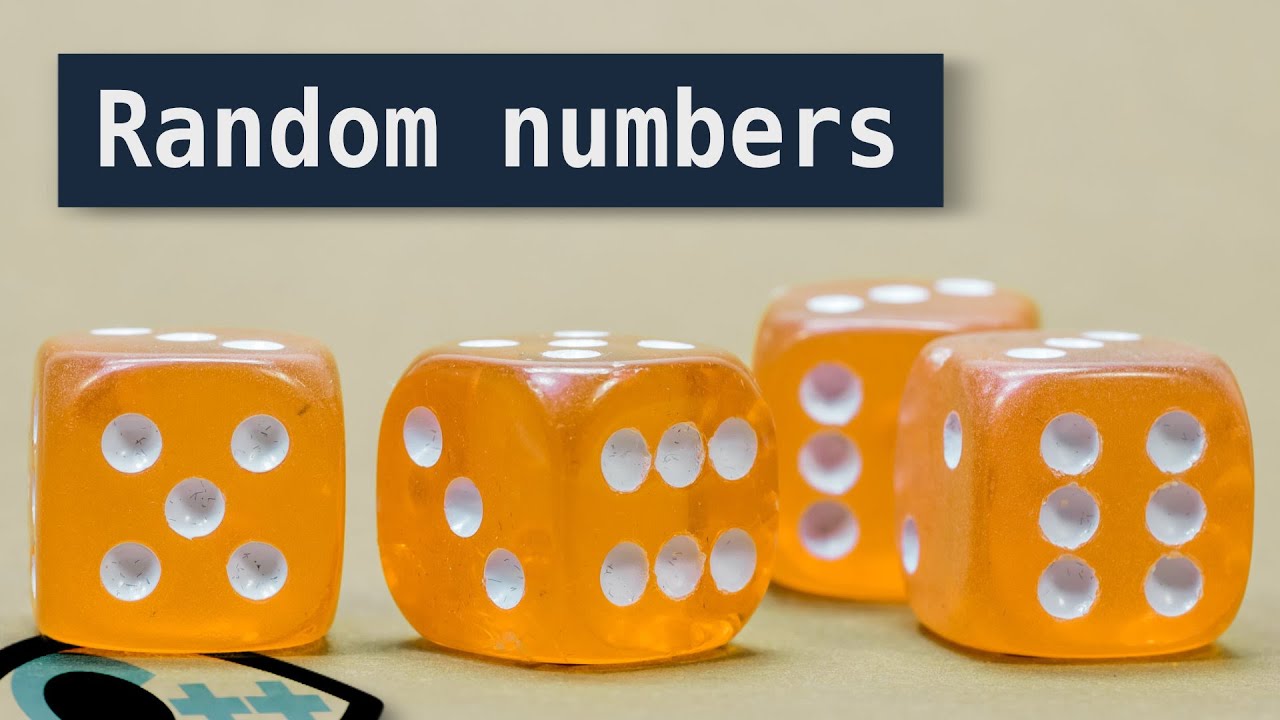](https://youtu.be/IUoqMTGGo6k)[Lecture script](lectures/random_numbers.md)
- What are random numbers
- How to generate them in modern C++
- Why not to use `rand()`
----------------------------------------------------------
Project
: the guessing game----------------------------------------------------------
[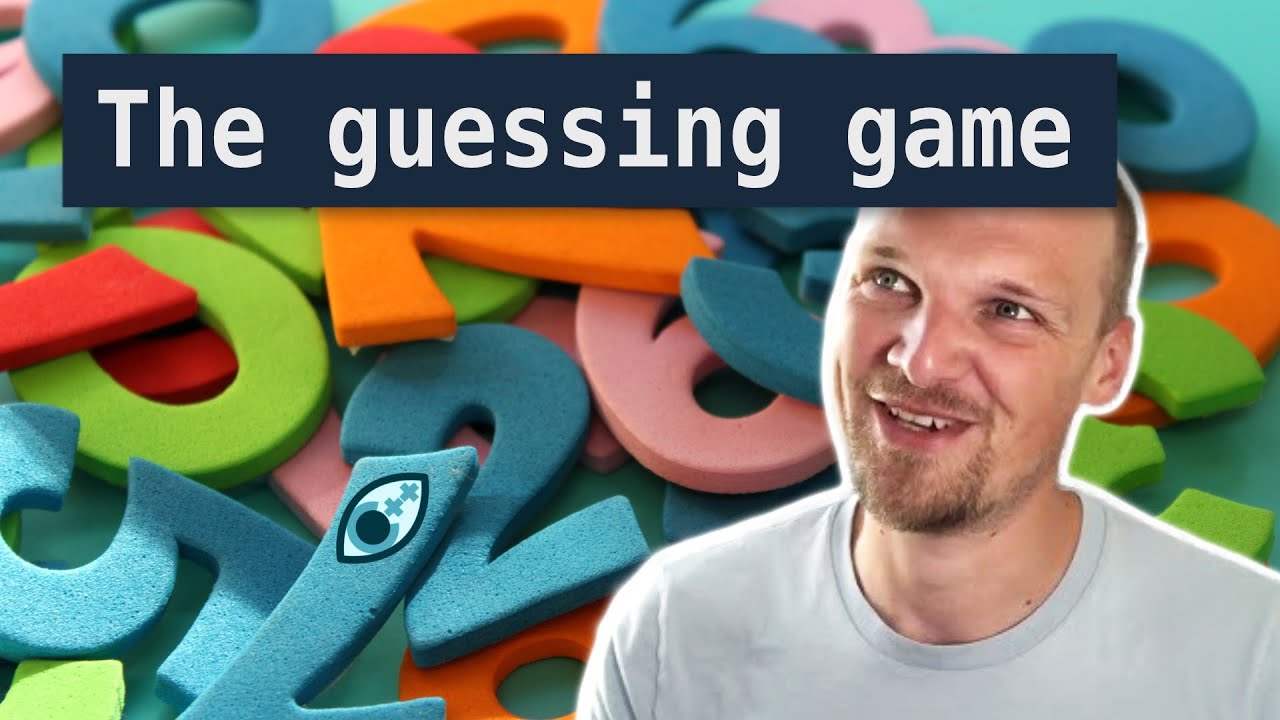](https://youtu.be/TYs_xwihCNc)[Homework script](homeworks/homework_3/homework.md)
- A program that generates a number
- The user guesses this number
- The program tells the user if they are above or below with their guess (or if they've won)
----------------------------------------------------------Compilation flags and debugging
----------------------------------------------------------
[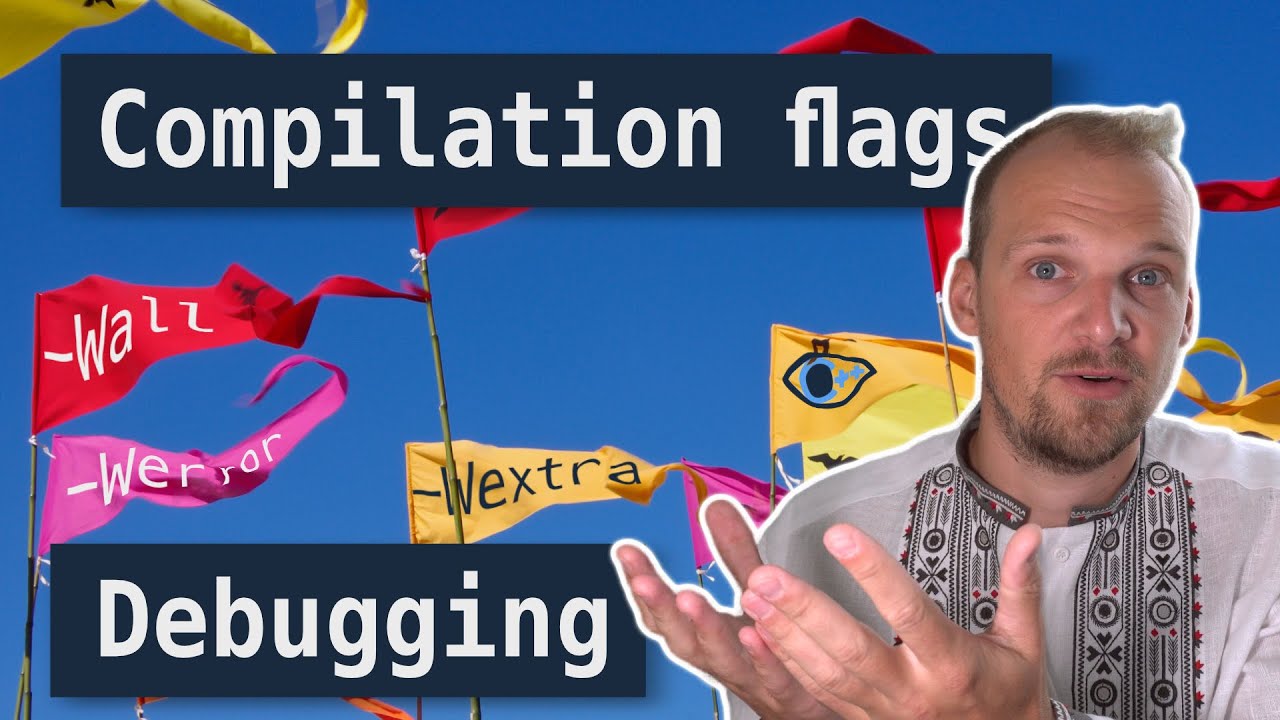](https://youtu.be/NTlcDv7W2-c)[Lecture script](lectures/compilation_debugging.md)
- Useful compilation flags
- Debugging a program with:
- Print statements
- `lldb` debugger
----------------------------------------------------------Functions
----------------------------------------------------------
[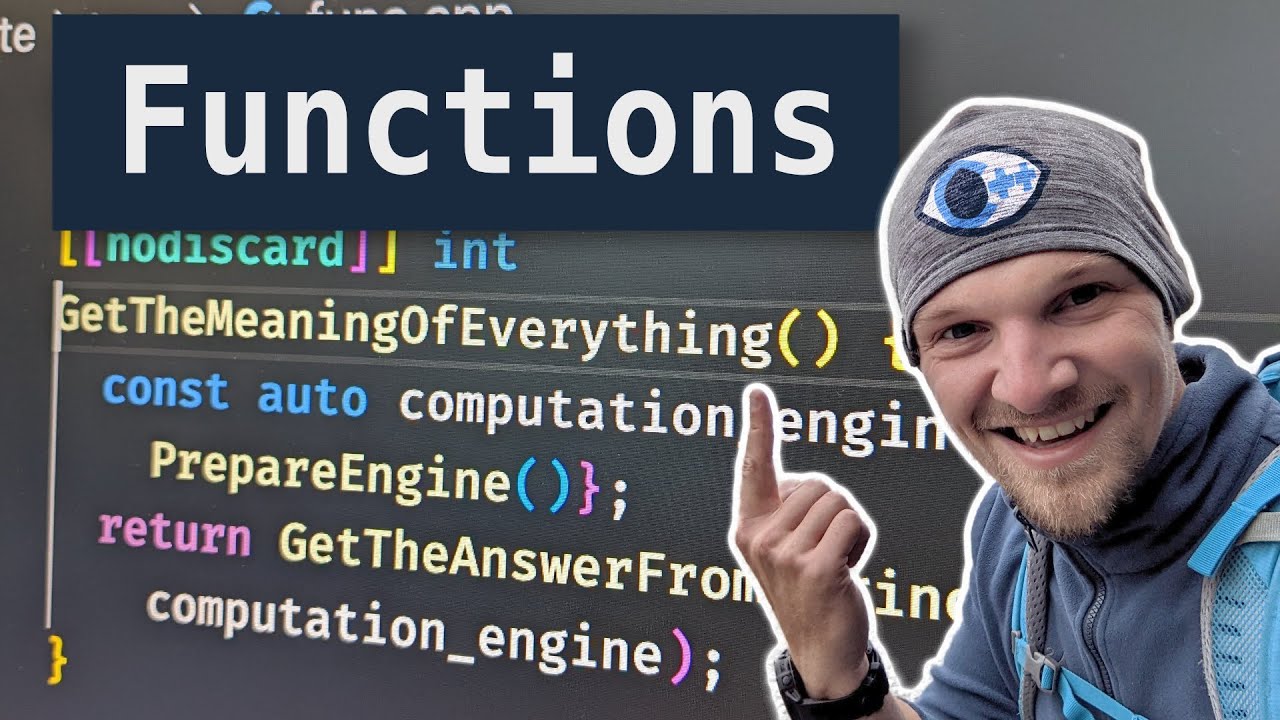](https://youtu.be/RaSw0g2aPig)[Lecture script](lectures/functions.md)
- What is a function
- Declaration and definition
- Passing by reference
- Overloading
- Using default arguments
----------------------------------------------------------Enumerations
----------------------------------------------------------
[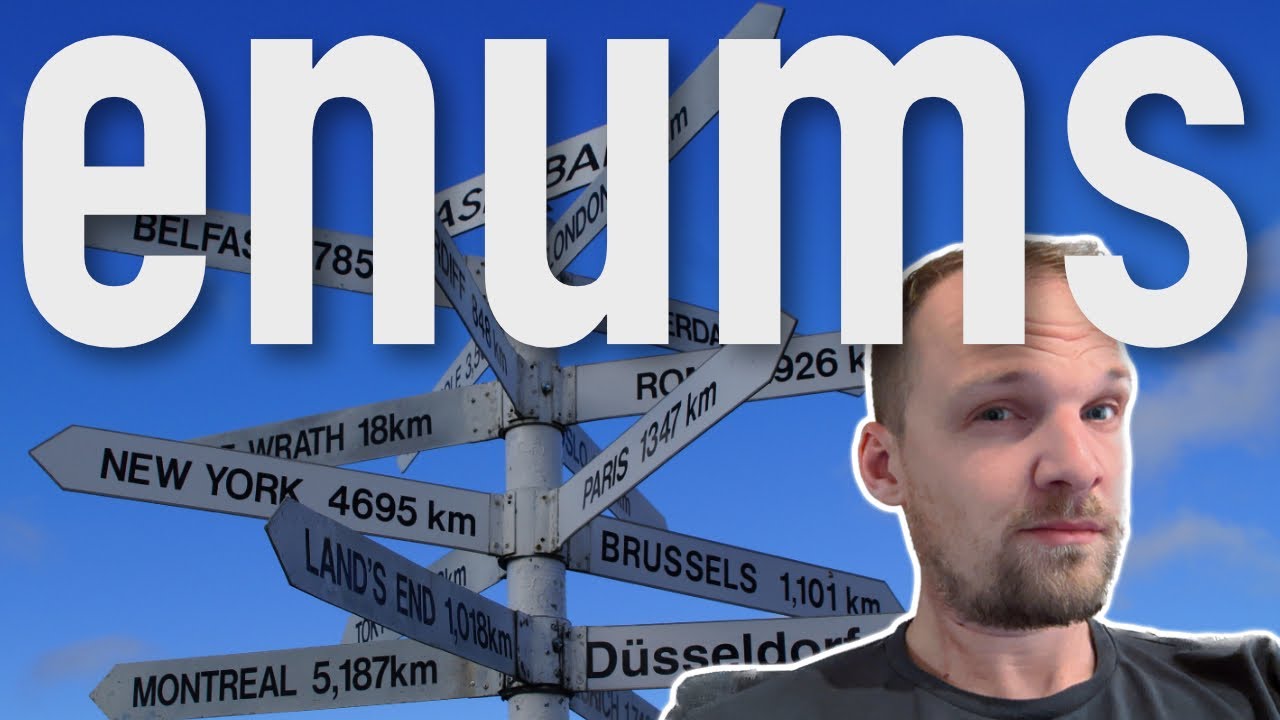](https://youtu.be/4kZyQ-TwH00)[Lecture script](lectures/enums.md)
- What are `enums`
- How to use them?
- Why not to use old style `enums`
----------------------------------------------------------Libraries and header files
----------------------------------------------------------
[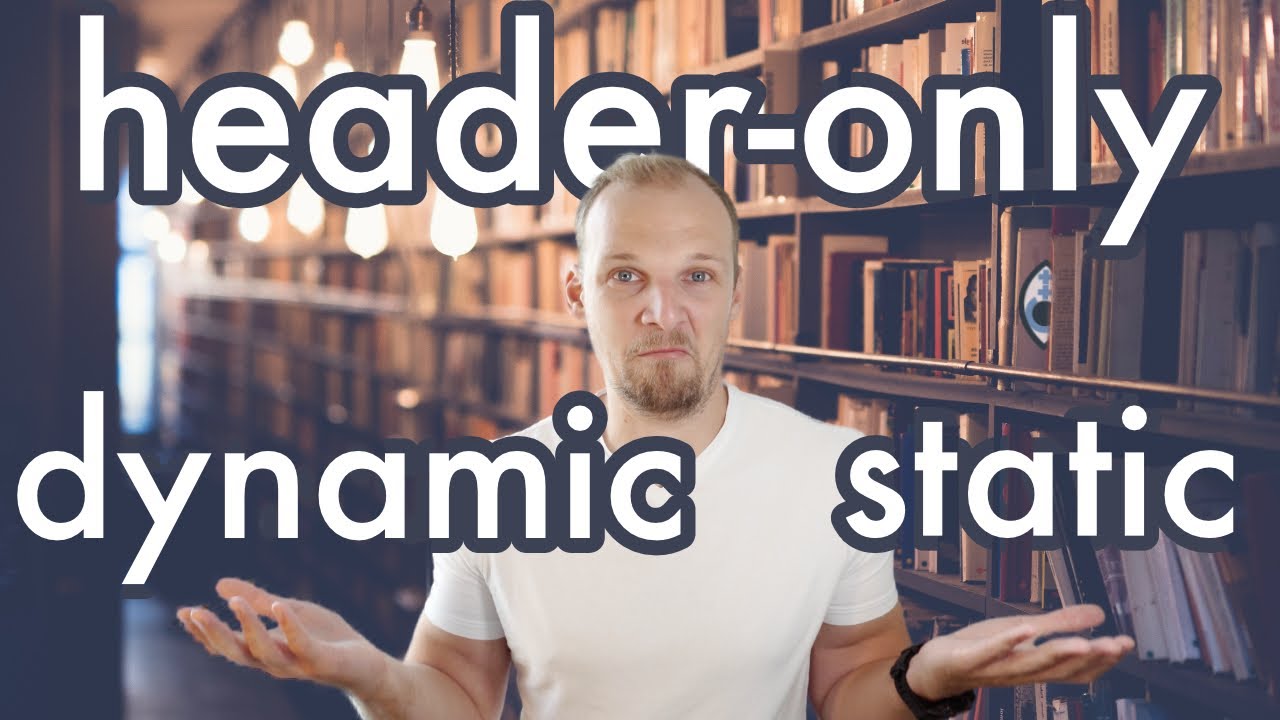](https://youtu.be/Lxo8ftglwXE)[Lecture script](lectures/headers_and_libraries.md)
- Different types of libraries
- Header-only
- Static
- Dynamic
- What is linking
- When to use the keyword `inline`
- Some common best practices
----------------------------------------------------------Build systems introduction
----------------------------------------------------------
[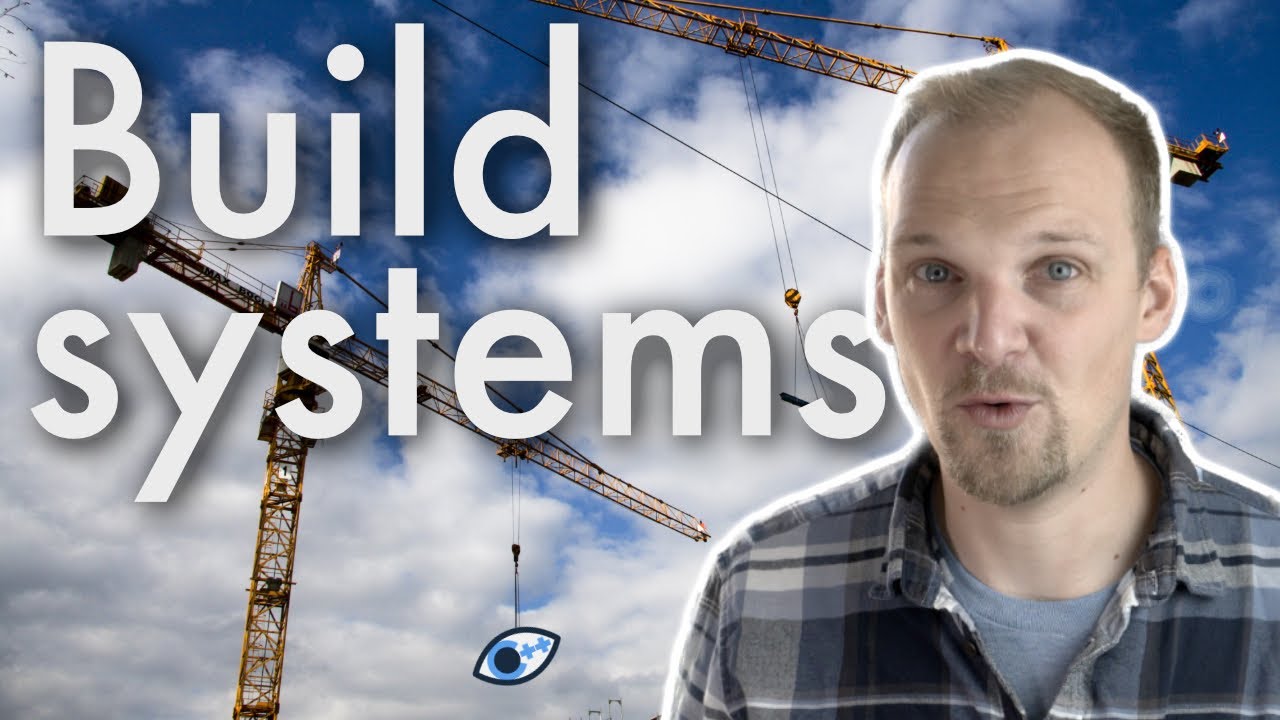](https://youtu.be/kbk4DphsYPU)[Lecture script](lectures/build_systems.md)
- Intro to build systems
- Build commands as a script
- Build commands in a `Makefile`
----------------------------------------------------------CMake introduction
----------------------------------------------------------
[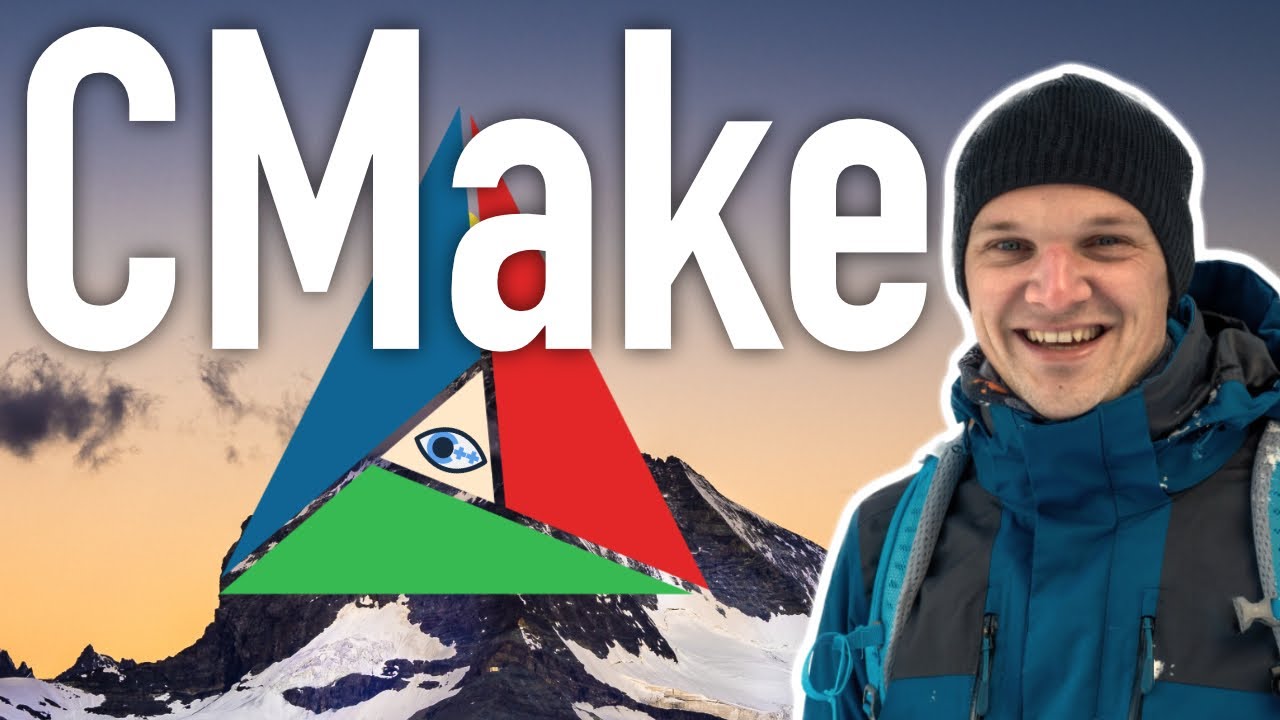](https://youtu.be/UH6F6ypdYbw)[Lecture script](lectures/cmake.md)
- Build process with CMake
- CMake Variables
- Targets and their properties
- Example CMake project
----------------------------------------------------------Using GoogleTest framework for testing code
----------------------------------------------------------
[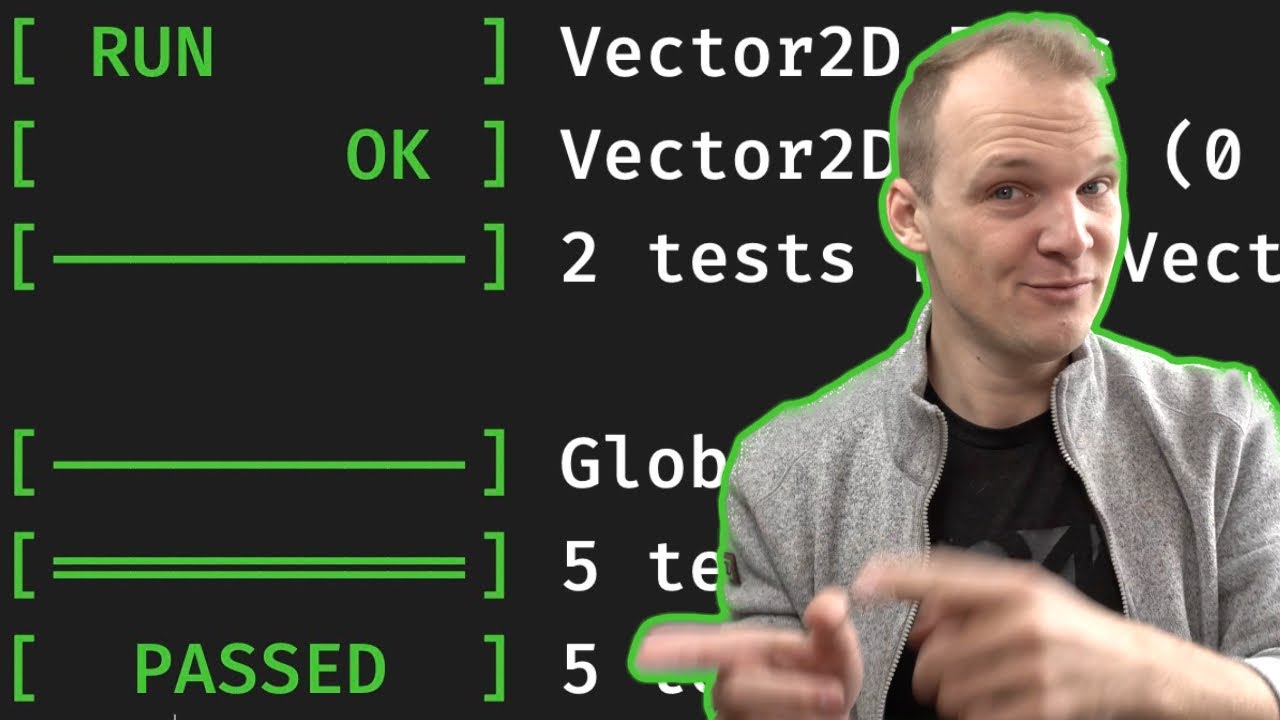](https://youtu.be/pxJoVRfpRPE)[Lecture script](lectures/googletest.md)
- Explain what testing is for
- Explain what testing is
- Show how to download and setup googletest
- Show how to write a simple test
----------------------------------------------------------
Project
: string processing library----------------------------------------------------------
[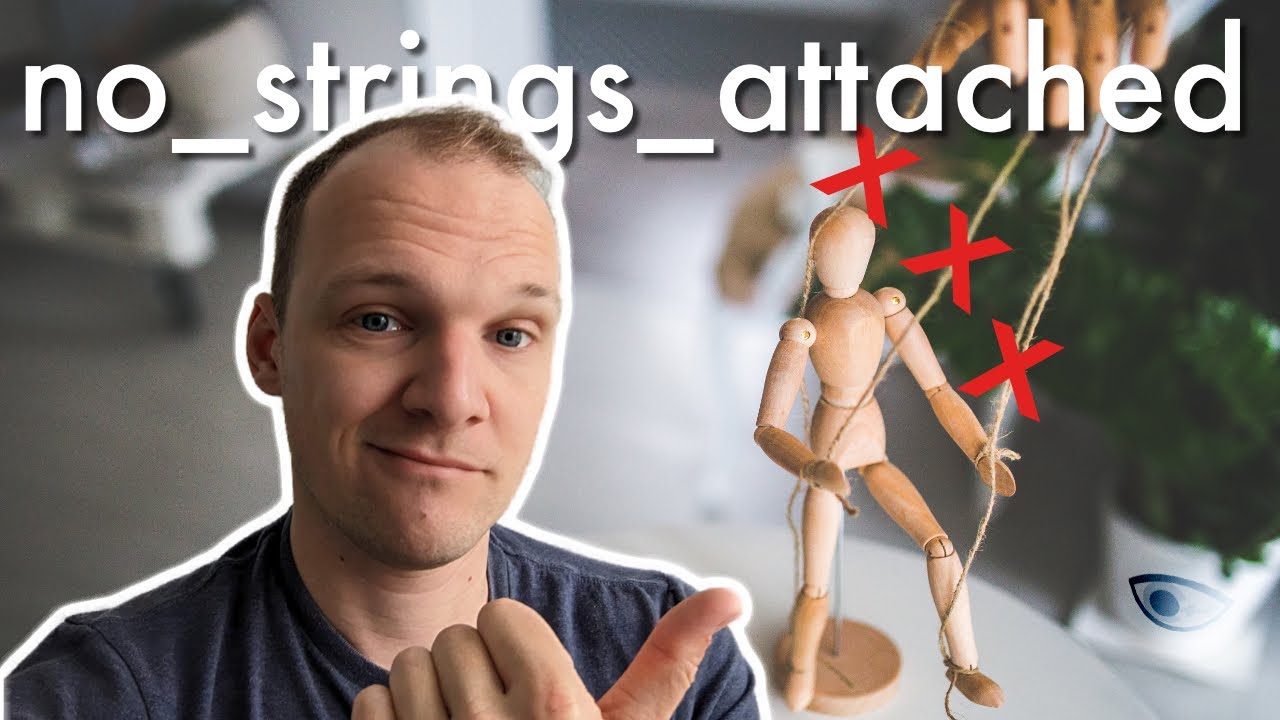](https://youtu.be/f0x2qcFgu5o)[Homework script](homeworks/homework_4/homework.md)
- You will write library that allows to split and trim strings
- You will learn how to:
- Write a CMake project from scratch
- Write your own libraries
- Test them with googletest
- Link them to binaries
----------------------------------------------------------Simple custom types with classes and structs
----------------------------------------------------------
[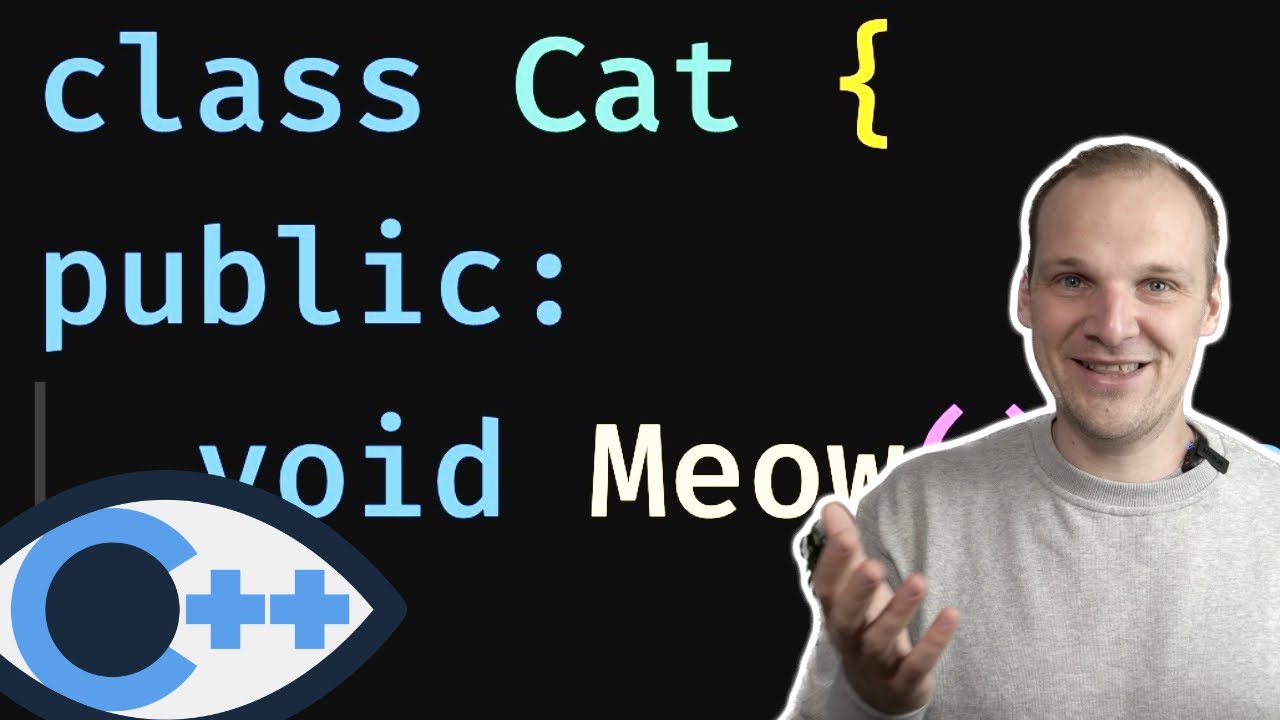](https://youtu.be/IijP--Xf5kQ)[Lecture script](lectures/classes_intro.md)
- Explain why the classes are needed
- Implement an example game about a car
- Define classes and structs more formally
----------------------------------------------------------Raw pointers
----------------------------------------------------------
[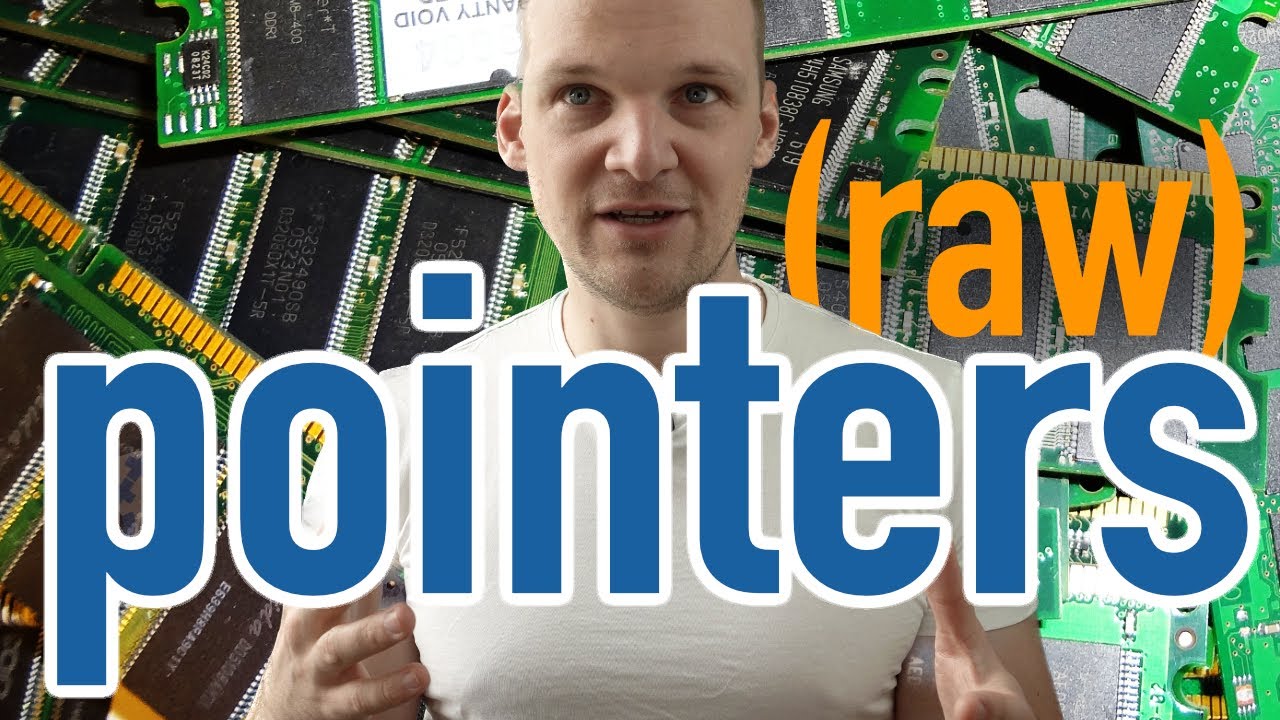](https://youtu.be/pptRG345jnU)[Lecture script](lectures/raw_pointers.md)
- The pointer type
- Pointers = variables of pointer types
- How to get the data?
- Initialization and assignment
- Using const with pointers
- Non-const pointer to const data
- Constant pointer to non-const data
- Constant pointer to constant data
----------------------------------------------------------Object lifecycle
----------------------------------------------------------
[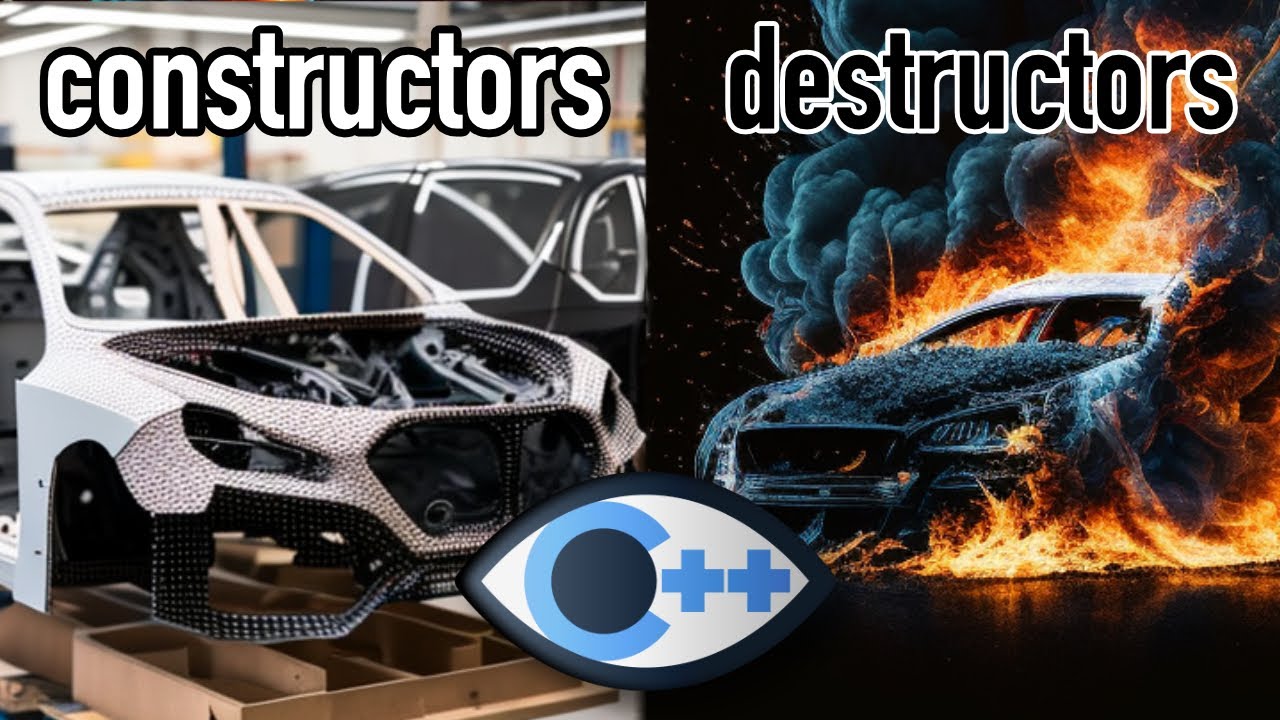](https://youtu.be/TFoav6vhgdg)[Lecture script](lectures/object_lifecycle.md)
- Creating a new object
- What happens when an object dies
- Full class lifecycle explained
----------------------------------------------------------Move semantics
----------------------------------------------------------
[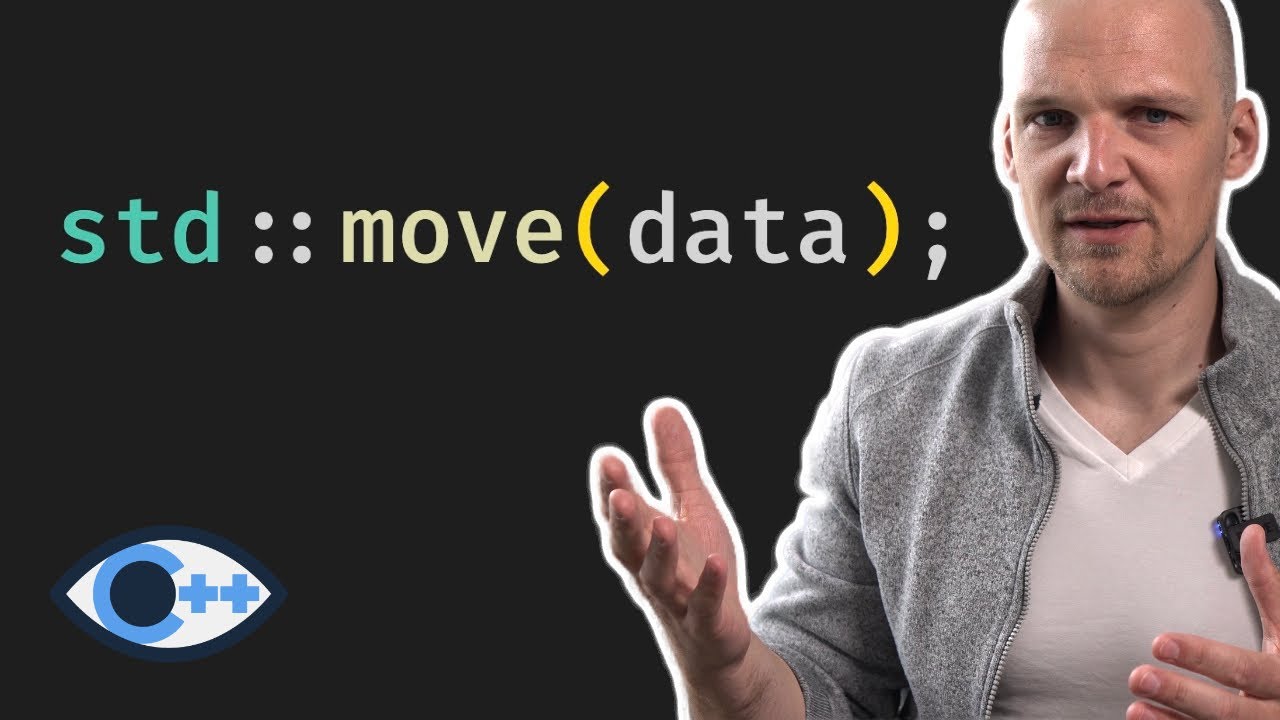](https://youtu.be/kqQ90R0_GFI)[Lecture script](lectures/move_semantics.md)
- Why we care about move semantics
- Let’s re-design move semantics from scratch
- How is it actually designed and called in Modern C++?----------------------------------------------------------
Constructors, operators, destructor - rule of all or nothing
----------------------------------------------------------
[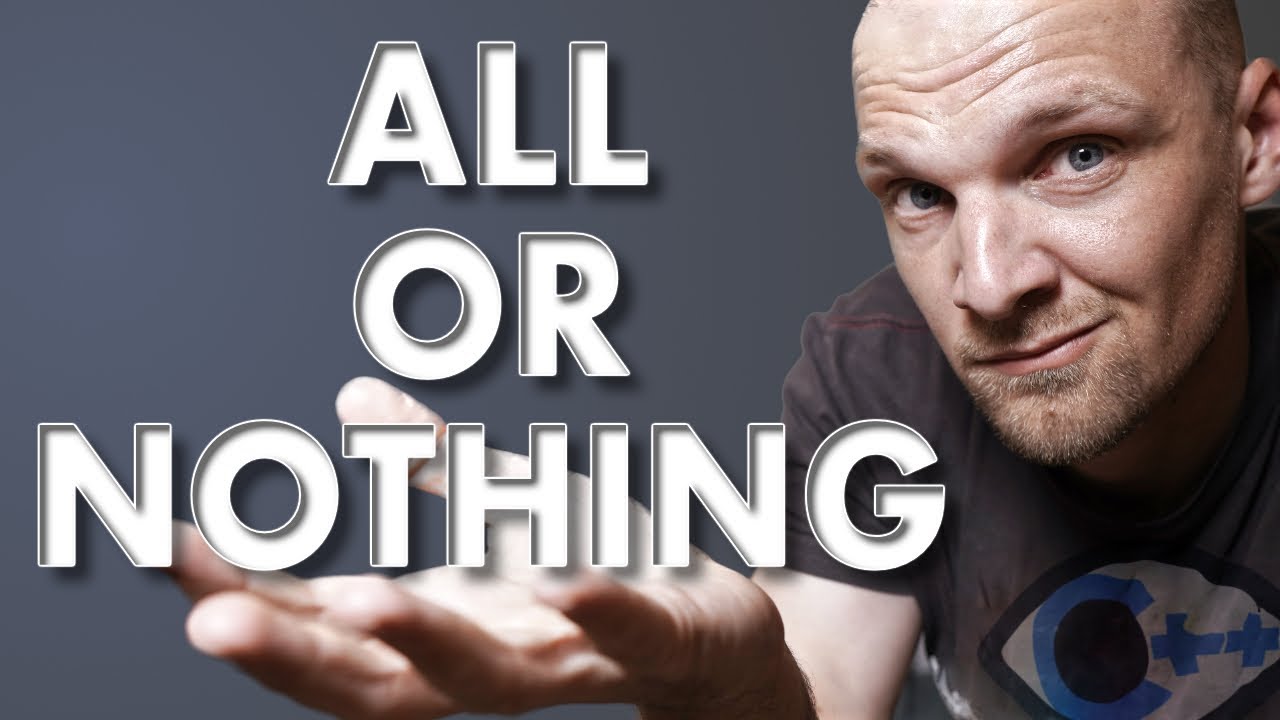](https://youtu.be/una89pkP9ms)[Lecture script](lectures/all_or_nothing.md)
- “Good style” as our guide
- What is “good style”
- Setting up the example
- Rule 1: destructor
- Rule 2: copy constructor
- Rule 3: copy assignment operator
- Rule 4: move constructor
- Rule 5: move assignment operator
- Now we (mostly) follow best practices
- Rule of 5 (and 3)
- The rule of “all or nothing”----------------------------------------------------------
Headers with classes
----------------------------------------------------------
[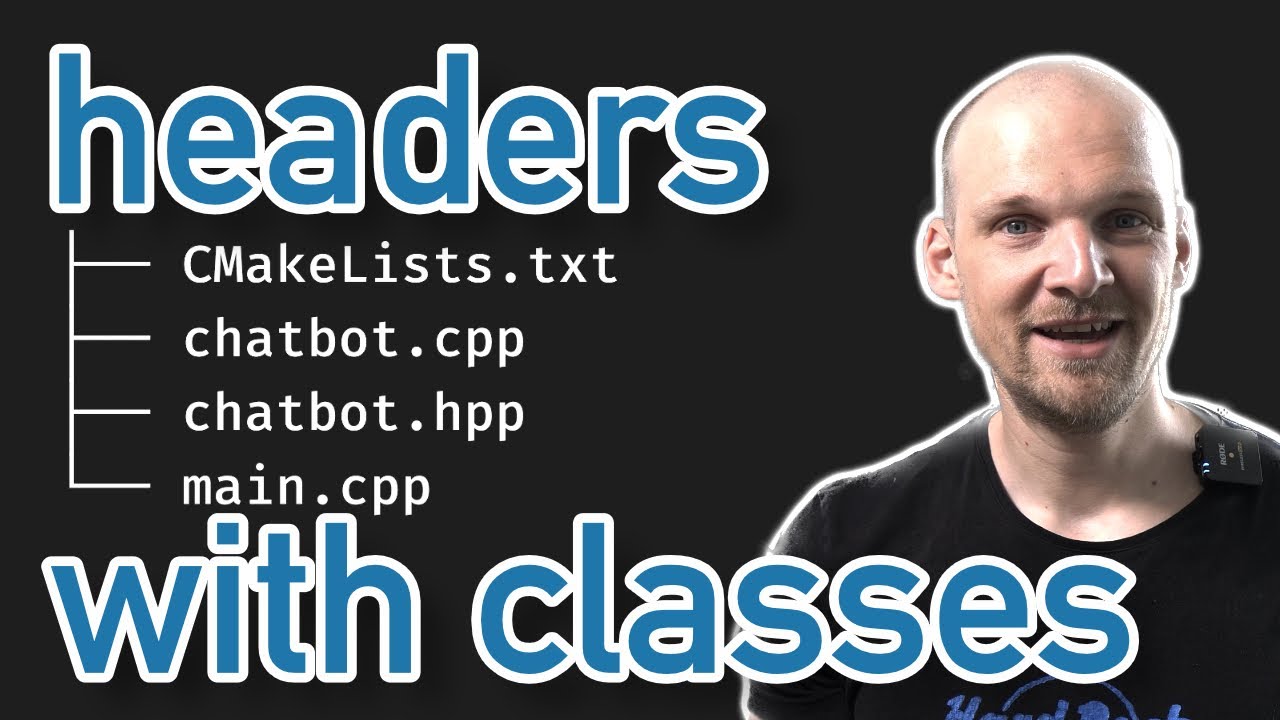](https://youtu.be/9MB1nHDIM64)[Lecture script](lectures/headers_with_classes.md)
- What stays the same
- What is different
- Example to show it all----------------------------------------------------------
Const correctness
----------------------------------------------------------
[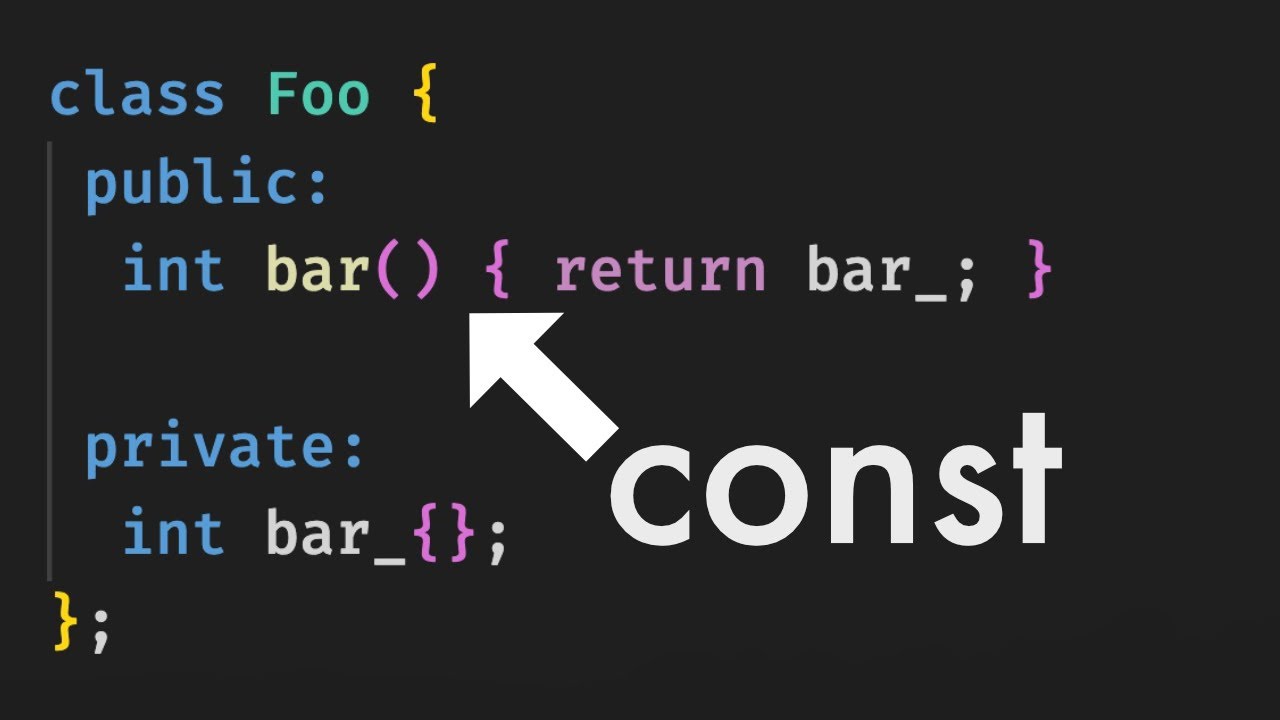](https://youtu.be/WsBdxq319OY)[Lecture script](lectures/const_correctness.md)
- What is const correctness
- Some rules and examples to follow in order to work with `const` correctly
----------------------------------------------------------
Project
: pixelate images in terminal----------------------------------------------------------
[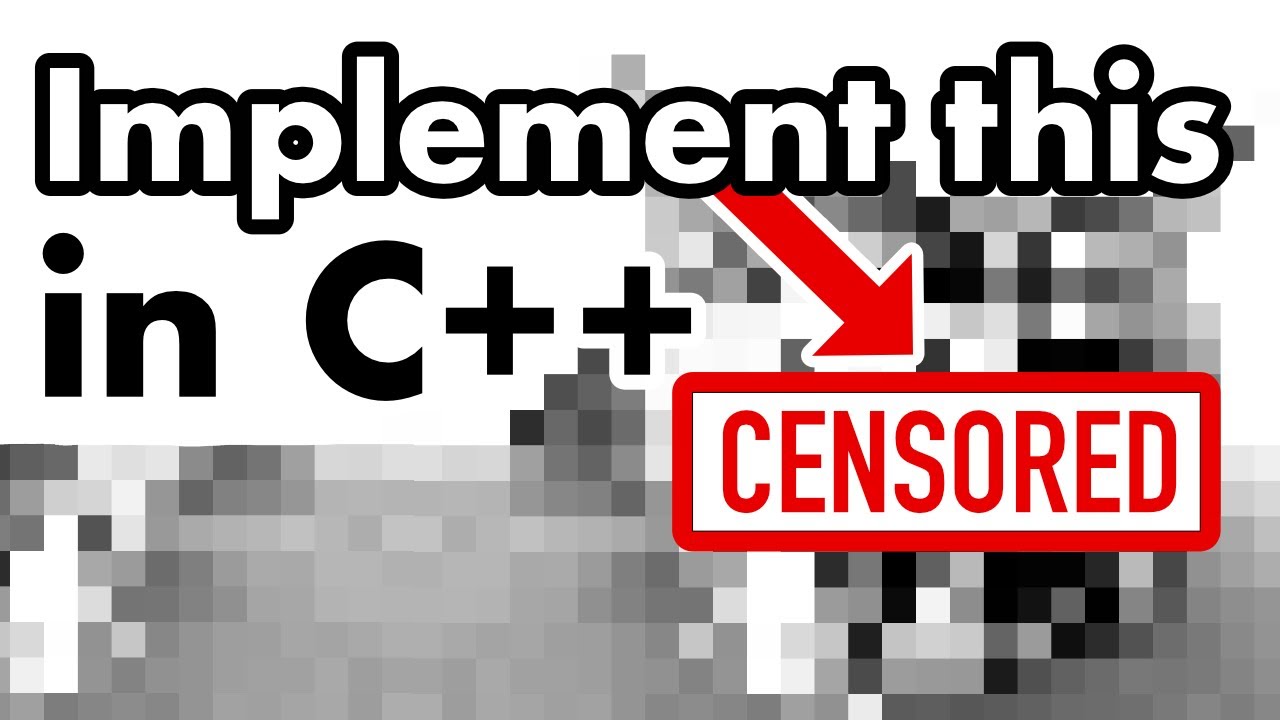](https://youtu.be/Cj3x51iJdvM)[Homework script](homeworks/homework_5/homework.md)
- You will write a library that allows to pixelate an image
- You will learn how to:
- Work with classes
- Use external libraries
- Read images from disk using `stb_image.h`
- Draw stuff in the terminal using `FTXUI` library
- Manage memory allocated elsewhere correctly
- Writing multiple libraries and binaries and linking them together
- Manage a larger CMake project
----------------------------------------------------------Keyword
static
outside of classes----------------------------------------------------------
[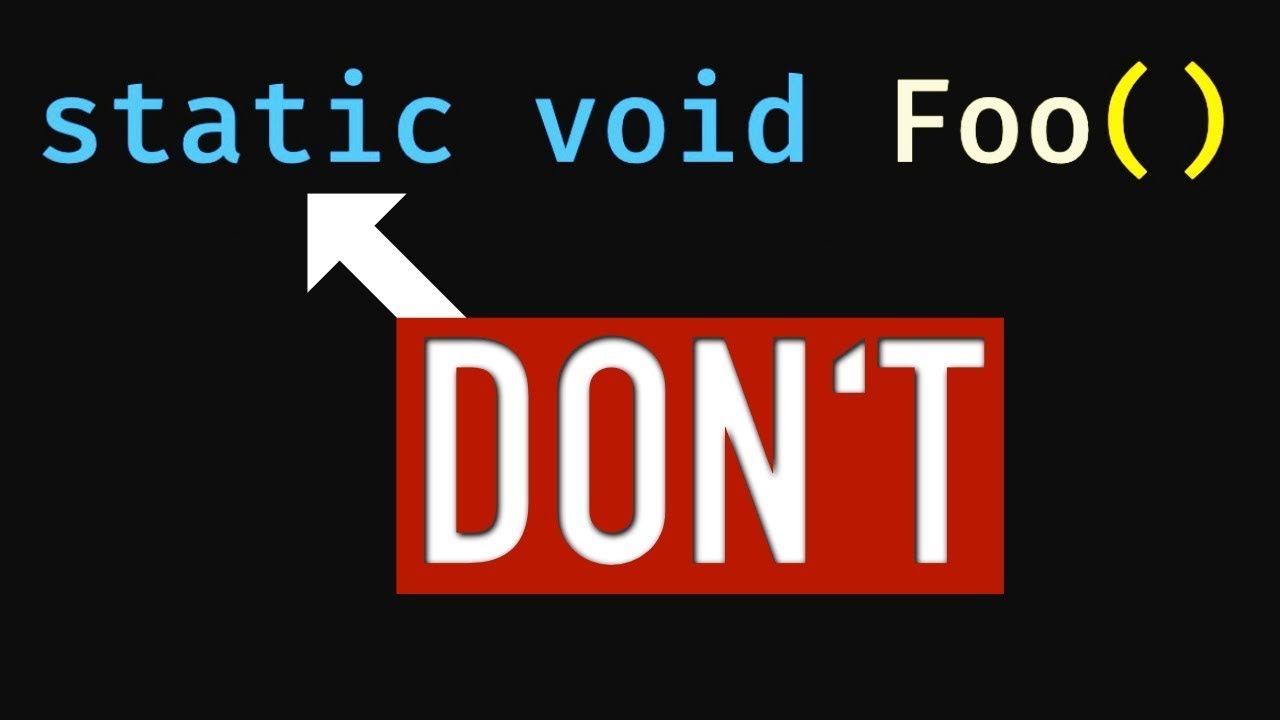](https://youtu.be/7cpPQunjv4s)[Lecture script](lectures/static_outside_classes.md)
- Why we should not use `static` outside of classes
- Relation to storage duration
- Relation to linkage
- Why we should use `inline` instead----------------------------------------------------------
Keyword
static
inside classes----------------------------------------------------------
[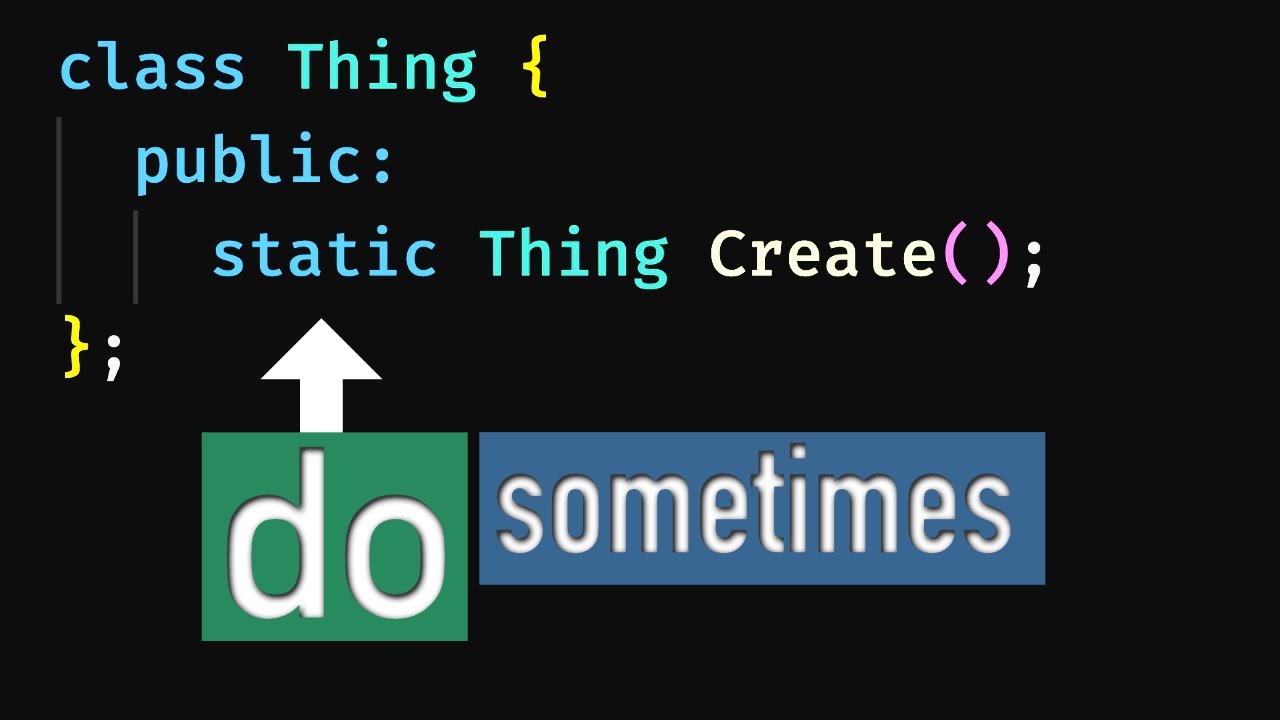](https://youtu.be/ggNCjDPShrA)[Lecture script](lectures/static_in_classes.md)
- Using `static` class methods
- Using `static` class data
- What is `static` in classes useful for?----------------------------------------------------------
Templates: why would we want to use them?
----------------------------------------------------------
[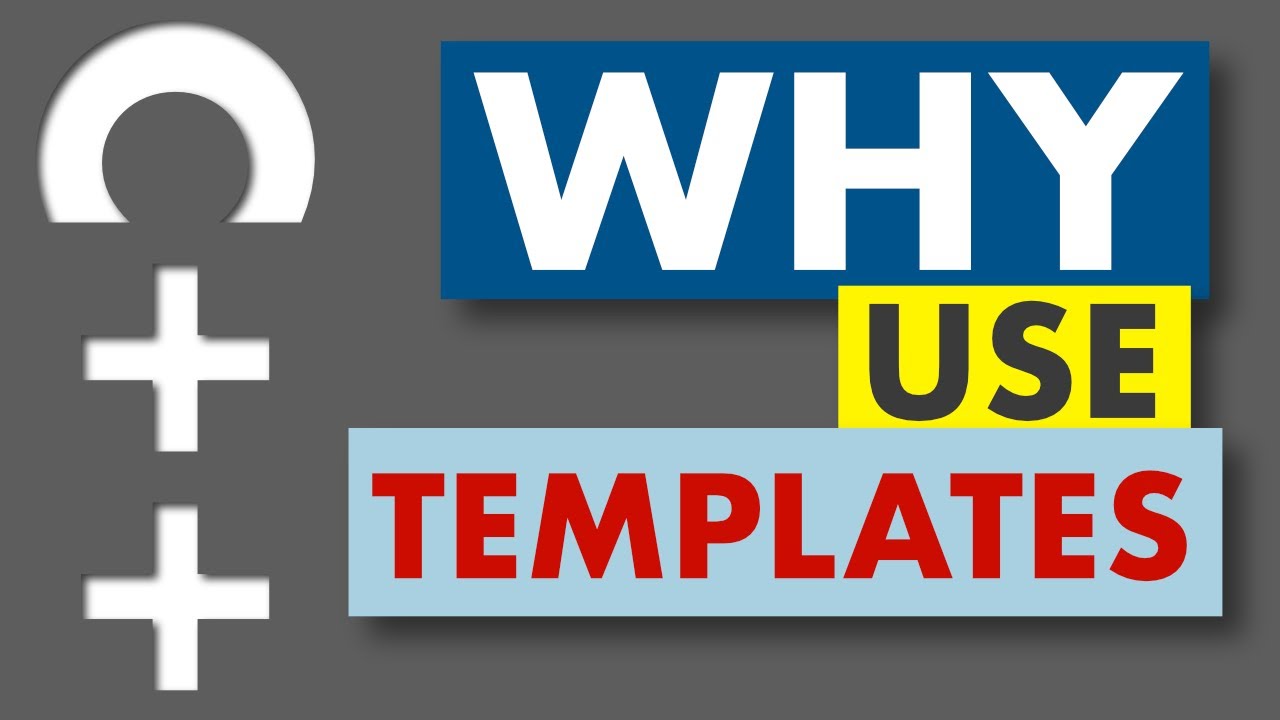](https://youtu.be/1Mrt1NM3KnI)[Lecture script](lectures/templates_why.md)
- Templates provide abstraction and separation of concerns
- Function templates
- Class and struct templates
- Generic algorithms and design patterns
- Zero runtime cost (almost)
- Compile-time meta-programming
- Summary----------------------------------------------------------
Templates: what do they do under the hood?
----------------------------------------------------------
[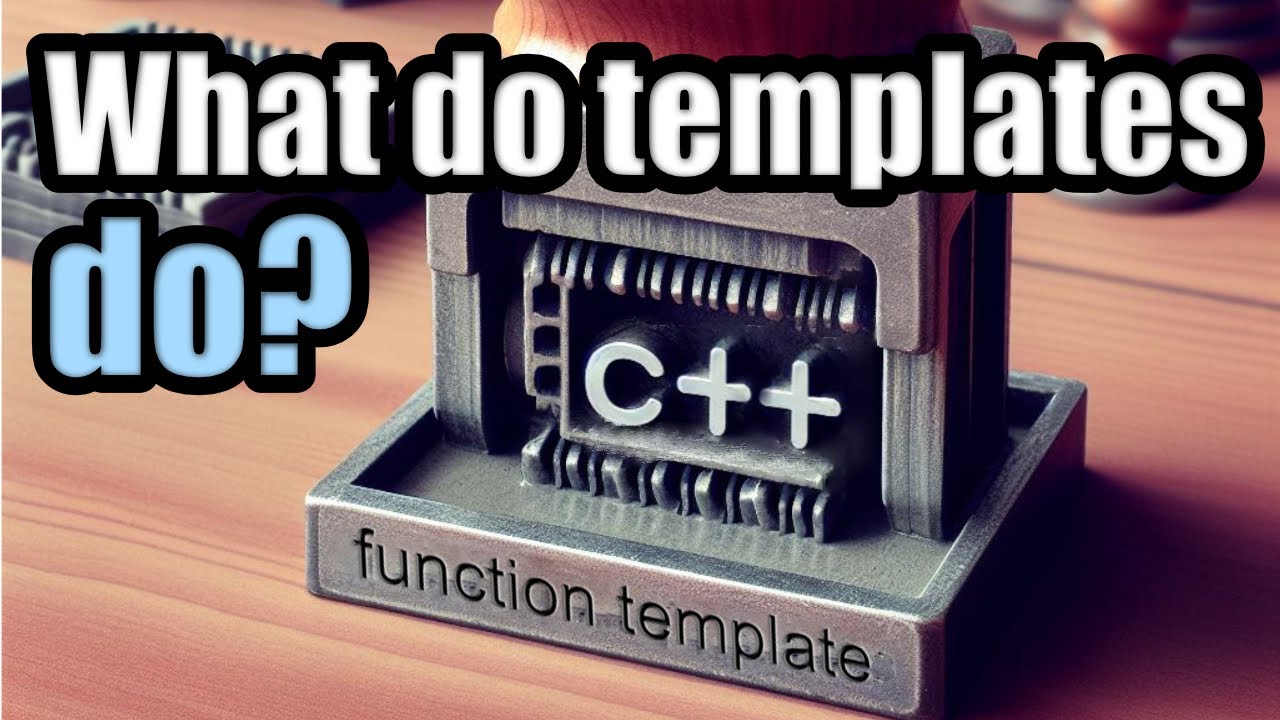](https://youtu.be/NKvEbPVllRE)[Lecture script](lectures/templates_what.md)
- Compilation process recap
- Compiler uses templates to generate code
- Hands-on example
- Compiler is lazy----------------------------------------------------------
How to write function templates
----------------------------------------------------------
[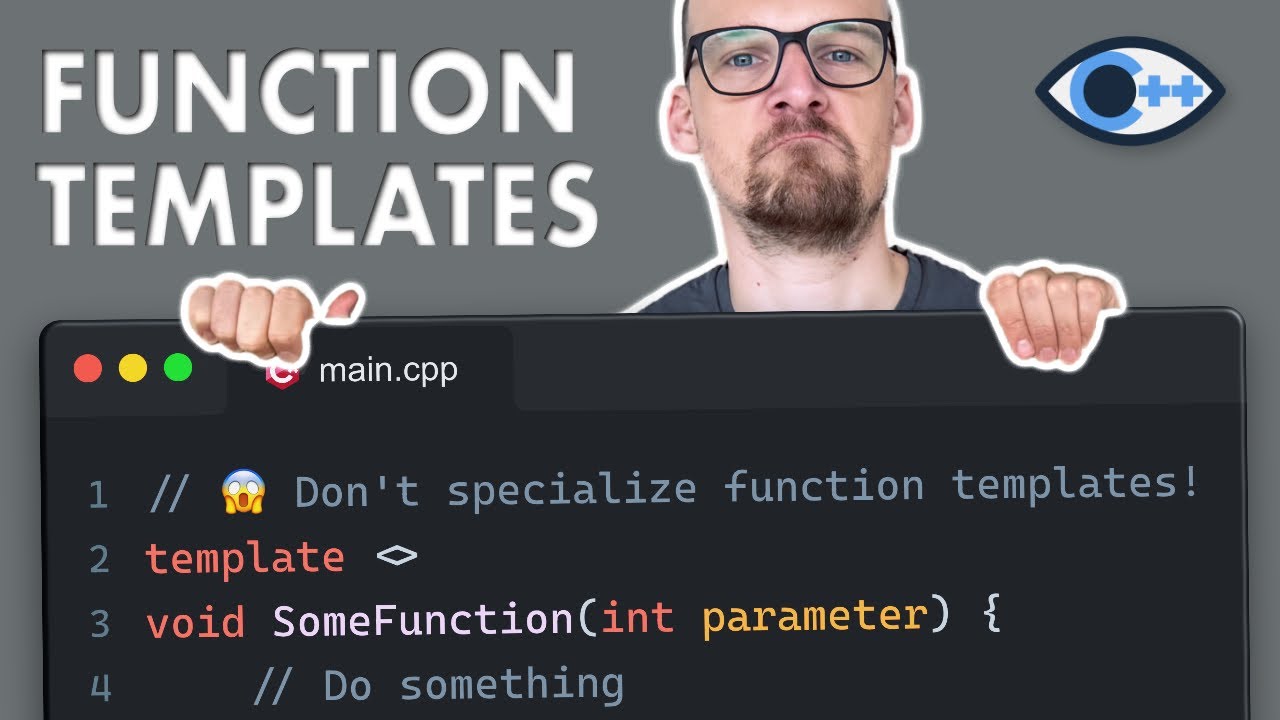](https://youtu.be/BZ626ZWPspc)[Lecture script](lectures/templates_how_functions.md)
- The basics of writing a function template
- Explicit template parameters
- Implicit template parameters
- Using both explicit and implicit template parameters at the same time
- Function overloading and templates
- Full function template specialization and why function overloading is better----------------------------------------------------------
How to write class templates
----------------------------------------------------------
[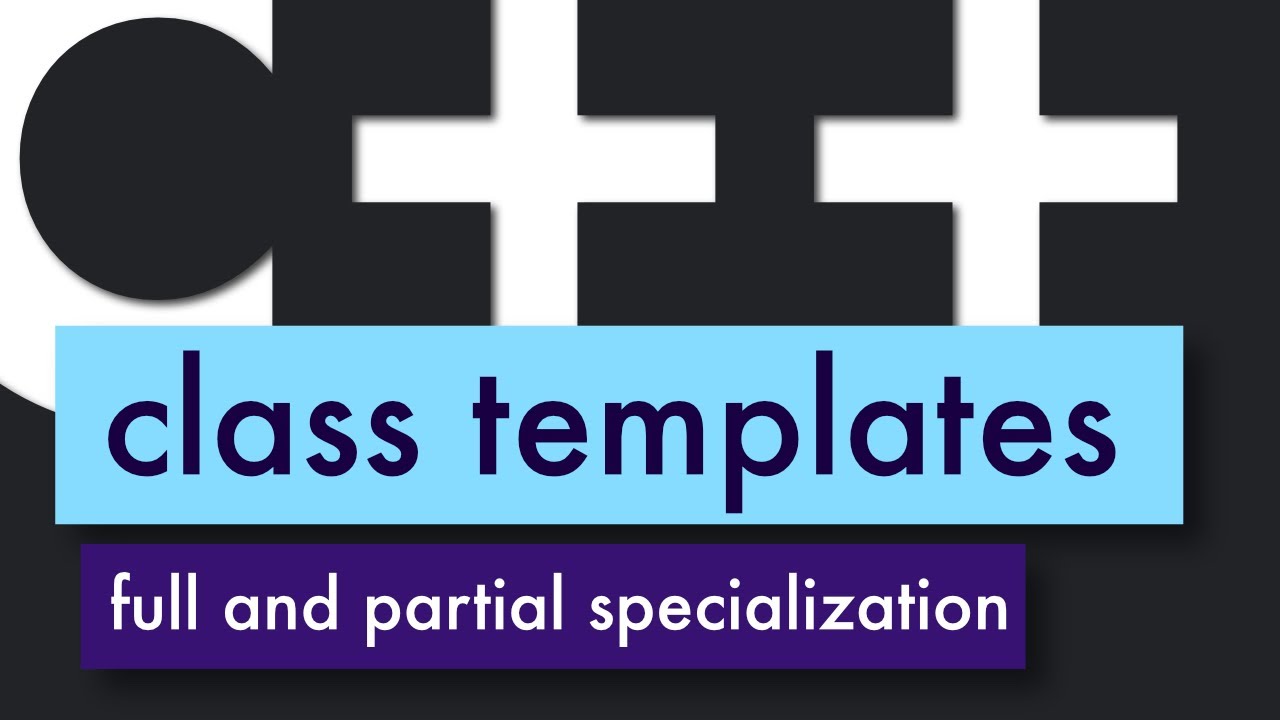](https://youtu.be/IQ62tA51Vag)- [How to use templates with classes in C++](lectures/templates_how_classes.md#how-to-use-templates-with-classes-in-c)
- [Class method templates](lectures/templates_how_classes.md#class-method-templates)
- [Prefer overloading to specialization of class method templates](lectures/templates_how_classes.md#prefer-overloading-to-specialization-of-class-method-templates)
- [Sometimes overloading is not possible --- specialize in this case](lectures/templates_how_classes.md#sometimes-overloading-is-not-possible-----specialize-in-this-case)
- [Class templates](lectures/templates_how_classes.md#class-templates)
- [Class template argument deduction (min. C++17)](lectures/templates_how_classes.md#class-template-argument-deduction-min-c17)
- [Class template specialization: implicit and explicit](lectures/templates_how_classes.md#class-template-specialization-implicit-and-explicit)
- [Full explicit template specialization](lectures/templates_how_classes.md#full-explicit-template-specialization)
- [How to fully specialize class templates](lectures/templates_how_classes.md#how-to-fully-specialize-class-templates)
- [Make sure a specialization follows the expected interface](lectures/templates_how_classes.md#make-sure-a-specialization-follows-the-expected-interface)
- [Historical reference for `std::vector`](lectures/templates_how_classes.md#historical-reference-for-stdvectorbool)
- [Specialize just one method of a class](lectures/templates_how_classes.md#specialize-just-one-method-of-a-class)
- [Specialize method templates of class templates](lectures/templates_how_classes.md#specialize-method-templates-of-class-templates)
- [Type traits and how to implement them using template specialization](lectures/templates_how_classes.md#type-traits-and-how-to-implement-them-using-template-specialization)
- [More generic traits using partial specialization](lectures/templates_how_classes.md#more-generic-traits-using-partial-specialization)
- [Difference between partial and full specializations](lectures/templates_how_classes.md#difference-between-partial-and-full-specializations)
- [How to tell partial template specialization apart from a new template class definition?](lectures/templates_how_classes.md#how-to-tell-partial-template-specialization-apart-from-a-new-template-class-definition)
- [How to tell a partial template specialization apart from a full class template specialization?](lectures/templates_how_classes.md#how-to-tell-a-partial-template-specialization-apart-from-a-full-class-template-specialization)
- [Partial template specialization with more types](lectures/templates_how_classes.md#partial-template-specialization-with-more-types)
- [Summary](lectures/templates_how_classes.md#summary)----------------------------------------------------------
Forwarding references
----------------------------------------------------------
[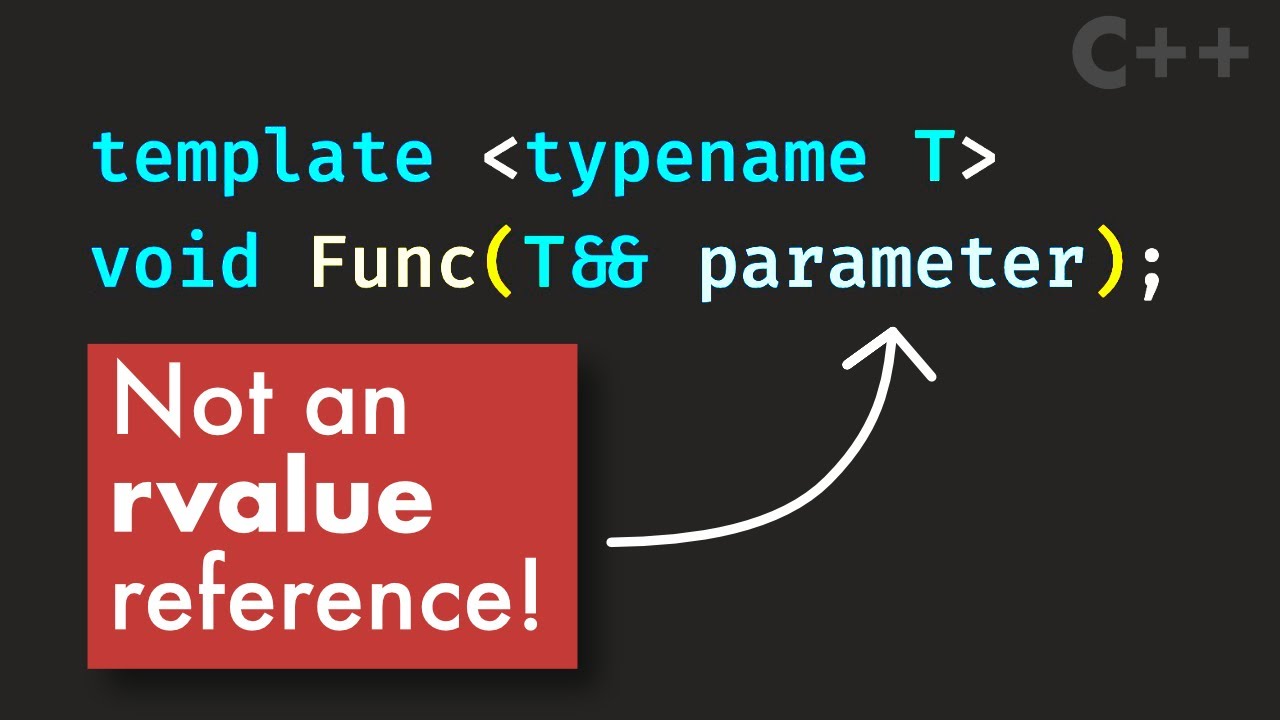](https://youtu.be/RW9KnqszYj4)- [The forwarding reference](lectures/forwarding_references.md#the-forwarding-reference)
- [Why use forwarding references](lectures/forwarding_references.md#why-use-forwarding-references)
- [Example setup](lectures/forwarding_references.md#example-setup)
- [How forwarding references simplify things](lectures/forwarding_references.md#how-forwarding-references-simplify-things)
- [When to prefer forwarding references](lectures/forwarding_references.md#when-to-prefer-forwarding-references)
- [How forwarding references work](lectures/forwarding_references.md#how-forwarding-references-work)
- [Reference collapsing](lectures/forwarding_references.md#reference-collapsing)
- [Remove reference using `std::remove_reference_t`](lectures/forwarding_references.md#remove-reference-using-stdremove_reference_t)
- [How `std::forward` works](lectures/forwarding_references.md#how-stdforward-works)
- [Passing an lvalue](lectures/forwarding_references.md#passing-an-lvalue)
- [Passing by rvalue](lectures/forwarding_references.md#passing-by-rvalue)
- [Summary](lectures/forwarding_references.md#summary)----------------------------------------------------------
Header and source files for templated code
----------------------------------------------------------
[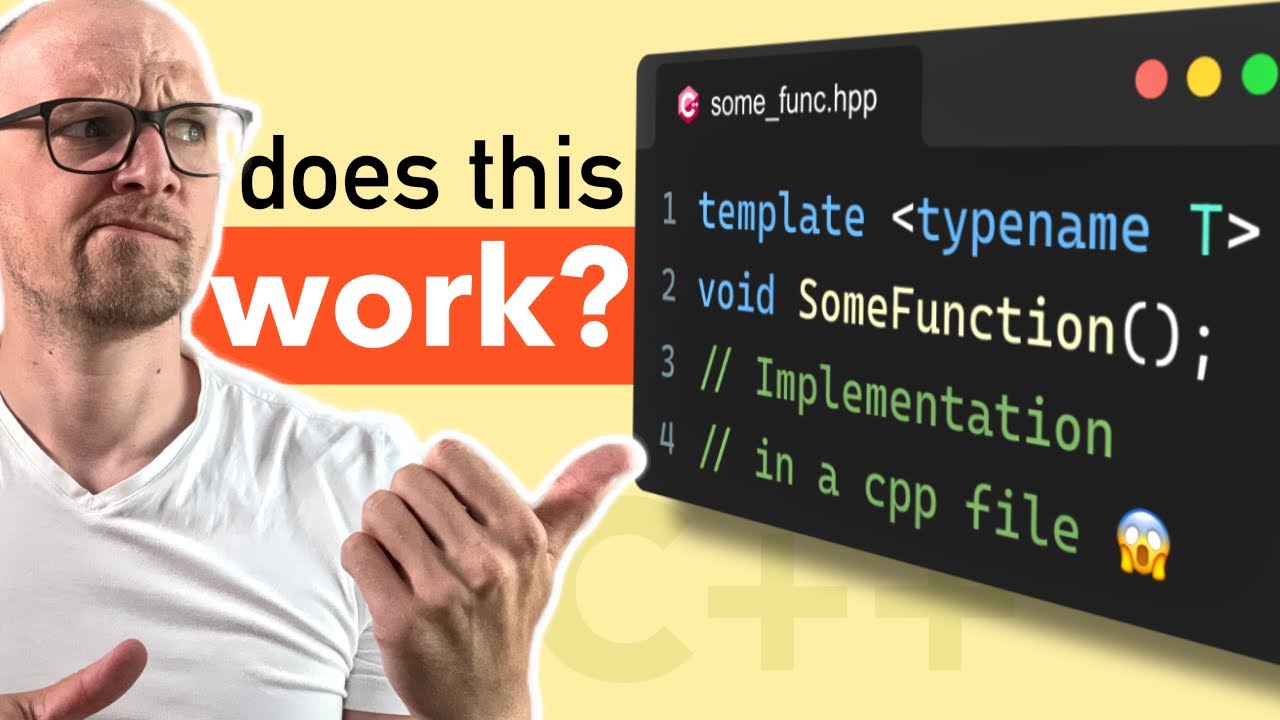](https://youtu.be/vjsr18XXMMQ)- [Why linker fails](lectures/templates_and_headers.md#why-linker-fails)
- [Compilation process for single `main.cpp` file](lectures/templates_and_headers.md#compilation-process-for-single-maincpp-file)
- [Compilation process for multiple files](lectures/templates_and_headers.md#compilation-process-for-multiple-files)
- [How to fix the linker error](lectures/templates_and_headers.md#how-to-fix-the-linker-error)
- [More complex explicit instantiations](lectures/templates_and_headers.md#more-complex-explicit-instantiations)
- [Summary](lectures/templates_and_headers.md#summary)----------------------------------------------------------
Almost everything about inheritance
----------------------------------------------------------
[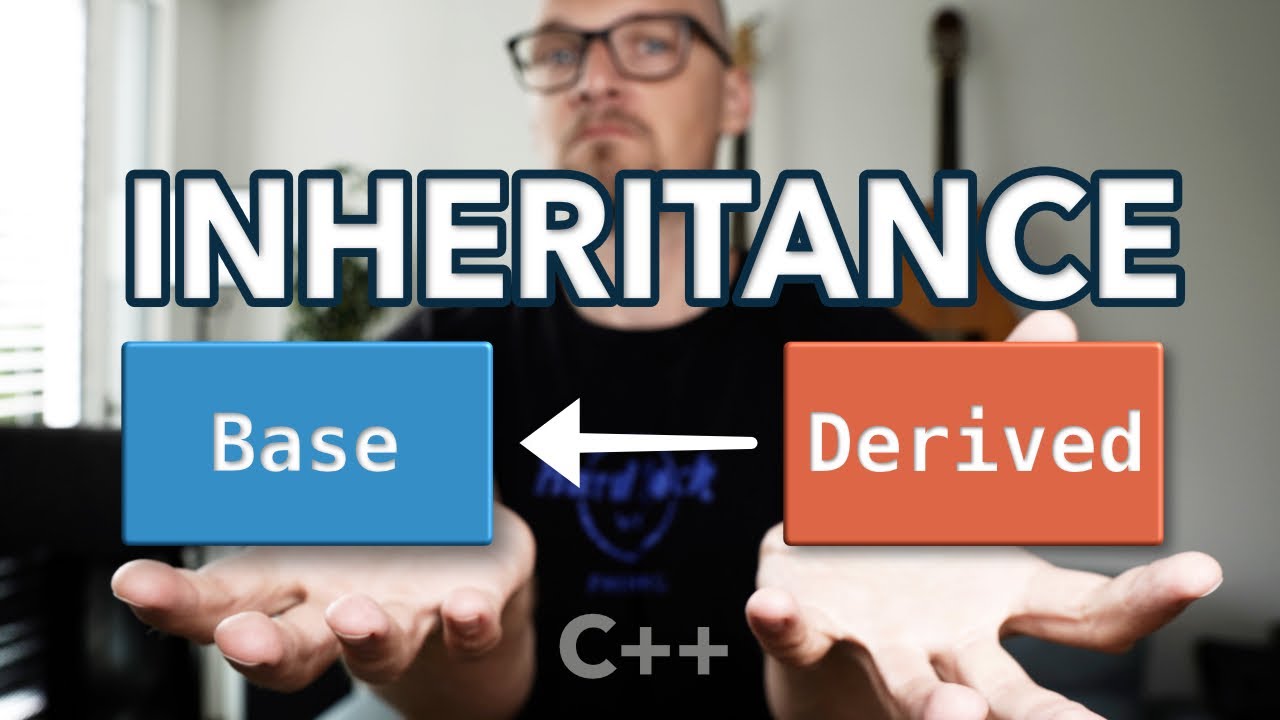](https://youtu.be/oUALDqvCbWs)- [Inheritance enables dependency inversion](lectures/inheritance.md#inheritance-enables-dependency-inversion)
- [The idea behind dependency inversion](lectures/inheritance.md#the-idea-behind-dependency-inversion)
- [Similarity to static polymorphism with templates](lectures/inheritance.md#similarity-to-static-polymorphism-with-templates)
- [How inheritance looks in C++](lectures/inheritance.md#how-inheritance-looks-in-c)
- [Implementation inheritance](lectures/inheritance.md#implementation-inheritance)
- [Access control with inheritance](lectures/inheritance.md#access-control-with-inheritance)
- [Implicit upcasting](lectures/inheritance.md#implicit-upcasting)
- [Real-world example of implementation inheritance](lectures/inheritance.md#real-world-example-of-implementation-inheritance)
- [Using `virtual` for interface inheritance and proper polymorphism](lectures/inheritance.md#using-virtual-for-interface-inheritance-and-proper-polymorphism)
- [How interface inheritance works](lectures/inheritance.md#how-interface-inheritance-works)
- [Runtime and memory overhead of using virtual](lectures/inheritance.md#runtime-and-memory-overhead-of-using-virtual)
- [Things to know about classes with `virtual` methods](lectures/inheritance.md#things-to-know-about-classes-with-virtual-methods)
- [A `virtual` destructor](lectures/inheritance.md#a-virtual-destructor)
- [Delete other special methods for polymorphic classes](lectures/inheritance.md#delete-other-special-methods-for-polymorphic-classes)
- [Downcasting using the `dynamic_cast`](lectures/inheritance.md#downcasting-using-the-dynamic_cast)
- [Don't mix implementation and interface inheritance](lectures/inheritance.md#dont-mix-implementation-and-interface-inheritance)
- [Implement pure interfaces](lectures/inheritance.md#implement-pure-interfaces)
- [Keyword `final`](lectures/inheritance.md#keyword-final)
- [Simple polymorphic class example following best practices](lectures/inheritance.md#simple-polymorphic-class-example-following-best-practices)
- [Multiple inheritance](lectures/inheritance.md#multiple-inheritance)
- [Detailed `Image` example following best practices](lectures/inheritance.md#detailed-image-example-following-best-practices)----------------------------------------------------------
Memory management and smart pointers
----------------------------------------------------------
[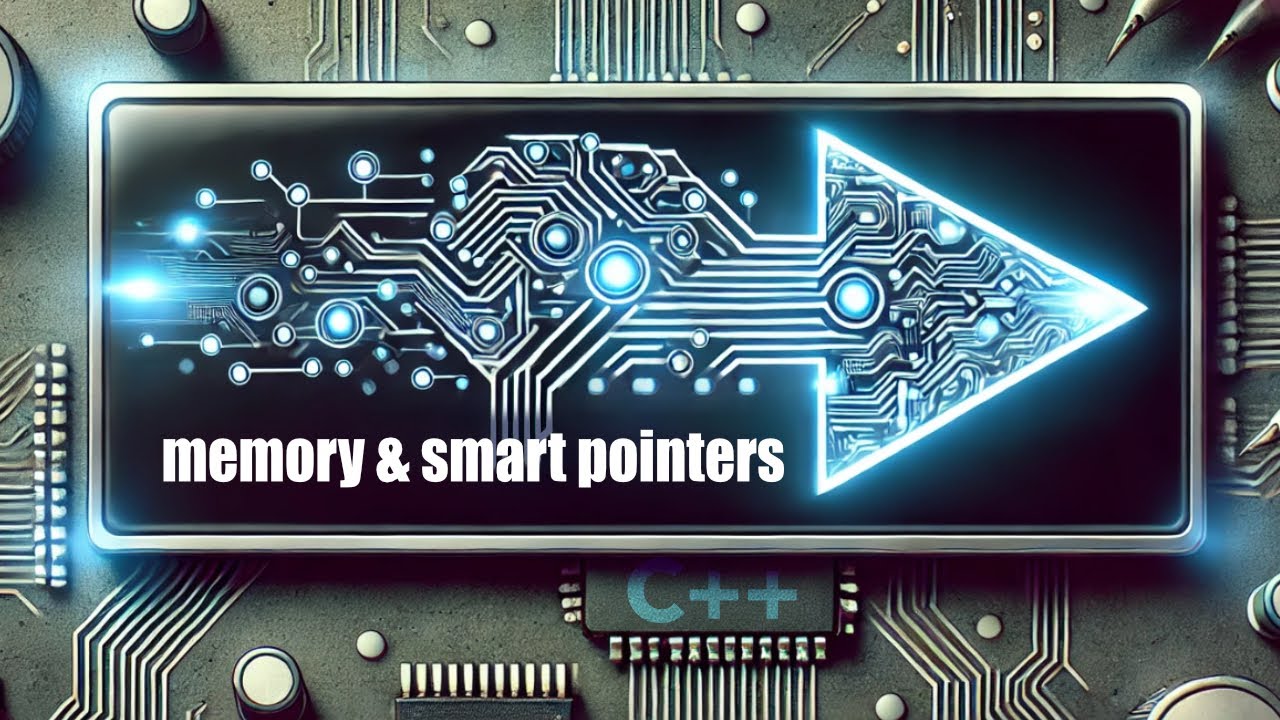](https://youtu.be/eHcdTytDZrI)- [Memory management and smart pointers](lectures/memory_and_smart_pointers.md#memory-management-and-smart-pointers)
- [Memory management in C++](lectures/memory_and_smart_pointers.md#memory-management-in-c)
- [Automatic memory management in other programming languages](lectures/memory_and_smart_pointers.md#automatic-memory-management-in-other-programming-languages)
- [The C++ way](lectures/memory_and_smart_pointers.md#the-c-way)
- [Memory allocation under the hood](lectures/memory_and_smart_pointers.md#memory-allocation-under-the-hood)
- [The stack](lectures/memory_and_smart_pointers.md#the-stack)
- [Why not keep persistent data on the stack](lectures/memory_and_smart_pointers.md#why-not-keep-persistent-data-on-the-stack)
- [The heap](lectures/memory_and_smart_pointers.md#the-heap)
- [Operators `new` and `delete`](lectures/memory_and_smart_pointers.md#operators-new-and-delete)
- [Typical pitfalls with data allocated on the heap](lectures/memory_and_smart_pointers.md#typical-pitfalls-with-data-allocated-on-the-heap)
- [Forgetting to call `delete`](lectures/memory_and_smart_pointers.md#forgetting-to-call-delete)
- [Performing shallow copy by mistake](lectures/memory_and_smart_pointers.md#performing-shallow-copy-by-mistake)
- [Performing shallow assignment by mistake](lectures/memory_and_smart_pointers.md#performing-shallow-assignment-by-mistake)
- [Calling a wrong `delete`](lectures/memory_and_smart_pointers.md#calling-a-wrong-delete)
- [Returning owning pointers from functions](lectures/memory_and_smart_pointers.md#returning-owning-pointers-from-functions)
- [RAII for memory safety](lectures/memory_and_smart_pointers.md#raii-for-memory-safety)
- [STL classes use RAII](lectures/memory_and_smart_pointers.md#stl-classes-use-raii)
- [Smart pointers to the rescue!](lectures/memory_and_smart_pointers.md#smart-pointers-to-the-rescue)
- [`std::unique_ptr`](lectures/memory_and_smart_pointers.md#stdunique_ptr)
- [`std::shared_ptr`](lectures/memory_and_smart_pointers.md#stdshared_ptr)
- [Prefer `std::unique_ptr`](lectures/memory_and_smart_pointers.md#prefer-stdunique_ptr)
- [Smart pointers are polymorphic](lectures/memory_and_smart_pointers.md#smart-pointers-are-polymorphic)
- [Summary](lectures/memory_and_smart_pointers.md#summary)----------------------------------------------------------
Lambdas in modern C++
----------------------------------------------------------
[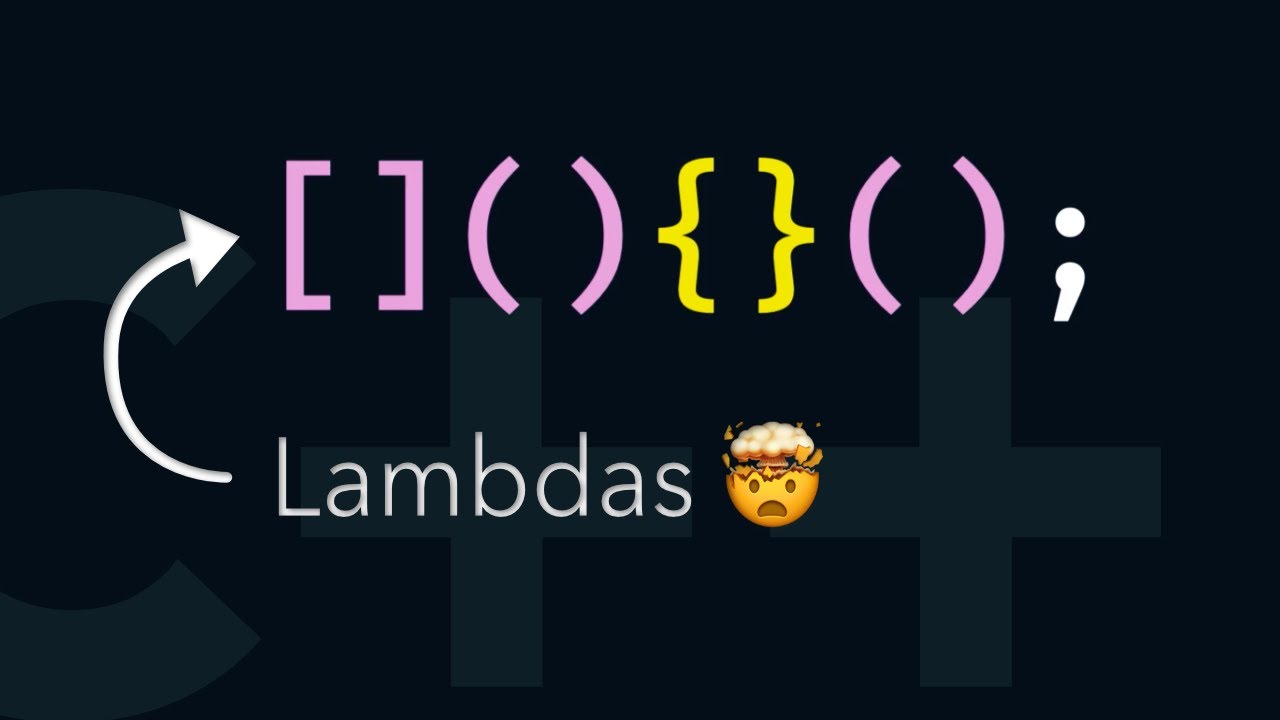](https://youtu.be/l0BgadhkUL8)- [Lambdas](lectures/lambdas.md#lambdas)
- [Overview](lectures/lambdas.md#overview)
- [What is a "callable"](lectures/lambdas.md#what-is-a-callable)
- [A function pointer is sometimes enough](lectures/lambdas.md#a-function-pointer-is-sometimes-enough)
- [Before lambdas we had function objects (or functors)](lectures/lambdas.md#before-lambdas-we-had-function-objects-or-functors)
- [How to implement generic algorithms like `std::sort`](lectures/lambdas.md#how-to-implement-generic-algorithms-like-stdsort)
- [Enter lambdas](lectures/lambdas.md#enter-lambdas)
- [Lambda syntax](lectures/lambdas.md#lambda-syntax)
- [When to use lambdas](lectures/lambdas.md#when-to-use-lambdas)
- [Summary](lectures/lambdas.md#summary)----------------------------------------------------------
## PS
### Most of the code snippets are validated automatically
If you **do** find an error in some of those, please open an issue in this repo!