Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/cr0hn/aiohttp-cache
A cache system for aiohttp server
https://github.com/cr0hn/aiohttp-cache
aiohttp cache memcached redis
Last synced: about 1 month ago
JSON representation
A cache system for aiohttp server
- Host: GitHub
- URL: https://github.com/cr0hn/aiohttp-cache
- Owner: cr0hn
- License: other
- Created: 2016-11-10T23:41:29.000Z (about 8 years ago)
- Default Branch: master
- Last Pushed: 2024-04-12T02:08:17.000Z (10 months ago)
- Last Synced: 2024-12-04T04:08:14.212Z (about 2 months ago)
- Topics: aiohttp, cache, memcached, redis
- Language: Python
- Homepage: https://aiohttp-cache.readthedocs.org/
- Size: 635 KB
- Stars: 44
- Watchers: 7
- Forks: 13
- Open Issues: 6
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
README
# Aiohttp-cache
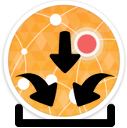
# What's aiohttp-cache
`aiohttp-cache` is a plugin for aiohttp.web server that allow to use a
cache system to improve the performance of your site.# How to use it
## With in-memory backend
```python
import asynciofrom aiohttp import web
from aiohttp_cache import ( # noqa
setup_cache,
cache,
)PAYLOAD = {"hello": "aiohttp_cache"}
WAIT_TIME = 2@cache()
async def some_long_running_view(
request: web.Request,
) -> web.Response:
await asyncio.sleep(WAIT_TIME)
payload = await request.json()
return web.json_response(payload)app = web.Application()
setup_cache(app)
app.router.add_post("/", some_long_running_view)web.run_app(app)
```## With redis backend
**Note**: redis should be available at
`$CACHE_URL` env variable or`redis://localhost:6379/0````python
import asyncioimport yarl
from aiohttp import web
from envparse import envfrom aiohttp_cache import ( # noqa
setup_cache,
cache,
RedisConfig,
)PAYLOAD = {"hello": "aiohttp_cache"}
WAIT_TIME = 2@cache()
async def some_long_running_view(
request: web.Request,
) -> web.Response:
await asyncio.sleep(WAIT_TIME)
payload = await request.json()
return web.json_response(payload)app = web.Application()
url = yarl.URL(
env.str("CACHE_URL", default="redis://localhost:6379/0")
)
setup_cache(
app,
cache_type="redis",
backend_config=RedisConfig(
db=int(url.path[1:]), host=url.host, port=url.port
),
)app.router.add_post("/", some_long_running_view)
web.run_app(app)
```## Example with a custom cache key
Let's say you would like to cache the requests just by the method and
json payload, then you can setup this as per the follwing example.**Note** default key_pattern is:
```python
DEFAULT_KEY_PATTERN = (
AvailableKeys.method,
AvailableKeys.host,
AvailableKeys.path,
AvailableKeys.postdata,
AvailableKeys.ctype,
)
``````python
import asynciofrom aiohttp import web
from aiohttp_cache import (
setup_cache,
cache,
AvailableKeys,
) # noqaPAYLOAD = {"hello": "aiohttp_cache"}
WAIT_TIME = 2@cache()
async def some_long_running_view(
request: web.Request,
) -> web.Response:
await asyncio.sleep(WAIT_TIME)
payload = await request.json()
return web.json_response(payload)custom_cache_key = (AvailableKeys.method, AvailableKeys.json)
app = web.Application()
setup_cache(app, key_pattern=custom_cache_key)
app.router.add_post("/", some_long_running_view)web.run_app(app)
```## Parametrize the cache decorator
```python
import asynciofrom aiohttp import web
from aiohttp_cache import ( # noqa
setup_cache,
cache,
)PAYLOAD = {"hello": "aiohttp_cache"}
WAIT_TIME = 2@cache(
expires=1 * 24 * 3600, # in seconds
unless=False, # anything what returns a bool. if True - skips cache
)
async def some_long_running_view(
request: web.Request,
) -> web.Response:
await asyncio.sleep(WAIT_TIME)
payload = await request.json()
return web.json_response(payload)app = web.Application()
setup_cache(app)
app.router.add_post("/", some_long_running_view)web.run_app(app)
```# License
This project is released under BSD license. Feel free
# Source Code
The latest developer version is available in a github repository:
# Development environment
1. docker-compose run tests