Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/darkghosthunter/rututils
A complete library for creating, manipulating and generating chilean RUTs or RUNs.
https://github.com/darkghosthunter/rututils
chile chilean chilean-id chilean-rut chilean-rut-utils composer php run rut user-id user-identification user-identity
Last synced: 2 months ago
JSON representation
A complete library for creating, manipulating and generating chilean RUTs or RUNs.
- Host: GitHub
- URL: https://github.com/darkghosthunter/rututils
- Owner: DarkGhostHunter
- License: mit
- Created: 2018-12-21T04:07:12.000Z (about 6 years ago)
- Default Branch: master
- Last Pushed: 2024-10-07T09:21:24.000Z (3 months ago)
- Last Synced: 2024-10-08T20:41:43.037Z (3 months ago)
- Topics: chile, chilean, chilean-id, chilean-rut, chilean-rut-utils, composer, php, run, rut, user-id, user-identification, user-identity
- Language: PHP
- Size: 96.7 KB
- Stars: 3
- Watchers: 1
- Forks: 0
- Open Issues: 1
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
- License: LICENSE
Awesome Lists containing this project
README
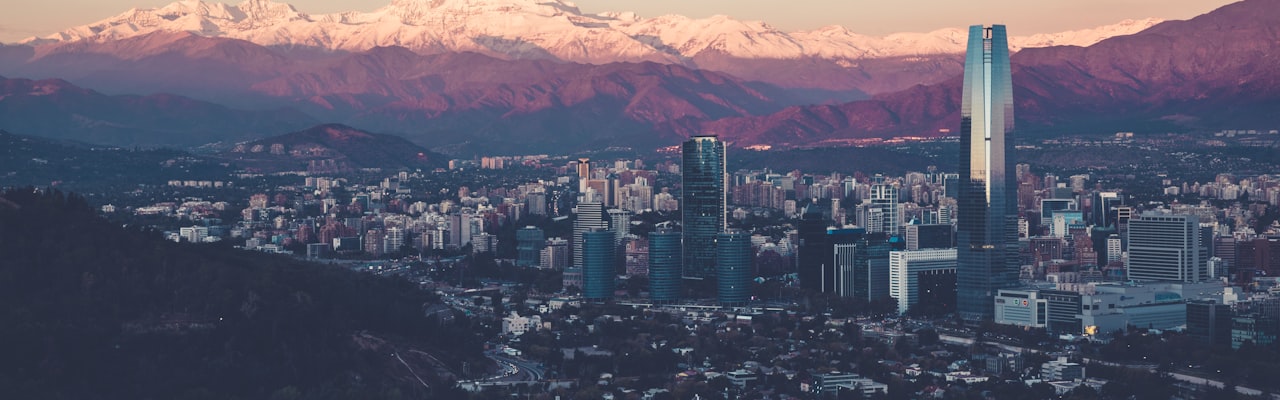
[](https://packagist.org/packages/darkghosthunter/rut-utils) [](https://packagist.org/packages/darkghosthunter/rut-utils)
 [](https://coveralls.io/github/DarkGhostHunter/RutUtils?branch=master) [](https://codeclimate.com/github/DarkGhostHunter/RutUtils/maintainability)# RUT Utilities
A complete library for creating, manipulating and generating chilean RUTs or RUNs.This package allows you to:
- **Create** a RUT object to conveniently hold the RUT information.
- **Validate**, clean and rectify RUTs.
- **Generate** random RUTs in a flexible manner.While this package works as a fire-and-forget utility for your project, ensure you read the documentation so you don't repeat yourself.
## Requirements
This package only needs PHP 7.3 and later.
It may work on older versions, but it will only support active PHP releases.
> Optional: Know what *el weón weón weón* means.
## Installation
Just fire Composer and require it into your project:
```bash
composer require darkghosthunter/rut-utils
```If you don't have Composer in your project, ~~you should be ashamed~~ just [install it](https://getcomposer.org/download/) .
## Usage
* [What is a RUT or RUN?](#what-is-a-rut-or-run)
* [Creating a RUT](#creating-a-rut)
* [Retrieving a RUT](#retrieving-a-rut)
* [Generating RUTs](#generating-ruts)
* [Helpers](#helpers)
* [Make Callbacks](#make-callbacks)
* [Serialization](#serialization)
* [Global helper](#global-helper)### What is a RUT or RUN?
A RUT (or RUN for people) is a string of numbers which identify a person or company. They're unique for each one, and they're never re-assigned to an individual, so the registry of RUTs is always growing.
The RUT its comprised of a random Number, like `18.765.432`, and a Verification Digit, which is the result of a mathematical algorithm [(Modulo 11)](https://www.google.com/search?q=modulo+11+algorithm) over that number. This Verification Digit vary between `0` and `9`, or a `K`. In the end. you get this:
```
18.765.432-1
```This identification information is a safe bet for chilean companies. It allows to attach one account to one individual (person or company), and can be cross-referenced with other data the user may have available through other services.
> What's the difference between RUTs and RUNs? RUT are meant for identifying a person or company taxes, RUNs are to identify single persons. For both cases, **they're practically the same**.
### Creating a RUT
There are two ways to create a RUT: manual instancing, which is strict, and using the `make()` static helper.
#### Manual instancing
Using manual instantiation allows you to create a RUT by the given number and verification digit quickly. For example, with data coming from a Database or any other trustful source.
```php
num; // 14328145
echo $rut->vd; // 0
```> For safety reasons, you cannot set the `num` and `vd` in the instance.
#### Lowercase or Uppercase `K`
A RUT can have the `K` character as verification *digit*. The Rut object doesn't discerns between lowercase `k` or uppercase `K` when creating one, but **it always stores uppercase as default**.
You can change this behaviour for all Rut instances using the `allUppercase()` or `allLowercase()` methods:
```php
vd; // "k"Rut::allUppercase();
echo Rut::make('12343580', 'K')->vd; // "K"
```Additionally, you can change this configuration for a single instance by using `uppercase()` and `lowercase()`.
```php
lowercase();echo $rut->vd; // "K"
$rut->uppercase();
echo $rut->vd; // "k"
```This may come in handy when your source of truth manages lowercase `k` and you need strict comparison for storing, or normalize it.
### Generating RUTs
Sometimes it's handy to create a RUT on the fly, usually for testing purposes when seeding and mocking.
You can do that using the `RutGenerator` class and use the methods to build how you want to generate your RUTs. The methods are fluent, meaning, you can chain them until you use the `generate()` method.
```php
generate();echo $rut; // "7.976.228-8"
```The default mode makes a RUT for normal people, which are bound between 1.000.000 and 50.000.000. You can use the `forCompany()` method, which will vary the result randomly between 50.000.000 and 100.000.000.
```php
asPerson()->generate();
// "15.846.327-K"echo $company = RutGenerator::make()->asCompany()->generate();
// "54.029.467-4"
```Of course one may be not enough. You can add a parameter to these methods with the number of RUTs you want to make. The result will be returned as an `array`.
```php
asPerson()->generate(10);
$companyRuts = RutGenerator::make()->asCompany()->generate(35);
```If for some reason you need them as raw strings instead of Rut instances, which is very good when generating thousands of them on strict memory usage, use the `asBasic()` and `asRaw()` method.
This will output the random strings like `22605071K`.
```php
asRaw()->generate(10);
$basic = RutGenerator::make()->asBasic()->generate(20);
$strict = RutGenerator::make()->asStrict()->generate(30);
```#### Generating random unique RUTs
If you need to create more than thousands of RUTs without the risk of having them duplicated, use the `withoutDuplicates()` method.
```php
withoutDuplicates()->generate(100000);
```##### Unique results between calls
You may have a custom seeder in your application that may call `generate()` every single time, increasing risk of collisions with each generation. Fear not! Using the `generateStatic()` you are guaranteed to get unique results during a single application lifecycle.
```php
'John'],
['name' => 'Clara'],
['name' => 'Mark'],
// ... and other 99.997 records
];$seeder = function ($user) {
return array_merge($user, [
'rut' => RutGenerator::make()->generateStatic()
]);
};// Call the seeder
foreach ($users as $key => $user) {
$users[$key] = $seeder($user);
}// Flush the static array for the next seeder call
RutGenerator::make()->flushStatic();
```### Helpers
You can manipulate and check strings quickly using the `RutHelper` class, which contains a wide variety of handy static methods you can use.
* [`cleanRut`](#cleanrut)
* [`separateRut`](#separaterut)
* [`validate`](#validate)
* [`validateStrict`](#validatestrict)
* [`filter`](#filter)
* [`rectify`](#rectify)
* [`isPerson`](#isperson)
* [`isCompany`](#iscompany)
* [`isEqual`](#isequal)
* [`getVd`](#getvd)#### `cleanRut`
Clears a RUT string from invalid characters. Additionally, you can set if you want the `K` verification character as uppercase or lowercase.
```php
18290743,
// 1 => 'k',
// ]
```#### `validate`
Checks if the RUTs issued are valid.
If there are more than one RUT, it will return `true` if all the RUTs are valid, and `false` if at least one is invalid.
```php
isValid(); // true
echo Rut::make('94.328.145-0')->isValid(); // false
echo Rut::make('cleanthis14328145-0-andthistoo')->isValid(); // true
```#### `validateStrict`
You can strictly validate a RUT. The RUT value being passed must be a string with thousand separator and hyphen preceding the RUT verification digit.
If there are more than one RUT, it will return `true` if all the RUTs are valid, and `false` if at least one is invalid.
```php
14328145-0
// [1] => 12343580-K
// }$rutsB = RutHelper::filter([
'14328145-0',
'12343580-K',
'94.328.145-0',
'not-a-rut'
]);var_dump($rutsB);
// array(1) {
// [0] => 14328145-0
// [1] => 12343580-K
// }
```
#### `rectify`Receives only the RUT number and returns a valid `Rut` instance with the corresponding verification digit.
```php
num; // "18765432"
echo $rut->vd; // "7"
```> If you pass down a whole RUT, you may get a new RUT with an appended Verification Digit. Ensure you pass down only the RUT number.
#### `isPerson`
Checks if the RUT below 50.000.000, which are usually used for normal people.
```php
isPerson(); // true
```#### `isCompany`
Checks if the RUT is over or equal 50.000.000, which are usually used for companies.
```php
isCompany(); // true
```#### `isEqual`
Returns if two or more RUTs are equal, independently of how these are formatted, **even if these are invalid**.
```php
isEqual('thisisARut12343580-K', '12343580-k'); // true
```#### `getVd`
Returns the verification digit for a given number.
```php
count($ruts),
]);
});$ruts = Rut::many([
// ...
]);var_dump($ruts);
// array(100) [
// // ...
// 'count' => 100,
// ]
```> If you register multiple callbacks, these will be executed in the order they were registered.
### Serialization
Sometimes you may want to store your Rut instance somewhere, or serialize it to JSON, or a string. With this package you're covered from all angles.
#### Serialize / Unserialize
By default, a Rut instance is serialized as a raw string, which is latter reconstructed quickly by just dividing the string into the number and verification digit:
```php
toStrictString(); // "22.605.071-K"
echo $rut->toBasicString(); // "22605071-K"
echo $rut->toRawString(); // "22605071K"
```#### JSON
By default, when casting to JSON, the result is a string. You can change this to be an array of the number and verification digit using static methods for all instances, or a per-instance case:
```php
jsonAsArray();echo json_encode($rut); // {"num":"22605071","vd":"K"}
$rut->jsonAsString();
echo json_encode($rut); // "22.605.071-K"
```## Global helper
In version 2.0, all helpers have been killed and now you have only one called `rut()`. It works as a proxy for `Rut::make`, but accepts a default in case of invalid ruts. If no parameter is issued, an instance of the Rut Generator is returned.
```php
generate();echo $rut; // '20.750.456-4'
```## License
This package is licenced by the [MIT License](LICENSE).