Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/darkroomengineering/tempus
Use only one requestAnimationFrame for your whole app
https://github.com/darkroomengineering/tempus
clock raf ticker time
Last synced: 4 days ago
JSON representation
Use only one requestAnimationFrame for your whole app
- Host: GitHub
- URL: https://github.com/darkroomengineering/tempus
- Owner: darkroomengineering
- License: mit
- Created: 2022-07-01T17:07:21.000Z (over 2 years ago)
- Default Branch: main
- Last Pushed: 2024-11-26T11:42:06.000Z (about 1 month ago)
- Last Synced: 2024-12-22T00:03:48.460Z (11 days ago)
- Topics: clock, raf, ticker, time
- Language: JavaScript
- Homepage:
- Size: 214 KB
- Stars: 166
- Watchers: 6
- Forks: 4
- Open Issues: 2
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
Awesome Lists containing this project
README
# Tempus
[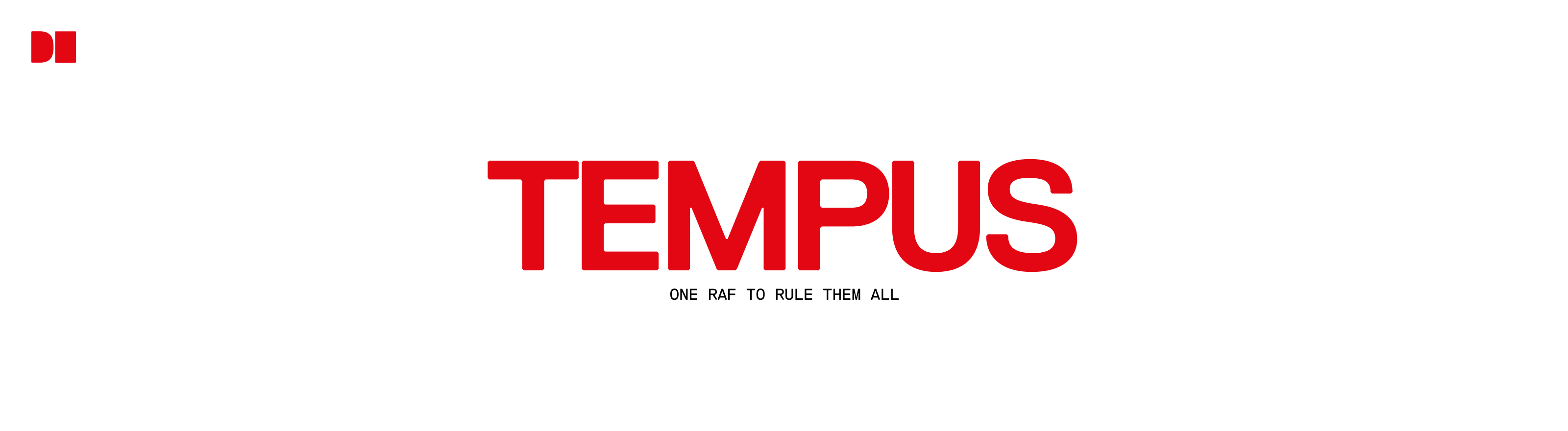](https://github.com/darkroomengineering/tempus)
A lightweight, high-performance animation frame manager for JavaScript applications.
## Features
- ð Merge multiple requestAnimationFrame loops for better performance
- ðŊ Control execution priority of different animations
- ⥠Support for custom frame rates (FPS)
- ð Compatible with popular animation libraries
- ðŠķ Zero dependencies
- ðĶ ~1KB gzipped## Installation
```bash
npm install tempus
```## Basic Usage
```javascript
import Tempus from tempus// Simple animation at maximum FPS
const animate = (time, deltaTime) => {
// Animation code here
}Tempus.add(animate)
```### Cleanup
```javascript
const unsubscribe = Tempus.add(animate)unsubscribe()
```
## Advanced Usage
### Custom Frame Rates
```javascript
// Run at specific FPS
Tempus.add(animate, {
fps: 30 // Will run at 30 FPS
})
```### Priority System
```javascript
// Higher priority (runs first)
Tempus.add(criticalAnimation, { priority: -1 })// Lower priority (runs after)
Tempus.add(secondaryAnimation)
```### Global RAF Patching
```javascript
// Patch native requestAnimationFrame
Tempus.patch()// Now regular requestAnimationFrame calls will use Tempus
requestAnimationFrame(animate, {
priority: 0,
fps: 60
})
```## Integration Examples
### With Lenis Smooth Scroll
```javascript
Tempus.add((time) => {
lenis.raf(time)
})
```### With GSAP
```javascript
// Remove GSAP's internal RAF
gsap.ticker.remove(gsap.updateRoot)// Add to Tempus
Tempus.add((time) => {
gsap.updateRoot(time / 1000)
})
```### With Three.js
```javascript
Tempus.add((time) => {
renderer.render(scene, camera)
}, { priority: 1 })
```## API Reference
### Tempus.add(callback, options)
Adds an animation callback to the loop.
- **callback**: `(time: number, deltaTime: number) => void`
- **options**:
- `priority`: `number` (default: 0) - Lower numbers run first
- `fps`: `number` (default: Infinity) - Target frame rate
- **Returns**: `() => void` - Unsubscribe function### Tempus.patch()
Patches the native `requestAnimationFrame` to use Tempus.
## Best Practices
- Use priorities wisely: critical animations (like scroll) should have higher priority
- Clean up animations when they're no longer needed
- Consider using specific FPS for non-critical animations to improve performance
- Always handle cleanup in component unmount/destroy methods## License
MIT ÂĐ [Darkroom Engineering](https://github.com/darkroomengineering)
# Shoutout
Thank you to [Keith Cirkel](https://github.com/keithamus) for having transfered us the npm package name ð.