https://github.com/devxoul/alertreactor
A ReactorKit extension for UIAlertController
https://github.com/devxoul/alertreactor
reactorkit uialertcontroller
Last synced: 8 days ago
JSON representation
A ReactorKit extension for UIAlertController
- Host: GitHub
- URL: https://github.com/devxoul/alertreactor
- Owner: devxoul
- License: mit
- Created: 2017-07-28T19:09:19.000Z (almost 8 years ago)
- Default Branch: master
- Last Pushed: 2019-06-15T06:10:10.000Z (about 6 years ago)
- Last Synced: 2025-03-25T04:51:59.026Z (4 months ago)
- Topics: reactorkit, uialertcontroller
- Language: Swift
- Homepage:
- Size: 34.2 KB
- Stars: 24
- Watchers: 2
- Forks: 2
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# AlertReactor

[](https://cocoapods.org/pods/AlertReactor)
[](https://travis-ci.org/devxoul/AlertReactor)
[](https://codecov.io/gh/devxoul/AlertReactor)AlertReactor is a ReactorKit extension for UIAlertController. It provides an elegant way to deal with an UIAlertController. Best fits if you have lazy-loaded alert actions.
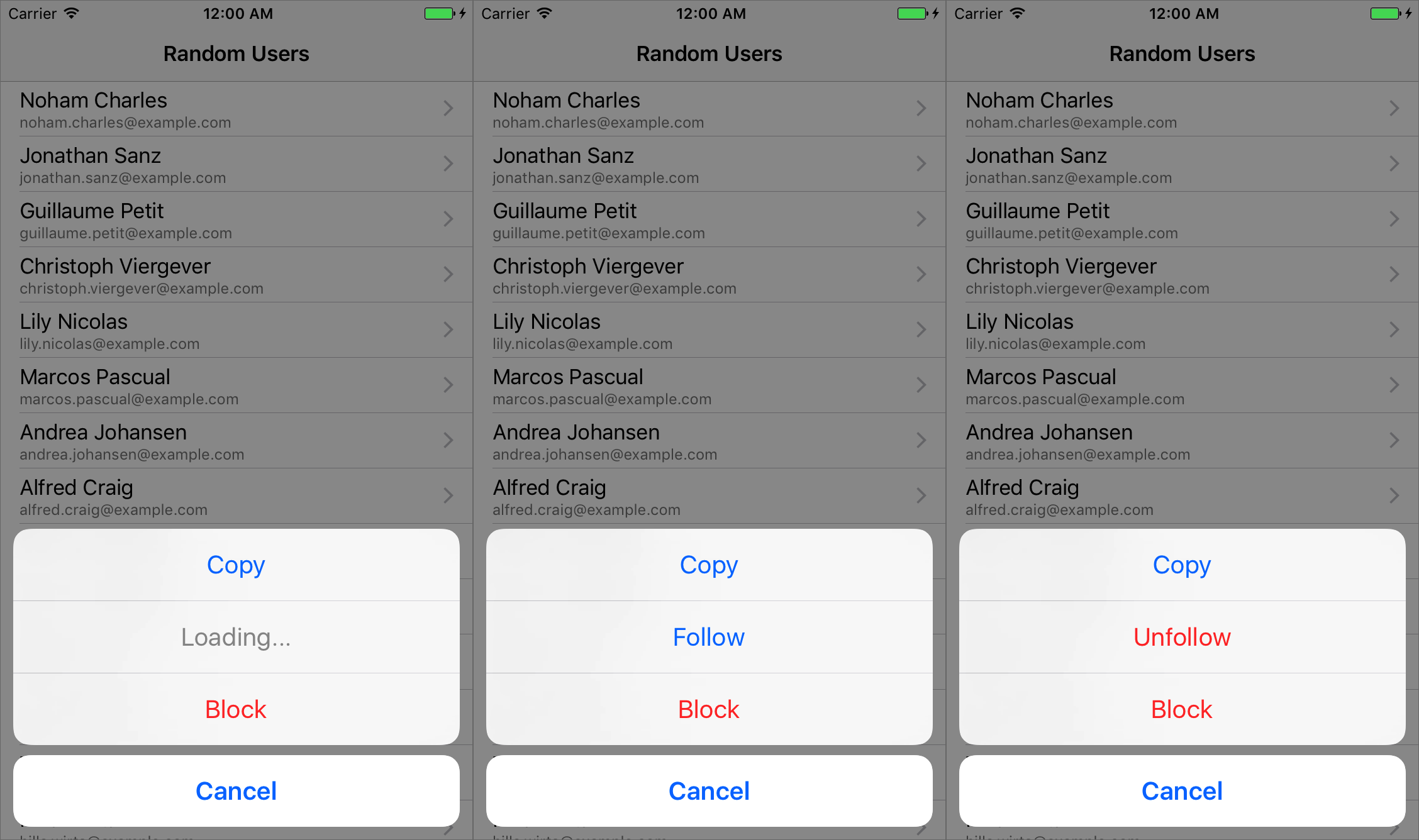
## Features
* ✏️ Statically typed alert actions
* 🕹 Reactive and dynamic action bindings## At a Glance
This is an example implementation of an `AlertReactor`:
```swift
final class UserAlertReactor: AlertReactor {
override func mutate(action: Action) -> Observable {
switch action {
case .prepare:
return .just(.setActions([.copy, .follow, .block, .cancel]))case let .selectAction(alertAction):
switch alertAction {
case .copy: return foo()
case .follow: return foo()
case .unfollow: return foo()
case .block: return foo()
case .cancel: return foo()
}
}
}
}
```## Getting Started
### 1. Defining an Alert Action
First you should define a new type which conforms to a protocol `AlertActionType`. This is an abstraction model of `UIAlertAction`. This protocol requires a `title`(required), `style`(optional) and `isEnabled`(optional) property.
```swift
enum UserAlertAction: AlertActionType {
case loading
case follow
case block
case cancel// required
var title: String {
switch self {
case .loading: return "Loading"
case .follow: return "Follow"
case .block: return "Block"
case .cancel: return "Cancel"
}
}// optional
var style: UIAlertActionStyle {
switch self {
case .loading: return .default
case .follow: return .default
case .block: return .destructive
case .cancel: return .cancel
}
}// optional
var isEnabled: Bool {
switch self {
case .loading: return false
default: return true
}
}
}
```### 2. Creating an Alert Reactor
`AlertReactor` is a generic reactor class. It takes a single generic type which conforms to a protocol `AlertActionType`. This reactor provides a default action, mutation and a state. Here is a simplified definition of `AlertReactor`. You may subclass this class and override `mutate(action:)` method to implement specific business logic.
```swift
class AlertReactor: Reactor {
enum Action {
case prepare // on viewDidLoad()
case selectAction(AlertAction) // on select action
}enum Mutation {
case setTitle(String?)
case setMessage(String?)
case setActions([AlertAction])
}struct State {
public var title: String?
public var message: String?
public var actions: [AlertAction]
}
}
```We're gonna use the `UserAlertAction` as a generic parameter to create a new subclass of `AlertReactor`.
```swift
final class UserAlertReactor: AlertReactor {
override func mutate(action: Action) -> Observable {
switch action {
case .prepare:
return .just(Mutation.setActions([.copy, .follow, .block, .cancel]))case let .selectAction(alertAction):
switch alertAction {
case .loading: return .empty()
case .follow: return UserAPI.follow()
case .block: return UserAPI.block()
case .cancel: return .empty()
}
}
}
}
```### 3. Presenting an Alert Controller
`AlertController` is a subclass of `UIAlertController` which conforms to a protocol `View` from ReactorKit. This class also takes a single generic type of `AlertActionType`. You can initialize this class with some optional parameters: `reactor` and `preferredStyle`. Just present it then the reactor will handle the business logic for you.
```swift
let reactor = UserAlertReactor()
let controller = AlertController(reactor: reactor, preferredStyle: .actionSheet)
self.present(controller, animated: true, completion: nil)
```## Installation
```ruby
pod 'AlertReactor'
```## License
AlertReactor is under MIT license. See the [LICENSE](LICENSE) for more info.