Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/diezo/Ensta
🔥 Fast & Reliable Python Package For Instagram API - 2024
https://github.com/diezo/Ensta
api-wrapper ensta instabot instagram instagram-account instagram-api instagram-api-python instagram-automation instagram-bot instagram-client instagram-crawler instagram-downloader instagram-photos instagram-private-api instagram-scraper instagram-sdk instagram-stories instapy instsgram-feed reels
Last synced: 3 months ago
JSON representation
🔥 Fast & Reliable Python Package For Instagram API - 2024
- Host: GitHub
- URL: https://github.com/diezo/Ensta
- Owner: diezo
- License: mit
- Created: 2023-06-07T07:56:42.000Z (over 1 year ago)
- Default Branch: master
- Last Pushed: 2024-07-07T14:53:24.000Z (4 months ago)
- Last Synced: 2024-07-27T01:42:54.443Z (4 months ago)
- Topics: api-wrapper, ensta, instabot, instagram, instagram-account, instagram-api, instagram-api-python, instagram-automation, instagram-bot, instagram-client, instagram-crawler, instagram-downloader, instagram-photos, instagram-private-api, instagram-scraper, instagram-sdk, instagram-stories, instapy, instsgram-feed, reels
- Language: Python
- Homepage: https://bit.ly/ensta-discord
- Size: 1.16 MB
- Stars: 340
- Watchers: 9
- Forks: 36
- Open Issues: 14
-
Metadata Files:
- Readme: README.md
- Funding: .github/FUNDING.yml
- License: LICENSE.txt
- Code of conduct: CODE_OF_CONDUCT.md
- Security: SECURITY.md
Awesome Lists containing this project
README
# Ensta - Simple Instagram API
[](https://pypi.org/project/ensta)
[]()
[](https://pepy.tech/project/ensta)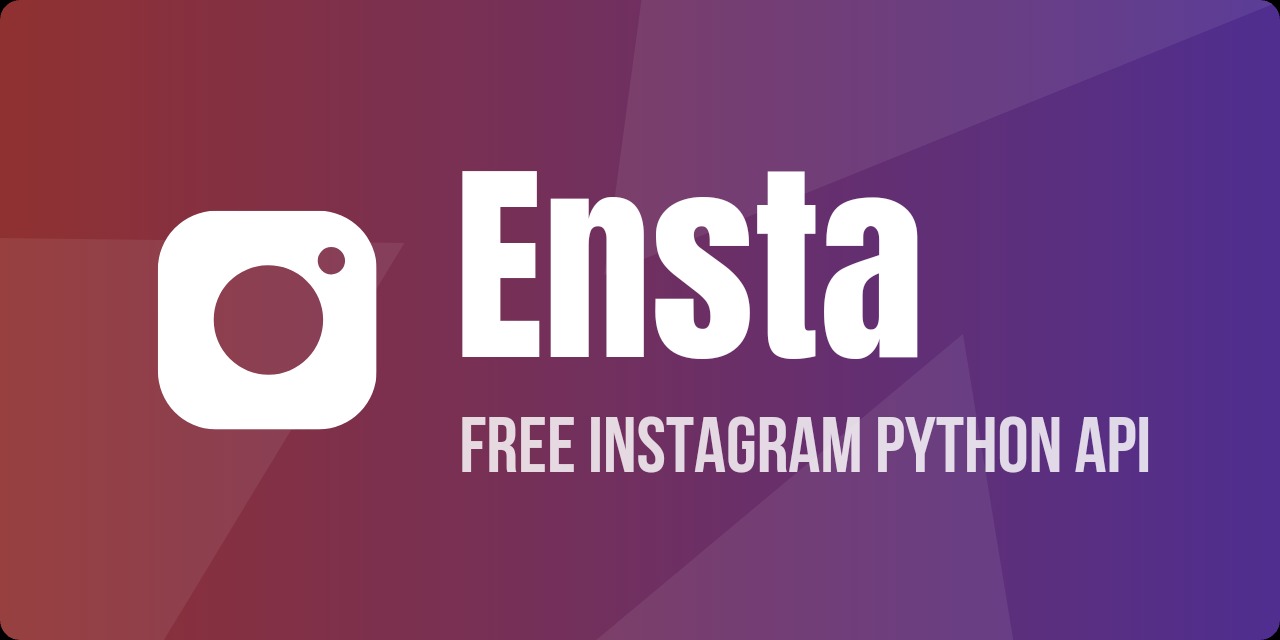
Ensta is a simple, reliable and up-to-date python package for Instagram API.
Both **authenticated** and **anonymous** requests are supported.
[
](https://buymeacoffee.com/sonii)
## Show Your Support! 🌟
If Ensta could add value to your projects, please do give this repo a star. Thanks!## Installation
Read the [**pre-requisites**](https://github.com/diezo/Ensta/wiki/Pre%E2%80%90requisites) here.pip install ensta
## Example
Fetching profile info by username:
```python
from ensta import Mobilemobile = Mobile(username, password)
profile = mobile.profile("leomessi")
print(profile.full_name)
print(profile.biography)
print(profile.profile_pic_url)
```## Features
These features use the **Mobile API**.Using Proxies
When to use a proxy:
- You're being rate limited.
- Ensta is not working because your Home IP is flagged.
- You're deploying Ensta to the cloud. (Instagram blocks requests from IPs of cloud providers, so a proxy must be used)```python
from ensta import Mobilemobile = Mobile(
username,
password,
proxy={
"http": "socks5://username:password@host:port",
"https": "socks5://username:password@host:port"
}
)
```Ensta uses the same proxy settings as the **requests** module.
Username-Password Login
Username is recommended to sign in. However, email can also be used.
```python
from ensta import Mobile# Recommended
mobile = Mobile(username, password)# This also works
mobile = Mobile(email, password)
```Change Profile Picture
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.change_profile_picture("image.jpg")
```Fetch Profile Information
```python
from ensta import Mobilemobile = Mobile(username, password)
profile = mobile.profile("leomessi")
print(profile.full_name)
print(profile.biography)
print(profile.follower_count)
```Follow/Unfollow Account
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.follow("leomessi")
mobile.unfollow("leomessi")
```Change Biography
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.change_biography("New bio here.")
```Switch to Private/Public Account
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.switch_to_private_account()
mobile.switch_to_public_account()
```Username to UserID / UserID to Username
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.username_to_userid("leomessi")
mobile.userid_to_username("12345678")
```Like/Unlike Post
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.like(media_id)
mobile.unlike(media_id)
```Fetch Followers/Followings
```python
from ensta import Mobilemobile = Mobile(username, password)
followers = mobile.followers("leomessi")
followings = mobile.followings("leomessi")for user in followers.list:
print(user.full_name)for user in followings.list:
print(user.full_name)# Fetching next chunk
followers = mobile.followers(
"leomessi",
next_cursor=followers.next_cursor
)
```Add Comment to Post
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.comment("Hello", media_id)
```Upload Photo
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.upload_photo(
upload_id=upload_id,
caption="Hello"
)
```Upload Sidecar (Multiple Photos)
```python
from ensta import Mobile
from ensta.structures import SidecarChildmobile = Mobile(username, password)
mobile.upload_sidecar(
children=[
SidecarChild(uploda_id),
SidecarChild(uploda_id),
SidecarChild(uploda_id)
],
caption="Hello"
)
```Fetch Private Information (Yours)
```python
from ensta import Mobilemobile = Mobile(username, password)
account = mobile.private_info()
print(account.email)
print(account.account_type)
print(account.phone_number)
```Update Display Name
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.update_display_name("Lionel Messi")
```Block/Unblock User
```python
from ensta import Mobilemobile = Mobile(username, password)
mobile.block(123456789) # Use UserID
mobile.unblock(123456789) # Use UserID
```Upload Story (Photo)
```python
from ensta import Mobilemobile = Mobile(username, password)
upload_id = mobile.get_upload_id("image.jpg")
mobile.upload_story(upload_id)
```Upload Story (Photo) + Link Sticker
```python
from ensta import Mobile
from ensta.structures import StoryLinkmobile = Mobile(username, password)
upload_id = mobile.get_upload_id("image.jpg")
mobile.upload_story(upload_id, entities=[
StoryLink(title="Google", url="https://google.com")
])
```Send Message (Text)
```python
from ensta import Mobilemobile = Mobile(username, password) # Or use email
direct = mobile.direct()direct.send_text("Hello", thread_id)
```Send Message (Picture)
```python
from ensta import Mobilemobile = Mobile(username, password) # Or use email
direct = mobile.direct()media_id = direct.fb_upload_image("image.jpg")
direct.send_photo(media_id, thread_id)
```Add Biography Link
```python
from ensta import Mobilemobile = Mobile(username, password) # Or use email
link_id = mobile.add_bio_link(
url="https://github.com/diezo",
title="Diezo's GitHub"
)
```Add Multiple Biography Links
```python
from ensta import Mobile
from ensta.structures import BioLinkmobile = Mobile(username, password) # Or use email
link_ids = mobile.add_bio_links([
BioLink(url="https://example.com", title="Link 1"),
BioLink(url="https://example.com", title="Link 2"),
BioLink(url="https://example.com", title="Link 3")
])
```Remove Biography Link
```python
from ensta import Mobilemobile = Mobile(username, password) # Or use email
mobile.remove_bio_link(link_id)
```Remove Multiple Biography Links
```python
from ensta import Mobilemobile = Mobile(username, password) # Or use email
mobile.remove_bio_links([
link_id_1,
link_id_2,
link_id_3
])
```Clear All Biography Links
```python
from ensta import Mobilemobile = Mobile(username, password) # Or use email
mobile.clear_bio_links()
```### Deprecated Features (Web API)
Features still using the **Web API**:Upload Reel
```python
from ensta import Webhost = Web(username, password)
video_id = host.upload_video_for_reel("Video.mp4", thumbnail="Thumbnail.jpg")
host.pub_reel(
video_id,
caption="Enjoying the winter! ⛄"
)
```Fetch Web Profile Data
```python
from ensta import Webhost = Web(username, password)
profile = host.profile("leomessi")print(profile.full_name)
print(profile.biography)
print(profile.follower_count)
```Fetch Someone's Feed
```python
from ensta import Webhost = Web(username, password)
posts = host.posts("leomessi", 100) # Want full list? Set count to '0'for post in posts:
print(post.caption_text)
print(post.like_count)
```Fetch Post's Likers
```python
from ensta import Webhost = Web(username, password)
post_id = host.get_post_id("https://www.instagram.com/p/Czr2yLmroCQ/")
likers = host.likers(post_id)for user in likers.users:
print(user.username)
print(user.profile_picture_url)
```They'll be migrated to the **Mobile API** soon.
## Supported Classes
> [!IMPORTANT]
> The **Web Class** is deprecated and it's features are being migrated to the **Mobile Class**. It'll be removed from Ensta upon completion.
Mobile Class (Authenticated)
Requires login, and has the most features.
```python
from ensta import Mobilemobile = Mobile(username, password)
profile = mobile.profile("leomessi")print(profile.full_name)
print(profile.biography)
print(profile.profile_pic_url)
```
Guest Class (Non-Authenticated)
Doesn't require login, but has limited features.
```python
from ensta import Guestguest = Guest()
profile = guest.profile("leomessi")print(profile.biography)
```
Web Class (Authenticated) (Deprecated)
```python
from ensta import Webhost = Web(username, password)
profile = host.profile("leomessi")print(profile.biography)
```## Discord Community
Ask questions, discuss upcoming features and meet other developers.[
](https://discord.com/invite/pU4knSwmQe)
## Buy Me A Coffee
Support me in the development of this project.[
](https://buymeacoffee.com/sonii)
## Contributors
[](https://github.com/diezo/ensta/graphs/contributors)## Disclaimer
This is a third party library and not associated with Instagram. We're strictly against spam. You are liable for all the actions you take.