https://github.com/dispatchcode/mandelbrot-explorer
A Mandelbrot Fractal explorer written in Rust using SDL2
https://github.com/dispatchcode/mandelbrot-explorer
fractals rust sdl2
Last synced: 3 months ago
JSON representation
A Mandelbrot Fractal explorer written in Rust using SDL2
- Host: GitHub
- URL: https://github.com/dispatchcode/mandelbrot-explorer
- Owner: DispatchCode
- Created: 2022-04-10T00:57:57.000Z (about 3 years ago)
- Default Branch: main
- Last Pushed: 2022-04-23T23:51:40.000Z (about 3 years ago)
- Last Synced: 2024-12-30T02:52:08.857Z (5 months ago)
- Topics: fractals, rust, sdl2
- Language: Rust
- Homepage:
- Size: 150 KB
- Stars: 1
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# Mandelbrot Fractal explorer
Inspired by my own [fraCtal](https://github.com/DispatchCode/fraCtal) project; code & formulas explanations are there too.
Information about the mandelbrot set can also be found on [Wikipedia](https://en.wikipedia.org/wiki/Mandelbrot_set).For better performance build & run with release flag 😎:
```
cargo run --release
```
The code produced by Rust heavily makes use of the MMX set, as you can see down below (`mandelbrot_fractal()` function):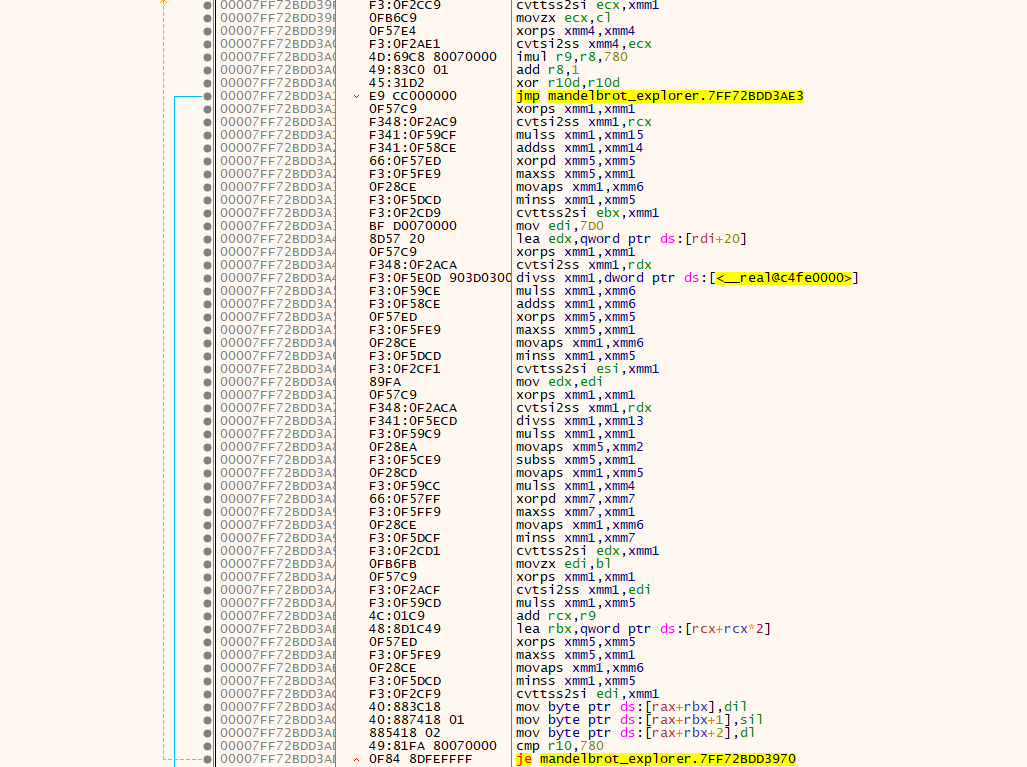
If your CPU support AVX2 or AVX512 - or other - consider using the appropriate `rustc` flag or change the configuration of Cargo to makes use of such sets.
### Features:
✅ Mandelbrot Fractal
✅ SDL2 (you need SDL2 to run the project)
✅ save the generated fractal as image (PNG)
✅ size, zoom, colors can bhe changed
❌ ImGui support (or a GUI menu where options can be changed)
❌ multithreading support### Future plans:
🎯 ImGui support---
## Generated images```Rust
let (val_in, val_out) = (32, 0);
let g = if i >= 32 {
(255.0
- (((i + val_in) as f32 / (max_iterations + val_in) as f32)
* (255 - val_out) as f32)) as u8
} else {
0 as u8
};
```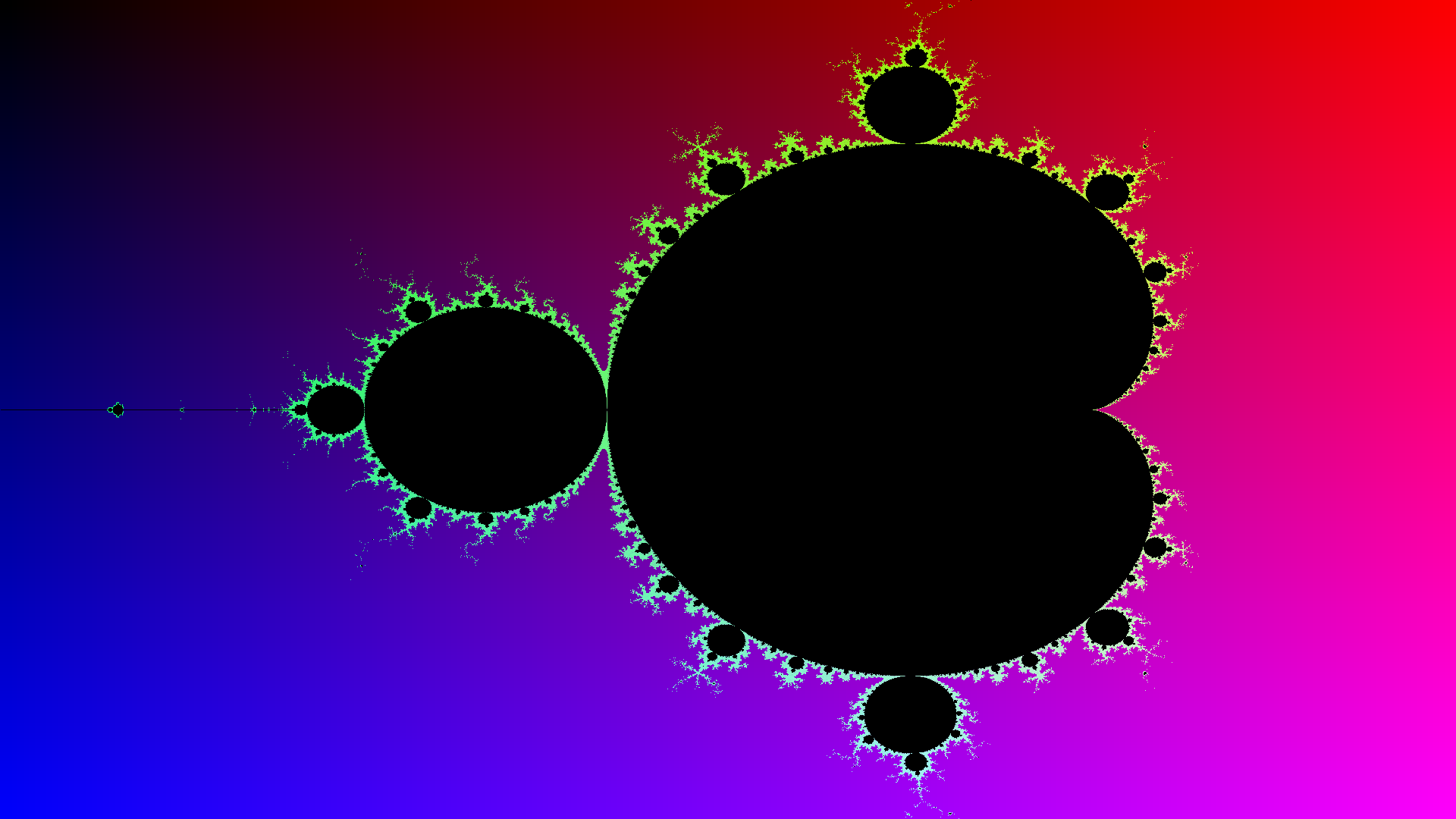
---
```Rust
let attenuation = false; // disable attenuation
// ...
let (val_in, val_out) = (32, 150);
let g = if i >= 32 {
(255.0
- (((i + val_in) as f32 / (max_iterations + val_in) as f32)
* (255 - val_out) as f32)) as u8
} else {
0 as u8
};
```
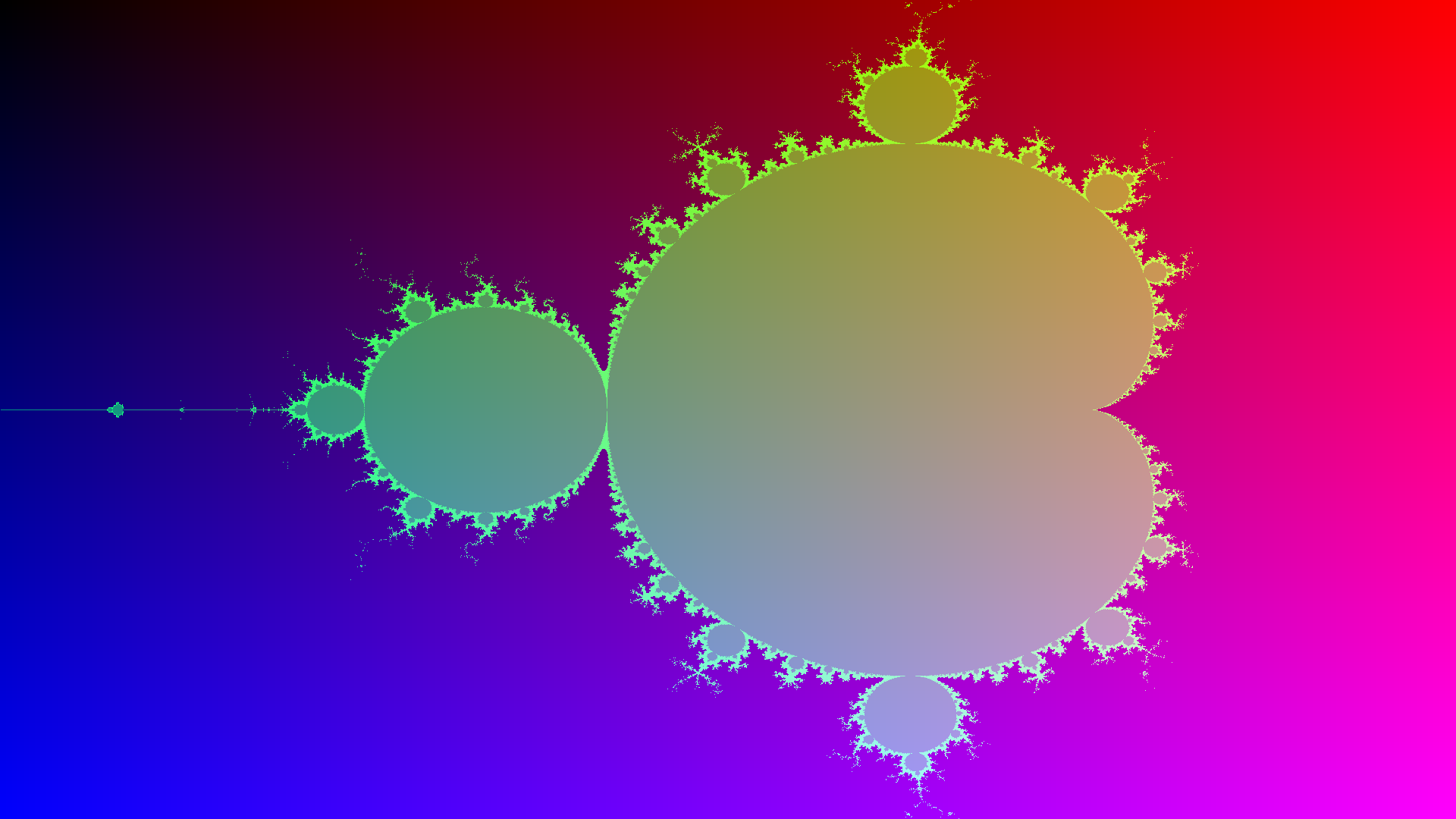---
```Rust
let attenuation = false;// ...
let (val_in, val_out) = (32, 0);
let g = if i >= 32 {
(255.0
- (((i + val_in) as f32 / (max_iterations + val_in) as f32)
* (255 - val_out) as f32)) as u8
} else {
0 as u8
};
```
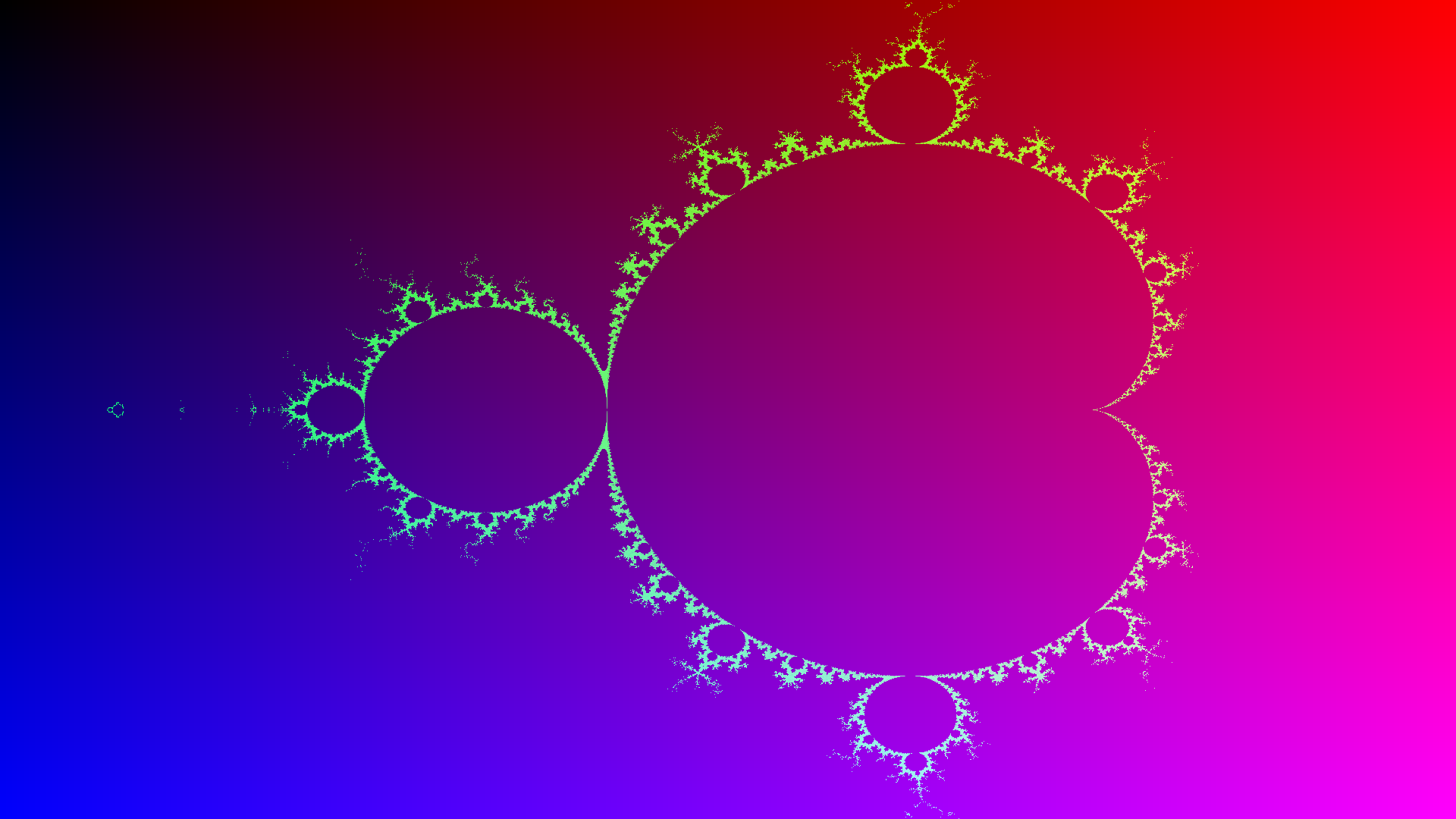---
```Rust
let attenuation = false;// ...
let (val_in, val_out) = (32, 0);
let g = if i >= 0 {
(255.0
- (((i + val_in) as f32 / (max_iterations + val_in) as f32)
* (255 - val_out) as f32)) as u8
} else {
0 as u8
};
```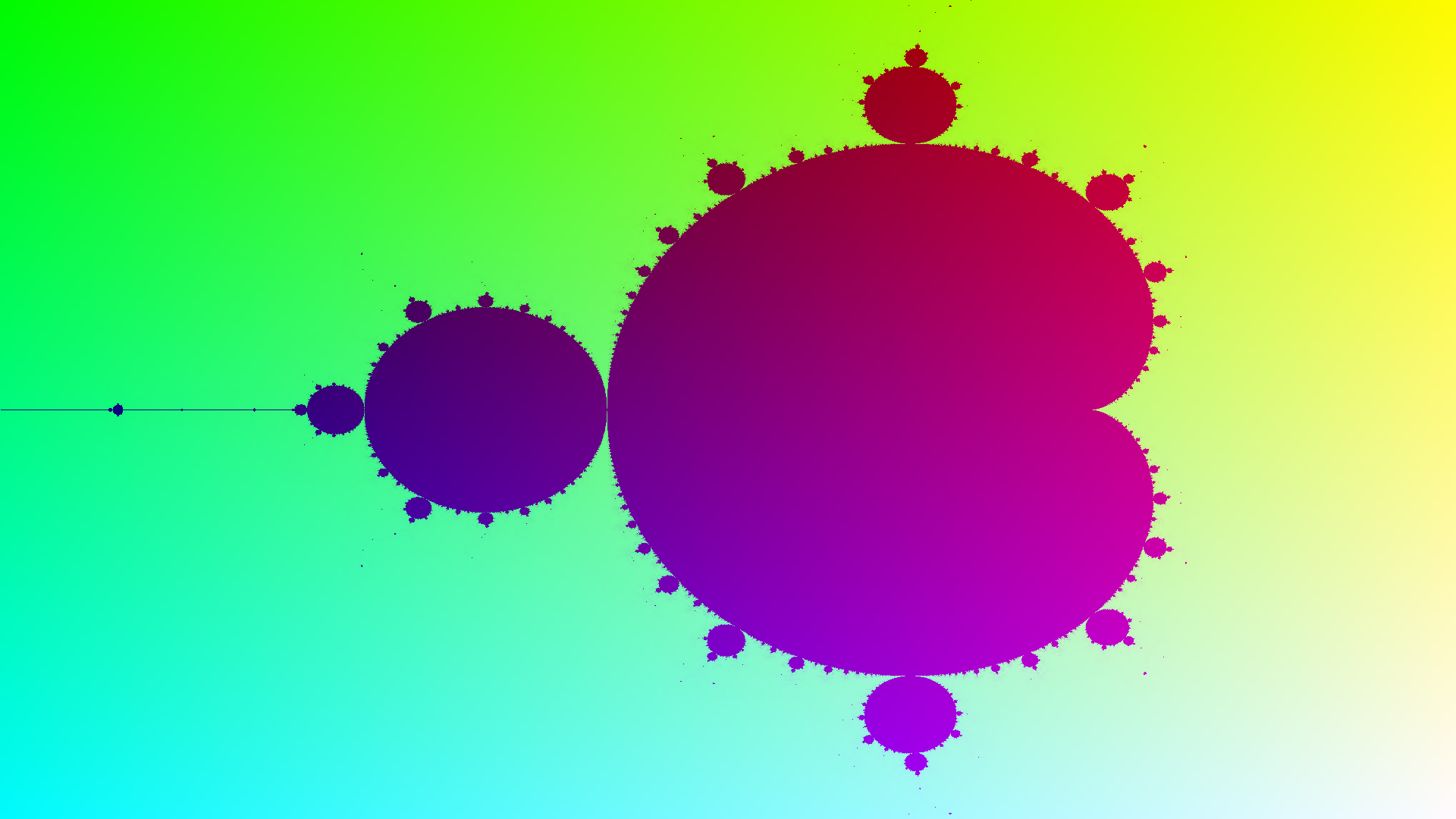
---
```Rust
let attenuation = true;let (blu_a, blu_b) = (255.0, 255.0);
let (red_a, red_b) = (0.0, 255.0);let (val_in, val_out) = (128, 0);
let g = if i >= 32 {
(255.0
- (((i + val_in) as f32 / (max_iterations + val_in) as f32)
* (255 - val_out) as f32)) as u8
} else {
0 as u8
};
```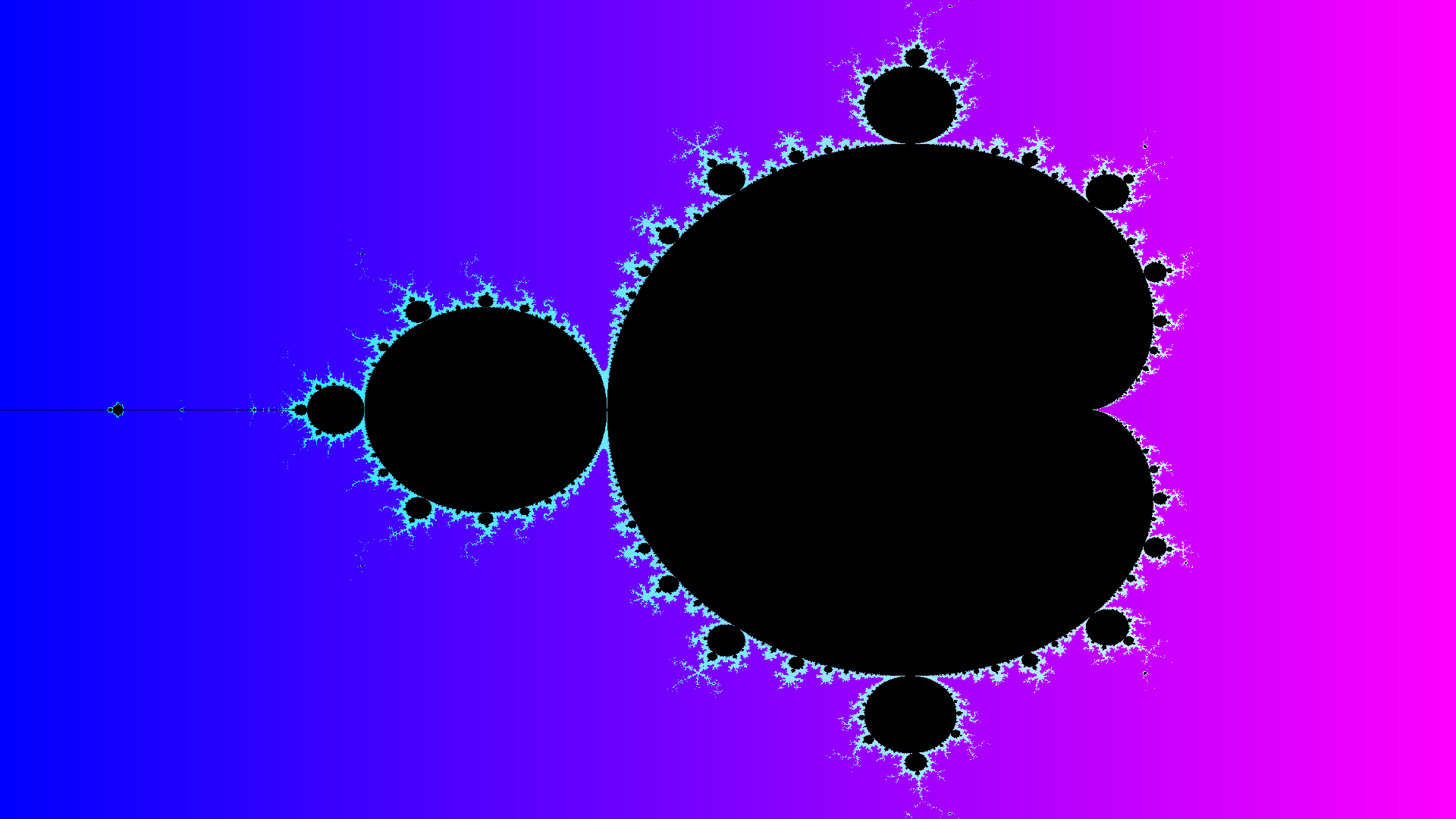
---
```Rust
let attenuation = true;
let (x_min, x_max, y_min, y_max) = (0.40, 0.37, 0.26, 0.21);// The colors around the lake
let (blu_a, blu_b) = (32.0, 255.0);
let (red_a, red_b) = (32.0, 255.0);let (val_in, val_out) = (180,0);
let g = if i >= 128 {
(255.0
- (((i + val_in) as f32 / (max_iterations + val_in) as f32)
* (255 - val_out) as f32)) as u8
} else {
32 as u8
};
```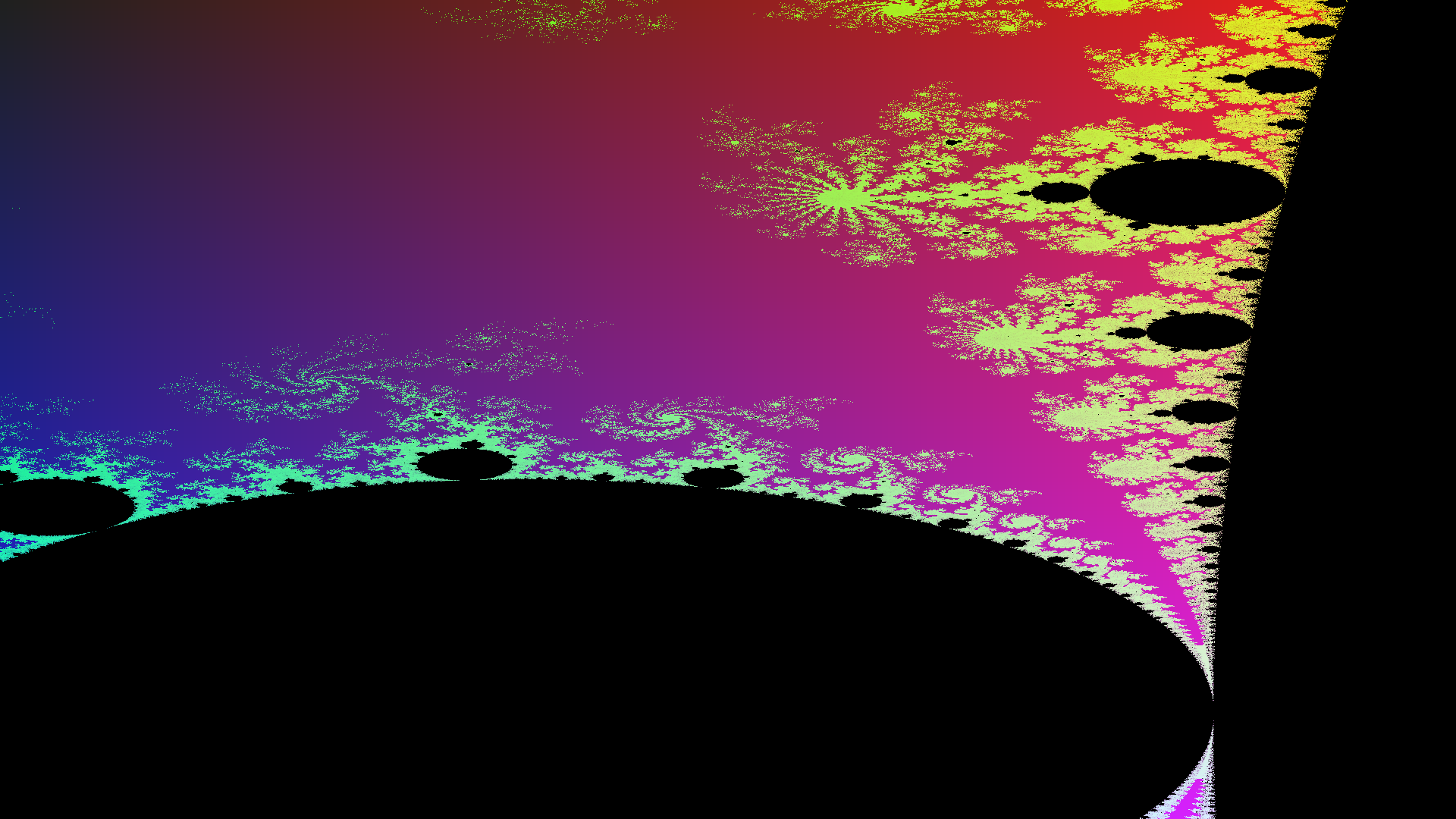