Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/djeada/numerical-methods
Comprehensive collection of numerical methods implemented in Python. It includes solutions to various mathematical problems, detailed explanations of each method, illustrative examples, and comparisons with prominent scientific libraries like Numpy, Scikit-Learn, and SciPy.
https://github.com/djeada/numerical-methods
jupyter-notebook linear-algebra matplotlib numerical-methods numpy python scikit-learn scipy
Last synced: about 4 hours ago
JSON representation
Comprehensive collection of numerical methods implemented in Python. It includes solutions to various mathematical problems, detailed explanations of each method, illustrative examples, and comparisons with prominent scientific libraries like Numpy, Scikit-Learn, and SciPy.
- Host: GitHub
- URL: https://github.com/djeada/numerical-methods
- Owner: djeada
- License: mit
- Created: 2019-03-07T19:57:48.000Z (almost 6 years ago)
- Default Branch: master
- Last Pushed: 2025-01-30T20:33:50.000Z (6 days ago)
- Last Synced: 2025-01-30T21:28:03.955Z (6 days ago)
- Topics: jupyter-notebook, linear-algebra, matplotlib, numerical-methods, numpy, python, scikit-learn, scipy
- Language: Jupyter Notebook
- Homepage: https://adamdjellouli.com/articles/numerical_methods
- Size: 1.99 MB
- Stars: 6
- Watchers: 2
- Forks: 4
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# Numerical Methods
This repository offers a comprehensive collection of numerical methods implemented in Python. It includes solutions to various mathematical problems, detailed explanations of each method, illustrative examples, and comparisons with prominent scientific libraries like Numpy, Scikit-Learn, and SciPy. Whether you're a student eager to delve into numerical methods or a researcher seeking efficient algorithms, this resource is tailored for you. You are encouraged to explore, utilize, and adapt the code according to your needs.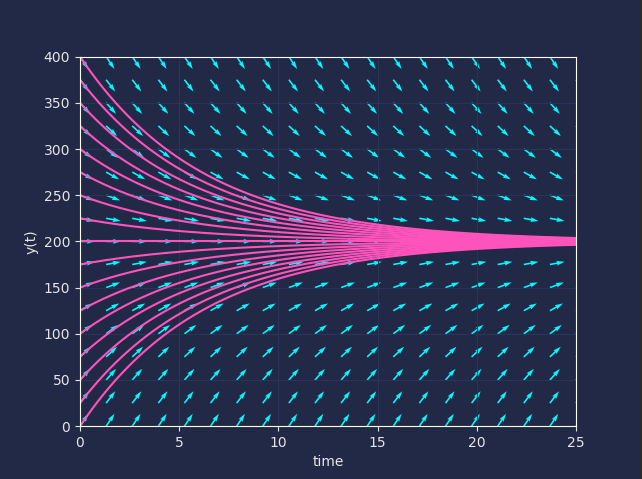
## Requirements
* Python 3.10+
* Whatever library is mentioned in the project's requirements.txt file.## Installation
To run *.py* scripts the recommended approach is to use virtualenv:
$ virtualenv env
$ source env/bin/activate
$ pip install -r requirements.txt
$ python path/to/main.pyFor *.ipynb* notebooks you do not need to install anything locally on your PC. You may run all of the examples on the official website of Jupyter Notebooks using a demo version:
https://jupyter.org/try
To run the notebooks locally, use the following command:
$ jupyter notebook path/to/notebook.ipynb
## Topics
### Root And Extrema Finding
Method | Notes | Implementation | Examples
------ | ----- | -------------- | --------
| Bisection Method ||
|
|
| Secant Method ||
|
|
| Relaxation Method ||
|
|
| Golden Ratio Search ||
|
|
| Newton Raphson ||
|
|
| Gradient Descent ||
|
|
### Systems Of Equations
Method | Notes | Implementation | Examples
------ | ----- | -------------- | --------
| Inverse Matrix ||
|
|
| Gaussian Elimination ||
|
|
| LU Decomposition ||
|
|
| Gauss Seidel Method ||
|
|
| Jacobi Method ||
|
|
### Differentiation
Method | Notes | Implementation | Examples
------ | ----- | -------------- | --------
| Taylor series ||
|
|
| Forward difference ||
|
|
| Backward difference ||
|
|
| Central difference ||
|
|
### Integration
Method | Notes | Implementation | Examples
------ | ----- | -------------- | --------
| Midpoint Rule ||
|
|
| Trapezoidal Rule ||
|
|
| Simpson's Rule ||
|
|
| Monte Carlo Integration ||
|
|
### Matrices
Method | Notes | Implementation | Examples
------ | ----- | -------------- | --------
| Eigenvalues and Eigenvectors ||
|
|
| Power Method ||
|
|
| QR Method ||
|
|
| Eigenvalue Decomposition (EVD) ||
|
|
| Singular Value Decomposition (SVD) ||
|
|
### Regression
Method | Notes | Implementation | Examples
------ | ----- | -------------- | --------
| Linear Interpolation ||
|
|
| Least Squares ||
|
|
| Cubic Spline ||
|
|
| Lagrange Polynomial ||
|
|
| Newton's Polynomial ||
|
|
| Gaussian Interpolation ||
|
|
| Thin Plate Spline Interpolation ||
|
|
### Ordinary Differential Equations
Method | Notes | Implementation | Examples
------ | ----- | -------------- | --------
| Euler's Method ||
|
|
| Heun's Method ||
|
|
| Runge Kutta ||
|
|
| Picard's Method ||
|
|
## References
- [MIT OpenCourseWare: Linear Algebra](https://ocw.mit.edu/courses/mathematics/18-06-linear-algebra-spring-2010/)
- [Wikiversity: Cubic Spline Interpolation](https://en.wikiversity.org/wiki/Cubic_Spline_Interpolation)
- [Statnotes: Statistical Concepts by Dr. Garson](https://faculty.chass.ncsu.edu/garson/PA765/statnote.htm)
- [Numerical Methods Lectures, SDSU](https://jmahaffy.sdsu.edu/courses/s18/math541/Lectures.html)
- [Numerical Analysis: U of A Engineering Courses](https://engcourses-uofa.ca/books/numericalanalysis)
- [Numerical Methods by John Foster, UT Austin](https://johnfoster.pge.utexas.edu/numerical-methods-book)
- [Numerical Methods Course Material, NYU](https://math.nyu.edu/~stadler/num1/material/)
- [Fundamentals of Numerical Computation by Tobin A. Driscoll and Richard J. Braun](https://fncbook.com/)## Contributing
Contributions are welcome! If you'd like to propose a major change, please open an issue first to discuss your ideas.
When contributing, ensure you update relevant tests as needed to maintain the integrity of the project.
## License
This project is licensed under the [MIT License](https://choosealicense.com/licenses/mit/).
## Star History
[](https://star-history.com/#djeada/Numerical-Methods&Date)