Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/dmnsgn/perspective-grid
Two point perspective grid on canvas
https://github.com/dmnsgn/perspective-grid
canvas grid perspective
Last synced: about 2 hours ago
JSON representation
Two point perspective grid on canvas
- Host: GitHub
- URL: https://github.com/dmnsgn/perspective-grid
- Owner: dmnsgn
- License: mit
- Created: 2015-10-20T17:09:38.000Z (about 9 years ago)
- Default Branch: main
- Last Pushed: 2021-11-13T16:01:48.000Z (about 3 years ago)
- Last Synced: 2024-04-27T03:40:47.548Z (7 months ago)
- Topics: canvas, grid, perspective
- Language: JavaScript
- Homepage: http://dmnsgn.github.io/perspective-grid/
- Size: 1.03 MB
- Stars: 6
- Watchers: 4
- Forks: 1
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE.md
Awesome Lists containing this project
README
# perspective-grid
[](https://www.npmjs.com/package/perspective-grid)
[](https://www.npmjs.com/package/perspective-grid)
[](https://bundlephobia.com/package/perspective-grid)
[](https://github.com/dmnsgn/perspective-grid/blob/main/package.json)
[](https://github.com/microsoft/TypeScript)
[](https://conventionalcommits.org)
[](https://github.com/prettier/prettier)
[](https://github.com/eslint/eslint)
[](https://github.com/dmnsgn/perspective-grid/blob/main/LICENSE.md)Two point perspective grid on canvas.
[](https://paypal.me/dmnsgn)
[](https://commerce.coinbase.com/checkout/56cbdf28-e323-48d8-9c98-7019e72c97f3)
[](https://twitter.com/dmnsgn)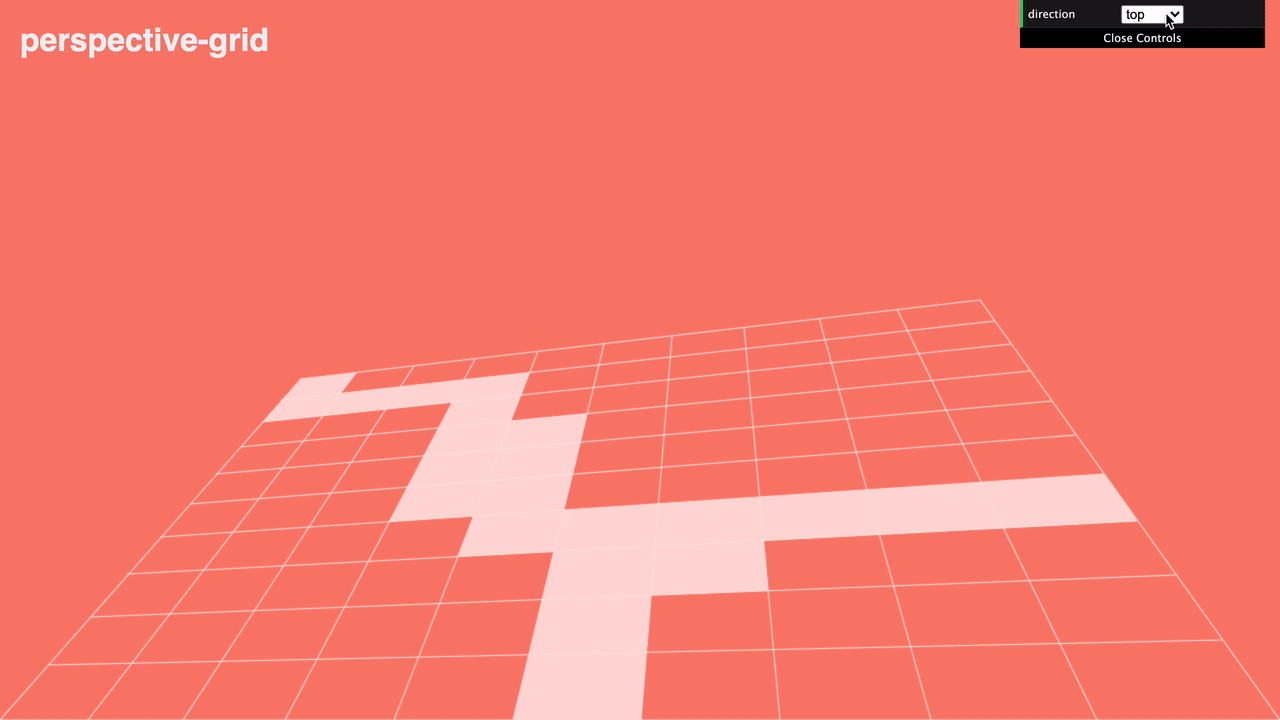
## Installation
```bash
npm install perspective-grid
```## Usage
```js
import { PerspectiveGrid, Point } from "perspective-grid";
import canvasContext from "canvas-context";const { context, canvas } = canvasContext("2d", {
width: window.innerWidth,
height: window.innerHeight,
});// Alternatively pass [rows, columns] for a grid with different rows and column units
const grid = new PerspectiveGrid(10);grid.init(
new Point(300, 380),
new Point(canvas.width - 300, 300),
new Point(canvas.width, canvas.height),
new Point(0, canvas.height)
);
grid.update();// Operations on lines...
context.save();
grid.drawLines(context);
context.restore();context.save();
grid.drawSquares(context);
context.restore();// ...or simply draw
context.save();
grid.draw(context);
context.restore();
```## API
## Modules
## LineEquation
- [LineEquation](#module_LineEquation)
- [LineEquation](#exp_module_LineEquation--LineEquation) ⏏
- [new LineEquation(lineParams, x)](#new_module_LineEquation--LineEquation_new)
- [.intersect(equation)](#module_LineEquation--LineEquation+intersect) ⇒ Point
### LineEquation ⏏
LineEquation defines a line equation or vertical
#### new LineEquation(lineParams, x)
| Param | Type | Description |
| ---------- | ----------------------- | --------------- |
| lineParams | LineParams
| Line parameters |
| x | number
| X position |
#### lineEquation.intersect(equation) ⇒ Point
Get intersection of two line equation
**Kind**: instance method of [LineEquation
](#exp_module_LineEquation--LineEquation)
| Param | Type |
| -------- | ------------------------- |
| equation | LineEquation
|
## LineType
### LineType ⏏
A faked ENUM for referencing line types.
**Kind**: Exported constant
| Param | Type |
| ---------- | ------------------- |
| VERTICAL | number
|
| HORIZONTAL | number
|
## MathHelper
- [MathHelper](#module_MathHelper)
- [.EPSILON](#module_MathHelper.EPSILON) : number
- [.PI](#module_MathHelper.PI) : number
- [.TWO_PI](#module_MathHelper.TWO_PI) : number
- [.getDistance(point1, point2)](#module_MathHelper.getDistance) ⇒ number
- [.getConvergencePoint(m1, c1, m2, c2)](#module_MathHelper.getConvergencePoint) ⇒ Point
- [.getVerticalConvergence(x, m, c)](#module_MathHelper.getVerticalConvergence) ⇒ number
- [.getLineParams(x1, y1, x2, y2)](#module_MathHelper.getLineParams) ⇒ Object
- [.getParallelLine(line, point)](#module_MathHelper.getParallelLine) ⇒ Object
- [.getDistanceToLine(line, point)](#module_MathHelper.getDistanceToLine) ⇒ number
- [.getProjectedPoint(line, point)](#module_MathHelper.getProjectedPoint) ⇒ Point
- [.getMassCenter(p1, p2, p3, p4)](#module_MathHelper.getMassCenter) ⇒ Point
### MathHelper.EPSILON : number
**Kind**: static constant of [MathHelper
](#module_MathHelper)
### MathHelper.PI : number
**Kind**: static constant of [MathHelper
](#module_MathHelper)
### MathHelper.TWO_PI : number
**Kind**: static constant of [MathHelper
](#module_MathHelper)
### MathHelper.getDistance(point1, point2) ⇒ number
Get the distance between two points
**Kind**: static method of [MathHelper
](#module_MathHelper)
**Returns**: number
- Distance between point1 and point2
| Param | Type | Description |
| ------ | ------------------- | ------------ |
| point1 | Object
| First point |
| point2 | Object
| Second point |
### MathHelper.getConvergencePoint(m1, c1, m2, c2) ⇒ Point
Get the convergence point of two line equation
**Kind**: static method of [MathHelper
](#module_MathHelper)
| Param | Type |
| ----- | ------------------- |
| m1 | number
|
| c1 | number
|
| m2 | number
|
| c2 | number
|
### MathHelper.getVerticalConvergence(x, m, c) ⇒ number
Get the vertical convergence point from a X position
**Kind**: static method of [MathHelper
](#module_MathHelper)
| Param | Type |
| ----- | ------------------- |
| x | number
|
| m | number
|
| c | number
|
### MathHelper.getLineParams(x1, y1, x2, y2) ⇒ Object
Return line parameters
**Kind**: static method of [MathHelper
](#module_MathHelper)
| Param | Type |
| ----- | ------------------- |
| x1 | number
|
| y1 | number
|
| x2 | number
|
| y2 | number
|
### MathHelper.getParallelLine(line, point) ⇒ Object
Get parallel line parameters
**Kind**: static method of [MathHelper
](#module_MathHelper)
| Param | Type |
| ----- | ------------------- |
| line | number
|
| point | Point
|
### MathHelper.getDistanceToLine(line, point) ⇒ number
Get the distance to a line parameters
**Kind**: static method of [MathHelper
](#module_MathHelper)
| Param | Type |
| ----- | ------------------- |
| line | Object
|
| point | Point
|
### MathHelper.getProjectedPoint(line, point) ⇒ Point
Get a projected point
**Kind**: static method of [MathHelper
](#module_MathHelper)
| Param | Type |
| ----- | ------------------- |
| line | Object
|
| point | Point
|
### MathHelper.getMassCenter(p1, p2, p3, p4) ⇒ Point
Get the center of four points
**Kind**: static method of [MathHelper
](#module_MathHelper)
| Param | Type |
| ----- | ------------------ |
| p1 | Point
|
| p2 | Point
|
| p3 | Point
|
| p4 | Point
|
## PerspectiveGrid
- [PerspectiveGrid](#module_PerspectiveGrid)
- [PerspectiveGrid](#exp_module_PerspectiveGrid--PerspectiveGrid) ⏏
- [new PerspectiveGrid(units, [squares])](#new_module_PerspectiveGrid--PerspectiveGrid_new)
- [.init(tl, tr, br, bl)](#module_PerspectiveGrid--PerspectiveGrid+init)
- [.draw(context)](#module_PerspectiveGrid--PerspectiveGrid+draw)
- [.update()](#module_PerspectiveGrid--PerspectiveGrid+update)
- [.getQuadAt(column, row)](#module_PerspectiveGrid--PerspectiveGrid+getQuadAt) ⇒ Array.<Point>
- [.getCenterAt(column, row)](#module_PerspectiveGrid--PerspectiveGrid+getCenterAt) ⇒ Point
- [.drawLines(context)](#module_PerspectiveGrid--PerspectiveGrid+drawLines)
- [.drawSquares(context)](#module_PerspectiveGrid--PerspectiveGrid+drawSquares)
- [.drawPoint(context, point, radius, color)](#module_PerspectiveGrid--PerspectiveGrid+drawPoint)
- [.\_getHorizon(vVanishing, hVanishing)](#module_PerspectiveGrid--PerspectiveGrid+_getHorizon) ⇒ Object
### PerspectiveGrid ⏏
Two point perspective grid on canvas.
Note: Does not work correctly when there is only one vanishing point.
#### new PerspectiveGrid(units, [squares])
Creates an instance of PerspectiveGrid.
| Param | Type | Description |
| --------- | -------------------------------------------------------- | ----------------------------------------------------- |
| units | number
\| Array.<number>
| Number of rows and columns (unit or [rows, columns]). |
| [squares] | Array.<Point>
| Highlighted squares in the grid |
#### perspectiveGrid.init(tl, tr, br, bl)
Reset the corners (clockwise starting from top left)
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
| Param | Type | Description |
| ----- | ------------------ | ------------------- |
| tl | Point
| Top left corner |
| tr | Point
| Top right corner |
| br | Point
| Bottom right corner |
| bl | Point
| Bottom left corner |
#### perspectiveGrid.draw(context)
Draw the grid in the instance context
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
| Param | Type | Description |
| ------- | ------------------------------------- | ------------------------------- |
| context | CanvasRenderingContext2D
| The context to draw the grid in |
#### perspectiveGrid.update()
Update grid segments
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
#### perspectiveGrid.getQuadAt(column, row) ⇒ Array.<Point>
Get the four vertices of a point in grid
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
| Param | Type |
| ------ | ------------------- |
| column | number
|
| row | number
|
#### perspectiveGrid.getCenterAt(column, row) ⇒ Point
Get the center point from grid unit to pixel eg. (1, 1) is the first top left point
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
| Param | Type |
| ------ | ------------------- |
| column | number
|
| row | number
|
#### perspectiveGrid.drawLines(context)
Actually draw the lines (vertical and horizontal) in the context
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
| Param | Type | Description |
| ------- | ------------------------------------- | ------------------------------- |
| context | CanvasRenderingContext2D
| The context to draw the grid in |
#### perspectiveGrid.drawSquares(context)
Draw highlighted squares in the grid
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
| Param | Type | Description |
| ------- | ------------------------------------- | ------------------------------- |
| context | CanvasRenderingContext2D
| The context to draw the grid in |
#### perspectiveGrid.drawPoint(context, point, radius, color)
Draw a single point in the grid useful for debug purpose
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
| Param | Type | Description |
| ------- | ------------------------------------- | ------------------------------- |
| context | CanvasRenderingContext2D
| The context to draw the grid in |
| point | Point
| |
| radius | number
| |
| color | string
| |
#### perspectiveGrid.\_getHorizon(vVanishing, hVanishing) ⇒ Object
Get a line parallel to the horizon
**Kind**: instance method of [PerspectiveGrid
](#exp_module_PerspectiveGrid--PerspectiveGrid)
| Param | Type |
| ---------- | ------------------ |
| vVanishing | Point
|
| hVanishing | Point
|
## Point
- [Point](#module_Point)
- [Point](#exp_module_Point--Point) ⏏
- [new Point(x, y)](#new_module_Point--Point_new)
- [.isInList(list)](#module_Point--Point+isInList) ⇒ boolean
### Point ⏏
An object that defines a Point
#### new Point(x, y)
| Param | Type | Description |
| ----- | ------------------- | ------------ |
| x | number
| x coordinate |
| y | number
| y coordinate |
#### point.isInList(list) ⇒ boolean
Check if a point is in a list of points
**Kind**: instance method of [Point
](#exp_module_Point--Point)
| Param | Type | Description |
| ----- | -------------------------------- | --------------- |
| list | Array.<Point>
| Array of Points |
## Segment
- [Segment](#module_Segment)
- [Segment](#exp_module_Segment--Segment) ⏏
- [new Segment(p1, p1)](#new_module_Segment--Segment_new)
- [.intersect(segment)](#module_Segment--Segment+intersect) ⇒ Point
### Segment ⏏
An object with two points that defines a segment
#### new Segment(p1, p1)
| Param | Type | Description |
| ----- | ------------------ | ------------ |
| p1 | Point
| First point |
| p1 | Point
| Second point |
#### segment.intersect(segment) ⇒ Point
Get intersection of two segments
**Kind**: instance method of [Segment
](#exp_module_Segment--Segment)
| Param | Type |
| ------- | -------------------- |
| segment | Segment
|
## License
MIT. See [license file](https://github.com/dmnsgn/perspective-grid/blob/main/LICENSE.md).