Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/dry-python/lambdas
Write short and fully-typed lambdas where you need them.
https://github.com/dry-python/lambdas
composition dry-python fp functional-programming lambda lambdas mypy mypy-plugins mypy-stubs python python3
Last synced: 8 days ago
JSON representation
Write short and fully-typed lambdas where you need them.
- Host: GitHub
- URL: https://github.com/dry-python/lambdas
- Owner: dry-python
- License: bsd-2-clause
- Created: 2019-10-20T12:57:31.000Z (about 5 years ago)
- Default Branch: master
- Last Pushed: 2023-12-11T18:03:10.000Z (11 months ago)
- Last Synced: 2024-10-29T20:18:10.622Z (15 days ago)
- Topics: composition, dry-python, fp, functional-programming, lambda, lambdas, mypy, mypy-plugins, mypy-stubs, python, python3
- Language: Python
- Homepage:
- Size: 633 KB
- Stars: 266
- Watchers: 6
- Forks: 5
- Open Issues: 15
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Contributing: CONTRIBUTING.md
- License: LICENSE
Awesome Lists containing this project
README
[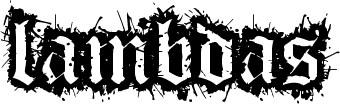](https://github.com/dry-python/lambdas)
-----
[](https://github.com/dry-python/lambdas/actions?query=workflow%3Atest)
[](https://codecov.io/gh/dry-python/lambdas)
[](https://lambdas.readthedocs.io/en/latest/?badge=latest)
[](https://pypi.org/project/lambdas/)
[](https://github.com/wemake-services/wemake-python-styleguide)
[](https://t.me/drypython)-----
Write short and fully-typed `lambda`s where you need them.
## Features
- Allows to write `lambda`s as `_`
- Fully typed with annotations and checked with `mypy`, [PEP561 compatible](https://www.python.org/dev/peps/pep-0561/)
- Has a bunch of helpers for better composition
- Easy to start: has lots of docs, tests, and tutorials## Installation
```bash
pip install lambdas
```You also need to configure `mypy` correctly and install our plugin:
```ini
# In setup.cfg or mypy.ini:
[mypy]
plugins =
lambdas.contrib.mypy.lambdas_plugin
```We recommend to use the same `mypy` settings [we use](https://github.com/wemake-services/wemake-python-styleguide/blob/master/styles/mypy.toml).
## Examples
Imagine that you need to sort an array of dictionaries like so:
```python
>>> scores = [
... {'name': 'Nikita', 'score': 2},
... {'name': 'Oleg', 'score': 1},
... {'name': 'Pavel', 'score': 4},
... ]>>> print(sorted(scores, key=lambda item: item['score']))
[{'name': 'Oleg', 'score': 1}, {'name': 'Nikita', 'score': 2}, {'name': 'Pavel', 'score': 4}]
```And it works perfectly fine.
Except, that you have to do a lot of typing for such a simple operation.That's where `lambdas` helper steps in:
```python
>>> from lambdas import _>>> scores = [
... {'name': 'Nikita', 'score': 2},
... {'name': 'Oleg', 'score': 1},
... {'name': 'Pavel', 'score': 4},
... ]>>> print(sorted(scores, key=_['score']))
[{'name': 'Oleg', 'score': 1}, {'name': 'Nikita', 'score': 2}, {'name': 'Pavel', 'score': 4}]
```It might really save you a lot of effort,
when you use a lot of `lambda` functions.
Like when using [`returns`](https://github.com/dry-python/returns) library.We can easily create math expressions:
```python
>>> from lambdas import _>>> math_expression = _ * 2 + 1
>>> print(math_expression(10))
21
>>> complex_math_expression = 50 / (_ ** 2) * 2
>>> print(complex_math_expression(5))
100.0
```Work in progress:
- `_.method()` is not supported yet for the same reason
- `TypedDict`s are not tested with `__getitem__`
- `__getitem__` does not work with list and tuples (collections), only dicts (mappings)For now you will have to use regular `lamdba`s in these cases.