https://github.com/dsazz/your_splash
A Flutter implementation of splash screen. Supports Android and IOS.
https://github.com/dsazz/your_splash
dart flutter splash-screen splashscreen
Last synced: 2 months ago
JSON representation
A Flutter implementation of splash screen. Supports Android and IOS.
- Host: GitHub
- URL: https://github.com/dsazz/your_splash
- Owner: Dsazz
- License: mit
- Created: 2020-08-29T15:56:54.000Z (almost 5 years ago)
- Default Branch: master
- Last Pushed: 2023-03-29T11:25:43.000Z (about 2 years ago)
- Last Synced: 2025-03-23T07:12:20.764Z (3 months ago)
- Topics: dart, flutter, splash-screen, splashscreen
- Language: Dart
- Homepage:
- Size: 90.8 KB
- Stars: 7
- Watchers: 1
- Forks: 2
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- License: LICENSE
Awesome Lists containing this project
README
# your_splash
[](https://pub.dev/packages/your_splash)
[](https://github.com/Dsazz/your_splash/blob/master/LICENSE)

[](https://codecov.io/gh/Dsazz/your_splash)
[](https://saythanks.io/to/dsazztazz%40gmail.com)A Flutter implementation of splash screen. Supports Android and IOS.
## Features
* Supports splash screen with the Future callback
* Supports splash screen with timer## Getting Started
To use this library, add `your_splash` as a [dependency](https://flutter.io/platform-plugins/) in your `pubspec.yaml` file:
```yaml
dependencies:
...
your_splash:
```In your library add the following import:
```dart
import 'package:your_splash/your_splash.dart';
```### Constructors
This package comes with 2 kinds of splash screen actions:
* `FuturedSplashScreen`
* `TimedSplashScreen`You can create a splash screen in two different ways:
* by calling `FuturedSplashScreen` or `TimedSplashScreen` constructor and passing required arguments.
* by calling `SplashScreen.futured` or `SplashScreen.timed` constructor respectively.A `FuturedSplashScreen` requires next arguments:
| Parameter | Description |
| ------------- | ------------- |
| `future` | This is your Future callback for delay |
| `route` | This is a dynamic argument. Can be `String` name of route or `PageRoute` instance |
| `body` | This is a body of your splash screen |A `TimedSplashScreen` requires next arguments:
| Parameter | Description |
| ------------- | ------------- |
| `seconds` | This is a duration in seconds for how long your splash screen will be displayed |
| `route` | This is a dynamic argument. Can be `String` name of route or `PageRoute` instance |
| `body` | This is a body of your splash screen |### Examples
Quick start example:
```dart
import 'package:flutter/material.dart';
import 'package:your_splash/your_splash.dart';void main() {
runApp(MyApp());
}class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: SplashScreen.timed(
seconds: 3,
route: MaterialPageRoute(builder: (_) => Home()),
body: Scaffold(
body: InkWell(
child: Container(
decoration: const BoxDecoration(
image: DecorationImage(
fit: BoxFit.cover,
image: NetworkImage('https://bit.ly/3hD5Tj8'),
),
),
),
),
),
),
);
}
}class Home extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Home screen!"),
),
body: Center(
child: Text(
"Welcome!",
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 40.0),
),
),
);
}
}```
How can I animate transition between the splash screen and navigation page?
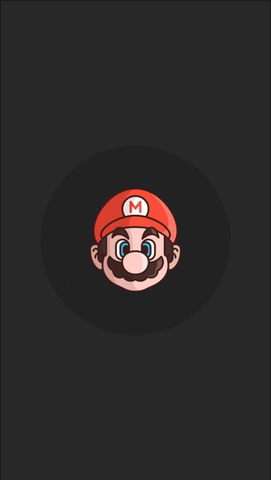
```
route: PageRouteBuilder(
pageBuilder: (context, animation, secondaryAnimation) => Home(),
transitionDuration: Duration(seconds: 1),
transitionsBuilder: (_, animation, secAnim, child) {
var tween = Tween(begin: 0.0, end: 1.0).chain(
CurveTween(curve: Curves.easeInOutCirc),
);
return FadeTransition(
opacity: animation.drive(tween), child: child);
},
```You can see all examples [here](https://github.com/Dsazz/your_splash/tree/master/example)