Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/emilwijayasekara/leetcode-13-roman-to-integer
LeetCode Problem 12. Integer to Roman - The task is to sum the values of these symbols according to the rules of Roman numeral representation, with the added complexity of subtraction when a smaller numeral precedes a larger one. The goal is to return the integer value equivalent to the given Roman numeral string.
https://github.com/emilwijayasekara/leetcode-13-roman-to-integer
java leetcode leetcode-java leetcode-solutions
Last synced: 2 days ago
JSON representation
LeetCode Problem 12. Integer to Roman - The task is to sum the values of these symbols according to the rules of Roman numeral representation, with the added complexity of subtraction when a smaller numeral precedes a larger one. The goal is to return the integer value equivalent to the given Roman numeral string.
- Host: GitHub
- URL: https://github.com/emilwijayasekara/leetcode-13-roman-to-integer
- Owner: EmilWijayasekara
- Created: 2023-12-17T05:59:19.000Z (11 months ago)
- Default Branch: main
- Last Pushed: 2023-12-17T07:33:26.000Z (11 months ago)
- Last Synced: 2023-12-18T07:19:35.888Z (11 months ago)
- Topics: java, leetcode, leetcode-java, leetcode-solutions
- Language: Java
- Homepage:
- Size: 5.86 KB
- Stars: 1
- Watchers: 1
- Forks: 0
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# LeetCode Practice (Day 3)
## Achievements
[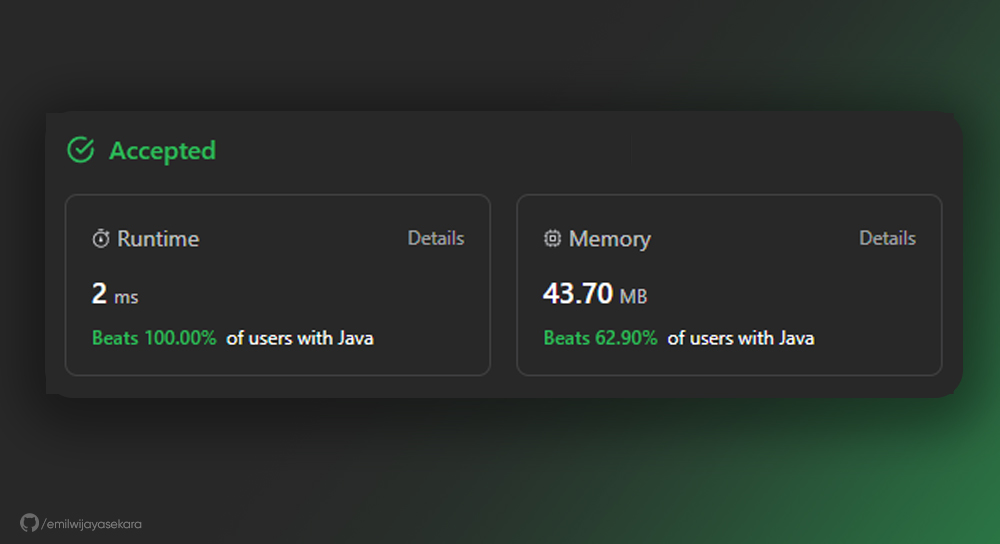](https://postimg.cc/jCJ22JPr)## About the problem
- *Problem Number* : 13
- *Problem Name* : [Roman to Integer](https://leetcode.com/problems/roman-to-integer/)
- *Problem difficulty* : Easy (60.01%) 🟢
- *Category* : Algorithms
- *Programming language used* - Java## Problem
Roman numerals are represented by seven different symbols: `I`, `V`, `X`, `L`, `C`, `D` and `M`.
```
Symbol Value
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
```For example, `2` is written as `II` in Roman numeral, just two ones added together. `12` is written as `XII`, which is simply `X + II`. The number `27` is written as `XXVII`, which is `XX + V + II`.
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not `IIII`. Instead, the number four is written as `IV`. Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as `IX`. There are six instances where subtraction is used:
- `I` can be placed before `V` (5) and `X` (10) to make 4 and 9.
- `X` can be placed before `L` (50) and `C` (100) to make 40 and 90.
- `C` can be placed before `D` (500) and `M` (1000) to make 400 and 900.Given a roman numeral, convert it to an integer.
**Example 1:**
```
Input: s = "III"
Output: 3
Explanation: III = 3.
```**Example 2:**
```
Input: s = "LVIII"
Output: 58
Explanation: L = 50, V= 5, III = 3.
```**Example 3:**
```
Input: s = "MCMXCIV"
Output: 1994
Explanation: M = 1000, CM = 900, XC = 90 and IV = 4.
```**Constraints:**
- `1 <= s.length <= 15`
- `s` contains only the characters `('I', 'V', 'X', 'L', 'C', 'D', 'M')`.
- It is **guaranteed** that `s` is a valid roman numeral in the range `[1, 3999]`.## Approach Explanation
I've approached this problem by iterating through the Roman numeral string from `right to left`.
For each numeral, I use a switch statement to map it to its corresponding numerical value, or this can be done using a HashMap also.I used three variables which are:
```cpp
int correspondingNaturalNumber = 0;
int answer = 0;
int previous =0;
```
`previous` variable used to get the previous number currently iterating through.
`answer` is final return value that i added all roman number values.
`correspondingNaturalNumber` in switch statement i mapped roman number to natural numbers.After that using if else statement, I compare the current numeral's value (`correspondingNaturalNumber`) with the value of the previous numeral (`previous`). If the current numeral is smaller than the previous one, its value is subtracted from the result; otherwise, it is added.
After processing each numeral, I update the `previous` variable to store the current numeral's value. This ensures that the correct value is considered in the next iteration.
### If you have suggestions for improvement or would like to contribute to this solution, feel free to create a pull request. 🙌😇