Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/felipeoriani/fynance
Fynance is a handy wrapper to get stock market quotes written in C# available at NuGet package manager for .NET applications.
https://github.com/felipeoriani/fynance
csharp dotnet dotnet-core finance stock-data stock-market stock-quotes yahoo yahoo-finance yahoo-finance-api
Last synced: 3 months ago
JSON representation
Fynance is a handy wrapper to get stock market quotes written in C# available at NuGet package manager for .NET applications.
- Host: GitHub
- URL: https://github.com/felipeoriani/fynance
- Owner: felipeoriani
- License: apache-2.0
- Created: 2020-05-28T03:02:47.000Z (over 4 years ago)
- Default Branch: main
- Last Pushed: 2024-06-04T17:55:54.000Z (8 months ago)
- Last Synced: 2024-11-16T05:04:10.077Z (3 months ago)
- Topics: csharp, dotnet, dotnet-core, finance, stock-data, stock-market, stock-quotes, yahoo, yahoo-finance, yahoo-finance-api
- Language: C#
- Homepage:
- Size: 102 KB
- Stars: 25
- Watchers: 5
- Forks: 9
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
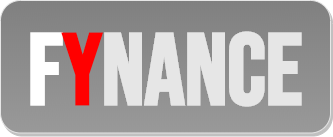
[](https://img.shields.io/github/actions/workflow/status/felipeoriani/Fynance/.github/workflows/pipeline.yml?branch=main)
[](https://www.nuget.org/packages/Fynance)
[](https://www.nuget.org/packages/Fynance)
[](https://github.com/felipeoriani/fynance/stargazers)
[](https://github.com/felipeoriani/fynance/issues)
[](LICENSE)
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=BRLZR87XSBQT8&source=url)Fynance is a handy wrapper to get stock market quotes written in .Net Standard. It is currently support the Yahoo Finance but it can be extended to other APIs.
## Installation - Nuget Package
This is available on [nuget package](https://www.nuget.org/packages/Fynance).
```
Install-Package Fynance
```To update the package to the last version use the following nuget statement:
```
Update-Package Fynance
```## Fynance Examples
What data you can get with `Fynance`:
- Get Security Info
- Get Quotes
- Get Events
- Get Dividends
- Get Splits#### General Usage
First you have to add the `Fynance` namespace to make the types available on your code:
```c#
using Fynance;
```The *Fynance* implements a fluent interface to read data from a stock market api. Given it, a instance of `Ticker` is a representation of a security on the stock market. Then you can make an instance of `Ticker` and use all the methods to configure what you want to have until call the `Get` method. There is a `async` version of this method called `GetAsync`. The following code is a sample of use:
```c#
var result = await Ticker.Build()
.SetSymbol("MSFT")
.SetPeriod(Period.OneMonth)
.SetInterval(Interval.OneDay)
.GetAsync();
```The `Build` method instance the `Ticker` object and all these `Set` methods configure what information you want to get from the available APIs.
To the the *events* as we call *dividends* or *splits* you can use `SetDividends` and `SetEvents` to define it:
```c#
var result = await Ticker.Build()
.SetSymbol("MSFT")
.SetPeriod(Period.OneMonth)
.SetInterval(Interval.OneDay)
.SetDividends(true)
.SetEvents(true)
.GetAsync();
```Alternatively, you can use the method `.SetEvents(true)` and it will set `Dividends` and `Splits`.
When you call the `Get` methods, you get a instance of `FyResult`. It contains all the data following the methods configured over the `Ticker`. The `FyResult` can contain all the information from the *Security*, the *OLHC* history, dividends and splits.
##### Results
There are many information you can read from the security, see some samples available:
```c#
var currenty = result.Currency;
var symbol = result.Symbol;
var symbol = result.ExchangeName;
```
Reading all the *OLHC* history:```c#
foreach (var item in result.Quotes)
{
var period = item.Period; // DateTime
var open = item.Open; // Decimal
var low = item.Low; // Decimal
var high = item.High; // Decimal
var close = item.Close; // Decimal
var close = item.Close; // Decimal
var adjClose = item.AdjClose; // Decimal
var volume = item.Volume; // Decimal
}
```Reading the dividends:
```c#
foreach (var item in result.Dividends)
{
var date = item.Date; // DateTime: Payment date
var value = item.value; // Decimal: Payment value
}
```Reading the splits:
```c#
foreach (var item in result.Splits)
{
var date = item.Date; // DateTime: Event Date
var numerator = item.Numberator; // Decimal: Numerator of splits
var denominator = item.Denominator; // Decimal: Denominator of split
}
```### Issue Reports
If you find any issue, please report them using the [GitHub issue tracker](https://github.com/felipeoriani/Fynance/issues). Would be great if you provide a sample of code or a repository with a sample project.
### Contributions
Contributions are welcome, feel free to fork the repository and send a pull request with your changes to contribute with the package.
### Licenses
This software is distributed under the terms of the *Apache License 2.0*. Please, read the [LICENSE](https://github.com/felipeoriani/Fynance/blob/master/LICENSE) file available on this repository.
### Credits
This project is a collab of [@FelipeOriani](https://github.com/felipeoriani/) and [@EduVencovsky](https://github.com/eduvencovsky/).
Many thanks for the [Newtonsoft.Json](https://www.newtonsoft.com/json) packaged used as a dependency of this project.
If you wanna colaborate with this project or it was useful to you, make a donate:
[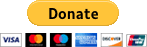](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=BRLZR87XSBQT8&source=url)