https://github.com/filipchalupa/pwa-install-handler
Handling PWA installation prompt made easier.
https://github.com/filipchalupa/pwa-install-handler
addtohomescreen installation npm pwa typescript
Last synced: 3 months ago
JSON representation
Handling PWA installation prompt made easier.
- Host: GitHub
- URL: https://github.com/filipchalupa/pwa-install-handler
- Owner: FilipChalupa
- Created: 2019-02-11T12:56:44.000Z (over 6 years ago)
- Default Branch: main
- Last Pushed: 2024-12-10T13:11:47.000Z (7 months ago)
- Last Synced: 2025-03-24T16:55:25.524Z (4 months ago)
- Topics: addtohomescreen, installation, npm, pwa, typescript
- Language: TypeScript
- Homepage: https://www.npmjs.com/package/pwa-install-handler
- Size: 440 KB
- Stars: 11
- Watchers: 2
- Forks: 0
- Open Issues: 5
-
Metadata Files:
- Readme: README.md
Awesome Lists containing this project
README
# PWA install handler
Handling PWA installation prompt made easier. [MDN docs](https://developer.mozilla.org/en-US/docs/Web/API/Window/onbeforeinstallprompt)
## Installation
```sh
npm install pwa-install-handler
```## Usage
### HTML
```html
Install
```### JavaScript
```javascript
import { pwaInstallHandler } from 'pwa-install-handler'const $button = document.querySelector('#installButton')
pwaInstallHandler.addListener((canInstall) => {
$button.style.display = canInstall ? 'inline-block' : 'none'
})$button.addEventListener('click', () => {
pwaInstallHandler.install()
})
```### React
For more information see [react-use-pwa-install](https://www.npmjs.com/package/react-use-pwa-install).
### Screencast
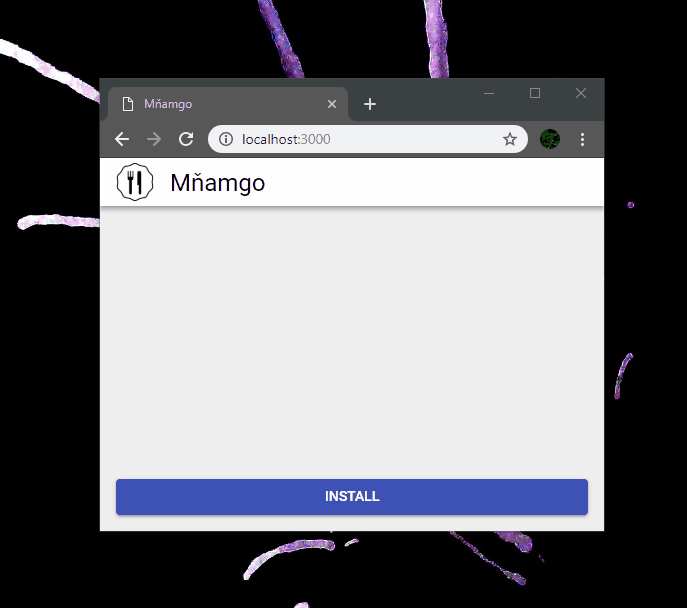
### Demo
Check [website](https://filipchalupa.cz/pwa-install-handler/) or [code](https://github.com/FilipChalupa/pwa-install-handler/tree/main/demo).
## Methods
```typescript
pwaInstallHandler.install: () => Promise
``````typescript
pwaInstallHandler.addListener: (
callback: (canInstall: boolean, install?: () => Promise) => void,
) => void
``````typescript
pwaInstallHandler.removeListener: (
callback: (canInstall: boolean, install?: () => Promise) => void,
) => void
``````typescript
pwaInstallHandler.canInstall: () => boolean
``````typescript
pwaInstallHandler.getEvent: () => BeforeInstallPromptEvent | null
```## Notes
You PWA must meet some requirements to be installable. Without that the `canInstall` will always be `false`. The requirements are browser specific. You can read more about it [here (MDN)](https://developer.mozilla.org/en-US/docs/Web/Progressive_web_apps/Installable_PWAs#Requirements) and [here (web.dev)](https://web.dev/install-criteria/).
Some browsers don't support custom install button. These will have `canInstall` always set to `false` too. For more information check [BeforeInstallPromptEvent support](https://caniuse.com/#feat=mdn-api_beforeinstallpromptevent).