Ecosyste.ms: Awesome
An open API service indexing awesome lists of open source software.
https://github.com/fortana-co/react-dropzone-uploader
React file dropzone and uploader
https://github.com/fortana-co/react-dropzone-uploader
dropzone file-upload input react s3 typescript uploader
Last synced: 6 days ago
JSON representation
React file dropzone and uploader
- Host: GitHub
- URL: https://github.com/fortana-co/react-dropzone-uploader
- Owner: fortana-co
- License: mit
- Created: 2018-10-21T00:34:51.000Z (over 6 years ago)
- Default Branch: master
- Last Pushed: 2024-04-01T14:04:19.000Z (11 months ago)
- Last Synced: 2025-02-08T06:06:56.962Z (13 days ago)
- Topics: dropzone, file-upload, input, react, s3, typescript, uploader
- Language: TypeScript
- Homepage: https://react-dropzone-uploader.js.org/
- Size: 6.09 MB
- Stars: 449
- Watchers: 12
- Forks: 186
- Open Issues: 156
-
Metadata Files:
- Readme: README.md
- License: LICENSE
Awesome Lists containing this project
README
# React Dropzone Uploader
[](https://www.npmjs.com/package/react-dropzone-uploader)
[](https://www.npmjs.com/package/react-dropzone-uploader)React Dropzone Uploader is a customizable file dropzone and uploader for React.
## Features
- Detailed file metadata and previews, especially for image, video and audio files
- Upload status and progress, upload cancellation and restart
- Easily set auth headers and additional upload fields ([see S3 examples](https://react-dropzone-uploader.js.org/docs/s3))
- Customize styles using CSS or JS
- Take full control of rendering with component injection props
- Take control of upload lifecycle
- Modular design; use as standalone dropzone, file input, or file uploader
- Cross-browser support, mobile friendly, including direct uploads from camera
- Lightweight and fast
- Excellent TypeScript definitions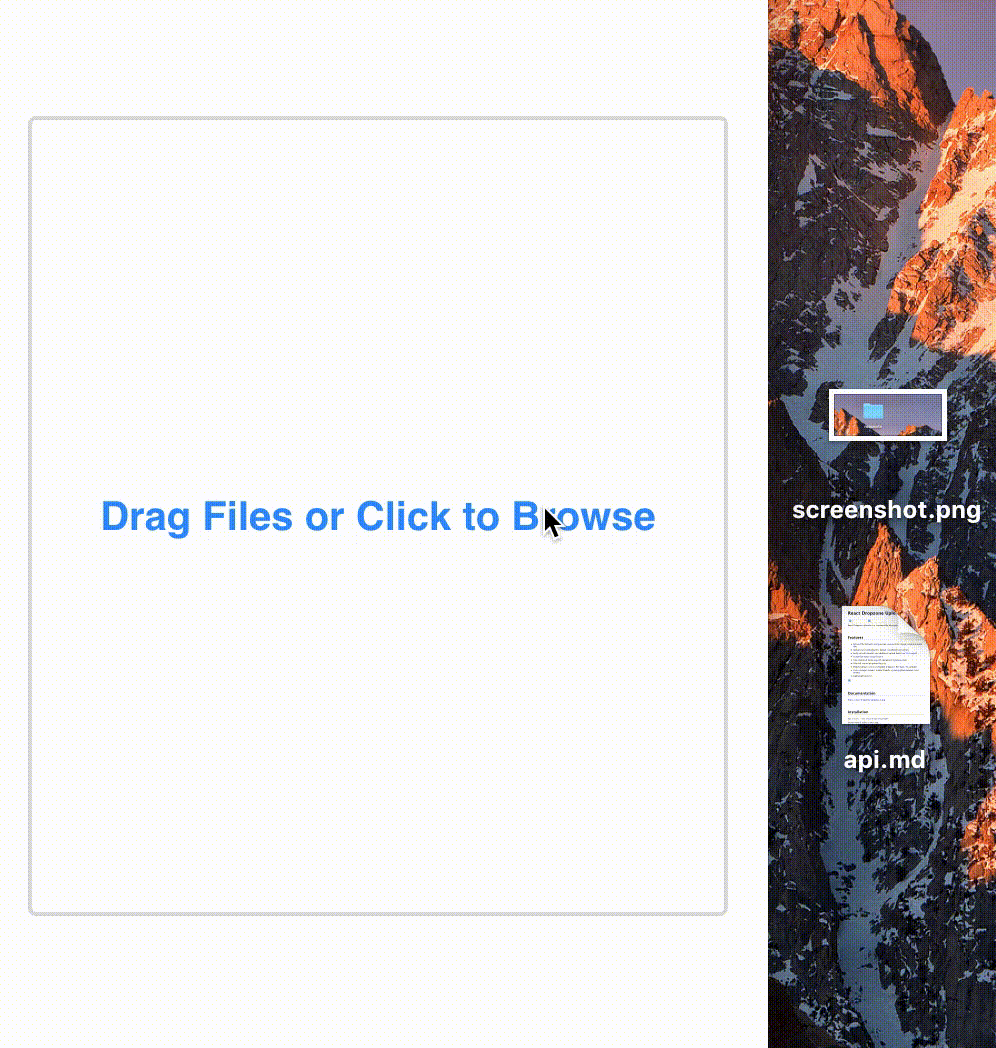
## Documentation
## Installation
`npm install --save react-dropzone-uploader`Import default styles in your app.
~~~js
import 'react-dropzone-uploader/dist/styles.css'
~~~## Quick Start
RDU handles common use cases with almost no config. The following code gives you a dropzone and clickable file input that accepts image, audio and video files. It uploads files to `https://httpbin.org/post`, and renders a button to submit files that are done uploading. [Check out a live demo](https://react-dropzone-uploader.js.org/docs/quick-start).~~~js
import 'react-dropzone-uploader/dist/styles.css'
import Dropzone from 'react-dropzone-uploader'const MyUploader = () => {
// specify upload params and url for your files
const getUploadParams = ({ meta }) => { return { url: 'https://httpbin.org/post' } }
// called every time a file's `status` changes
const handleChangeStatus = ({ meta, file }, status) => { console.log(status, meta, file) }
// receives array of files that are done uploading when submit button is clicked
const handleSubmit = (files) => { console.log(files.map(f => f.meta)) }return (
)
}
~~~## Examples
See more live examples here: .## Props
Check out [the full table of RDU's props](https://react-dropzone-uploader.js.org/docs/props).## Browser Support
| Chrome | Firefox | Edge | Safari | IE | iOS Safari | Chrome for Android |
| --- | --- | --- | --- | --- | --- | --- |
| ✔ | ✔ | ✔ | 10+, 9\* | 11\* | ✔ | ✔ |\* requires `Promise` polyfill, e.g. [@babel/polyfill](https://babeljs.io/docs/en/babel-polyfill)
## UMD Build
This library is available as an ES Module at .If you want to include it in your page, you need to include the dependencies and CSS as well.
~~~html
~~~
## Contributing
There are a number of places RDU could be improved; [see here](https://github.com/fortana-co/react-dropzone-uploader/labels/help%20wanted).For example, RDU has solid core functionality, but has a minimalist look and feel. It would be more beginner-friendly with a larger variety of built-in components.
### Shout Outs
Thanks to @nchen63 for helping with [TypeScript defs](https://github.com/fortana-co/react-dropzone-uploader/blob/master/src/Dropzone.d.ts)!### Running Dev
Clone the project, install dependencies, and run the dev server.~~~sh
git clone git://github.com/fortana-co/react-dropzone-uploader.git
cd react-dropzone-uploader
yarn
npm run dev
~~~This runs code in `examples/src/index.js`, which has many examples that use `Dropzone`. The library source code is in the `/src` directory.
## Thanks
Thanks to `react-dropzone`, `react-select`, and `redux-form` for inspiration.