https://github.com/freesuraj/bulldog
Simple yet powerful json parser for iOS and Mac
https://github.com/freesuraj/bulldog
json json-parser swift swiftyjson
Last synced: about 1 month ago
JSON representation
Simple yet powerful json parser for iOS and Mac
- Host: GitHub
- URL: https://github.com/freesuraj/bulldog
- Owner: freesuraj
- License: mit
- Created: 2016-11-19T01:26:37.000Z (over 8 years ago)
- Default Branch: master
- Last Pushed: 2019-04-03T06:20:20.000Z (over 6 years ago)
- Last Synced: 2025-04-07T14:17:33.874Z (3 months ago)
- Topics: json, json-parser, swift, swiftyjson
- Language: Swift
- Size: 182 KB
- Stars: 5
- Watchers: 1
- Forks: 1
- Open Issues: 0
-
Metadata Files:
- Readme: README.md
- Changelog: CHANGELOG.md
- Contributing: CONTRIBUTING.md
- License: LICENSE
Awesome Lists containing this project
README
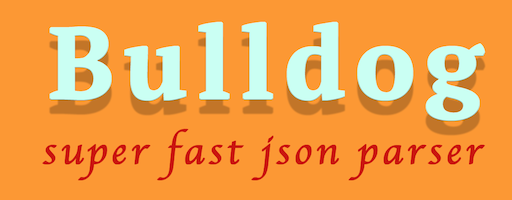
[](https://travis-ci.org/freesuraj/Bulldog)
[](https://coveralls.io/github/freesuraj/Bulldog?branch=master)
[](https://codecov.io/gh/freesuraj/Bulldog)
[](https://codeclimate.com/github/freesuraj/Bulldog)
[](https://codebeat.co/projects/github-com-freesuraj-bulldog)
[](https://codeclimate.com/github/freesuraj/Bulldog)
[](http://cocoadocs.org/docsets/Bulldog/)
[](https://github.com/Carthage/Carthage)
[](http://cocoadocs.org/docsets/Bulldog)
[](http://twitter.com/iosCook)
[](https://github.com/freesuraj/bulldog)
[](https://github.com/freesuraj/bulldog)# Bulldog
Bulldog is a super-fast json parser that will keep attacking until it gets the value you desire, or you give up. Just like a bulldog.## Why Bulldog?
- ✅ Super-light
- ✅ Easily extendible
- ✅ Can easily traverse through path of a json object to get the final value while ignoring the type of intermediate objects
- ✅ Utilises the apple's JSONSerialization underneath
- ✅ Well tested with 100% test coverage## Example Usage
Let's suppose we want to parse the following JSON object
### response.json
```
{
"id":123,
"first_name":"Conor",
"last_name":"McGregor",
"age":28,
"height": 171,
"weight":70.5,
"fights":[
{
"id":"UFC 205",
"opponent":"Eddie Alvarez",
"venue":"New York",
"win":true
},
{
"id":"UFC 202",
"opponent":"Nate Diaz",
"venue":"Las Vegas",
"win":true
},
{
"id":"UFC 196",
"opponent":"Nate Diaz",
"venue":"Las Vegas",
"win":false
}
]
}
```### parser.swift
Now to parse the above json file,
```swift
let json: Any = ... // Json from network
let bulldog = Bulldog(json: json)
let name = bulldog.string("first_name") + " " + bulldog.string("last_name") // Conor McGregorlet height = bulldog.int("height") // 171
let weight = bulldog.double("weight") // 70.5// Get first opponent of his fights
let firstOpponent = bulldog.string("fights", 0, "opponent") // Eddie Alvarez
// Get fights array
let fights = bulldog.array("fights") // Returns array of fights// Return the first fight dictionary
let firstFight = bulldog.dictionary("fights", 0) // Returns first fight dictionary// Check if first fight was a win
let isWin = Bulldog(json: fights[0]).boolean("win") // Returns true```
## Installation
### Carthage
Bulldog is available through [Carthage](https://github.com/Carthage/Carthage). To install
it, simply add the following line to your Cartfile:```ruby
git "freesuraj/Bulldog"
```### Cocoapods
Add the following line to your Podfile:
```ruby
pod 'Bulldog'
```
### Manual
Perhaps the easiest way to use is to just copy the file [Bulldog.swift](https://github.com/freesuraj/Bulldog/blob/master/Source/Bulldog.swift) and put it in your project. That's it !!## Example
Please check the [BulldogTests.swift](https://github.com/freesuraj/Bulldog/blob/master/Tests/BulldogTests.swift) file for all possilbe use cases
## About
[](https://github.com/freesuraj)
If you found this little tool useful, I'd love to hear about it. You can also follow me on Twitter at [@iosCook](https://twitter.com/ioscook)
## License
Bulldog is available under the MIT license. See the LICENSE file for more info.